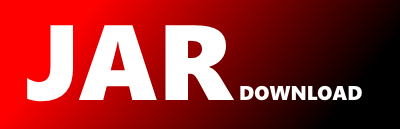
com.g2forge.alexandria.java.nestedstate.StackThreadState Maven / Gradle / Ivy
package com.g2forge.alexandria.java.nestedstate;
import java.util.Stack;
import java.util.function.Function;
import java.util.function.Supplier;
import com.g2forge.alexandria.java.close.ICloseable;
import com.g2forge.alexandria.java.function.LiteralSupplier;
public class StackThreadState implements INestedState {
protected final ThreadLocal> local;
public StackThreadState() {
this.local = ThreadLocal.withInitial(Stack::new);
}
public StackThreadState(Supplier extends T> initial) {
this.local = ThreadLocal.withInitial(() -> {
final Stack stack = new Stack<>();
stack.add(initial.get());
return stack;
});
}
public StackThreadState(T initial) {
this(new LiteralSupplier<>(initial));
}
protected void close(final Stack expectedStack, final T expectedValue) {
if (expectedStack != local.get()) throw new IllegalStateException("Closed from the wrong thread!");
if (expectedStack.pop() != expectedValue) throw new IllegalStateException();
}
public int depth() {
return local.get().size();
}
@Override
public T get() {
return local.get().peek();
}
public ICloseable modify(Function super T, ? extends T> function) {
final Stack stack = local.get();
final T value = function.apply(stack.peek());
stack.push(value);
return () -> close(stack, value);
}
@Override
public ICloseable open(T value) {
final Stack stack = local.get();
stack.push(value);
return () -> close(stack, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy