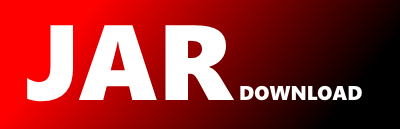
web.TestNGMethods Maven / Gradle / Ivy
package web;
import core.annotations.AttributesConfig;
import core.annotations.ClassAttributes;
import org.testng.ITestContext;
import org.testng.ITestResult;
import org.testng.annotations.*;
import java.lang.reflect.Method;
import static web.WebDriverInitializer.*;
import static web.WebPageObject.removeElements;
/**
* Created by Ismail on 5/30/2018.
*/
public class TestNGMethods extends core.TestNGMethods {
/*************** Class Variables Section ***************/
AttributesConfig attributesConfig = new AttributesConfig();
/*************** Class Methods Section ***************/
// This method run automatically before each web TestNG Suite
@BeforeSuite(alwaysRun = true)
public void setUpSuite(ITestContext testContext) {
// Run Super class method
super.setUpSuite(testContext);
}
// This method run automatically after each web TestNG Suite
@AfterSuite(alwaysRun = true)
public void tearDownSuite() {
// Run Super class method
super.tearDownSuite();
}
// This method run automatically before each Web TestNG Test
@BeforeTest(alwaysRun = true)
public void setUpTest(ITestContext testContext) {
// Run Super class method
super.setUpTest(testContext);
// Save params and start WebDriver
// Save browser from testNG file to browser variable
browser = testContext.getSuite().getParameter(browserParam) != null ? testContext.getSuite().getParameter(browserParam).toLowerCase() : "chrome";
// Save baseUrl from testNG file to baseUrl variable
baseUrl = testContext.getSuite().getParameter(baseUrlParam) != null ? testContext.getSuite().getParameter(baseUrlParam) : "";
// Start WebDriver as Web Application
WebDriverInitializer.setUpWebDriver();
}
// This method run automatically after each Web TestNG Test
@AfterTest(alwaysRun = true)
public void tearDownTest() {
// Call close WebDriver for Web applications
WebDriverInitializer.tearDownWebDriver();
// Run Super class method
super.tearDownTest();
}
// This method run automatically before each Web TestNG Class
@BeforeClass(alwaysRun = true)
public void setUpClass() {
// Run Super class method
super.setUpClass();
/******************** Test Custom Annotation Section Start ********************/
// Retrieve baseURL Test attribute from @ClassAttributes annotation
String baseURL = "";
// Check if Annotation class is defined then save to object
ClassAttributes classAttributes = null;
if (this.getClass().getAnnotation(ClassAttributes.class) != null)
classAttributes = this.getClass().getAnnotation(ClassAttributes.class);
// Retrieve baseURL attribute values
if (classAttributes != null) {
baseURL = attributesConfig.getBaseURL(classAttributes);
}
// Save Project BaseURL to global variable BaseURL in case baseURL isn't already provided
if (baseUrl.isEmpty())
// Check if baseURL isn't empty the save to static variable and navigate browser to URL
if (!baseURL.isEmpty()) {
baseUrl = baseURL;
WebActions.navigateTo(webDriver, baseURL);
}
/******************** Test Custom Annotation Section End ********************/
// Run initElements for WebPageObject in case user using Page Object Model
if (WebPageObject.childClass.size() > 0) {
WebPageObject.initElements();
}
}
// This method run automatically after each web TestNG Class
@AfterClass(alwaysRun = true)
public void tearDownClass() {
// Run Super class method
super.tearDownClass();
// Remove WebPageObjects in case user using Page Object Model
if (WebPageObject.childClass.size() > 0) {
removeElements();
}
}
// This method run automatically before each web TestNG Method
@BeforeMethod(alwaysRun = true)
public void setUpMethod(ITestContext testContext, Method method) {
// Run Super class method
super.setUpMethod(testContext, method);
}
// This method run automatically after each Web TestNG Method
@AfterMethod(alwaysRun = true)
public void tearDownMethod(ITestResult testResult) {
/******************** Method Report Section Start ********************/
// Provide a screenShot when Test case fail and add to report
TakeScreenShot.captureScreenshotOnFail(testResult);
/******************** Method Report Section End ********************/
/******************** Method Reset vars Section Start ********************/
WebElementHandler.element = null;
/******************** Method Reset vars Section End ********************/
// Run Super class method
super.tearDownMethod(testResult);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy