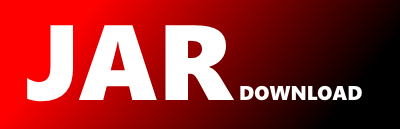
automated.platforms.web.RunWebDriver Maven / Gradle / Ivy
The newest version!
package automated.platforms.web;
import automated.core.enums.TimeEnum;
import io.github.bonigarcia.wdm.*;
import automated.test_manage.config.Platform;
import automated.core.enums.TimeEnum;
import automated.reporting.TestReporter;
import automated.test_manage.config.Platform;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxOptions;
import org.openqa.selenium.ie.InternetExplorerDriver;
import org.openqa.selenium.opera.OperaDriver;
import automated.reporting.TestReporter;
import org.openqa.selenium.opera.OperaOptions;
import java.util.concurrent.TimeUnit;
import static automated.test_manage.config.Platform.*;
/**
* Created by Ismail on 12/25/2017.
* This Class contains methods related to manage WebDriver
* to run, initialize and stop WebDriver instance for web applications
*/
public class RunWebDriver {
/*************** Class Methods Section ***************/
// This method to run WebDriver as Web Application
// and open specific browser
public static void setUp() {
// Check browser case to run suitable one
// Running and opening browser using DriverManager plugin
// This plugin will take responsibility instead of drivers files
// for each browser and download suitable one from internet
switch (Platform.browser.toLowerCase()) {
case "chrome":
case "google chrome":
case "googlechrome": {
// Define Browser Options
ChromeOptions chromeOptions = new ChromeOptions();
// Disable any info bar to appear on automated browser
chromeOptions.addArguments("disable-infobars");
// Run driver file
ChromeDriverManager.getInstance().setup();
// Initialize WebDriver with its options
Platform.driver = new ChromeDriver(chromeOptions);
break;
}
case "firefox":
case "ff": {
// Define Browser Options
FirefoxOptions firefoxOptions = new FirefoxOptions();
// Disable any info bar to appear on automated browser
firefoxOptions.addArguments("disable-infobars");
// Run driver file
FirefoxDriverManager.getInstance().setup();
// Initialize WebDriver with its options
Platform.driver = new FirefoxDriver(firefoxOptions);
break;
}
case " opera": {
// Define Browser Options
OperaOptions operaOptions = new OperaOptions();
// Disable any info bar to appear on automated browser
operaOptions.addArguments("disable-infobars");
// Run driver file
OperaDriverManager.getInstance().setup();
// Initialize WebDriver with its options
Platform.driver = new OperaDriver(operaOptions);
break;
}
case "ie":
case "internet":
case "internet explorer":
case "internetexplorer": {
// Run driver file
InternetExplorerDriverManager.getInstance().setup();
// Initialize WebDriver with its options
Platform.driver = new InternetExplorerDriver();
break;
}
case "edge": {
// Run driver file
EdgeDriverManager.getInstance().setup();
// Initialize WebDriver with its options
Platform.driver = new EdgeDriver();
break;
}
default: {
TestReporter.error("Wrong Browser Type" +
Platform.browser, true);
}
}// Here to run some specifications for browsers
try {
// Set focus on browser in case running on Mac platforms
((JavascriptExecutor) Platform.driver).executeScript("alert('Test')");
Platform.driver.switchTo().alert().accept();
// Provide Maximize for browser window
Platform.driver.manage().window().maximize();
// Apply implicitlyWait for WebDriver to wait between actions
// just for a reason not always
Platform.driver.manage().timeouts().implicitlyWait
(TimeEnum.IMPLICIT_WAIT_TIMEOUT.value(), TimeUnit.SECONDS);
// Apply page load timeout, it's default in WebDriver as 30 sec
Platform.driver.manage().timeouts().pageLoadTimeout
(TimeEnum.PAGE_LOAD_TIMEOUT.value(), TimeUnit.SECONDS);
// Apply script timeout
Platform.driver.manage().timeouts().setScriptTimeout
(TimeEnum.SCRIPT_TIMEOUT.value(), TimeUnit.SECONDS);
} catch (Throwable throwable) {
// If fail for any reason test case will fail and send to report
TestReporter.error("Something went wrong, Please check message: " + throwable.getMessage(), true);
}// If everything goes fine then will provide info step done to report
TestReporter.info(Platform.browser + " Browser opened and Running...");
}
// This method to close the browser
public static void tearDown() {
try {// Check if WebDriver initialized as browser and still running
if (Platform.driver.toString() != null) {
Platform.driver.close();// If yes then will close the browser
// After browser closed will send info step done to report
TestReporter.info("Browser Window Closed...");
}
} catch (Throwable throwable) {
// If fail will send error to report without failing the test case
TestReporter.error("Unable to close Browser, Please check message: " + throwable.getMessage(), false);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy