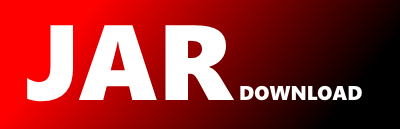
com.g2forge.alexandria.java.function.IFunction3 Maven / Gradle / Ivy
package com.g2forge.alexandria.java.function;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Supplier;
@FunctionalInterface
public interface IFunction3 extends IFunction, IConsumer3 {
public static IFunction3 create(IFunction3 function) {
return function;
}
public default void accept(I0 i0, I1 i1, I2 i2) {
apply(i0, i1, i2);
}
public default <_O> IFunction3 andThen(Function super O, ? extends _O> after) {
Objects.requireNonNull(after);
return (I0 i0, I1 i1, I2 i2) -> after.apply(apply(i0, i1, i2));
}
public O apply(I0 i0, I1 i1, I2 i2);
public default IFunction2 compose0(Supplier extends I0> before) {
Objects.requireNonNull(before);
return (i1, i2) -> apply(before.get(), i1, i2);
}
public default IFunction2 compose1(Supplier extends I1> before) {
Objects.requireNonNull(before);
return (i0, i2) -> apply(i0, before.get(), i2);
}
public default IFunction2 compose2(Supplier extends I2> before) {
Objects.requireNonNull(before);
return (i0, i1) -> apply(i0, i1, before.get());
}
public default IFunction2 curry0(I0 input0) {
return (input1, input2) -> this.apply(input0, input1, input2);
}
public default IFunction2 curry1(I1 input1) {
return (input0, input2) -> this.apply(input0, input1, input2);
}
public default IFunction2 curry2(I2 input2) {
return (input0, input1) -> this.apply(input0, input1, input2);
}
public default IFunction3 lift0(IFunction1 lift) {
return (i0, i1, i2) -> {
final I0 i0l = lift.apply(i0);
return apply(i0l, i1, i2);
};
}
public default IFunction3 lift1(IFunction1 lift) {
return (i0, i1, i2) -> {
final I1 i1l = lift.apply(i1);
return apply(i0, i1l, i2);
};
}
public default IFunction3 lift2(IFunction1 lift) {
return (i0, i1, i2) -> {
final I2 i2l = lift.apply(i2);
return apply(i0, i1, i2l);
};
}
public default IConsumer3 noReturn() {
return (i0, i1, i2) -> apply(i0, i1, i2);
}
public default IFunction3 sync(Object lock) {
if (lock == null) return this;
return (i0, i1, i2) -> {
synchronized (lock) {
return apply(i0, i1, i2);
}
};
}
public default IConsumer3 toConsumer() {
return this::apply;
}
public default <_O> IFunction3 toFunction(_O retVal) {
return (i0, i1, i2) -> {
apply(i0, i1, i2);
return retVal;
};
}
public default IFunction3 wrap(IRunnable pre, IRunnable post) {
return (i0, i1, i2) -> {
if (pre != null) pre.run();
try {
return apply(i0, i1, i2);
} finally {
if (post != null) post.run();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy