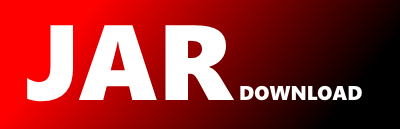
core.files.Conversion.ConvertCSVToExcel Maven / Gradle / Ivy
package core.files.Conversion;
import core.reports.TestReporter;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import static core.files.reader.ReadTXTFile.readTxtFile;
import static core.files.writer.WriteExcelFile.writeHeaderToExcelSheet;
import static core.files.writer.WriteExcelFile.writeRowToExcelSheet;
/**
* Created by Ismail on 10/23/2018.
* This class converts a CSV files to excel file with csv content
*/
public class ConvertCSVToExcel {
/*************** Class Methods Section ***************/
// This method converts a CSV file to excel file
public static void convertCSVToExcel(String csvFilePath, String excelFilePath) {
// Read csv file content
List fileContent = readTxtFile(csvFilePath);
// Generate sheet name
String sheetName = new File(csvFilePath).getName().replaceAll(".csv", "");
// Iterate file content to convert them to excel sheet
int counter = 0;
for (String line : fileContent) {
// Check if counter is zero then write the header to excel sheet
ArrayList lineList = new ArrayList<>();
lineList.add(line);
if (counter == 0) {
// Write excel sheet header
writeHeaderToExcelSheet(excelFilePath, sheetName, lineList);
counter++;
} else {
// Write excel sheet row
writeRowToExcelSheet(excelFilePath, sheetName, counter, lineList);
counter++;
}
}
}
// This method converts a list of CSV files to excel sheets
public static void convertListCSVsToExcel(String csvFolderPath, String excelFilePath) {
// Check if folder exists and contains files
if (!new File(csvFolderPath).isDirectory())
TestReporter.error("CSV Folder Path Provided isn't directory or hasn't files, Please check: " + csvFolderPath, false);
// Retrieve list of csv Files
File[] files = new File(csvFolderPath).listFiles();
// Iterate the list of files to convert them to excel sheets
for (File file : files) {
convertCSVToExcel(file.getPath(), excelFilePath);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy