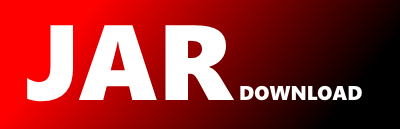
core.files.reader.ReadJSONFile Maven / Gradle / Ivy
package core.files.reader;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import static core.files.FileHelper.getFileAbsolutePath;
import static core.files.validator.FileExtensionValidator.verifyJSONFileType;
import static core.files.validator.FileStatusValidator.isFileExist;
import static core.files.validator.FileStatusValidator.verifyFileStatus;
import static core.reports.TestReporter.error;
/**
* Created by ismail on 8/24/18.
* This class contains all related methods to Read From JSON File
*/
public class ReadJSONFile {
/*************** Class Methods Section ***************/
// This method to read JSON File and return it's content as Object
public static JsonNode readJsonFile(String jsonFileName) {
// Check if provided file name only without path then add test resources full path
if (!isFileExist(jsonFileName))
// build full path for json file
jsonFileName = getFileAbsolutePath(jsonFileName);
// Verify jsonFile Status
verifyFileStatus(jsonFileName);
// Verify jsonFile content Type
verifyJSONFileType(jsonFileName);
// Define JsonNode variable
JsonNode jsonNode = null;
//read json file data to String
try {
byte[] jsonData = Files.readAllBytes(Paths.get(jsonFileName));
// Create ObjectMapper instance
ObjectMapper objectMapper = new ObjectMapper();
// Save JsonNode from Mapper
return jsonNode = objectMapper.readTree(jsonData);
} catch (Throwable throwable) {
// In case something went wrong Throw an error to Report and fail test case
error("Something went wrong while Reading JSON File, Please check log: " + throwable.getMessage(), true);
return null;
}
}
// This method retrieve a value as jsonNode
public static JsonNode getJsonValue(String jsonFileName, String fieldName) {
return readJsonFile(jsonFileName).findValue(fieldName);
}
// This method retrieve a values as jsonNode
public static List getJsonValues(String jsonFileName, String fieldName) {
return readJsonFile(jsonFileName).findValues(fieldName);
}
// This method to retrieve a String value from jsonNode
public static String getJsonValueAsString(String jsonFileName, String fieldName) {
if (getJsonValue(jsonFileName, fieldName) != null)
return getJsonValue(jsonFileName, fieldName).asText();
else {
//error("There is no field with name: " + fieldName + " in json object.", false);
return "";
}
}
// This method retrieve a String values from jsonNode
public static List getJsonValuesAsString(String jsonFileName, String fieldName) {
// Define a list to save all values inside
List values = new ArrayList<>();
// Iterate all JsonNodes and retrieve all values as string
getJsonValues(jsonFileName, fieldName).listIterator().forEachRemaining(node ->
values.add(node.asText()));
// Return List values
return values;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy