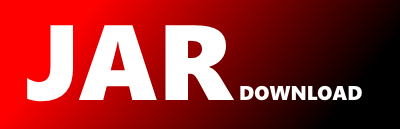
com.freedomotic.reactions.Command Maven / Gradle / Ivy
/**
*
* Copyright (c) 2009-2014 Freedomotic team http://freedomotic.com
*
* This file is part of Freedomotic
*
* This Program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 2, or (at your option) any later version.
*
* This Program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* Freedomotic; see the file COPYING. If not, see
* .
*/
//Copyright 2009 Enrico Nicoletti
//eMail: [email protected]
//
//This file is part of EventEngine.
//
//EventEngine is free software; you can redistribute it and/or modify
//it under the terms of the GNU General Public License as published by
//the Free Software Foundation; either version 2 of the License, or
//any later version.
//
//EventEngine is distributed in the hope that it will be useful,
//but WITHOUT ANY WARRANTY; without even the implied warranty of
//MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
//GNU General Public License for more details.
//
//You should have received a copy of the GNU General Public License
//along with EventEngine; if not, write to the Free Software
//Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
package com.freedomotic.reactions;
import com.freedomotic.model.ds.Config;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map.Entry;
import java.util.UUID;
import java.util.logging.Logger;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlTransient;
/**
*
* @author enrico
*/
@XmlRootElement
public final class Command implements Serializable, Cloneable {
private static final long serialVersionUID = -7287958816826580426L;
private static final Logger LOG = Logger.getLogger(Command.class.getName());
public static final String PROPERTY_BEHAVIOR = "behavior";
public static final String PROPERTY_OBJECT_CLASS = "object.class";
public static final String PROPERTY_OBJECT_ADDRESS = "object.address";
public static final String PROPERTY_OBJECT_NAME = "object.name";
public static final String PROPERTY_OBJECT_PROTOCOL = "object.protocol";
public static final String PROPERTY_OBJECT_INCLUDETAGS = "object.includetags";
public static final String PROPERTY_OBJECT_EXCLUDETAGS = "object.excludetags";
public static final String PROPERTY_OBJECT_ENVIRONMENT = "object.environment";
public static final String PROPERTY_OBJECT_ZONE = "object.zone";
public static final String PROPERTY_OBJECT = "object";
private String name;
private String receiver;
private String uuid;
private int delay;
private int timeout;
private String description;
private String stopIf;
private HashSet tags = new HashSet();
//by default a command is userLevel, this means that can be used in reactions.
//Hardware level commands cannot be used in reactions but only linked to an object action
private boolean hardwareLevel;
private boolean editable;
private boolean executed;
@XmlElement
private Config properties = new Config();
/**
*
*/
public Command() {
this.uuid = UUID.randomUUID().toString();
if (isHardwareLevel()) { //an hardware level command
setEditable(false); //it has not to me stored in root/data folder
}
}
/**
*
* @return
*/
public HashSet getTags() {
if (tags == null) {
tags = new HashSet();
tags.addAll(Arrays.asList(getName().toLowerCase().split(" ")));
}
return tags;
}
/**
*
* @param tags
*/
public void setTags(HashSet tags) {
this.tags = tags;
}
/**
*
* @return
*/
public String getDescription() {
if (description == null) {
description = getName();
}
return description;
}
/**
*
* @param description
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* @return
*/
public String getUuid() {
if (uuid == null || uuid.trim().equals("")){
uuid= UUID.randomUUID().toString();
}
return uuid;
}
/**
*
* @param uuid
*/
public void setUUID(String uuid) {
this.uuid = uuid;
}
/**
*
* @return
*/
public boolean isHardwareLevel() {
return hardwareLevel;
}
/**
*
* @param hardwareLevel
*/
public void setHardwareLevel(boolean hardwareLevel) {
this.hardwareLevel = hardwareLevel;
}
/**
*
* @return
*/
public boolean isEditable() {
return editable;
}
/**
*
* @param persistence
*/
public void setEditable(boolean persistence) {
this.editable = persistence;
}
/**
*
* @return
*/
public String getStopIf() {
return stopIf;
}
/**
*
* @param continueIf
*/
public void setStopIf(String continueIf) {
this.stopIf = continueIf;
}
/**
*
* @return
*/
@XmlTransient
public String getBehavior() {
if (properties.getProperty("behavior") != null) {
return properties.getProperty("behavior");
} else {
LOG.warning("Undefined property 'behavior' in command '" + this.getName() + "'");
return "undefined-behavior";
}
}
/**
*
* @param key
* @return
*/
public String getProperty(String key) {
return properties.getProperty(key);
}
/**
*
* @param key
* @param value
*/
public void setProperty(String key, String value) {
if (!key.isEmpty()) {
properties.setProperty(key, value);
}
}
/**
*
* @return
*/
public Config getProperties() {
return properties;
}
/**
* Creates an oredred list reading the command properties writed in format
* "parameter[AN_INT_FROM_0_TO_999]" other properties format are ignored
* (not added to the returned List) The indexs must be contiguous
* (1,2,3,...) for example:
*
*
parameter[0] = foo parameter[1] = bar
* parameter[3] = asd object = Light 1 another-param =
* myValue
*
* The returned ArrayList is [0]->foo [1]->bar
* because the index = 2 is missing.
*
* @return an ordered ArrayList of command parameter values
*/
@XmlTransient
public ArrayList getParametersAsList() {
ArrayList output = new ArrayList();
//99 is the max num of elements in list
for (int i = 0; i < 99; i++) {
String value = null;
value = properties.getProperties().getProperty("parameter[" + i + "]");
if (value != null) {
//add to array
output.add(value);
} else {
//if the elements are not contiguous return
return output;
}
}
return output;
}
/**
*
* @return
*/
public int getDelay() {
if (delay > 0) {
return delay;
} else {
return 0;
}
}
/**
*
* @param delay
*/
public void setDelay(int delay) {
this.delay = delay;
}
/**
*
* @return
*/
public String getName() {
return name;
}
/**
*
* @param name
*/
public void setName(String name) {
this.name = name.trim();
}
/**
*
* @return
*/
public String getReceiver() {
return receiver;
}
/**
*
* @param receiver
*/
public void setReceiver(String receiver) {
this.receiver = receiver;
}
/**
*
* @param value
*/
public void setExecuted(boolean value) {
executed = value;
}
/**
*
* @return
*/
public boolean isExecuted() {
return executed;
}
/**
* Two commands are considered equals if they have the same name
* @param obj
* @return
*/
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final Command other = (Command) obj;
if ((this.name == null) ? (other.name != null) : (!this.name.equals(other.name))) {
return false;
}
return true;
}
/**
*
* @return
*/
@Override
public int hashCode() {
int hash = 5;
hash = (53 * hash) + ((this.name != null) ? this.name.hashCode() : 0);
return hash;
}
/**
*
* @return
* @throws CloneNotSupportedException
*/
@Override
public Command clone()
throws CloneNotSupportedException {
super.clone();
Command clonedCmd = new Command();
clonedCmd.setName(getName());
clonedCmd.setDescription(getDescription());
clonedCmd.setReceiver(getReceiver());
clonedCmd.setDelay(getDelay());
clonedCmd.setReplyTimeout(getReplyTimeout());
clonedCmd.setExecuted(executed);
clonedCmd.setHardwareLevel(hardwareLevel);
Iterator> it = getProperties().entrySet().iterator();
while (it.hasNext()) {
Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy