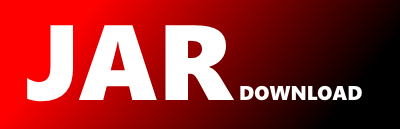
automated.process.io.read.ReadFile Maven / Gradle / Ivy
package automated.process.io.read;
import automated.core.enums.PathEnum;
import automated.reporting.TestReporter;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.filefilter.TrueFileFilter;
import java.io.File;
import java.util.Iterator;
/**
* Created by Ismail on 1/1/2018.
* This Class contains all generic methods related to Read Files
*/
public class ReadFile {
/*************** Class Methods Section ***************/
// This method search in Test Resources Folder and sub-Folders
// for all files and try to find The Provided File name then return
// Absolute Path for that file
public static String getFileAbsolutePath(String fileName) {
// Initialize Iterator and get all sub-Directories and files
// This method Provided from library read Test Resources and return
// all files
Iterator iterator = FileUtils.iterateFiles(
new File(PathEnum.RESOURCES_TEST.toString()),
TrueFileFilter.INSTANCE, TrueFileFilter.INSTANCE);
// Iterate all Files content and search for Provided File name
// once reach one equals to file name then will return as string
// Note: the search for file name insensitive case and first one
// be found will be returned
while (iterator.hasNext()) {
// Get File Path as File
File file = (File) iterator.next();
// Check if File name is equal to Provided one
if (file.getName().equalsIgnoreCase(fileName)) {
// for windows we need to convert \ to /
// Then Will return the Absolute Path as String
return file.toString().replace("\\", "/");
}
}// Default return is null String
// Check if file isn't exist then report
TestReporter.error("File isn't exist, please check: " +
"file name >> ( " + fileName + " )", true);
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy