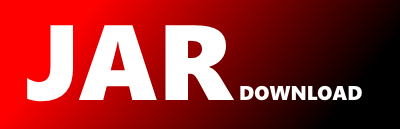
com.freedomotic.util.LogFormatter Maven / Gradle / Ivy
/**
*
* Copyright (c) 2009-2014 Freedomotic team http://freedomotic.com
*
* This file is part of Freedomotic
*
* This Program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 2, or (at your option) any later version.
*
* This Program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* Freedomotic; see the file COPYING. If not, see
* .
*/
package com.freedomotic.util;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.logging.Formatter;
import java.util.logging.Handler;
import java.util.logging.Level;
import java.util.logging.LogRecord;
import java.util.logging.Logger;
/**
*
* @author Enrico
*/
public class LogFormatter
extends Formatter {
SimpleDateFormat date = new SimpleDateFormat("HH:mm ss,S");
/**
*
*/
public LogFormatter() {
super();
}
/**
*
* @param record
* @return
*/
@Override
public String format(LogRecord record) {
return ("" + date.format(record.getMillis()) + " "
+ getShortClassName(record.getSourceClassName()) + " "
+ formatTextToHTML(formatMessage(record)) + " \n");
}
private String getColor(int level) {
String htmlColor = "#EAEAE1";
if (level == Level.SEVERE.intValue()) {
htmlColor = "#FFDDDD";
} else if (level == Level.WARNING.intValue()) {
htmlColor = "#FFFFDD";
} else if (level == Level.INFO.intValue()) {
htmlColor = "#DDFFDD";
}
return htmlColor;
}
private String getShortClassName(String name) {
return name.substring(name.lastIndexOf('.') + 1,
name.length());
}
private static String convertSpaces(String input) {
return input.replace("\n", "
");
}
/**
*
* @param input
* @return
*/
public static String formatTextToHTML(String input) {
if (input.startsWith("---- ") && (input.endsWith(" ----"))) {
//it's a title
input = input.replace("----", "").trim();
input = "
" + input + "
";
}
if (input.startsWith("--- ") && (input.endsWith(" ---"))) {
//it's a title
input = input.replace("---", "").trim();
input = "
" + input + "
";
}
if (input.startsWith("-- ") && (input.endsWith(" --"))) {
//it's a title
input = input.replace("--", "").trim();
input = "
" + input + "
";
}
input = input.replace("{{; ", "- "); //to overcome format error
input = input.replace("{{", "
- ");
input = input.replace("; ", "
- ");
input = input.replace("}}", "
");
return convertSpaces(input);
}
/**
*
* @param h
* @return
*/
@Override
public String getHead(Handler h) {
return ("\n " + "\n" + "Freedomotic Developers Log - " + new Date().toString() + "
"
+ "Press F5 to update the page while Freedomotic is running
"
+ "Here is the logger of Freedomotic. It is mainly usefull for developers. We are currently in beta so it is enabled by default."
+ "You can get a more user/configurator perspective on the project website at http://www.freedomotic.com/."
+ "If you don't know where to start take a look at the getting started tutorial
"
+ "Scroll this page till the end to read the latest log records. Information records have green background, warnings and exceptions are highlighted with red background.
"
+ "If the pop-up of this logger annoys you you can disable setting the parameter 'KEY_LOGGER_POPUP = false' in "
+ Info.PATHS.PATH_CONFIG_FOLDER + "/config.xml
"
+ "This file can still be opened from " + Info.PATHS.PATH_WORKDIR
+ "/log/freedomlog.html
." + "\n"
+ "\n"
+ "" + " Time " + " Log Message " + " \n");
}
/**
*
* @param h
* @return
*/
@Override
public String getTail(Handler h) {
return ("
\n\n\n");
}
private static final Logger LOG = Logger.getLogger(LogFormatter.class.getName());
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy