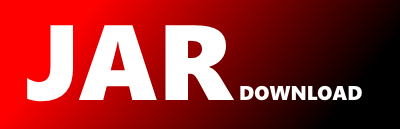
core.os_ops.Services Maven / Gradle / Ivy
package core.os_ops;
import core.reports.TestReporter;
import org.apache.commons.lang3.StringUtils;
import org.testng.Assert;
import java.io.*;
import java.net.ServerSocket;
import java.util.concurrent.TimeUnit;
/**
* Created by Ismail on 2/23/2018.
* This class contains methods related to operations
* that made on OS level (like: change, stop or start services)
*/
public class Services {
/*************** Class Variables Section ***************/
/*************** Class Methods Section ***************/
// This method to check if a specific port running and in use or there is a service reserve it and return Boolean value as status of port usage status
public static Boolean checkPortInUse(int portNo) {
// Define Boolean variable
Boolean isServerRunning = false;
// Checking if port in use
try {// Initialize ServerSocket and assign the port for
ServerSocket serverSocket = new ServerSocket(portNo);
// Try to close assigned ServerSocket
} catch (IOException e) {
// In case port in use will throw exception
isServerRunning = true;
}
// Return result of isServerRunning variable
return isServerRunning;
}
/* This method still not fully implemented */
// This method to check if a specific program installed on current machine and return Boolean value of program status is installed or not
public static Boolean checkProgramExists(String programCommand) {
// Define Program exist Boolean variable
Boolean isProgramExists = false;
try {
// Check if program is installed or not
// Define ProcessBuilder variable and assign command
ProcessBuilder processBuilder = new ProcessBuilder(programCommand);
// Define Process and start ProcessBuilder with assigned command
Process process = processBuilder.start();
// Wait until Process object execution ends
process.waitFor();
// Check command execution success
// Need a way to verify the program is installed
} catch (Throwable e) {
// Do action here
}
// Return result of isProgramExists variable
return isProgramExists;
}
// This method to run specific process and return process object
public static Process getProcess(String processCommand) {
// Define Process object to execute processCommand
Process process = null;
// Define String Buffer to save process Input Stream
StringBuffer output = new StringBuffer();
// Save OS type in a variable
String os = System.getProperty("os.name").toLowerCase();
try {
// Start Processing command to be executed
// Define Command variable array as a ProcessBuilder parameter
String[] command;
// Check if OS is Windows then assign Windows command
if (os.contains("win"))
command = new String[]{"CMD", "/C", processCommand};
// Check if OS is Mac then assign Mac command
else if (os.contains("mac"))
command = new String[]{"/bin/bash", "-c", processCommand};
// Else OS is linux/unix and assign linux/unix command
else
command = new String[]{"/bin/bash", "-c", processCommand};
// Define ProcessBuilder and send full command to be executed
ProcessBuilder processBuilder = new ProcessBuilder(command);
// Define redirectErrorStream in case error then will return the error value
processBuilder.redirectErrorStream(true);
// Run Command with Process object
process = processBuilder.start();
// Wait for process ends
process.waitFor(5, TimeUnit.SECONDS);
// In case exception error happen then will fail the process
} catch (IOException | InterruptedException e) {
Assert.fail("Something went wrong, please check: " + e.getMessage());
}
// Return Process
return process;
}
// This method to run multi processes and return process object
public static Process getProcess(String... processCommands) {
// Get Process from first command
Process process = getProcess(processCommands[0]);
OutputStream outputStream = process.getOutputStream();
for (String command : processCommands) {
// Skip first command
if (command.equals(processCommands[0]))
continue;
// Verify command isn't empty
if (!command.isEmpty()) {
try {
outputStream.write(command.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
return process;
}
// This method to run specific process and return process result object
public static String getProcessOutput(String processCommand) {
// Define String Buffer to save process Input Stream
StringBuffer output = new StringBuffer();
try {
// Save all output of process to Buffered Reader object with getProcess() Method
BufferedReader reader = new BufferedReader(new InputStreamReader(getProcess(processCommand).getInputStream()));
// Define String to save each line of Buffered Reader
String line;
while ((line = reader.readLine()) != null) {
// Save all Buffered Reader lines to String Buffer
output.append(line + "\n");
}
// In case exception error happen then will fail the process
} catch (IOException e) {
Assert.fail("Something went wrong, please check: " + e.getMessage());
}
// Check if output isn't null then return output.toString() value else return empty value
return output.toString() != null ? output.toString() : "";
}
// This method to run multi processes and return process result object
public static String getProcessOutput(String... processCommands) {
// Define String Buffer to save process Input Stream
StringBuffer output = new StringBuffer();
try {
// Save all output of process to Buffered Reader object with getProcess() Method
BufferedReader reader = new BufferedReader(new InputStreamReader(getProcess(processCommands).getInputStream()));
// Define String to save each line of Buffered Reader
String line;
while ((line = reader.readLine()) != null) {
// Save all Buffered Reader lines to String Buffer
output.append(line + "\n");
}
// In case exception error happen then will fail the process
} catch (IOException e) {
Assert.fail("Something went wrong, please check: " + e.getMessage());
}
// Check if output isn't null then return output.toString() value else return empty value
return output.toString() != null ? output.toString() : "";
}
// This method to run specific process and return process result as lines
public static String[] getProcessOutputLines(String processCommand) {
return getProcessOutput(processCommand).split("\\n");
}
// This method to run specific process and return specific index of process result object
public static String getProcessOutput(String processCommand, int resultIndex) {
// Call getProcessOutput(String processCommand) and save full result from the method
String fullResult = getProcessOutput(processCommand);
// Check if resultIndex isn't null then return else return empty result
return fullResult.split("\\n")[resultIndex] != null ? fullResult.split("\\n")[resultIndex] : "";
}
// This method to run specific process and return specific String of process result object
public static String getProcessOutput(String processCommand, String resultContainsString) {
// Call getProcessOutput(String processCommand) and save full result as array from the method
String[] fullResult = getProcessOutput(processCommand).split("\\n");
// Define resultString to save String that containsString
String resultString = "";
// loop fullResult indexes and check if any index contains resultContainsString then return that index
for (int counter = 0; counter < fullResult.length; counter++) {
if (fullResult[counter].contains(resultContainsString)) {
resultString = fullResult[counter];
break;
}
}
// Check if resultString is empty then fail the test case
if (resultString.isEmpty())
TestReporter.error("There is no String in terminal result." + "\nCommand String : " + processCommand + "\nresultContainsString Param: " + resultContainsString + "\nTerminal result:\n" + StringUtils.join(fullResult, " "), false);
// Return resultString value
return resultString;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy