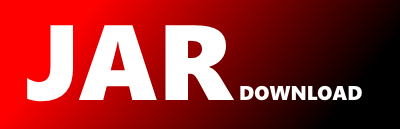
web.Assert Maven / Gradle / Ivy
package web;
import core.reports.TestReporter;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import static core.asserts.AssertString.assertEqualStrings;
import static web.ExpectedConditionsHandler.fluentWait;
import static web.ExpectedConditionsHandler.isWebElementExists;
import static web.ExpectedConditionsHandler.validate;
import static web.TakeScreenShot.captureScreenshotOnStep;
import static web.WebElementHandler.getElement;
import static web.WebActions.isWebElementDisplayed;
/**
* Created by Ismail on 5/30/2018.
* This class contains all assertion methods related to Web test automation
* When assertion fail then web test case will fail
*/
public class Assert {
/*************** Class Methods Section ***************/
// This method check if condition is true
public static void isTrue(Boolean condition, String errMessage) {
try {
org.testng.Assert.assertTrue(condition, errMessage);
} catch (Throwable throwable) {
TestReporter.fail(errMessage, true);
}
}
// This method check if condition is true
public static void isFalse(Boolean condition, String errMessage) {
try {
org.testng.Assert.assertFalse(condition, errMessage);
} catch (Throwable throwable) {
TestReporter.fail(errMessage, true);
}
}
// This method check if condition is null
public static void isNull(Object condition, String errMessage) {
try {
org.testng.Assert.assertNull(condition, errMessage);
} catch (Throwable throwable) {
TestReporter.fail(errMessage, true);
}
}
// This method check if condition is not null
public static void isNotNull(Object condition, String errMessage) {
try {
org.testng.Assert.assertNotNull(condition, errMessage);
} catch (Throwable throwable) {
TestReporter.fail(errMessage, true);
}
}
// This method check current title of current web page from active session
public static void titleIs(String expectedTitle, String errMessage) {
isTrue(validate(WebDriverInitializer.webDriver, expectedTitle, "titleIs"), errMessage);
// Report step to reporter
TestReporter.pass("Current Title is correct: " + expectedTitle, captureScreenshotOnStep());
}
// This method check if url contains a String
public static void titleContains(WebDriver webDriver, String expectedContains, String errMessage) {
isTrue(validate(webDriver, expectedContains, "titleContains"), errMessage);
// Report step to reporter
TestReporter.pass("Current Title contains: " + expectedContains, captureScreenshotOnStep());
}
// This method check current URL of current web page from active session
public static void urlIs(String expectedURL, String errMessage) {
isTrue(validate(WebDriverInitializer.webDriver, expectedURL, "urlIs"), errMessage);
// Report step to reporter
TestReporter.pass("Current URL is correct: " + expectedURL, captureScreenshotOnStep());
}
// This method check if url contains a String
public static void urlContains(WebDriver webDriver, String expectedContains, String errMessage) {
isTrue(validate(webDriver, expectedContains, "urlContains"), errMessage);
// Report step to reporter
TestReporter.pass("Current URL contains: " + expectedContains, captureScreenshotOnStep());
}
// This method check two strings provided as method params
public static void stringsEqual(String actual, String expected, String errMessage) {
// Call assertEqualStrings method from StringAssertions class
assertEqualStrings(actual, expected, errMessage);
// If assertion passed then will write step passed in report
TestReporter.pass("The Provided Strings are equals, actual: " + actual + " and Expected: " + expected, captureScreenshotOnStep());
}
// This method check if WebElement displayed on current web page of String parameter
public static void isDisplayed(String webElement, String errMessage) {
isTrue(isWebElementDisplayed(webElement), errMessage);
}
// This method check if WebElement displayed on current web page of WebElement parameter
public static void isDisplayed(WebElement webElement, String errMessage) {
isTrue(isWebElementDisplayed(webElement), errMessage);
}
// This method check if WebElement exists on current web page for String parameter
public static void isExists(WebDriver webDriver, String webElement, String errMessage) {
isTrue(isWebElementExists(webDriver, webElement), errMessage);
}
// This method check if Alert is present or not
public static void alertIsPresent(WebDriver webDriver, String errMessage) {
isNotNull(fluentWait(webDriver, "alertIsPresent"), errMessage);
}
// This method check if WebElement attribute value for String parameter
public static void webElementAttributeIs(WebDriver webDriver, String webElement, String attributeName, String attributeValue, String errMessage) {
webElementAttributeIs(webDriver, getElement(webElement), attributeName, attributeValue, errMessage);
}
// This method check if WebElement attribute value for WebElement parameter
public static void webElementAttributeIs(WebDriver webDriver, WebElement webElement, String attributeName, String attributeValue, String errMessage) {
isTrue((Boolean) fluentWait(webDriver, "attributeIs", webElement, attributeName, attributeValue), errMessage);
}
// This method check if WebElement attribute contains in value for String parameter
public static void webElementAttributeContains(WebDriver webDriver, String webElement, String attributeName, String attributeContainsValue, String errMessage) {
webElementAttributeContains(webDriver, getElement(webElement), attributeName, attributeContainsValue, errMessage);
}
// This method check if WebElement attribute contains in value for WebElement parameter
public static void webElementAttributeContains(WebDriver webDriver, WebElement webElement, String attributeName, String attributeContainsValue, String errMessage) {
isTrue((Boolean) fluentWait(webDriver, "attributeContains", webElement, attributeName, attributeContainsValue), errMessage);
}
// This method check if WebElement attribute not empty for String parameter
public static void webElementAttributeNotEmpty(WebDriver webDriver, String webElement, String attributeName, String errMessage) {
webElementAttributeNotEmpty(webDriver, getElement(webElement), attributeName, errMessage);
}
// This method check if WebElement attribute not empty for WebElement parameter
public static void webElementAttributeNotEmpty(WebDriver webDriver, WebElement webElement, String attributeName, String errMessage) {
isTrue((Boolean) fluentWait(webDriver, "attributeNotEmpty", webElement, attributeName), errMessage);
}
// This method check if WebElement contains text for String parameter
public static void webElementContainsText(WebDriver webDriver, String webElement, String containsText, String errMessage) {
webElementContainsText(webDriver, getElement(webElement), containsText, errMessage);
}
// This method check if WebElement contains text for WebElement parameter
public static void webElementContainsText(WebDriver webDriver, WebElement webElement, String containsText, String errMessage) {
isTrue((Boolean) fluentWait(webDriver, "attributeNotEmpty", webElement, containsText), errMessage);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy