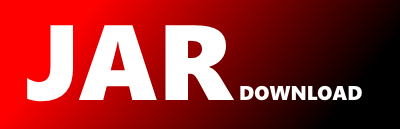
automated.test_manage.config.ConfigMethods Maven / Gradle / Ivy
The newest version!
package automated.test_manage.config;
import automated.core.annotations.AttributesConfig;
import automated.core.annotations.ClassAttributes;
import automated.core.annotations.MethodAttributes;
import automated.platforms.mobile.RunMobileDriver;
import automated.platforms.web.RunWebDriver;
import automated.test_manage.run.TestCaseFiles;
import org.testng.ITestContext;
import org.testng.ITestResult;
import org.testng.annotations.*;
import automated.reporting.TestReporter;
import java.lang.reflect.Method;
/**
* Created by Ismail on 12/25/2017.
* This class contains all configuration methods related to TestNG
*/
public class ConfigMethods {
/*************** Class Variables Section ***************/
AttributesConfig attributesConfig = new AttributesConfig();
/*************** Class Methods Section ***************/
// This method run automatically before each TestNG Suite
// AlwaysRun means should be run(no exclude for any reason)
@BeforeSuite(alwaysRun = true)
public void setUpSuite(ITestContext testContext) {
// Create and Start report and log test run status
TestReporter.prepareReport(testContext);
}
// This method run automatically after each TestNG Suite
// AlwaysRun means should be run(no exclude for any reason)
@AfterSuite(alwaysRun = true)
public void tearDownSuite() {
// Save all report to report file and close the file
TestReporter.generateFinalReport();
}
// This method run automatically before each TestNG Groups
// AlwaysRun means should be run(no exclude for any reason)
@BeforeGroups(alwaysRun = true)
public void setUpGroups() {
}
// This method run automatically after each TestNG Groups
// AlwaysRun means should be run(no exclude for any reason)
@AfterGroups(alwaysRun = true)
public void tearDownGroups() {
}
// This method run automatically before each TestNG Test
// AlwaysRun means should be run(no exclude for any reason)
@BeforeTest(alwaysRun = true)
public void setUpTest(ITestContext testContext) {
// Prepare Test Report
// Start logging Test case status in report
TestReporter.prepareTestReport(testContext);
// Check Test Platform to initialize correct WebDriver
switch (Platform.platform.toLowerCase()) {
// If Platform Web then will start initialize for Web
case "web": {
// If browser Parameter provided from
// TestNG File then Save it to static
// variable browser from Platform class
if (testContext.getSuite().getParameter(Platform.browserParam) != null)
Platform.browser = testContext.getSuite().
getParameter(Platform.browserParam);
// If baseUrl Parameter provided from TestNG File then
// Save it to static variable baseUrl from Platform class
if (testContext.getSuite().getParameter(Platform.baseUrlParam) != null)
Platform.baseUrl = testContext.getSuite().
getParameter(Platform.baseUrlParam);
RunWebDriver.setUp();// Start WebDriver as Web Application
break;
}
case "mobile": {// If Platform Mobile then will start initialize for Mobile
// If Mobile Parameter provided from TestNG File then
// Save it to static variable mobile from Platform class
if (testContext.getSuite().getParameter(Platform.mobileParam) != null)
Platform.mobile = testContext.getSuite().getParameter(Platform.mobileParam);
// If Virtual Mobile Parameter provided from TestNG File then
// Save it to static variable virtualMobile from Platform class
if (testContext.getSuite().getParameter(Platform.virtualMobileParam) != null)
Platform.virtualMobile = testContext.getSuite().
getParameter(Platform.virtualMobileParam);
// Start WebDriver as Web Application
RunMobileDriver.setUp();
break;
}
default: {// If not match any case then will add error to report
// then fail Test case
TestReporter.error("Something went wrong initializing" +
" Platform, check Platform provided: "
+ Platform.platform, true);
}
}
}
// This method run automatically after each TestNG Test
// AlwaysRun means should be run(no exclude for any reason)
@AfterTest(alwaysRun = true)
public void tearDownTest() {// Check current running Platform
switch (Platform.platform.toLowerCase()) {
case "web": {// If web then call close WebDriver for Web applications
RunWebDriver.tearDown();
break;
}
case "mobile": {// If web then call close WebDriver for Mobile applications
RunMobileDriver.tearDown();
break;
}
default:// Here nothing to do
}
/******************** Method Report Section Start ********************/
// Generate Test Report and write to full report
TestReporter.generateTestReport();
/******************** Method Report Section End ********************/
}
// This method run automatically before each TestNG Class
// AlwaysRun means should be run(no exclude for any reason)
@BeforeClass(alwaysRun = true)
public void setUpClass() {
/******************** Test Custom Annotation Section Start ********************/
// Retrieve Test attributes from @ClassAttributes annotation
// Define all test attribute variables
String testLocatorsFile = "", testDataFile = "", baseURL = "";
// Check if Annotation class is defined then save to object
ClassAttributes classAttributes = null;
if (this.getClass().getAnnotation(ClassAttributes.class) != null)
classAttributes = this.getClass().getAnnotation(ClassAttributes.class);
// Retrieve all related test attribute values
if (classAttributes != null) {
testLocatorsFile = attributesConfig.getLocatorsFile(classAttributes);
testDataFile = attributesConfig.getDataFile(classAttributes);
baseURL = attributesConfig.getBaseURL(classAttributes);
}
// Save testLocatorsFile
// Check if test locators file isn't empty to assign
if (!testLocatorsFile.isEmpty())
TestCaseFiles.setTestLocatorsFile(testLocatorsFile);
// Save testDataFile
// Check if test data file isn't empty to assign
if (!testDataFile.isEmpty())
TestCaseFiles.setTestDataFile(testDataFile);
// Save Project BaseURL to global variable BaseURL
// in case baseURL isn't already provided
if (Platform.baseUrl.isEmpty())
if (!baseURL.isEmpty()) // Check if baseURL isn't empty to assign
Platform.baseUrl = baseURL;
/******************** Test Custom Annotation Section End ********************/
}
// This method run automatically after each TestNG Class
// AlwaysRun means should be run(no exclude for any reason)
@AfterClass(alwaysRun = true)
public void tearDownClass() {
// Reset all old class files by calling TestCaseFiles.resetTestFilesValues
TestCaseFiles.resetTestFilesValues();
}
// This method run automatically before each TestNG Method
// AlwaysRun means should be run(no exclude for any reason)
@BeforeMethod(alwaysRun = true)
public void setUpMethod(ITestContext testContext, Method method) {
/******************** Method Custom Annotation Section Start ********************/
// Retrieve Method attributes from @ClassAttributes annotation
// Define all method attribute variables
int dataRow = -1, dataColumns[] = {};
// Check if Annotation class is defined then save to object
MethodAttributes methodAttributes = null;
try {
if (this.getClass().getMethod(method.getName()).getAnnotation(MethodAttributes.class) != null)
methodAttributes = this.getClass().getMethod(method.getName()).getAnnotation(MethodAttributes.class);
} catch (NoSuchMethodException e) {
TestReporter.error("Warning: Something went wrong while define method attributes",
false);
}
// Retrieve all related test attribute values
if (methodAttributes != null) {
dataRow = attributesConfig.getDataRow(methodAttributes);
dataColumns = attributesConfig.getDataColumns(methodAttributes);
}
// Save dataRow
// Check if method data row isn't equal -1 to assign
if (dataRow != -1)
TestCaseFiles.setDataRow(TestCaseFiles.getTestCaseDataRow(dataRow));
// Save dataColumns
// Check if method data columns isn't empty to assign
// This under implementation
if (dataColumns.length != 0)
;
/******************** Method Custom Annotation Section End ********************/
/******************** Method Report Section Start ********************/
// Start logging Test case status in report
TestReporter.prepareMethodReport(method);
// Add Categories of Test Case to report
TestReporter.addMethodCategories(testContext, method);
/******************** Method Report Section End ********************/
}
// This method run automatically after each TestNG Method
// AlwaysRun means should be run(no exclude for any reason)
@AfterMethod(alwaysRun = true)
public void tearDownMethod(ITestResult testResult) {
/*************** Method Variables Reset Section Start ***************/
// Reset Method Variables values by calling TestCaseFiles.resetTestDataValues
TestCaseFiles.resetTestDataValues();
/*************** Method Variables Reset Section End ***************/
/******************** Method Report Section Start ********************/
// Provide a screenShot when Test case fail and add to report
TakeScreenShot.captureScreenshotOnFail(testResult);
// Generate Test Case Report and write to full report
TestReporter.generateMethodReport();
/******************** Method Report Section End ********************/
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy