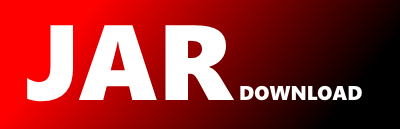
automated.reporting.extent.BuildReportActions Maven / Gradle / Ivy
The newest version!
package automated.reporting.extent;
import com.relevantcodes.extentreports.LogStatus;
import automated.reporting.TestReporter;
import automated.test_manage.config.TakeScreenShot;
import org.testng.ITestContext;
import org.testng.annotations.Test;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import java.util.UUID;
/**
* Created by Ismail on 12/25/2017.
* This class contains all related methods for report actions
* of type ExtentReport
*/
public class BuildReportActions {
/*************** Class Methods Section ***************/
// This method to add Info to the report
protected static void addInfo(String infoString) {
// If Test Method logger still not initialized
// then this info will be added to test logger instead
if (TestReporter.logger == null)
TestReporter.testLogger.log(LogStatus.INFO, infoString);
else// Else will be added to Method logger
TestReporter.logger.log(LogStatus.INFO, infoString);
}
// This method to add Pass to the report
protected static void addPass(String passString) {
// If Test Method logger still not initialized
// then this pass will be added to test logger instead
if (TestReporter.logger == null)
TestReporter.testLogger.log(LogStatus.PASS, passString);
else {// Else will be added to Method logger
// Generate Screen Shot for passed step with random name
String image = TakeScreenShot.
captureScreenshot(UUID.randomUUID().toString());
if (image != null)
// If image captured then save to report log message
passString += TestReporter.logger.addScreenCapture(image);
// Write step message and image to report
TestReporter.logger.log(LogStatus.PASS, passString);
}
}
// This method to add Error to the report
protected static void addError(String errorString) {
// If Test Method logger still not initialized
// then this error will be added to test logger instead
if (TestReporter.logger == null)
TestReporter.testLogger.log(LogStatus.ERROR, errorString);
else// Else will be added to Method logger
TestReporter.logger.log(LogStatus.ERROR, errorString);
}
// This method to add Fail to the report
protected static void addFail(String failString) {
// If Test Method logger still not initialized
// then this fail will be added to test logger instead
if (TestReporter.logger == null)
TestReporter.testLogger.log(LogStatus.FAIL, failString);
else// Else will be added to Method logger
TestReporter.logger.log(LogStatus.FAIL, failString);
}
// This method to add Description to Method Logger
protected static void addDescription(String testDescription) {
TestReporter.logger.setDescription(testDescription);
}
// This method to add Groups for Test Method Logger
protected static void addMethodGroups(ITestContext testContext, Method method) {
// Add Test as category for all methods
TestReporter.logger.assignCategory(TestReporter.testLogger.getDescription());
// Get if there is include Groups in testNG.xml file
List includedGroups = testContext.getCurrentXmlTest().getIncludedGroups();
// Get Method Groups
List methodGroups = Arrays.asList(method.getAnnotation(Test.class).groups());
// If include groups not empty then
// just update test report with each group as category
// Check if Logger isn't null
if (TestReporter.logger != null) {// Check if includedGroups not null
if (includedGroups.size() != 0) {
// Start Assigning groups as categories for Test Method
for (String groupName : includedGroups) {
TestReporter.logger.assignCategory(groupName);
}
} else {// If testNG.xml isn't contains include Groups
// Start Assigning all Test Method Groups
// as Test Report categories
for (String groupName : methodGroups) {
TestReporter.logger.assignCategory(groupName);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy