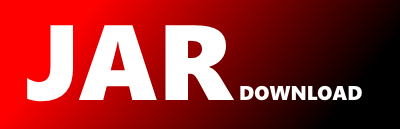
core.reports.TestReporter Maven / Gradle / Ivy
package core.reports;
import core.reports.extent.BuildReportActions;
import com.relevantcodes.extentreports.ExtentReports;
import com.relevantcodes.extentreports.ExtentTest;
import core.internal.EnvironmentInterface;
import org.testng.Assert;
import org.testng.ITestContext;
import java.lang.reflect.Method;
/**
* Created by Ismail on 12/25/2017.
* This class contains all related methods for reporting
* and inherit report action methods from BuildReportActions
*/
public class TestReporter extends BuildReportActions {
/*************** Class Variables Section ***************/
// Define variable of ExtentReports to write all logs to report file
public static ExtentReports report;
// Define Logger for Test to be used with @BeforeTest
public static ExtentTest testLogger;
// Define Logger for Test Method to be used with @BeforeMethod
public static ExtentTest logger;
/*************** Class Methods Section ***************/
// This method to create Report File name
public static void prepareReport(ITestContext testContext) {
// Save current execution time
String reportTime = EnvironmentInterface.CURRENT_TIME;
// Save current running SuiteName
String suiteName = testContext.getSuite().getName().replace(" ", "-");
// Initialize Report File name with current saved time and suite
report = new ExtentReports(EnvironmentInterface.REPORT_PATH + suiteName + reportTime + ".html", true);
}
// This method to initialize and add test description
public static void prepareTestReport(ITestContext testContext) {
// Save current running Test Name
testLogger = report.startTest(testContext.getName());
// Set Description for testLogger
testLogger.setDescription("Test of: " + testContext.getName());
}
// This method to initialize and add Method categories
public static void prepareMethodReport(Method method) {
// Save current running Test Method Name
logger = report.startTest(method.getName());
// Assign class name of current method as category
logger.assignCategory(method.getDeclaringClass().getName());
}
// This method to add categories for Provided method
public static void addMethodCategories(ITestContext testContext, Method method) {
// Send testContext and Method to addMethodGroups Method
// This method inherited from BuildReportActions
addMethodGroups(testContext, method);
}
// This method to finalize and write all outputs from Test Method Logger
public static void generateMethodReport() {
// End Test Method logger and save all output to report
report.endTest(logger);
// Define Test Method Logger as null again
TestReporter.logger = null;
}
// This method to finalize and write all outputs from Test Logger
public static void generateTestReport() {
// End Test logger and save all output to report
report.endTest(testLogger);
// Define Test Logger as null again
TestReporter.testLogger = null;
}
// This method to write report file and close
public static void generateFinalReport() {
// Write all data to report file
report.flush();
// Close report file
report.close();
}
// This method to add info step to the report file of current running test
public static void info(String infoString) {
// This method inherited from BuildReportActions
addInfo(infoString);
}
// This method to add pass step to the report file of current running test
public static void pass(String passString, String screenShotName) {
// This method inherited from BuildReportActions
addPass(passString, screenShotName);
}
// This method to add error step to the report file of current running test without Exception Error parameter
public static void error(String errorString, Boolean failStatus) {
// Save class and method names and line number that parse the error
String className = Thread.currentThread().getStackTrace()[2].getClassName();
String methodName = Thread.currentThread().getStackTrace()[2].getMethodName();
int lineNumber = Thread.currentThread().getStackTrace()[2].getLineNumber();
// This method inherited from BuildReportActions and send errorString to report
addError("Something went wrong while running method " + methodName + " in class " + className + " Line " + lineNumber + "Error as below." + errorString);
// If true then fail current test case
if (failStatus)
Assert.fail(errorString);
}
// This method to add error step to the report file of current running test
public static void error(String errorString, Throwable exceptionError, Boolean failStatus) {
// Save class and method names and line number that parse the error
String className = Thread.currentThread().getStackTrace()[2].getClassName();
String methodName = Thread.currentThread().getStackTrace()[2].getMethodName();
int lineNumber = Thread.currentThread().getStackTrace()[2].getLineNumber();
// This method inherited from BuildReportActions and send errorString to report
addError("Something went wrong while running method " + methodName + " in class " + className + " Line " + lineNumber + "Error as below." + errorString + "Please check message: " + exceptionError.getMessage());
// If true then fail current test case
if (failStatus)
Assert.fail(errorString);
}
// This method to add fail step to the report file of current running test without Exception Error parameter
public static void fail(String failString, Boolean failStatus) {
// Save class and method names and line number that parse the error
String className = Thread.currentThread().getStackTrace()[2].getClassName();
String methodName = Thread.currentThread().getStackTrace()[2].getMethodName();
int lineNumber = Thread.currentThread().getStackTrace()[2].getLineNumber();
// This method inherited from BuildReportActions and send failString to report
addFail("Test case fail due to error in running method " + methodName + " in class " + className + " Line " + lineNumber + "Fail reason as below." + failString);
// If true then fail current test case
if (failStatus)
Assert.fail(failString);
}
// This method to add fail step to the report file of current running test
public static void fail(String failString, Throwable exceptionError, Boolean failStatus) {
// Save class and method names and line number that parse the error
String className = Thread.currentThread().getStackTrace()[2].getClassName();
String methodName = Thread.currentThread().getStackTrace()[2].getMethodName();
int lineNumber = Thread.currentThread().getStackTrace()[2].getLineNumber();
// This method inherited from BuildReportActions and send failString to report
addFail("Test case fail due to error in running method " + methodName + " in class " + className + " Line " + lineNumber + "Fail reason as below." + failString + "Please check message: " + exceptionError.getMessage());
// If true then fail current test case
if (failStatus)
Assert.fail(failString);
}
// This method to add description for current test
public static void testCaseDescription(String testDescription) {
// This method inherited from BuildReportActions
addDescription(testDescription);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy