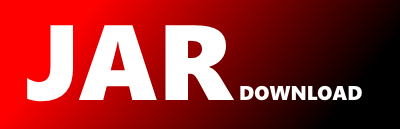
core.files.reader.ReadTXTFile Maven / Gradle / Ivy
package core.files.reader;
import core.reports.TestReporter;
import org.apache.commons.lang3.StringUtils;
import org.testng.annotations.Test;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStreamReader;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import static core.StringHandler.isStringBlank;
import static core.files.FileHelper.getFileAbsolutePath;
import static core.files.validator.FileStatusValidator.isFileExist;
import static core.files.validator.FileStatusValidator.verifyFileStatus;
/**
* Created by Ismail on 12/25/2017.
* This class contains all related methods for reading TXT File
*/
public class ReadTXTFile {
/*************** Class Methods Section ***************/
// This method to read TXT File and return it's content as List of string
public static List readTxtFile(String txtFile) {
// Check if file exist and get file absolute path name
if (!isFileExist(txtFile))
// build full path for txtFile
txtFile = getFileAbsolutePath(txtFile);
// Verify txtFile Status
verifyFileStatus(txtFile);
// Get file content line by line and save them to List of string
try (Stream lines = new BufferedReader(new InputStreamReader(new FileInputStream(txtFile), "utf-8")).lines()) {
// Return File content after removing null or empty lines
return removeListNullEmptyValues(lines.collect(Collectors.toList()));
} catch (Throwable throwable) {
// In case something went wrong
// Throw an error to Report and fail test case
TestReporter.error("Something went wrong Read TXT File, Please check log: " + throwable.getMessage(), true);
return null;
}
}
// This method to read TXT File and returns the content as String
public static String readTxtFileAsString(String txtFile) {
// Read Txt File as List then join it to String
return StringUtils.join(readTxtFile(txtFile));
}
// This method to read TXT File and return specific row of the file
public static String getRowOfTxtFile(String fileName, int rowNumber) {
// Define List of strings to save all file content inside the list
List textContent = readTxtFile(new File(fileName).getName());
// Check if Row number is provided and valid
rowNumber = rowNumber >= 0 ? rowNumber : 0;
// Check if Text file has content and not empty and the row contains data
if (textContent.size() > 0 && textContent.size() > rowNumber && !isStringBlank(textContent.get(rowNumber)))
return textContent.get(rowNumber);
else {
TestReporter.error("Something went wrong while reading Txt File.\n" +
"File name: " + fileName + ".\nRow Number: " + (rowNumber + 1), true);
return "";
}
}
// This method remove all null or empty lines from provided List
public static List removeListNullEmptyValues(List listContent) {
// Remove any line similar to "" or null (equals to empty and null)
listContent.removeAll(Arrays.asList("", null));
// Return cleaned List
return listContent;
}
@Test
public void test() {
readTxtFile("C:\\Users\\Ismail\\IdeaProjects\\core-automation\\src\\test\\resources\\testing_json\\input_hamza.txt");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy