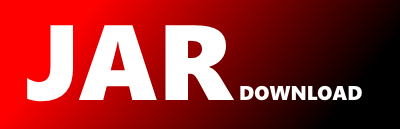
automated.database.DBConnection Maven / Gradle / Ivy
package automated.database;
import automated.reporting.TestReporter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
import static java.sql.ResultSet.CONCUR_UPDATABLE;
import static java.sql.ResultSet.TYPE_SCROLL_INSENSITIVE;
/**
* Created by Ismail on 3/23/2018.
* This class contains generic methods for DB Connection
*/
public class DBConnection {
// This method is a generic method to connect specific DB
// You send driver name that specify DB type with connectionString
public static Statement dbConnect(String driverName, String connectionString) {
// Define Statement results that will be returned
Statement statement = null;
try {// Start connecting to DB server
Class.forName(driverName);// Assign driver name for DB type
// Define connection with connectionString of username, password
// and connection for DB type
Connection connection = DriverManager.getConnection(connectionString);
// Save statement of connected DB to use it for
// building queries
statement = connection.createStatement
(TYPE_SCROLL_INSENSITIVE, CONCUR_UPDATABLE);
} catch (Throwable throwable) {// In case fail report
TestReporter.fail("Fail connect to Database Server, " +
"please check connection : "
+ connectionString + "\n"
+ throwable.getMessage(), true);
}// Return statement connected to DB type
return statement;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy