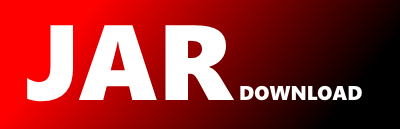
com.g2forge.alexandria.java.function.IConsumer2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ax-java Show documentation
Show all versions of ax-java Show documentation
Standard Java library and the basis of the ${alexandria.name} project.
package com.g2forge.alexandria.java.function;
import java.util.Objects;
import java.util.function.BiConsumer;
@FunctionalInterface
public interface IConsumer2 extends BiConsumer, IConsumer {
public static IConsumer2 create(IConsumer2 consumer) {
return consumer;
}
public default IConsumer2 andThen(BiConsumer super I0, ? super I1> after) {
Objects.requireNonNull(after);
return (input0, input1) -> {
accept(input0, input1);
after.accept(input0, input1);
};
}
public default IConsumer1 curry0(I0 input0) {
return input1 -> this.accept(input0, input1);
}
public default IConsumer1 curry1(I1 input1) {
return input0 -> this.accept(input0, input1);
}
public default IConsumer2 lift0(IFunction1 lift) {
return (i0, i1) -> {
final I0 i0l = lift.apply(i0);
accept(i0l, i1);
};
}
public default IConsumer2 lift1(IFunction1 lift) {
return (i0, i1) -> {
final I1 i1l = lift.apply(i1);
accept(i0, i1l);
};
}
public default IConsumer2 sync(Object lock) {
if (lock == null) return this;
return (i0, i1) -> {
synchronized (lock) {
accept(i0, i1);
}
};
}
public default IFunction2 toFunction(O retVal) {
return (i0, i1) -> {
accept(i0, i1);
return retVal;
};
}
public default IConsumer2 wrap(IRunnable pre, IRunnable post) {
return (i0, i1) -> {
pre.run();
try {
accept(i0, i1);
} finally {
post.run();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy