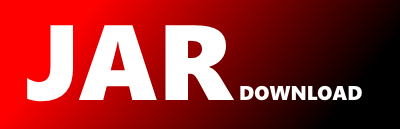
automated.test_manage.actions.Actions Maven / Gradle / Ivy
package automated.test_manage.actions;
import automated.platforms.web.WebActions;
/**
* Created by Ismail on 12/25/2017.
* This class contains implementation of action methods
* Code implementation of these actions exists in
* different classes in actions package
*/
public class Actions {
/*************** Class Methods Section ***************/
// This method apply click action
public static void click(String selector) {
// Call SeleniumActions.click method
SeleniumActions.click(selector);
}
// This method apply sendText action
public static void sendText(String selector, String text) {
// Call SeleniumActions.sendText method
SeleniumActions.sendText(selector, text);
}
// This method apply NavigateTo URL action
public static void goTo(String URL) {
// Call WebActions.navigateTo method
WebActions.navigateTo(URL);
}
// This method apply NavigateTo Back button from browser action
public static void goBack() {
// Call WebActions.navigateTo method with sending back action
WebActions.navigateTo("back");
}
// This method to apply getText from element action
public static String getText(String selector) {
// Call SeleniumActions.getText method
return SeleniumActions.getText(selector);
}
// This method to apply wait for time in seconds action
public static void waitFor(int timeInSeconds) {
try {// Wait for provided time in seconds
Thread.sleep(timeInSeconds * 1000);
} catch (Throwable throwable) {
// In case throw exception do nothing
}
}
// This method to apply wait until element be ready
public static void waitUntil(String selector) {
// Call SeleniumActions.waitUntil method
SeleniumActions.waitUntil(selector);
}
// This method to apply sendKeys action
public static void sendKeys(String selector, CharSequence... keyboardKeys) {
// Call SeleniumActions.sendKeys method
SeleniumActions.sendKeys(selector, keyboardKeys);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy