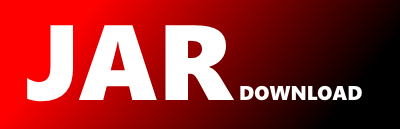
com.galenframework.runner.EventHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of galen-core Show documentation
Show all versions of galen-core Show documentation
A library for layout testing of websites
/*******************************************************************************
* Copyright 2018 Ivan Shubin http://galenframework.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package com.galenframework.runner;
import java.util.LinkedList;
import java.util.List;
import com.galenframework.reports.GalenTestInfo;
import com.galenframework.runner.events.TestEvent;
import com.galenframework.runner.events.TestFilterEvent;
import com.galenframework.runner.events.TestSuiteEvent;
import com.galenframework.reports.GalenTestInfo;
import com.galenframework.runner.events.TestEvent;
import com.galenframework.runner.events.TestFilterEvent;
import com.galenframework.runner.events.TestRetryEvent;
import com.galenframework.runner.events.TestSuiteEvent;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class EventHandler {
private final static Logger LOG = LoggerFactory.getLogger(EventHandler.class);
private List beforeTestEvents = new LinkedList<>();
private List afterTestEvents = new LinkedList<>();
private List testFilterEvents = new LinkedList<>();
private List testRetryEvents = new LinkedList<>();
private List beforeTestSuiteEvents = new LinkedList<>();
private List afterTestSuiteEvents = new LinkedList<>();
public List getBeforeTestEvents() {
return beforeTestEvents;
}
public void setBeforeTestEvents(List beforeTestEvents) {
this.beforeTestEvents = beforeTestEvents;
}
public List getAfterTestEvents() {
return afterTestEvents;
}
public void setAfterTestEvents(List afterTestEvents) {
this.afterTestEvents = afterTestEvents;
}
public List getAfterTestSuiteEvents() {
return afterTestSuiteEvents;
}
public void setAfterTestSuiteEvents(List afterTestSuiteEvents) {
this.afterTestSuiteEvents = afterTestSuiteEvents;
}
public List getBeforeTestSuiteEvents() {
return beforeTestSuiteEvents;
}
public void setBeforeTestSuiteEvents(List beforeTestSuiteEvents) {
this.beforeTestSuiteEvents = beforeTestSuiteEvents;
}
public void invokeBeforeTestSuiteEvents() {
execute(getBeforeTestSuiteEvents());
}
public void invokeAfterTestSuiteEvents() {
execute(getAfterTestSuiteEvents());
}
private void execute(List events) {
if (events != null) {
for (TestSuiteEvent event : events) {
if (event != null) {
try {
event.execute();
} catch (Throwable ex) {
LOG.error("Unknown error during executing test suites.", ex);
}
}
}
}
}
public void invokeBeforeTestEvents(GalenTestInfo testInfo) {
execute(getBeforeTestEvents(), testInfo);
}
public void invokeAfterTestEvents(GalenTestInfo testInfo) {
execute(getAfterTestEvents(), testInfo);
}
private void execute(List events, GalenTestInfo testInfo) {
if (events != null) {
for (TestEvent event : events) {
if (event != null) {
try {
event.execute(testInfo);
} catch (Throwable ex) {
LOG.error("Unknown error during executing test events.", ex);
}
}
}
}
}
public List getTestFilterEvents() {
return testFilterEvents;
}
public void setTestFilterEvents(List testFilterEvents) {
this.testFilterEvents = testFilterEvents;
}
public List getTestRetryEvents() {
return testRetryEvents;
}
public void setTestRetryEvents(List testRetryEvents) {
this.testRetryEvents = testRetryEvents;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy