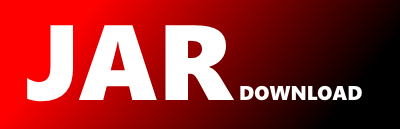
web.ExpectedConditionsHandler Maven / Gradle / Ivy
package web;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.testng.Assert;
import java.util.concurrent.TimeUnit;
import static web.ByHandler.getSelector;
/**
* Created by Ismail on 6/2/2018.
* This class contains all methods related to validate any ExpectedConditions of Selenium
* and return the condition value
*/
public class ExpectedConditionsHandler extends WebElementHelper {
/*************** Class Variables Section ***************/
/*************** Class Methods Section ***************/
// This method validate some functions for specific expected condition
public static Boolean validate(WebDriver webDriver, String expectedResult, String URLorTitleIsOrContainsOrMatch) {
switch (URLorTitleIsOrContainsOrMatch.toLowerCase()) {
case "titleis": {
return (Boolean) fluentWait(webDriver, ExpectedConditions.titleIs(expectedResult));
}
case "titlecontains": {
return (Boolean) fluentWait(webDriver, ExpectedConditions.titleContains(expectedResult));
}
case "urltobe":
case "urlis": {
return (Boolean) fluentWait(webDriver, ExpectedConditions.urlToBe(expectedResult));
}
case "urlcontains": {
return (Boolean) fluentWait(webDriver, ExpectedConditions.urlContains(expectedResult));
}
case "urlmatches": {
return (Boolean) fluentWait(webDriver, ExpectedConditions.urlMatches(expectedResult));
}
default:
Assert.fail("You provided wrong expected Condition parameter in fluentWait method.");
}
return false;
}
// This method validate WebElement is exist or not for String parameter
public static Boolean isWebElementExists(WebDriver webDriver, String webElement) {
webDriver.manage().timeouts().implicitlyWait(0, TimeUnit.MILLISECONDS);
boolean exists = !webDriver.findElements(getSelector(webElement)).isEmpty() ? true : false;
webDriver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS);
return exists;
}
// This method is a fluent wait that returns WebElement object for specific expected condition
public static Object fluentWait(WebDriver webDriver, String expectedCondition, Object... parameters) {
switch (expectedCondition.toLowerCase()) {
case "alertispresent": {
return fluentWait(webDriver, ExpectedConditions.alertIsPresent());
}
case "attributetobe":
case "attributeis": {
return fluentWait(webDriver, ExpectedConditions.attributeToBe((WebElement) parameters[0], (String) parameters[1], (String) parameters[2]));
}
case "attributecontains": {
return fluentWait(webDriver, ExpectedConditions.attributeContains((WebElement) parameters[0], (String) parameters[1], (String) parameters[2]));
}
case "attributenotempty": {
return fluentWait(webDriver, ExpectedConditions.attributeToBeNotEmpty((WebElement) parameters[0], (String) parameters[1]));
}
case "texttobepresentinelement":
case "textinsideelemenet": {
return fluentWait(webDriver, ExpectedConditions.textToBePresentInElement((WebElement) parameters[0], (String) parameters[1]));
}
default:
Assert.fail("You provided wrong expected Condition parameter in fluentWait method.");
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy