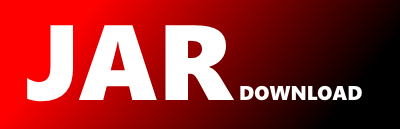
com.frostwire.jlibtorrent.swig.file_storage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jlibtorrent Show documentation
Show all versions of jlibtorrent Show documentation
A swig Java interface for libtorrent by the makers of FrostWire.
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (http://www.swig.org).
* Version 3.0.10
*
* Do not make changes to this file unless you know what you are doing--modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package com.frostwire.jlibtorrent.swig;
public class file_storage {
private transient long swigCPtr;
protected transient boolean swigCMemOwn;
protected file_storage(long cPtr, boolean cMemoryOwn) {
swigCMemOwn = cMemoryOwn;
swigCPtr = cPtr;
}
protected static long getCPtr(file_storage obj) {
return (obj == null) ? 0 : obj.swigCPtr;
}
protected void finalize() {
delete();
}
public synchronized void delete() {
if (swigCPtr != 0) {
if (swigCMemOwn) {
swigCMemOwn = false;
libtorrent_jni.delete_file_storage(swigCPtr);
}
swigCPtr = 0;
}
}
public file_storage() {
this(libtorrent_jni.new_file_storage__SWIG_0(), true);
}
public file_storage(file_storage arg0) {
this(libtorrent_jni.new_file_storage__SWIG_1(file_storage.getCPtr(arg0), arg0), true);
}
public boolean is_valid() {
return libtorrent_jni.file_storage_is_valid(swigCPtr, this);
}
public void reserve(int num_files) {
libtorrent_jni.file_storage_reserve(swigCPtr, this, num_files);
}
public void add_file_borrow(String filename, int filename_len, String path, long file_size, long file_flags, String filehash, long mtime, string_view symlink_path) {
libtorrent_jni.file_storage_add_file_borrow__SWIG_0(swigCPtr, this, filename, filename_len, path, file_size, file_flags, filehash, mtime, string_view.getCPtr(symlink_path), symlink_path);
}
public void add_file_borrow(String filename, int filename_len, String path, long file_size, long file_flags, String filehash, long mtime) {
libtorrent_jni.file_storage_add_file_borrow__SWIG_1(swigCPtr, this, filename, filename_len, path, file_size, file_flags, filehash, mtime);
}
public void add_file_borrow(String filename, int filename_len, String path, long file_size, long file_flags, String filehash) {
libtorrent_jni.file_storage_add_file_borrow__SWIG_2(swigCPtr, this, filename, filename_len, path, file_size, file_flags, filehash);
}
public void add_file_borrow(String filename, int filename_len, String path, long file_size, long file_flags) {
libtorrent_jni.file_storage_add_file_borrow__SWIG_3(swigCPtr, this, filename, filename_len, path, file_size, file_flags);
}
public void add_file_borrow(String filename, int filename_len, String path, long file_size) {
libtorrent_jni.file_storage_add_file_borrow__SWIG_4(swigCPtr, this, filename, filename_len, path, file_size);
}
public void add_file(String path, long file_size, int file_flags, int mtime, string_view symlink_path) {
libtorrent_jni.file_storage_add_file__SWIG_0(swigCPtr, this, path, file_size, file_flags, mtime, string_view.getCPtr(symlink_path), symlink_path);
}
public void add_file(String path, long file_size, int file_flags, int mtime) {
libtorrent_jni.file_storage_add_file__SWIG_1(swigCPtr, this, path, file_size, file_flags, mtime);
}
public void add_file(String path, long file_size, int file_flags) {
libtorrent_jni.file_storage_add_file__SWIG_2(swigCPtr, this, path, file_size, file_flags);
}
public void add_file(String path, long file_size) {
libtorrent_jni.file_storage_add_file__SWIG_3(swigCPtr, this, path, file_size);
}
public void rename_file(int index, String new_filename) {
libtorrent_jni.file_storage_rename_file(swigCPtr, this, index, new_filename);
}
public file_slice_vector map_block(int piece, long offset, int size) {
return new file_slice_vector(libtorrent_jni.file_storage_map_block(swigCPtr, this, piece, offset, size), true);
}
public peer_request map_file(int file, long offset, int size) {
return new peer_request(libtorrent_jni.file_storage_map_file(swigCPtr, this, file, offset, size), true);
}
public int num_files() {
return libtorrent_jni.file_storage_num_files(swigCPtr, this);
}
public long total_size() {
return libtorrent_jni.file_storage_total_size(swigCPtr, this);
}
public void set_num_pieces(int n) {
libtorrent_jni.file_storage_set_num_pieces(swigCPtr, this, n);
}
public int num_pieces() {
return libtorrent_jni.file_storage_num_pieces(swigCPtr, this);
}
public void set_piece_length(int l) {
libtorrent_jni.file_storage_set_piece_length(swigCPtr, this, l);
}
public int piece_length() {
return libtorrent_jni.file_storage_piece_length(swigCPtr, this);
}
public int piece_size(int index) {
return libtorrent_jni.file_storage_piece_size(swigCPtr, this, index);
}
public void set_name(String n) {
libtorrent_jni.file_storage_set_name(swigCPtr, this, n);
}
public String name() {
return libtorrent_jni.file_storage_name(swigCPtr, this);
}
public void swap(file_storage ti) {
libtorrent_jni.file_storage_swap(swigCPtr, this, file_storage.getCPtr(ti), ti);
}
public void unload() {
libtorrent_jni.file_storage_unload(swigCPtr, this);
}
public boolean is_loaded() {
return libtorrent_jni.file_storage_is_loaded(swigCPtr, this);
}
public void optimize(int pad_file_limit, int alignment, boolean tail_padding) {
libtorrent_jni.file_storage_optimize__SWIG_0(swigCPtr, this, pad_file_limit, alignment, tail_padding);
}
public void optimize(int pad_file_limit, int alignment) {
libtorrent_jni.file_storage_optimize__SWIG_1(swigCPtr, this, pad_file_limit, alignment);
}
public void optimize(int pad_file_limit) {
libtorrent_jni.file_storage_optimize__SWIG_2(swigCPtr, this, pad_file_limit);
}
public void optimize() {
libtorrent_jni.file_storage_optimize__SWIG_3(swigCPtr, this);
}
public sha1_hash hash(int index) {
return new sha1_hash(libtorrent_jni.file_storage_hash(swigCPtr, this, index), true);
}
public String symlink(int index) {
return libtorrent_jni.file_storage_symlink(swigCPtr, this, index);
}
public int mtime(int index) {
return libtorrent_jni.file_storage_mtime(swigCPtr, this, index);
}
public String file_path(int index, String save_path) {
return libtorrent_jni.file_storage_file_path__SWIG_0(swigCPtr, this, index, save_path);
}
public String file_path(int index) {
return libtorrent_jni.file_storage_file_path__SWIG_1(swigCPtr, this, index);
}
public string_view file_name(int index) {
return new string_view(libtorrent_jni.file_storage_file_name(swigCPtr, this, index), true);
}
public long file_size(int index) {
return libtorrent_jni.file_storage_file_size(swigCPtr, this, index);
}
public boolean pad_file_at(int index) {
return libtorrent_jni.file_storage_pad_file_at(swigCPtr, this, index);
}
public long file_offset(int index) {
return libtorrent_jni.file_storage_file_offset(swigCPtr, this, index);
}
public string_vector paths() {
return new string_vector(libtorrent_jni.file_storage_paths(swigCPtr, this), false);
}
public int file_flags(int index) {
return libtorrent_jni.file_storage_file_flags(swigCPtr, this, index);
}
public boolean file_absolute_path(int index) {
return libtorrent_jni.file_storage_file_absolute_path(swigCPtr, this, index);
}
public int file_index_at_offset(long offset) {
return libtorrent_jni.file_storage_file_index_at_offset(swigCPtr, this, offset);
}
public final static class flags_t {
public final static file_storage.flags_t pad_file = new file_storage.flags_t("pad_file", libtorrent_jni.file_storage_pad_file_get());
public final static file_storage.flags_t attribute_hidden = new file_storage.flags_t("attribute_hidden", libtorrent_jni.file_storage_attribute_hidden_get());
public final static file_storage.flags_t attribute_executable = new file_storage.flags_t("attribute_executable", libtorrent_jni.file_storage_attribute_executable_get());
public final static file_storage.flags_t attribute_symlink = new file_storage.flags_t("attribute_symlink", libtorrent_jni.file_storage_attribute_symlink_get());
public final int swigValue() {
return swigValue;
}
public String toString() {
return swigName;
}
public static flags_t swigToEnum(int swigValue) {
if (swigValue < swigValues.length && swigValue >= 0 && swigValues[swigValue].swigValue == swigValue)
return swigValues[swigValue];
for (int i = 0; i < swigValues.length; i++)
if (swigValues[i].swigValue == swigValue)
return swigValues[i];
throw new IllegalArgumentException("No enum " + flags_t.class + " with value " + swigValue);
}
private flags_t(String swigName) {
this.swigName = swigName;
this.swigValue = swigNext++;
}
private flags_t(String swigName, int swigValue) {
this.swigName = swigName;
this.swigValue = swigValue;
swigNext = swigValue+1;
}
private flags_t(String swigName, flags_t swigEnum) {
this.swigName = swigName;
this.swigValue = swigEnum.swigValue;
swigNext = this.swigValue+1;
}
private static flags_t[] swigValues = { pad_file, attribute_hidden, attribute_executable, attribute_symlink };
private static int swigNext = 0;
private final int swigValue;
private final String swigName;
}
public final static class file_flags_t {
public final static file_storage.file_flags_t flag_pad_file = new file_storage.file_flags_t("flag_pad_file", libtorrent_jni.file_storage_flag_pad_file_get());
public final static file_storage.file_flags_t flag_hidden = new file_storage.file_flags_t("flag_hidden", libtorrent_jni.file_storage_flag_hidden_get());
public final static file_storage.file_flags_t flag_executable = new file_storage.file_flags_t("flag_executable", libtorrent_jni.file_storage_flag_executable_get());
public final static file_storage.file_flags_t flag_symlink = new file_storage.file_flags_t("flag_symlink", libtorrent_jni.file_storage_flag_symlink_get());
public final int swigValue() {
return swigValue;
}
public String toString() {
return swigName;
}
public static file_flags_t swigToEnum(int swigValue) {
if (swigValue < swigValues.length && swigValue >= 0 && swigValues[swigValue].swigValue == swigValue)
return swigValues[swigValue];
for (int i = 0; i < swigValues.length; i++)
if (swigValues[i].swigValue == swigValue)
return swigValues[i];
throw new IllegalArgumentException("No enum " + file_flags_t.class + " with value " + swigValue);
}
private file_flags_t(String swigName) {
this.swigName = swigName;
this.swigValue = swigNext++;
}
private file_flags_t(String swigName, int swigValue) {
this.swigName = swigName;
this.swigValue = swigValue;
swigNext = swigValue+1;
}
private file_flags_t(String swigName, file_flags_t swigEnum) {
this.swigName = swigName;
this.swigValue = swigEnum.swigValue;
swigNext = this.swigValue+1;
}
private static file_flags_t[] swigValues = { flag_pad_file, flag_hidden, flag_executable, flag_symlink };
private static int swigNext = 0;
private final int swigValue;
private final String swigName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy