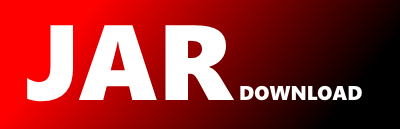
core.files.FileHelper Maven / Gradle / Ivy
package core.files;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.filefilter.TrueFileFilter;
import org.testng.annotations.Test;
import java.io.File;
import java.util.Iterator;
import static core.files.validator.FileStatusValidator.isFileExist;
import static core.files.writer.WriteTXTFile.writeTxtFile;
import static core.internal.EnvironmentInterface.RESOURCES_TEST_PATH;
import static core.internal.EnvironmentInterface.TEMP_RESOURCES_PATH;
/**
* Created by Ismail on 1/1/2018.
* This Class contains all generic methods related to Read Files
*/
public class FileHelper {
/*************** Class Methods Section ***************/
// This method search in Test Resources Folders/sub to retrieve the file
public static String getFileAbsolutePath(String fileName) {
// Save file partial path if user provides fileName with path, Like: test/admin/ismail.csv
String filePath = fileName.replace(new File(fileName).getName(), "");
fileName = new File(fileName).getName();
// Initialize Iterator and get all sub-Directories and files
Iterator iterator = FileUtils.iterateFiles(new File("src/"), TrueFileFilter.INSTANCE, TrueFileFilter.INSTANCE);
// Iterate all Files content and search for File name
while (iterator.hasNext()) {
// Get File Path as File
File file = (File) iterator.next();
// Check if File name is equal to Provided one
if (file.getName().equalsIgnoreCase(fileName)) {
// Check fileName contains partial path
if (file.getPath().contains(filePath))
return file.getAbsolutePath();
}
}
// If file not exists
return null;
}
// This method creates a temp file
public static String createTempFile(String fileName, String content) {
String filePath = TEMP_RESOURCES_PATH + fileName;
// If temp file exists then delete it
if (isFileExist(filePath))
deleteFile(filePath);
// Create a new file and write content
writeTxtFile(filePath, content);
return filePath;
}
// This method creates directory and sub-directories the file
public static void createFolders(String filePath) {
// Create file parent directories
// Here a bug in case the folders exists and need to get absolute path
if (new File(filePath).getParentFile() != null)
new File(filePath).getParentFile().mkdirs();
}
// This method deletes existing file
public static void deleteFile(String fileName) {
// Check if file exist and get file absolute path name
if (!isFileExist(fileName))
// build full path for txtFile
fileName = getFileAbsolutePath(fileName);
// Check if file exists then delete it
if (isFileExist(fileName))
new File(fileName).delete();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy