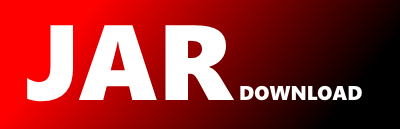
core.database.reader.MySql Maven / Gradle / Ivy
package core.database.reader;
import core.database.DBConnection;
import core.files.reader.ReadPropFile;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
/**
* Created by Ismail on 3/23/2018.
* This class contains specific methods to connect and read
* data from mySql DB type and return results in multi-types
*/
public class MySql {
// This method to connect and return a Statement for MySql DB Type
public static Statement getMySqlConnection(String dbInfoFile) {
// Read DB data info from the file
String driver = ReadPropFile.getProperty
(dbInfoFile, "driver_name");
String connectionURL = ReadPropFile.getProperty
(dbInfoFile, "connection_url");
String hostURL = ReadPropFile.getProperty
(dbInfoFile, "host_url");
String username = ReadPropFile.getProperty
(dbInfoFile, "username");
String password = ReadPropFile.getProperty
(dbInfoFile, "password");
// Build connectionString for mySql connection
String connectionString = connectionURL + hostURL + username + password;
// Connect to DB and return Statement
Statement statement = DBConnection.dbConnect(driver, connectionString);
// Return Statement
return statement;
}
// This method to connect and return a result of
// all data retrieved from a specific query
public static ResultSet getQueryResult(String dbInfoFile) {
// Save Query from Info file
String queryString = ReadPropFile.getProperty(dbInfoFile, "query");
// Use getQueryResult with two parameters to retrieve query result
return getQueryResult(dbInfoFile, queryString);
}
// This method to connect and return a result of
// all data retrieved from a specific query
public static ResultSet getQueryResult(String dbInfoFile, String query) {
// Connect to DB and return Statement from getMySqlConnection method
Statement statement = getMySqlConnection(dbInfoFile);
// Define Query result variable
ResultSet queryResult = null;
try {// Execute mySql query and get ResultSet
queryResult = statement.executeQuery(query);
} catch (SQLException e) {
e.printStackTrace();
}
return queryResult;
}
// This method to connect and return a result data of
// specific column data retrieved from a specific query
// All data provided from file
public static List getColumnResult(String dbInfoFile) {
// Extract query and column name from dbInfoFile
String query = ReadPropFile.getProperty(dbInfoFile, "query");
String columnName = ReadPropFile.getProperty(dbInfoFile, "column");
// Return column data results from getColumnResult with three parameters
return getColumnResult(dbInfoFile, query, columnName);
}
// This method to connect and return a result data of
// specific column data retrieved from a specific query
// All data provided from file except column name
public static List getColumnResult(String dbInfoFile, String columnName) {
// Extract query from dbInfoFile
String query = ReadPropFile.getProperty(dbInfoFile, "query");
// Return column data results from getColumnResult with three parameters
return getColumnResult(dbInfoFile, query, columnName);
}
// This method to connect and return a result data of
// specific column data retrieved from a specific query
// All data provided from file except query and column name
public static List getColumnResult(String dbInfoFile, String query, String columnName) {
// Save Query data results
ResultSet resultSet = getQueryResult(dbInfoFile, query);
// Define List to save column data results
List columnResult = new ArrayList<>();
// Save column data results
try {
while (resultSet.next()) {
columnResult.add(resultSet.getString(columnName));
}
} catch (SQLException e) {
e.printStackTrace();
}
// Return column data results
return columnResult;
}
// This method to connect and return a result data of
// specific row data retrieved from a specific query
// All data provided from file
public static String getRowResult(String dbInfoFile){
// Extract query and row number from dbInfoFile
String query = ReadPropFile.getProperty(dbInfoFile, "query");
String rowNumber = ReadPropFile.getProperty(dbInfoFile, "row");
// Return row data results from getRowResult with three parameters
return getRowResult(dbInfoFile, query, rowNumber);
}
// This method to connect and return a result data of
// specific row data retrieved from a specific query
// All data provided from file except rowNumber
public static String getRowResult(String dbInfoFile, String rowNumber){
// Extract query from dbInfoFile
String query = ReadPropFile.getProperty(dbInfoFile, "query");
// Return row data results from getRowResult with three parameters
return getRowResult(dbInfoFile, query, rowNumber);
}
// This method to connect and return a result data of
// specific row data retrieved from a specific query
// All data provided from file except query and rowNumber
private static String getRowResult(String dbInfoFile, String query, String rowNumber) {
// Save Query data results
ResultSet resultSet = getQueryResult(dbInfoFile, query);
// Define String to save Row data results
String rowResult = null;
// Save row data results
try {
rowResult = resultSet.getRowId(rowNumber).toString();
} catch (SQLException e) {
e.printStackTrace();
}
// Return row String value
return rowResult;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy