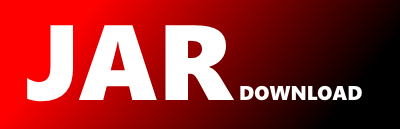
automated.process.io.read.ReadTXTFile Maven / Gradle / Ivy
package automated.process.io.read;
import automated.process.io.validations.ValidateFile;
import automated.reporting.TestReporter;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Created by Ismail on 12/25/2017.
* This class contains all related methods for reading TXT File
*/
public class ReadTXTFile {
/*************** Class Methods Section ***************/
// This method to read TXT File and return it's content as List of string
public static List readTxtFile(String fileName) {
// Define full name variable
String fullName = "";
// Check if file exist and get file absolute path name
if (ReadFile.getFileAbsolutePath(fileName) != null)
fullName = ReadFile.getFileAbsolutePath(fileName);
else {// send error step to report and fail test case
TestReporter.error("File name provided isn't exist"
+ fileName, true);
}
// Verify File status in case has any error
ValidateFile.verifyFileStatus(fullName);
// Get file content line by line and save them to List of string
try (Stream lines = Files.lines(Paths.get(fullName))) {
// Return File content after removing null or empty lines
return removeListNullEmptyValues(lines.collect(Collectors.toList()));
} catch (Throwable throwable) {// In case something went wrong
// Throw an error to Report and fail test case
TestReporter.error("Something went wrong Read TXT File," +
"Please check log: " + throwable.getMessage(), true);
}// Default return is null List
return null;
}
// This method to read TXT File and return specific row of the file
public static String getRowOfTxtFile(String fileName, int rowNumber) {
// Define List of strings to save all file content inside the list
// Use readTxtFile to read all file content
// new File(fileName).getName() >> This to send only file name without path
List textContent = readTxtFile(new File(fileName).getName());
// Check if Row number is provided and valid
if (rowNumber < 0)
TestReporter.error("DataRow attribute isn't provided.\nYou can provide Data Row for test method with DataRow attribute.", true);
// Check if Text file has content isn't empty
if (textContent.size() != 0)
if (textContent.size() > rowNumber)// Check if rowNumber within file content list
// Check if rowNumber String isn't empty
if (!textContent.get(rowNumber).isEmpty())
return textContent.get(rowNumber);
// If rowNumber is larger than file content list then report
if (textContent.size() <= rowNumber)
TestReporter.error("DataRow No " + (rowNumber + 1) + " of Text File you Provided is empty, Please check file : (" + fileName + ")", true);
else// If row data is empty then report
TestReporter.error("Text File you Provided is empty, please check: " + fileName, true);
return null;
}
// This method remove all null or empty lines from provided List
public static List removeListNullEmptyValues(List listContent) {
// Remove any line similar to "" or null (equals to empty and null)
listContent.removeAll(Arrays.asList("", null));
// Return cleaned List
return listContent;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy