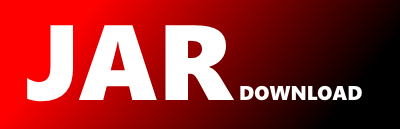
automated.process.io.read.ReadPropFile Maven / Gradle / Ivy
The newest version!
package automated.process.io.read;
import automated.core.enums.PathEnum;
import automated.process.io.validations.ValidateExtension;
import automated.process.io.validations.ValidateFile;
import automated.reporting.TestReporter;
import java.io.FileInputStream;
import java.io.InputStream;
import java.util.Properties;
import java.util.Set;
/**
* Created by Ismail on 12/26/2017.
* This class contains all related methods for Read From
* Properties File (*.properties) and do actions or retrieve data
* from the file
*/
public class ReadPropFile {
/*************** Class Methods Section ***************/
// This method read File Properties content
// and return all contents as Properties Object
private static Properties readPropFile(String propFile) {
// get file path without resource path if provided
propFile = propFile.replace(PathEnum.RESOURCES_TEST.toString(), "");
// build full path for properties file
propFile = PathEnum.RESOURCES_TEST + propFile;
// Verify propFile Status
ValidateFile.verifyFileStatus(propFile);
// Verify propFile content Type
ValidateExtension.verifyPropertiesFileType(propFile);
// Initialize Properties Object
Properties properties = new Properties();
// Start operating to Load Properties from file
try {// Read The Provided File content as inputStream
InputStream inputStream = new FileInputStream(propFile);
properties.load(inputStream);// Load inputStream Object to Properties
// Convert all Properties Keys to Lower Case
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy