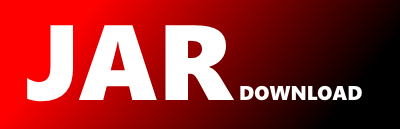
com.g2forge.alexandria.java.fluent.optional.AOptional Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ax-java Show documentation
Show all versions of ax-java Show documentation
Standard Java library and the basis of the ${alexandria.name} project.
package com.g2forge.alexandria.java.fluent.optional;
import java.util.NoSuchElementException;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import com.g2forge.alexandria.java.fluent.IFluent1_;
public abstract class AOptional implements IOptional {
protected final T value;
protected AOptional() {
this.value = null;
}
protected AOptional(T value) {
this.value = require(value);
}
protected abstract AOptional create();
protected AOptional create(IFluent1_ value) {
if (getClass().isInstance(value)) return (NonNullOptional) value;
return value.isEmpty() ? create() : create(value.get());
}
protected abstract AOptional create(U value);
@Override
public boolean equals(Object obj) {
if (this == obj) { return true; }
if (!(obj instanceof AOptional)) { return false; }
final AOptional> that = (AOptional>) obj;
return Objects.equals(isEmpty(), that.isEmpty()) && Objects.equals(value, that.value);
}
public AOptional filter(Predicate super T> predicate) {
Objects.requireNonNull(predicate);
if (isEmpty()) return this;
else return predicate.test(value) ? this : create();
}
public IOptional flatMap(Function super T, ? extends IFluent1_> mapper) {
Objects.requireNonNull(mapper);
if (isEmpty()) return create();
else return create(Objects.requireNonNull(mapper.apply(value)));
}
public T get() {
if (isEmpty()) {
throw new NoSuchElementException("No value present");
}
return value;
}
@Override
public int hashCode() {
return Objects.hash(Boolean.valueOf(isEmpty()), value);
}
public AOptional map(Function super T, ? extends U> mapper) {
Objects.requireNonNull(mapper);
if (isEmpty()) return create();
else return create(mapper.apply(value));
}
public T or(T other) {
return isEmpty() ? other : value;
}
public T orGet(Supplier extends T> other) {
return isEmpty() ? other.get() : value;
}
public T orThrow(Supplier extends X> exception) throws X {
if (isEmpty()) throw exception.get();
else return value;
}
protected <_T> _T require(_T value) {
return value;
}
@Override
public String toString() {
final String name = getClass().getSimpleName();
return isEmpty() ? String.format("%s.empty", name) : String.format("%s[%s]", name, value);
}
public void visit(Consumer super T> consumer) {
if (!isEmpty()) consumer.accept(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy