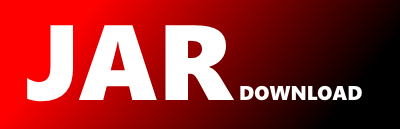
web.WebActions Maven / Gradle / Ivy
package web;
import core.annotations.AnnotationsHandler;
import core.files.StringHandler;
import core.reports.TestReporter;
import org.openqa.selenium.*;
import org.testng.Assert;
import static core.internal.EnvironmentInterface.SLEEP_TIMEOUT;
import static web.TakeScreenShot.captureScreenshotOnStep;
/**
* Created by Ismail on 12/27/2017.
* This class contains code implementation of selenium actions
*/
public class WebActions extends WebElementHandler {
/*************** Class Variables Section ***************/
// This variable to save error message if action fail to execute
private static String errMessage = null;
/*************** Class Methods Section ***************/
// This method to log step if passed or failed on test report
private static void reportStepStatus(String passMessage, String errMessage, String screenShot, Boolean failTestCase) {
if (errMessage != null) {
// Save error message step
String errReport = "Something went wrong, Please check message: " + errMessage;
// Report step log to reporter
TestReporter.fail(errReport, failTestCase);
} else
TestReporter.pass(passMessage, screenShot);
}
/*************** WebElement related Actions Section Start ***************/
// This method to perform click action for String parameter
public static void click(String webElement) {
click(getElement(webElement));
}
// This method to perform click action for WebElement parameter
public static void click(WebElement webElement) {
// Try to getElement from WebElementHandler.getElement method then send click action the element
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement).click();
// If success then no errors
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// If keep throwing an error and finish all tries then will fail the action
if (errMessage != null) {
// Save error message step
String errReport = "Unable to click element, Error message: " + errMessage;
// Reset errMessage variable
errMessage = null;
// If fail report a step as fail with fail test case
TestReporter.fail(errReport, true);
}
// If pass report step as pass
TestReporter.pass("Element has been clicked", captureScreenshotOnStep());
}
// This method to perform sendText action for String parameter
public static void sendText(String webElement, String text) {
//Try to send Text to Element
sendText(getElement(webElement), text);
}
// This method to perform sendText action for WebElement parameter
public static void sendText(WebElement webElement, String text) {
// Try to getElement from WebElementHandler.getElement method Then will send text to the element
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement).sendKeys(text);
// If success then reset errMessage
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// If keep throwing an error and finish all tries then will fail the action
if (errMessage != null) {
// Save error message step
String errReport = "Unable to type in element, Please check message: " + errMessage;
// Reset errMessage variable
errMessage = null;
// If fail report step as fail with fail test case
TestReporter.fail(errReport, true);
}
// If pass report step as pass
TestReporter.pass("(" + text + ") Text is sent " + "to the element.", captureScreenshotOnStep());
}
// This method to perform Send Key from Keyboard action for String parameter
public static void sendKeyboardKeys(String webElement, Keys key) {
sendKeyboardKeys(getElement(webElement), key);
}
// This method to perform Send Key from Keyboard action for WebElement parameter
public static void sendKeyboardKeys(WebElement webElement, Keys key) {
// Try to getElement from WebElementHandler.getElement method Then will send all provided keys to the element
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement).sendKeys(key);
// If success then no errors
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// If keep throwing an error and finish all tries then will fail the action
if (errMessage != null) {
// Save error message step
String errReport = "Something went wrong in sending Keys, Please check log: " + errMessage;
// Reset errMessage variable
errMessage = null;
// If fail report step as fail with fail test case
TestReporter.fail(errReport, true);
}
// If pass report step as pass
TestReporter.pass("Keyboard Keys are sent", captureScreenshotOnStep());
}
// This method to perform Get Text from element action for String parameter
public static String getText(String webElement) {
return getText(getElement(webElement));
}
// This method to perform Get Text from element action for WebElement parameter
public static String getText(WebElement webElement) {
// Define String to save WebElementHandler text
String text = null;
// Try to getElement from WebElementHandler.getElement method Then will get Text from the element
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement);
// Try to get Text from Element with getText
text = !element.getText().isEmpty() ? element.getText() : null;
// If text variable null use getAttribute to get Text
if (text == null)
text = !element.getAttribute("value").isEmpty() ? element.getAttribute("value") : null;
// If text variable null use getAttribute to get Text
if (text == null)
text = !element.getAttribute("value").isEmpty() ? element.getAttribute("value") : null;
// If success then no errors
errMessage = null;
// If success leave for-loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// If keep throwing an error and finish all tries then will fail the action
if (errMessage != null) {
// Save error message step
String errReport = "Unable to get Text from element, Please check message: " + errMessage;
// Reset errMessage variable
errMessage = null;
// If fail report step as fail with fail test case
TestReporter.fail(errReport, true);
}
// If pass report step as pass
TestReporter.pass("(" + text + ") Text is extracted from the element.", captureScreenshotOnStep());
// Return Text Value
return text;
}
// This method to perform wait until element be ready for String parameter
public static void waitUntil(String webElement) {
waitUntil(getElement(webElement));
}
// This method to perform wait until element be ready for WebElement parameter
public static void waitUntil(WebElement webElement) {
try {
// Call WebElementHandler.getElement and check if element founded and everything working fine
if (moveToElement(webElement).getClass().isInstance(WebElement.class)) {
// If true report step as pass
TestReporter.pass("waitUntil action found the WebElement.", captureScreenshotOnStep());
} else {
Assert.fail("waitUntil action can't find WebElement");
}
} catch (Throwable throwable) {
// If something went wrong
TestReporter.fail("WaitUntil action failed due to: " + throwable.getMessage(), true);
}
}
// This method to perform sendKeys action for String parameter
public static void sendKeys(String webElement, CharSequence... keyboardKeys) {
sendKeys(getElement(webElement), keyboardKeys);
}
// This method to perform sendKeys action for WebElement parameter
public static void sendKeys(WebElement webElement, CharSequence... keyboardKeys) {
// Try to getElement from WebElementHandler.getElement method Then will send keys to the element
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement).sendKeys(keyboardKeys);
// If success then no errors
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// If keep throwing an error and finish all tries then will fail the action
if (errMessage != null) {
// Save error message step
String errReport = "Unable to enter keys in element, Please check message: " + errMessage;
// Reset errMessage variable
errMessage = null;
// If fail report step as fail with fail test case
TestReporter.fail(errReport, true);
}
// If pass report step as pass
TestReporter.pass("(" + keyboardKeys + ") Text is sent " + "to the element.", captureScreenshotOnStep());
}
// This method to perform clean action for String parameter
public static void clear(String webElement) {
clear(getElement(webElement));
}
// This method to perform clean action for WebElement parameter
public static void clear(WebElement webElement) {
// Try to clear text from WebElement
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement).clear();
// If success then no errors
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// Report step log to reporter
reportStepStatus("WebElement has been cleared.", errMessage, captureScreenshotOnStep(), false);
}
// This method to perform submit action for String parameter
public static void submit(String webElement) {
submit(getElement(webElement));
}
// This method to perform submit action for WebElement parameter
public static void submit(WebElement webElement) {
// Try to submit WebElement
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement).submit();
// If success then no errors
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// Report step log to reporter
reportStepStatus("Submit action is applied.", errMessage, captureScreenshotOnStep(), true);
}
// This method to get tag name for String parameter
public static String getTagName(String webElement) {
return getTagName(getElement(webElement));
}
// This method to get tag name for WebElement parameter
public static String getTagName(WebElement webElement) {
// Define tagName variable
String tagName = "";
// Try to get tag name from WebElement
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
focusToElement(webElement).getTagName();
// If success then no errors
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// Report step log to reporter
reportStepStatus("WebElement Tag Name extracted.", errMessage, captureScreenshotOnStep(), false);
return tagName;
}
// This method to check if WebElement is Displayed for String parameter
public static Boolean isWebElementDisplayed(String webElement) {
return isWebElementDisplayed(getElement(webElement));
}
// This method to check if WebElement is Displayed for WebElement parameter
public static Boolean isWebElementDisplayed(WebElement webElement) {
// Define isDisplayed variable
Boolean isDisplayed = false;
// Check if WebElement displayed
for (int attempts = 0; attempts < 3; attempts++) {
try {
// Move to element then apply the action
isDisplayed = focusToElement(webElement).isDisplayed();
// If success then no errors
errMessage = null;
// If success leave for loop
break;
} catch (Throwable throwable) {
// If fail parse throw Get Throw exception and save to errMessage
errMessage = throwable.getMessage();
// If NullPointerException then throwable.getMessage() return null so get throwable.toString()
if (errMessage == null)
errMessage = throwable.toString();
// Wait a time then try again
try {
Thread.sleep(SLEEP_TIMEOUT);
} catch (InterruptedException e) {
}
}
}
// Report step log to reporter
reportStepStatus("WebElement appears on the page.", errMessage, captureScreenshotOnStep(), !isDisplayed);
return isDisplayed;
}
/*************** WebElement related Actions Section Ends ***************/
/*************** WebDriver related Actions Section Start ***************/
// This method return current Title for current active browser tab
public static String getCurrentTitle(WebDriver webDriver) {
return webDriver.getTitle();
}
// This method return current URL for current active browser tab
public static String getCurrentURL(WebDriver webDriver) {
// Wait in case webDriver still no previous page
waitFor(2);
return webDriver.getCurrentUrl();
}
// This method do action navigateTo
public static void navigateTo(WebDriver webDriver, String url) {
// Check if the Provided is one of navigateTo actions or URL
// and execute the action
switch (url.toLowerCase()) {
case "back": {
webDriver.navigate().back();
TestReporter.pass("Navigation to Back action applied", captureScreenshotOnStep());
break;
}
case "forward":
case "next": {
webDriver.navigate().forward();
TestReporter.pass("Navigation to Forward action applied", captureScreenshotOnStep());
break;
}
case "refresh":
case "reload":
case "load": {
webDriver.navigate().refresh();
TestReporter.pass("Page Reload action applied", captureScreenshotOnStep());
break;
}
default: {
if (StringHandler.validateURL(url))
webDriver.navigate().to(url);
else {
// If fail to read navigateTo action then will report a fail step and fail test case
TestReporter.fail("The Provided URL is wrong, Please check URL: " + url, true);
}// If action executed then will pass step in report
TestReporter.pass("Navigated to URL: " + url, captureScreenshotOnStep());
}
}
}
// This method to apply wait for time in seconds
public static void waitFor(int timeInSeconds) {
try {
Thread.sleep(timeInSeconds * 1000);
} catch (Throwable throwable) {
}
}
// This method apply NavigateTo URL action
public static void goToURL(WebDriver webDriver, String url) {
// Check if URL Provided from TestCase File
if (!AnnotationsHandler.getTestCaseProperty(url).isEmpty())
url = AnnotationsHandler.getTestCaseProperty(url);
// Check if baseUrl provided then concat with Url string
if (!WebDriverInitializer.baseUrl.isEmpty())
url = WebDriverInitializer.baseUrl + url;
// Navigate to URL
navigateTo(webDriver, url);
}
// This method apply NavigateTo Back button from browser action
public static void goBack(WebDriver webDriver) {
navigateTo(webDriver, "back");
}
/*************** WebDriver related Actions Section Ends ***************/
}
// Need to implement below actions:
// 1. getAttribute
// 2. getCSSValue
// 3. getLocation
// 4. getRect
// 5. getSize
// 6. isSelected and isEnabled methods
© 2015 - 2025 Weber Informatics LLC | Privacy Policy