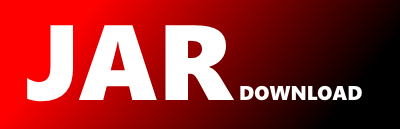
automated.test_manage.run.TestCaseFiles Maven / Gradle / Ivy
The newest version!
package automated.test_manage.run;
import automated.process.io.read.ReadFile;
import automated.process.io.read.ReadPropFile;
import automated.process.io.read.ReadTXTFile;
import automated.reporting.TestReporter;
/**
* Created by Ismail on 12/26/2017.
* This class contains all related methods to read/write related
* Test Case Files and do required and suitable implementation
*/
public class TestCaseFiles {
/*************** Class Variables Section ***************/
// Define static testLocatorsFile variable to save Path of the file
private static String testLocatorsFile = null;
// Define static testDataFile variable to save Path of the file
private static String testDataFile = null;
// Define static Data Row variable to save String comes
// from getTestCaseDataRow method
private static String dataRow = null;
/*************** Class Methods Section ***************/
// This method return Path of test file provided in testLocatorsFile
public static String getTestLocatorsFile() {
return TestCaseFiles.testLocatorsFile;
}
// This method return Path of test data provided in getTestDataFile
public static String getTestDataFile() {
return TestCaseFiles.testDataFile;
}
// This method Set Path of test file provided inside testLocatorsFile
public static void setTestLocatorsFile(String fileName) {
// Reset Selector File Value in case send null param
if (fileName == null)
TestCaseFiles.testLocatorsFile = null;
else {
// Save Absolute File Path from File name to testLocatorsFile
// Call getFileAbsolutePath Method from ReadFile Class
TestCaseFiles.testLocatorsFile = ReadFile.getFileAbsolutePath(fileName);
}
}
// This method Set Path of test file provided inside testDataFile
public static void setTestDataFile(String fileName) {
// Reset Data File Value in case send null param
if (fileName == null)
TestCaseFiles.testDataFile = null;
else {
// Save Absolute File Path from File name to testDataFile
// Call getFileAbsolutePath Method from ReadFile Class
TestCaseFiles.testDataFile = ReadFile.getFileAbsolutePath(fileName);
}
}
// This method to return a Property from Test case File as String
public static String getTestCaseProperty(String propertyName) {
if (getTestLocatorsFile() != null)// Verify TestCase File not null
if (!getTestLocatorsFile().isEmpty())// Verify TestCase File not empty
// Verify Property name has value then return the value
if (ReadPropFile.getProperty(getTestLocatorsFile(), propertyName) != null)
return ReadPropFile.getProperty(getTestLocatorsFile(), propertyName);
else
TestReporter.error("Unable to find property with name " + propertyName
+ "inside test case locators file " + getTestLocatorsFile(), false);
return "";// Default return empty String
}
// This method to return a DataRow from Test data File as String
public static String getTestCaseDataRow(int rowNumber) {
if (getTestDataFile() != null) {// Verify TestCase File not null
if (!getTestDataFile().isEmpty())// Verify TestCase File not empty
return ReadTXTFile.getRowOfTxtFile(getTestDataFile(), rowNumber);
} else
TestReporter.error("Test Data File has no data or " +
"incorrect path, please check: " + getTestDataFile() +
"
null means file path isn't exist...", true);
return "";// Default return null
}
// This method to set dataRow variable value
public static void setDataRow(String dataRow) {
TestCaseFiles.dataRow = dataRow;
}
// This method to return dataRow variable value
public static String getDataRow() {
return TestCaseFiles.dataRow;
}
// This method to retrieve first value of current DataRow
public static String getValueOfDataRow() {
// Check data row isn't empty to continue processing
if (dataRow.isEmpty())
TestReporter.error("DataRow has no data, Please check if you provide method DataRow attribute or provided Row number has data", true);
// Split all data row by comma , then save first value
// from the array to a variable
String dataRowValue = getDataRow().split(",")[0];
// remove from DataRow the value we are going to use
String newDataRow = getDataRow().replaceFirst(dataRowValue, "");
// Check if newDataRow startWith comma to remove it
if (newDataRow.startsWith(","))
newDataRow = newDataRow.replaceFirst(",", "");
// Replace newDataRow with old one
setDataRow(newDataRow);
// Return first value of data row
return dataRowValue;
}
// This method to reset all test case data variables
public static void resetTestDataValues() {
// Assign null value for dataRow variable to remove
// old test case data
TestCaseFiles.dataRow = null;
}
// This method to reset all test case files variables
public static void resetTestFilesValues() {
// Assign null value for testLocatorsFile
TestCaseFiles.testLocatorsFile = null;
// Assign null value for testDataFile
TestCaseFiles.testDataFile = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy