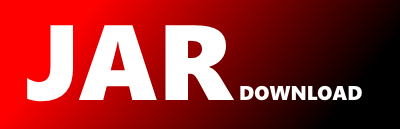
automated.test_manage.actions.locator.Selector Maven / Gradle / Ivy
The newest version!
package automated.test_manage.actions.locator;
import automated.reporting.TestReporter;
import org.openqa.selenium.By;
import org.testng.Assert;
/**
* Created by Ismail on 12/27/2017.
* This class contains generate Selector Type as By object
*/
public class Selector {
/*************** Class Methods Section ***************/
// This method to build By(selector type) for element
// and return By object to be used in finding WebElement
public static By getSelector(String selector) {
// Define selector splitter between selector type and value
String splitter = "=";
// Define String format length after splitting
int selectorSplitLength = 2;
//Check String format is correct
try {
Assert.assertTrue(selector.split(splitter).length >= selectorSplitLength, "The provided Selector string format is invalid, it should be (selectorType=selectorValue), Please check selector string: " + selector);
} catch (Throwable throwable) {
// If fail will add step to report and fail test case
TestReporter.error(throwable.getMessage() +
"\nOR maybe you forget to add selectorsFile", true);
}
// Define Select Type and Value
String selectorType, selectorValue;
// Check if Selector String split length more than 2
if (selector.split(splitter).length > 2) {
// Extract Type as always will be first index
selectorType = selector.split(splitter)[0];
// get all other indexes to be Selector Value
// by removing selectorType string and = from all string
// selector string so in this way will get remaining as value
selectorValue = selector.replace(selectorType + "=", "");
} else {
// Extract Type and value from string if length just 2
selectorType = selector.split(splitter)[0];
selectorValue = selector.split(splitter)[1];
}
// Return By object of provided selector
return getSelector(selectorType, selectorValue);
}
// This method to build By(selector type) for element
// and return By object to be used in finding WebElement
public static By getSelector(String selectorType, String selectorValue) {
// Define selector By
By selectorBy = null;
// Check selector type and build By object according
switch (selectorType.toLowerCase()) {
case "id": {
selectorBy = By.id(selectorValue);
break;
}
case "name": {
selectorBy = By.name(selectorValue);
break;
}
case "class":
case "classname": {
selectorBy = By.className(selectorValue);
break;
}
case "css":
case "cssselector":
case "selector": {
selectorBy = By.cssSelector(selectorValue);
break;
}
case "linktext": {
selectorBy = By.linkText(selectorValue);
break;
}
case "partiallinktext": {
selectorBy = By.partialLinkText(selectorValue);
break;
}
case "tagname": {
selectorBy = By.tagName(selectorValue);
break;
}
case "xpath": {
selectorBy = By.xpath(selectorValue);
break;
}
default: {// If selector type is wrong will fail test case
TestReporter.fail("The provided Selector Type" +
" isn't exist, Please check Selector Type is: "
+ selectorType, true);
}
}// Here return the result as By object
return selectorBy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy