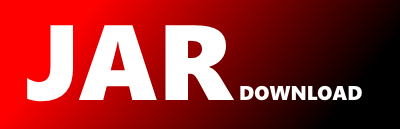
automated.test_manage.actions.locator.Element Maven / Gradle / Ivy
The newest version!
package automated.test_manage.actions.locator;
import automated.core.enums.TimeEnum;
import automated.process.io.read.ReadPropFile;
import automated.reporting.TestReporter;
import automated.test_manage.config.Platform;
import automated.test_manage.run.TestCaseFiles;
import org.openqa.selenium.*;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.FluentWait;
import java.util.concurrent.TimeUnit;
import static automated.test_manage.actions.locator.Selector.getSelector;
/**
* Created by Ismail on 12/25/2017.
* This class generate and return WebElement as object
*/
public class Element {
/*************** Class Variables Section ***************/
// Initialize WebElement as static variable
public static WebElement element = null;
// This variable to save old WebElement border color
private static String elementOldBorderColor = "white";
// This variable to save old WebElement border width
private static String elementOldBorderWidth = "1px";
// This variable to save old WebElement border style
private static String elementOldBorderStyle = "solid";
/*************** Class Methods Section ***************/
// This method to return WebElement object from findElement method
// This method is accessible from any method on framework level
// In case you have a generic action need to be apply on
// the WebElement before send it to methods
public static WebElement getElement(String selector) {
// Remove border highlight from previous element
// Check if element isn't null
if (element != null)
// Remove red border color
((JavascriptExecutor) Platform.driver).executeScript(
"$(arguments[0]).css({'border-width':'" +
elementOldBorderWidth + "'," +
"'border-style':'" + elementOldBorderStyle +
"','border-color':'" + elementOldBorderColor +
"'})", element);
// Save WebElement from findElement method to variable
element = findElement(selector);
// Save WebElement border style, this to remove border style
// that applied once selenium finish apply actions on WebElement
elementOldBorderColor =
element.getCssValue("border-bottom-color");
elementOldBorderWidth =
element.getCssValue("border-width");
elementOldBorderStyle =
element.getCssValue("border-style");
// Highlight the WebElement before sending any action
// This code will circle the element with red border
// to notify tester that the element is current active
// To change color style you can change javaScript sentence
// $(arguments[0]).css({'border-width':'2px','border-style':'solid',
// 'border-color':'red'});
// arguments[0].style.borderColor = '#FF0000'
((JavascriptExecutor) Platform.driver).executeScript(
"$(arguments[0]).css({'border-width':'2px','border-style'" +
":'solid','border-color':'red'})", element);
// Return WebElement variable
return element;
}
// This method to return WebElement object from String parameter
private static WebElement findElement(String selector) {
// If selectorsFile not empty and selectors saved
// to file then will return selector value from the file
String selectorsFile = TestCaseFiles.getTestLocatorsFile();
if (selectorsFile != null)// Check if selectorsFile contains value
if (!ReadPropFile.getProperty(selectorsFile, selector).isEmpty())
// If yes then will retrieve a new selector value
selector = ReadPropFile.getProperty(selectorsFile, selector);
// If selector isn't exist in Prop File then
// Select Element and save to element static variable
return element = findElement(getSelector(selector));
}
// This method to return WebElement object from By parameter
private static WebElement findElement(By selectorBy) {
try {// FluentWait to wait the element till be visible on the page
// Then select the element and return as WebElement object
element = (new FluentWait<>(Platform.driver).
withTimeout(TimeEnum.EXPLICIT_WAIT_TIMEOUT.value(),
TimeUnit.SECONDS).
pollingEvery(1, TimeUnit.SECONDS)
.ignoring(StaleElementReferenceException.class,
NoSuchElementException.class)
.until(ExpectedConditions.
visibilityOfElementLocated(selectorBy)));
if (Platform.platform.equalsIgnoreCase("web"))
// Move to element location on web page
moveToElement(element);
// Return element value
return element;
} catch (Throwable throwable) {// If something went wrong
TestReporter.fail("Unable to find the element, " +
"Please check message: " + throwable.getMessage(), true);
}
return null;
}
// This method move to WebElement location on web page
private static void moveToElement(WebElement webElement) {
new Actions(Platform.driver).moveToElement(webElement).build().perform();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy