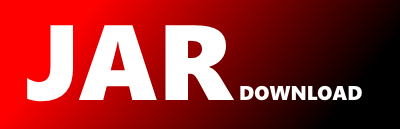
core.annotations.AttributesHandler Maven / Gradle / Ivy
package core.annotations;
import core.files.FileHelper;
import core.files.reader.ReadPropFile;
import core.reports.TestReporter;
import java.util.HashMap;
import static core.StringHandler.getHashMapFromString;
import static core.StringHandler.isStringBlank;
import static core.files.reader.ReadTXTFile.getRowOfTxtFile;
/**
* Created by Ismail on 12/26/2017.
* This class contains all related methods to read/write related
* Test Case Files and do required and suitable implementation
*/
public class AttributesHandler {
/*************** Class Variables Section ***************/
// This to save data file for current running test class
private static String classDataFile = "";
// Saves test case default file for current test class
private static String classTestFile = "";
// This to save test file for current running test method
private static String methodTestFile = "";
// This to save data row for current running test method
private static String methodDataRow = "";
/*************** Class Methods Section ***************/
/*************** Attributes Set/Get Methods Section Start ***************/
// This method Set Path of test file provided inside classDataFile
public static void setClassDataFile(String dataFile) {
// Reset Data File Value in case send null param
if (dataFile == null)
classDataFile = "";
else {
// Save Absolute File Path from File name to classDataFile
classDataFile = FileHelper.getFileAbsolutePath(dataFile);
}
}
// This method return Path of test data provided in getClassDataFile
public static String getClassDataFile() {
return classDataFile;
}
// Saves default test case file
public static void setClassTestFile(String testFile) {
// Reset Data File Value in case send null param
if (testFile == null)
classTestFile = "";
else {
// Save Absolute File Path from File name to classTestFile
classTestFile = FileHelper.getFileAbsolutePath(testFile);
}
}
// Saves default test case file
public static String getClassTestFile() {
return classTestFile;
}
// This method to set methodDataRow variable value
public static void setMethodDataRow(String dataRow) {
// IF dataRow parameter value is null then save empty value in methodDataRow
if (dataRow == null)
methodDataRow = "";
// Save dataRow value inside methodDataRow
methodDataRow = dataRow;
}
// This method to return methodDataRow variable value
public static String getMethodDataRow() {
return methodDataRow;
}
// This method to set MethodTestFile variable value
public static void setMethodTestFile(String testFile) {
if (testFile == null)
methodTestFile = "";
methodTestFile = testFile;
}
// This method to return MethodTestFile variable value
public static String getMethodTestFile() {
return methodTestFile;
}
/*************** Attributes Set/Get Methods Section End ***************/
/*************** Handle Attributes Values Methods Section Start ***************/
// This method to return a DataRow from Test data File as String
public static String getDataRow(String fileType, int rowNumber) {
// Check TestCase File not empty
if (isStringBlank(getClassDataFile()))
TestReporter.error("Test Data File Path is Empty.", true);
// Check Test Data File type to extract Row
if (fileType.equalsIgnoreCase("csv"))
return getCSVDataRow(getClassDataFile(), rowNumber).toString();
else
return getTXTDataRow(getClassDataFile(), rowNumber);
}
// This method to return a DataRow from Test data File as String
private static String getTXTDataRow(String filePath, int rowNumber) {
return getRowOfTxtFile(filePath, rowNumber);
}
// This method retrieves a DataRow of CSV Test Data File as String
private static HashMap getCSVDataRow(String filePath, int rowNumber) {
// Retrieve header line
String[] header = getTXTDataRow(filePath, 0).split(",");
String[] row = getTXTDataRow(filePath, rowNumber).split(",");
HashMap rowData = new HashMap<>();
for (int counter = 0; counter < header.length; counter++) {
rowData.put(header[counter], row[counter]);
}
return rowData;
}
// This method retrieves a value of DataRow of txt file
public static String getValueOfDataRow() {
// Check data row isn't empty to continue processing
if (isStringBlank(methodDataRow))
TestReporter.error("DataRow has no data, Please check if you provide method DataRow attribute or provided Row number has data", true);
// Split all data row by comma , then save first value from the array to a variable
String dataRowValue = getMethodDataRow().split(",")[0];
// remove from DataRow the value we are going to use
String newDataRow = getMethodDataRow().replaceFirst(dataRowValue, "");
// Check if newDataRow startWith comma to remove it
if (newDataRow.startsWith(","))
newDataRow = newDataRow.replaceFirst(",", "");
// Replace newDataRow with old one
setMethodDataRow(newDataRow);
// Return first value of data row
return dataRowValue;
}
// This method retrieves a value of DataRow of csv file
public static String getValueOfDataRow(String key) {
// concatenate empty string in case the key isn't exists then convert null as "null"
return getHashMapFromString("=", getMethodDataRow()).get(key) + "";
}
/*************** Handle Attributes Values Methods Section End ***************/
/*************** Reset Methods Section Start ***************/
// Reset method attributes values
public static void resetMethodAttributes() {
setMethodTestFile(null);
setMethodDataRow(null);
}
// Reset class attributes values
public static void resetClassAttributes() {
setClassTestFile(null);
setClassDataFile(null);
}
/*************** Reset Methods Section End ***************/
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy