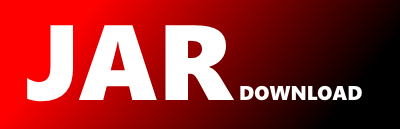
rock.protobuf.Rock Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: rock.proto
package rock.protobuf;
public final class Rock {
private Rock() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface RockRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.RockRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return Whether the userInfo field is set.
*/
boolean hasUserInfo();
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return The userInfo.
*/
rock.protobuf.Rock.UserInfo getUserInfo();
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
rock.protobuf.Rock.UserInfoOrBuilder getUserInfoOrBuilder();
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @return Whether the data field is set.
*/
boolean hasData();
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code rock.RockRequest}
*/
public static final class RockRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.RockRequest)
RockRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RockRequest.newBuilder() to construct.
private RockRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RockRequest() {
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RockRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RockRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
rock.protobuf.Rock.UserInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = userInfo_.toBuilder();
}
userInfo_ = input.readMessage(rock.protobuf.Rock.UserInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(userInfo_);
userInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
bitField0_ |= 0x00000002;
data_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_RockRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_RockRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.RockRequest.class, rock.protobuf.Rock.RockRequest.Builder.class);
}
private int bitField0_;
public static final int USERINFO_FIELD_NUMBER = 1;
private rock.protobuf.Rock.UserInfo userInfo_;
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return Whether the userInfo field is set.
*/
@java.lang.Override
public boolean hasUserInfo() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return The userInfo.
*/
@java.lang.Override
public rock.protobuf.Rock.UserInfo getUserInfo() {
return userInfo_ == null ? rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
@java.lang.Override
public rock.protobuf.Rock.UserInfoOrBuilder getUserInfoOrBuilder() {
return userInfo_ == null ? rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
}
public static final int DATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString data_;
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasUserInfo()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasData()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getUserInfo());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, data_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getUserInfo());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.RockRequest)) {
return super.equals(obj);
}
rock.protobuf.Rock.RockRequest other = (rock.protobuf.Rock.RockRequest) obj;
if (hasUserInfo() != other.hasUserInfo()) return false;
if (hasUserInfo()) {
if (!getUserInfo()
.equals(other.getUserInfo())) return false;
}
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUserInfo()) {
hash = (37 * hash) + USERINFO_FIELD_NUMBER;
hash = (53 * hash) + getUserInfo().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.RockRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.RockRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.RockRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.RockRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.RockRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.RockRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.RockRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.RockRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.RockRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.RockRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.RockRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.RockRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.RockRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code rock.RockRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.RockRequest)
rock.protobuf.Rock.RockRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_RockRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_RockRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.RockRequest.class, rock.protobuf.Rock.RockRequest.Builder.class);
}
// Construct using rock.protobuf.Rock.RockRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getUserInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (userInfoBuilder_ == null) {
userInfo_ = null;
} else {
userInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_RockRequest_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.RockRequest getDefaultInstanceForType() {
return rock.protobuf.Rock.RockRequest.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.RockRequest build() {
rock.protobuf.Rock.RockRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.RockRequest buildPartial() {
rock.protobuf.Rock.RockRequest result = new rock.protobuf.Rock.RockRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (userInfoBuilder_ == null) {
result.userInfo_ = userInfo_;
} else {
result.userInfo_ = userInfoBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.data_ = data_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.RockRequest) {
return mergeFrom((rock.protobuf.Rock.RockRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.RockRequest other) {
if (other == rock.protobuf.Rock.RockRequest.getDefaultInstance()) return this;
if (other.hasUserInfo()) {
mergeUserInfo(other.getUserInfo());
}
if (other.hasData()) {
setData(other.getData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasUserInfo()) {
return false;
}
if (!hasData()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.RockRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.RockRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private rock.protobuf.Rock.UserInfo userInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.UserInfo, rock.protobuf.Rock.UserInfo.Builder, rock.protobuf.Rock.UserInfoOrBuilder> userInfoBuilder_;
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return Whether the userInfo field is set.
*/
public boolean hasUserInfo() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return The userInfo.
*/
public rock.protobuf.Rock.UserInfo getUserInfo() {
if (userInfoBuilder_ == null) {
return userInfo_ == null ? rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
} else {
return userInfoBuilder_.getMessage();
}
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder setUserInfo(rock.protobuf.Rock.UserInfo value) {
if (userInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userInfo_ = value;
onChanged();
} else {
userInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder setUserInfo(
rock.protobuf.Rock.UserInfo.Builder builderForValue) {
if (userInfoBuilder_ == null) {
userInfo_ = builderForValue.build();
onChanged();
} else {
userInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder mergeUserInfo(rock.protobuf.Rock.UserInfo value) {
if (userInfoBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
userInfo_ != null &&
userInfo_ != rock.protobuf.Rock.UserInfo.getDefaultInstance()) {
userInfo_ =
rock.protobuf.Rock.UserInfo.newBuilder(userInfo_).mergeFrom(value).buildPartial();
} else {
userInfo_ = value;
}
onChanged();
} else {
userInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder clearUserInfo() {
if (userInfoBuilder_ == null) {
userInfo_ = null;
onChanged();
} else {
userInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public rock.protobuf.Rock.UserInfo.Builder getUserInfoBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getUserInfoFieldBuilder().getBuilder();
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public rock.protobuf.Rock.UserInfoOrBuilder getUserInfoOrBuilder() {
if (userInfoBuilder_ != null) {
return userInfoBuilder_.getMessageOrBuilder();
} else {
return userInfo_ == null ?
rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
}
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.UserInfo, rock.protobuf.Rock.UserInfo.Builder, rock.protobuf.Rock.UserInfoOrBuilder>
getUserInfoFieldBuilder() {
if (userInfoBuilder_ == null) {
userInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.UserInfo, rock.protobuf.Rock.UserInfo.Builder, rock.protobuf.Rock.UserInfoOrBuilder>(
getUserInfo(),
getParentForChildren(),
isClean());
userInfo_ = null;
}
return userInfoBuilder_;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
data_ = value;
onChanged();
return this;
}
/**
*
*需要发送数据
*
*
* required bytes data = 2;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.RockRequest)
}
// @@protoc_insertion_point(class_scope:rock.RockRequest)
private static final rock.protobuf.Rock.RockRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.RockRequest();
}
public static rock.protobuf.Rock.RockRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RockRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RockRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.RockRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RockResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.RockResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return Whether the userInfo field is set.
*/
boolean hasUserInfo();
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return The userInfo.
*/
rock.protobuf.Rock.UserInfo getUserInfo();
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
rock.protobuf.Rock.UserInfoOrBuilder getUserInfoOrBuilder();
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @return Whether the data field is set.
*/
boolean hasData();
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code rock.RockResponse}
*/
public static final class RockResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.RockResponse)
RockResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RockResponse.newBuilder() to construct.
private RockResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RockResponse() {
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RockResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RockResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
rock.protobuf.Rock.UserInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = userInfo_.toBuilder();
}
userInfo_ = input.readMessage(rock.protobuf.Rock.UserInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(userInfo_);
userInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
bitField0_ |= 0x00000002;
data_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_RockResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_RockResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.RockResponse.class, rock.protobuf.Rock.RockResponse.Builder.class);
}
private int bitField0_;
public static final int USERINFO_FIELD_NUMBER = 1;
private rock.protobuf.Rock.UserInfo userInfo_;
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return Whether the userInfo field is set.
*/
@java.lang.Override
public boolean hasUserInfo() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return The userInfo.
*/
@java.lang.Override
public rock.protobuf.Rock.UserInfo getUserInfo() {
return userInfo_ == null ? rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
@java.lang.Override
public rock.protobuf.Rock.UserInfoOrBuilder getUserInfoOrBuilder() {
return userInfo_ == null ? rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
}
public static final int DATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString data_;
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasUserInfo()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasData()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getUserInfo());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, data_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getUserInfo());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.RockResponse)) {
return super.equals(obj);
}
rock.protobuf.Rock.RockResponse other = (rock.protobuf.Rock.RockResponse) obj;
if (hasUserInfo() != other.hasUserInfo()) return false;
if (hasUserInfo()) {
if (!getUserInfo()
.equals(other.getUserInfo())) return false;
}
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUserInfo()) {
hash = (37 * hash) + USERINFO_FIELD_NUMBER;
hash = (53 * hash) + getUserInfo().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.RockResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.RockResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.RockResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.RockResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.RockResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.RockResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.RockResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.RockResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.RockResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.RockResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.RockResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.RockResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.RockResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code rock.RockResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.RockResponse)
rock.protobuf.Rock.RockResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_RockResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_RockResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.RockResponse.class, rock.protobuf.Rock.RockResponse.Builder.class);
}
// Construct using rock.protobuf.Rock.RockResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getUserInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (userInfoBuilder_ == null) {
userInfo_ = null;
} else {
userInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_RockResponse_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.RockResponse getDefaultInstanceForType() {
return rock.protobuf.Rock.RockResponse.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.RockResponse build() {
rock.protobuf.Rock.RockResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.RockResponse buildPartial() {
rock.protobuf.Rock.RockResponse result = new rock.protobuf.Rock.RockResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (userInfoBuilder_ == null) {
result.userInfo_ = userInfo_;
} else {
result.userInfo_ = userInfoBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.data_ = data_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.RockResponse) {
return mergeFrom((rock.protobuf.Rock.RockResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.RockResponse other) {
if (other == rock.protobuf.Rock.RockResponse.getDefaultInstance()) return this;
if (other.hasUserInfo()) {
mergeUserInfo(other.getUserInfo());
}
if (other.hasData()) {
setData(other.getData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasUserInfo()) {
return false;
}
if (!hasData()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.RockResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.RockResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private rock.protobuf.Rock.UserInfo userInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.UserInfo, rock.protobuf.Rock.UserInfo.Builder, rock.protobuf.Rock.UserInfoOrBuilder> userInfoBuilder_;
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return Whether the userInfo field is set.
*/
public boolean hasUserInfo() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
* @return The userInfo.
*/
public rock.protobuf.Rock.UserInfo getUserInfo() {
if (userInfoBuilder_ == null) {
return userInfo_ == null ? rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
} else {
return userInfoBuilder_.getMessage();
}
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder setUserInfo(rock.protobuf.Rock.UserInfo value) {
if (userInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userInfo_ = value;
onChanged();
} else {
userInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder setUserInfo(
rock.protobuf.Rock.UserInfo.Builder builderForValue) {
if (userInfoBuilder_ == null) {
userInfo_ = builderForValue.build();
onChanged();
} else {
userInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder mergeUserInfo(rock.protobuf.Rock.UserInfo value) {
if (userInfoBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
userInfo_ != null &&
userInfo_ != rock.protobuf.Rock.UserInfo.getDefaultInstance()) {
userInfo_ =
rock.protobuf.Rock.UserInfo.newBuilder(userInfo_).mergeFrom(value).buildPartial();
} else {
userInfo_ = value;
}
onChanged();
} else {
userInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public Builder clearUserInfo() {
if (userInfoBuilder_ == null) {
userInfo_ = null;
onChanged();
} else {
userInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public rock.protobuf.Rock.UserInfo.Builder getUserInfoBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getUserInfoFieldBuilder().getBuilder();
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
public rock.protobuf.Rock.UserInfoOrBuilder getUserInfoOrBuilder() {
if (userInfoBuilder_ != null) {
return userInfoBuilder_.getMessageOrBuilder();
} else {
return userInfo_ == null ?
rock.protobuf.Rock.UserInfo.getDefaultInstance() : userInfo_;
}
}
/**
*
*基础用户相关信息
*
*
* required .rock.UserInfo UserInfo = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.UserInfo, rock.protobuf.Rock.UserInfo.Builder, rock.protobuf.Rock.UserInfoOrBuilder>
getUserInfoFieldBuilder() {
if (userInfoBuilder_ == null) {
userInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.UserInfo, rock.protobuf.Rock.UserInfo.Builder, rock.protobuf.Rock.UserInfoOrBuilder>(
getUserInfo(),
getParentForChildren(),
isClean());
userInfo_ = null;
}
return userInfoBuilder_;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
data_ = value;
onChanged();
return this;
}
/**
*
*服务器返回数据
*
*
* required bytes data = 2;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.RockResponse)
}
// @@protoc_insertion_point(class_scope:rock.RockResponse)
private static final rock.protobuf.Rock.RockResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.RockResponse();
}
public static rock.protobuf.Rock.RockResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RockResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RockResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.RockResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DeviceInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.DeviceInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return Whether the uUIDOne field is set.
*/
boolean hasUUIDOne();
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return The uUIDOne.
*/
java.lang.String getUUIDOne();
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return The bytes for uUIDOne.
*/
com.google.protobuf.ByteString
getUUIDOneBytes();
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return Whether the uUIDTwo field is set.
*/
boolean hasUUIDTwo();
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return The uUIDTwo.
*/
java.lang.String getUUIDTwo();
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return The bytes for uUIDTwo.
*/
com.google.protobuf.ByteString
getUUIDTwoBytes();
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return Whether the imei field is set.
*/
boolean hasImei();
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return The imei.
*/
java.lang.String getImei();
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return The bytes for imei.
*/
com.google.protobuf.ByteString
getImeiBytes();
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @return Whether the deviceID field is set.
*/
boolean hasDeviceID();
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @return The deviceID.
*/
com.google.protobuf.ByteString getDeviceID();
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return Whether the deviceName field is set.
*/
boolean hasDeviceName();
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return The deviceName.
*/
java.lang.String getDeviceName();
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return The bytes for deviceName.
*/
com.google.protobuf.ByteString
getDeviceNameBytes();
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return Whether the timeZone field is set.
*/
boolean hasTimeZone();
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return The timeZone.
*/
java.lang.String getTimeZone();
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return The bytes for timeZone.
*/
com.google.protobuf.ByteString
getTimeZoneBytes();
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return Whether the language field is set.
*/
boolean hasLanguage();
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return The language.
*/
java.lang.String getLanguage();
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return The bytes for language.
*/
com.google.protobuf.ByteString
getLanguageBytes();
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return Whether the deviceBrand field is set.
*/
boolean hasDeviceBrand();
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return The deviceBrand.
*/
java.lang.String getDeviceBrand();
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return The bytes for deviceBrand.
*/
com.google.protobuf.ByteString
getDeviceBrandBytes();
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return Whether the realCountry field is set.
*/
boolean hasRealCountry();
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return The realCountry.
*/
java.lang.String getRealCountry();
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return The bytes for realCountry.
*/
com.google.protobuf.ByteString
getRealCountryBytes();
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return Whether the iphoneVer field is set.
*/
boolean hasIphoneVer();
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return The iphoneVer.
*/
java.lang.String getIphoneVer();
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return The bytes for iphoneVer.
*/
com.google.protobuf.ByteString
getIphoneVerBytes();
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return Whether the bundleID field is set.
*/
boolean hasBundleID();
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return The bundleID.
*/
java.lang.String getBundleID();
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return The bytes for bundleID.
*/
com.google.protobuf.ByteString
getBundleIDBytes();
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return Whether the osType field is set.
*/
boolean hasOsType();
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return The osType.
*/
java.lang.String getOsType();
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return The bytes for osType.
*/
com.google.protobuf.ByteString
getOsTypeBytes();
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return Whether the adSource field is set.
*/
boolean hasAdSource();
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return The adSource.
*/
java.lang.String getAdSource();
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return The bytes for adSource.
*/
com.google.protobuf.ByteString
getAdSourceBytes();
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return Whether the osTypeNumber field is set.
*/
boolean hasOsTypeNumber();
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return The osTypeNumber.
*/
java.lang.String getOsTypeNumber();
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return The bytes for osTypeNumber.
*/
com.google.protobuf.ByteString
getOsTypeNumberBytes();
/**
* optional uint32 CoreCount = 15;
* @return Whether the coreCount field is set.
*/
boolean hasCoreCount();
/**
* optional uint32 CoreCount = 15;
* @return The coreCount.
*/
int getCoreCount();
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return Whether the carrierName field is set.
*/
boolean hasCarrierName();
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return The carrierName.
*/
java.lang.String getCarrierName();
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return The bytes for carrierName.
*/
com.google.protobuf.ByteString
getCarrierNameBytes();
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return Whether the softTypeXML field is set.
*/
boolean hasSoftTypeXML();
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return The softTypeXML.
*/
java.lang.String getSoftTypeXML();
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return The bytes for softTypeXML.
*/
com.google.protobuf.ByteString
getSoftTypeXMLBytes();
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return Whether the clientCheckDataXML field is set.
*/
boolean hasClientCheckDataXML();
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return The clientCheckDataXML.
*/
java.lang.String getClientCheckDataXML();
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return The bytes for clientCheckDataXML.
*/
com.google.protobuf.ByteString
getClientCheckDataXMLBytes();
}
/**
*
* DeviceInfo 设备信息
*
*
* Protobuf type {@code rock.DeviceInfo}
*/
public static final class DeviceInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.DeviceInfo)
DeviceInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeviceInfo.newBuilder() to construct.
private DeviceInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeviceInfo() {
uUIDOne_ = "";
uUIDTwo_ = "";
imei_ = "";
deviceID_ = com.google.protobuf.ByteString.EMPTY;
deviceName_ = "";
timeZone_ = "";
language_ = "";
deviceBrand_ = "";
realCountry_ = "";
iphoneVer_ = "";
bundleID_ = "";
osType_ = "";
adSource_ = "";
osTypeNumber_ = "";
carrierName_ = "";
softTypeXML_ = "";
clientCheckDataXML_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DeviceInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DeviceInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
uUIDOne_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
uUIDTwo_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
imei_ = bs;
break;
}
case 34: {
bitField0_ |= 0x00000008;
deviceID_ = input.readBytes();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
deviceName_ = bs;
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
timeZone_ = bs;
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
language_ = bs;
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000080;
deviceBrand_ = bs;
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
realCountry_ = bs;
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000200;
iphoneVer_ = bs;
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
bundleID_ = bs;
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000800;
osType_ = bs;
break;
}
case 106: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00001000;
adSource_ = bs;
break;
}
case 114: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00002000;
osTypeNumber_ = bs;
break;
}
case 120: {
bitField0_ |= 0x00004000;
coreCount_ = input.readUInt32();
break;
}
case 130: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00008000;
carrierName_ = bs;
break;
}
case 138: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00010000;
softTypeXML_ = bs;
break;
}
case 146: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00020000;
clientCheckDataXML_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_DeviceInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_DeviceInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.DeviceInfo.class, rock.protobuf.Rock.DeviceInfo.Builder.class);
}
private int bitField0_;
public static final int UUIDONE_FIELD_NUMBER = 1;
private volatile java.lang.Object uUIDOne_;
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return Whether the uUIDOne field is set.
*/
@java.lang.Override
public boolean hasUUIDOne() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return The uUIDOne.
*/
@java.lang.Override
public java.lang.String getUUIDOne() {
java.lang.Object ref = uUIDOne_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uUIDOne_ = s;
}
return s;
}
}
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return The bytes for uUIDOne.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUUIDOneBytes() {
java.lang.Object ref = uUIDOne_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uUIDOne_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UUIDTWO_FIELD_NUMBER = 2;
private volatile java.lang.Object uUIDTwo_;
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return Whether the uUIDTwo field is set.
*/
@java.lang.Override
public boolean hasUUIDTwo() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return The uUIDTwo.
*/
@java.lang.Override
public java.lang.String getUUIDTwo() {
java.lang.Object ref = uUIDTwo_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uUIDTwo_ = s;
}
return s;
}
}
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return The bytes for uUIDTwo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUUIDTwoBytes() {
java.lang.Object ref = uUIDTwo_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uUIDTwo_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IMEI_FIELD_NUMBER = 3;
private volatile java.lang.Object imei_;
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return Whether the imei field is set.
*/
@java.lang.Override
public boolean hasImei() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return The imei.
*/
@java.lang.Override
public java.lang.String getImei() {
java.lang.Object ref = imei_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
imei_ = s;
}
return s;
}
}
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return The bytes for imei.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getImeiBytes() {
java.lang.Object ref = imei_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imei_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVICEID_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString deviceID_;
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @return Whether the deviceID field is set.
*/
@java.lang.Override
public boolean hasDeviceID() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @return The deviceID.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDeviceID() {
return deviceID_;
}
public static final int DEVICENAME_FIELD_NUMBER = 5;
private volatile java.lang.Object deviceName_;
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return Whether the deviceName field is set.
*/
@java.lang.Override
public boolean hasDeviceName() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return The deviceName.
*/
@java.lang.Override
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceName_ = s;
}
return s;
}
}
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return The bytes for deviceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMEZONE_FIELD_NUMBER = 6;
private volatile java.lang.Object timeZone_;
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return Whether the timeZone field is set.
*/
@java.lang.Override
public boolean hasTimeZone() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return The timeZone.
*/
@java.lang.Override
public java.lang.String getTimeZone() {
java.lang.Object ref = timeZone_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timeZone_ = s;
}
return s;
}
}
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return The bytes for timeZone.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTimeZoneBytes() {
java.lang.Object ref = timeZone_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeZone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LANGUAGE_FIELD_NUMBER = 7;
private volatile java.lang.Object language_;
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return Whether the language field is set.
*/
@java.lang.Override
public boolean hasLanguage() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return The language.
*/
@java.lang.Override
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
}
}
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return The bytes for language.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVICEBRAND_FIELD_NUMBER = 8;
private volatile java.lang.Object deviceBrand_;
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return Whether the deviceBrand field is set.
*/
@java.lang.Override
public boolean hasDeviceBrand() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return The deviceBrand.
*/
@java.lang.Override
public java.lang.String getDeviceBrand() {
java.lang.Object ref = deviceBrand_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceBrand_ = s;
}
return s;
}
}
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return The bytes for deviceBrand.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDeviceBrandBytes() {
java.lang.Object ref = deviceBrand_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceBrand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REALCOUNTRY_FIELD_NUMBER = 9;
private volatile java.lang.Object realCountry_;
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return Whether the realCountry field is set.
*/
@java.lang.Override
public boolean hasRealCountry() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return The realCountry.
*/
@java.lang.Override
public java.lang.String getRealCountry() {
java.lang.Object ref = realCountry_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
realCountry_ = s;
}
return s;
}
}
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return The bytes for realCountry.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRealCountryBytes() {
java.lang.Object ref = realCountry_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
realCountry_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IPHONEVER_FIELD_NUMBER = 10;
private volatile java.lang.Object iphoneVer_;
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return Whether the iphoneVer field is set.
*/
@java.lang.Override
public boolean hasIphoneVer() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return The iphoneVer.
*/
@java.lang.Override
public java.lang.String getIphoneVer() {
java.lang.Object ref = iphoneVer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
iphoneVer_ = s;
}
return s;
}
}
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return The bytes for iphoneVer.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIphoneVerBytes() {
java.lang.Object ref = iphoneVer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
iphoneVer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BUNDLEID_FIELD_NUMBER = 11;
private volatile java.lang.Object bundleID_;
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return Whether the bundleID field is set.
*/
@java.lang.Override
public boolean hasBundleID() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return The bundleID.
*/
@java.lang.Override
public java.lang.String getBundleID() {
java.lang.Object ref = bundleID_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
bundleID_ = s;
}
return s;
}
}
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return The bytes for bundleID.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBundleIDBytes() {
java.lang.Object ref = bundleID_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bundleID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OSTYPE_FIELD_NUMBER = 12;
private volatile java.lang.Object osType_;
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return Whether the osType field is set.
*/
@java.lang.Override
public boolean hasOsType() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return The osType.
*/
@java.lang.Override
public java.lang.String getOsType() {
java.lang.Object ref = osType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osType_ = s;
}
return s;
}
}
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return The bytes for osType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOsTypeBytes() {
java.lang.Object ref = osType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADSOURCE_FIELD_NUMBER = 13;
private volatile java.lang.Object adSource_;
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return Whether the adSource field is set.
*/
@java.lang.Override
public boolean hasAdSource() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return The adSource.
*/
@java.lang.Override
public java.lang.String getAdSource() {
java.lang.Object ref = adSource_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
adSource_ = s;
}
return s;
}
}
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return The bytes for adSource.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdSourceBytes() {
java.lang.Object ref = adSource_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adSource_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OSTYPENUMBER_FIELD_NUMBER = 14;
private volatile java.lang.Object osTypeNumber_;
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return Whether the osTypeNumber field is set.
*/
@java.lang.Override
public boolean hasOsTypeNumber() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return The osTypeNumber.
*/
@java.lang.Override
public java.lang.String getOsTypeNumber() {
java.lang.Object ref = osTypeNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osTypeNumber_ = s;
}
return s;
}
}
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return The bytes for osTypeNumber.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOsTypeNumberBytes() {
java.lang.Object ref = osTypeNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osTypeNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CORECOUNT_FIELD_NUMBER = 15;
private int coreCount_;
/**
* optional uint32 CoreCount = 15;
* @return Whether the coreCount field is set.
*/
@java.lang.Override
public boolean hasCoreCount() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
* optional uint32 CoreCount = 15;
* @return The coreCount.
*/
@java.lang.Override
public int getCoreCount() {
return coreCount_;
}
public static final int CARRIERNAME_FIELD_NUMBER = 16;
private volatile java.lang.Object carrierName_;
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return Whether the carrierName field is set.
*/
@java.lang.Override
public boolean hasCarrierName() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return The carrierName.
*/
@java.lang.Override
public java.lang.String getCarrierName() {
java.lang.Object ref = carrierName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
carrierName_ = s;
}
return s;
}
}
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return The bytes for carrierName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCarrierNameBytes() {
java.lang.Object ref = carrierName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
carrierName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOFTTYPEXML_FIELD_NUMBER = 17;
private volatile java.lang.Object softTypeXML_;
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return Whether the softTypeXML field is set.
*/
@java.lang.Override
public boolean hasSoftTypeXML() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return The softTypeXML.
*/
@java.lang.Override
public java.lang.String getSoftTypeXML() {
java.lang.Object ref = softTypeXML_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
softTypeXML_ = s;
}
return s;
}
}
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return The bytes for softTypeXML.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSoftTypeXMLBytes() {
java.lang.Object ref = softTypeXML_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
softTypeXML_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENTCHECKDATAXML_FIELD_NUMBER = 18;
private volatile java.lang.Object clientCheckDataXML_;
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return Whether the clientCheckDataXML field is set.
*/
@java.lang.Override
public boolean hasClientCheckDataXML() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return The clientCheckDataXML.
*/
@java.lang.Override
public java.lang.String getClientCheckDataXML() {
java.lang.Object ref = clientCheckDataXML_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientCheckDataXML_ = s;
}
return s;
}
}
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return The bytes for clientCheckDataXML.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getClientCheckDataXMLBytes() {
java.lang.Object ref = clientCheckDataXML_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientCheckDataXML_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uUIDOne_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, uUIDTwo_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, imei_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBytes(4, deviceID_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, deviceName_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, timeZone_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, language_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, deviceBrand_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, realCountry_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, iphoneVer_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, bundleID_);
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, osType_);
}
if (((bitField0_ & 0x00001000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, adSource_);
}
if (((bitField0_ & 0x00002000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, osTypeNumber_);
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeUInt32(15, coreCount_);
}
if (((bitField0_ & 0x00008000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, carrierName_);
}
if (((bitField0_ & 0x00010000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, softTypeXML_);
}
if (((bitField0_ & 0x00020000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, clientCheckDataXML_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uUIDOne_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, uUIDTwo_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, imei_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, deviceID_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, deviceName_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, timeZone_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, language_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, deviceBrand_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, realCountry_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, iphoneVer_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, bundleID_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, osType_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, adSource_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, osTypeNumber_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(15, coreCount_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, carrierName_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, softTypeXML_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, clientCheckDataXML_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.DeviceInfo)) {
return super.equals(obj);
}
rock.protobuf.Rock.DeviceInfo other = (rock.protobuf.Rock.DeviceInfo) obj;
if (hasUUIDOne() != other.hasUUIDOne()) return false;
if (hasUUIDOne()) {
if (!getUUIDOne()
.equals(other.getUUIDOne())) return false;
}
if (hasUUIDTwo() != other.hasUUIDTwo()) return false;
if (hasUUIDTwo()) {
if (!getUUIDTwo()
.equals(other.getUUIDTwo())) return false;
}
if (hasImei() != other.hasImei()) return false;
if (hasImei()) {
if (!getImei()
.equals(other.getImei())) return false;
}
if (hasDeviceID() != other.hasDeviceID()) return false;
if (hasDeviceID()) {
if (!getDeviceID()
.equals(other.getDeviceID())) return false;
}
if (hasDeviceName() != other.hasDeviceName()) return false;
if (hasDeviceName()) {
if (!getDeviceName()
.equals(other.getDeviceName())) return false;
}
if (hasTimeZone() != other.hasTimeZone()) return false;
if (hasTimeZone()) {
if (!getTimeZone()
.equals(other.getTimeZone())) return false;
}
if (hasLanguage() != other.hasLanguage()) return false;
if (hasLanguage()) {
if (!getLanguage()
.equals(other.getLanguage())) return false;
}
if (hasDeviceBrand() != other.hasDeviceBrand()) return false;
if (hasDeviceBrand()) {
if (!getDeviceBrand()
.equals(other.getDeviceBrand())) return false;
}
if (hasRealCountry() != other.hasRealCountry()) return false;
if (hasRealCountry()) {
if (!getRealCountry()
.equals(other.getRealCountry())) return false;
}
if (hasIphoneVer() != other.hasIphoneVer()) return false;
if (hasIphoneVer()) {
if (!getIphoneVer()
.equals(other.getIphoneVer())) return false;
}
if (hasBundleID() != other.hasBundleID()) return false;
if (hasBundleID()) {
if (!getBundleID()
.equals(other.getBundleID())) return false;
}
if (hasOsType() != other.hasOsType()) return false;
if (hasOsType()) {
if (!getOsType()
.equals(other.getOsType())) return false;
}
if (hasAdSource() != other.hasAdSource()) return false;
if (hasAdSource()) {
if (!getAdSource()
.equals(other.getAdSource())) return false;
}
if (hasOsTypeNumber() != other.hasOsTypeNumber()) return false;
if (hasOsTypeNumber()) {
if (!getOsTypeNumber()
.equals(other.getOsTypeNumber())) return false;
}
if (hasCoreCount() != other.hasCoreCount()) return false;
if (hasCoreCount()) {
if (getCoreCount()
!= other.getCoreCount()) return false;
}
if (hasCarrierName() != other.hasCarrierName()) return false;
if (hasCarrierName()) {
if (!getCarrierName()
.equals(other.getCarrierName())) return false;
}
if (hasSoftTypeXML() != other.hasSoftTypeXML()) return false;
if (hasSoftTypeXML()) {
if (!getSoftTypeXML()
.equals(other.getSoftTypeXML())) return false;
}
if (hasClientCheckDataXML() != other.hasClientCheckDataXML()) return false;
if (hasClientCheckDataXML()) {
if (!getClientCheckDataXML()
.equals(other.getClientCheckDataXML())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUUIDOne()) {
hash = (37 * hash) + UUIDONE_FIELD_NUMBER;
hash = (53 * hash) + getUUIDOne().hashCode();
}
if (hasUUIDTwo()) {
hash = (37 * hash) + UUIDTWO_FIELD_NUMBER;
hash = (53 * hash) + getUUIDTwo().hashCode();
}
if (hasImei()) {
hash = (37 * hash) + IMEI_FIELD_NUMBER;
hash = (53 * hash) + getImei().hashCode();
}
if (hasDeviceID()) {
hash = (37 * hash) + DEVICEID_FIELD_NUMBER;
hash = (53 * hash) + getDeviceID().hashCode();
}
if (hasDeviceName()) {
hash = (37 * hash) + DEVICENAME_FIELD_NUMBER;
hash = (53 * hash) + getDeviceName().hashCode();
}
if (hasTimeZone()) {
hash = (37 * hash) + TIMEZONE_FIELD_NUMBER;
hash = (53 * hash) + getTimeZone().hashCode();
}
if (hasLanguage()) {
hash = (37 * hash) + LANGUAGE_FIELD_NUMBER;
hash = (53 * hash) + getLanguage().hashCode();
}
if (hasDeviceBrand()) {
hash = (37 * hash) + DEVICEBRAND_FIELD_NUMBER;
hash = (53 * hash) + getDeviceBrand().hashCode();
}
if (hasRealCountry()) {
hash = (37 * hash) + REALCOUNTRY_FIELD_NUMBER;
hash = (53 * hash) + getRealCountry().hashCode();
}
if (hasIphoneVer()) {
hash = (37 * hash) + IPHONEVER_FIELD_NUMBER;
hash = (53 * hash) + getIphoneVer().hashCode();
}
if (hasBundleID()) {
hash = (37 * hash) + BUNDLEID_FIELD_NUMBER;
hash = (53 * hash) + getBundleID().hashCode();
}
if (hasOsType()) {
hash = (37 * hash) + OSTYPE_FIELD_NUMBER;
hash = (53 * hash) + getOsType().hashCode();
}
if (hasAdSource()) {
hash = (37 * hash) + ADSOURCE_FIELD_NUMBER;
hash = (53 * hash) + getAdSource().hashCode();
}
if (hasOsTypeNumber()) {
hash = (37 * hash) + OSTYPENUMBER_FIELD_NUMBER;
hash = (53 * hash) + getOsTypeNumber().hashCode();
}
if (hasCoreCount()) {
hash = (37 * hash) + CORECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getCoreCount();
}
if (hasCarrierName()) {
hash = (37 * hash) + CARRIERNAME_FIELD_NUMBER;
hash = (53 * hash) + getCarrierName().hashCode();
}
if (hasSoftTypeXML()) {
hash = (37 * hash) + SOFTTYPEXML_FIELD_NUMBER;
hash = (53 * hash) + getSoftTypeXML().hashCode();
}
if (hasClientCheckDataXML()) {
hash = (37 * hash) + CLIENTCHECKDATAXML_FIELD_NUMBER;
hash = (53 * hash) + getClientCheckDataXML().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.DeviceInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.DeviceInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.DeviceInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.DeviceInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DeviceInfo 设备信息
*
*
* Protobuf type {@code rock.DeviceInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.DeviceInfo)
rock.protobuf.Rock.DeviceInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_DeviceInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_DeviceInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.DeviceInfo.class, rock.protobuf.Rock.DeviceInfo.Builder.class);
}
// Construct using rock.protobuf.Rock.DeviceInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uUIDOne_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
uUIDTwo_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
imei_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
deviceID_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
deviceName_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
timeZone_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
language_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
deviceBrand_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
realCountry_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
iphoneVer_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
bundleID_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
osType_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
adSource_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
osTypeNumber_ = "";
bitField0_ = (bitField0_ & ~0x00002000);
coreCount_ = 0;
bitField0_ = (bitField0_ & ~0x00004000);
carrierName_ = "";
bitField0_ = (bitField0_ & ~0x00008000);
softTypeXML_ = "";
bitField0_ = (bitField0_ & ~0x00010000);
clientCheckDataXML_ = "";
bitField0_ = (bitField0_ & ~0x00020000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_DeviceInfo_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.DeviceInfo getDefaultInstanceForType() {
return rock.protobuf.Rock.DeviceInfo.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.DeviceInfo build() {
rock.protobuf.Rock.DeviceInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.DeviceInfo buildPartial() {
rock.protobuf.Rock.DeviceInfo result = new rock.protobuf.Rock.DeviceInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.uUIDOne_ = uUIDOne_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.uUIDTwo_ = uUIDTwo_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.imei_ = imei_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.deviceID_ = deviceID_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.deviceName_ = deviceName_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.timeZone_ = timeZone_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.language_ = language_;
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.deviceBrand_ = deviceBrand_;
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.realCountry_ = realCountry_;
if (((from_bitField0_ & 0x00000200) != 0)) {
to_bitField0_ |= 0x00000200;
}
result.iphoneVer_ = iphoneVer_;
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000400;
}
result.bundleID_ = bundleID_;
if (((from_bitField0_ & 0x00000800) != 0)) {
to_bitField0_ |= 0x00000800;
}
result.osType_ = osType_;
if (((from_bitField0_ & 0x00001000) != 0)) {
to_bitField0_ |= 0x00001000;
}
result.adSource_ = adSource_;
if (((from_bitField0_ & 0x00002000) != 0)) {
to_bitField0_ |= 0x00002000;
}
result.osTypeNumber_ = osTypeNumber_;
if (((from_bitField0_ & 0x00004000) != 0)) {
result.coreCount_ = coreCount_;
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
to_bitField0_ |= 0x00008000;
}
result.carrierName_ = carrierName_;
if (((from_bitField0_ & 0x00010000) != 0)) {
to_bitField0_ |= 0x00010000;
}
result.softTypeXML_ = softTypeXML_;
if (((from_bitField0_ & 0x00020000) != 0)) {
to_bitField0_ |= 0x00020000;
}
result.clientCheckDataXML_ = clientCheckDataXML_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.DeviceInfo) {
return mergeFrom((rock.protobuf.Rock.DeviceInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.DeviceInfo other) {
if (other == rock.protobuf.Rock.DeviceInfo.getDefaultInstance()) return this;
if (other.hasUUIDOne()) {
bitField0_ |= 0x00000001;
uUIDOne_ = other.uUIDOne_;
onChanged();
}
if (other.hasUUIDTwo()) {
bitField0_ |= 0x00000002;
uUIDTwo_ = other.uUIDTwo_;
onChanged();
}
if (other.hasImei()) {
bitField0_ |= 0x00000004;
imei_ = other.imei_;
onChanged();
}
if (other.hasDeviceID()) {
setDeviceID(other.getDeviceID());
}
if (other.hasDeviceName()) {
bitField0_ |= 0x00000010;
deviceName_ = other.deviceName_;
onChanged();
}
if (other.hasTimeZone()) {
bitField0_ |= 0x00000020;
timeZone_ = other.timeZone_;
onChanged();
}
if (other.hasLanguage()) {
bitField0_ |= 0x00000040;
language_ = other.language_;
onChanged();
}
if (other.hasDeviceBrand()) {
bitField0_ |= 0x00000080;
deviceBrand_ = other.deviceBrand_;
onChanged();
}
if (other.hasRealCountry()) {
bitField0_ |= 0x00000100;
realCountry_ = other.realCountry_;
onChanged();
}
if (other.hasIphoneVer()) {
bitField0_ |= 0x00000200;
iphoneVer_ = other.iphoneVer_;
onChanged();
}
if (other.hasBundleID()) {
bitField0_ |= 0x00000400;
bundleID_ = other.bundleID_;
onChanged();
}
if (other.hasOsType()) {
bitField0_ |= 0x00000800;
osType_ = other.osType_;
onChanged();
}
if (other.hasAdSource()) {
bitField0_ |= 0x00001000;
adSource_ = other.adSource_;
onChanged();
}
if (other.hasOsTypeNumber()) {
bitField0_ |= 0x00002000;
osTypeNumber_ = other.osTypeNumber_;
onChanged();
}
if (other.hasCoreCount()) {
setCoreCount(other.getCoreCount());
}
if (other.hasCarrierName()) {
bitField0_ |= 0x00008000;
carrierName_ = other.carrierName_;
onChanged();
}
if (other.hasSoftTypeXML()) {
bitField0_ |= 0x00010000;
softTypeXML_ = other.softTypeXML_;
onChanged();
}
if (other.hasClientCheckDataXML()) {
bitField0_ |= 0x00020000;
clientCheckDataXML_ = other.clientCheckDataXML_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.DeviceInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.DeviceInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object uUIDOne_ = "";
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return Whether the uUIDOne field is set.
*/
public boolean hasUUIDOne() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return The uUIDOne.
*/
public java.lang.String getUUIDOne() {
java.lang.Object ref = uUIDOne_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uUIDOne_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return The bytes for uUIDOne.
*/
public com.google.protobuf.ByteString
getUUIDOneBytes() {
java.lang.Object ref = uUIDOne_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uUIDOne_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @param value The uUIDOne to set.
* @return This builder for chaining.
*/
public Builder setUUIDOne(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
uUIDOne_ = value;
onChanged();
return this;
}
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @return This builder for chaining.
*/
public Builder clearUUIDOne() {
bitField0_ = (bitField0_ & ~0x00000001);
uUIDOne_ = getDefaultInstance().getUUIDOne();
onChanged();
return this;
}
/**
*
*`json:"uuidone"`
*
*
* optional string UUIDOne = 1;
* @param value The bytes for uUIDOne to set.
* @return This builder for chaining.
*/
public Builder setUUIDOneBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
uUIDOne_ = value;
onChanged();
return this;
}
private java.lang.Object uUIDTwo_ = "";
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return Whether the uUIDTwo field is set.
*/
public boolean hasUUIDTwo() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return The uUIDTwo.
*/
public java.lang.String getUUIDTwo() {
java.lang.Object ref = uUIDTwo_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uUIDTwo_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return The bytes for uUIDTwo.
*/
public com.google.protobuf.ByteString
getUUIDTwoBytes() {
java.lang.Object ref = uUIDTwo_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uUIDTwo_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @param value The uUIDTwo to set.
* @return This builder for chaining.
*/
public Builder setUUIDTwo(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
uUIDTwo_ = value;
onChanged();
return this;
}
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @return This builder for chaining.
*/
public Builder clearUUIDTwo() {
bitField0_ = (bitField0_ & ~0x00000002);
uUIDTwo_ = getDefaultInstance().getUUIDTwo();
onChanged();
return this;
}
/**
*
*`json:"uuidtwo"`
*
*
* optional string UUIDTwo = 2;
* @param value The bytes for uUIDTwo to set.
* @return This builder for chaining.
*/
public Builder setUUIDTwoBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
uUIDTwo_ = value;
onChanged();
return this;
}
private java.lang.Object imei_ = "";
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return Whether the imei field is set.
*/
public boolean hasImei() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return The imei.
*/
public java.lang.String getImei() {
java.lang.Object ref = imei_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
imei_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return The bytes for imei.
*/
public com.google.protobuf.ByteString
getImeiBytes() {
java.lang.Object ref = imei_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imei_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @param value The imei to set.
* @return This builder for chaining.
*/
public Builder setImei(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
imei_ = value;
onChanged();
return this;
}
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @return This builder for chaining.
*/
public Builder clearImei() {
bitField0_ = (bitField0_ & ~0x00000004);
imei_ = getDefaultInstance().getImei();
onChanged();
return this;
}
/**
*
*`json:"imei"`
*
*
* optional string Imei = 3;
* @param value The bytes for imei to set.
* @return This builder for chaining.
*/
public Builder setImeiBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
imei_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString deviceID_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @return Whether the deviceID field is set.
*/
@java.lang.Override
public boolean hasDeviceID() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @return The deviceID.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDeviceID() {
return deviceID_;
}
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @param value The deviceID to set.
* @return This builder for chaining.
*/
public Builder setDeviceID(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
deviceID_ = value;
onChanged();
return this;
}
/**
*
*`json:"deviceid"`
*
*
* optional bytes DeviceID = 4;
* @return This builder for chaining.
*/
public Builder clearDeviceID() {
bitField0_ = (bitField0_ & ~0x00000008);
deviceID_ = getDefaultInstance().getDeviceID();
onChanged();
return this;
}
private java.lang.Object deviceName_ = "";
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return Whether the deviceName field is set.
*/
public boolean hasDeviceName() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return The deviceName.
*/
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return The bytes for deviceName.
*/
public com.google.protobuf.ByteString
getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @param value The deviceName to set.
* @return This builder for chaining.
*/
public Builder setDeviceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
deviceName_ = value;
onChanged();
return this;
}
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @return This builder for chaining.
*/
public Builder clearDeviceName() {
bitField0_ = (bitField0_ & ~0x00000010);
deviceName_ = getDefaultInstance().getDeviceName();
onChanged();
return this;
}
/**
*
*`json:"devicename"`
*
*
* optional string DeviceName = 5;
* @param value The bytes for deviceName to set.
* @return This builder for chaining.
*/
public Builder setDeviceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
deviceName_ = value;
onChanged();
return this;
}
private java.lang.Object timeZone_ = "";
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return Whether the timeZone field is set.
*/
public boolean hasTimeZone() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return The timeZone.
*/
public java.lang.String getTimeZone() {
java.lang.Object ref = timeZone_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timeZone_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return The bytes for timeZone.
*/
public com.google.protobuf.ByteString
getTimeZoneBytes() {
java.lang.Object ref = timeZone_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeZone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @param value The timeZone to set.
* @return This builder for chaining.
*/
public Builder setTimeZone(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
timeZone_ = value;
onChanged();
return this;
}
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @return This builder for chaining.
*/
public Builder clearTimeZone() {
bitField0_ = (bitField0_ & ~0x00000020);
timeZone_ = getDefaultInstance().getTimeZone();
onChanged();
return this;
}
/**
*
*`json:"timezone"`
*
*
* optional string TimeZone = 6;
* @param value The bytes for timeZone to set.
* @return This builder for chaining.
*/
public Builder setTimeZoneBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
timeZone_ = value;
onChanged();
return this;
}
private java.lang.Object language_ = "";
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return Whether the language field is set.
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return The language.
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return The bytes for language.
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @param value The language to set.
* @return This builder for chaining.
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
language_ = value;
onChanged();
return this;
}
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @return This builder for chaining.
*/
public Builder clearLanguage() {
bitField0_ = (bitField0_ & ~0x00000040);
language_ = getDefaultInstance().getLanguage();
onChanged();
return this;
}
/**
*
*`json:"language"`
*
*
* optional string Language = 7;
* @param value The bytes for language to set.
* @return This builder for chaining.
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
language_ = value;
onChanged();
return this;
}
private java.lang.Object deviceBrand_ = "";
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return Whether the deviceBrand field is set.
*/
public boolean hasDeviceBrand() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return The deviceBrand.
*/
public java.lang.String getDeviceBrand() {
java.lang.Object ref = deviceBrand_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceBrand_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return The bytes for deviceBrand.
*/
public com.google.protobuf.ByteString
getDeviceBrandBytes() {
java.lang.Object ref = deviceBrand_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceBrand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @param value The deviceBrand to set.
* @return This builder for chaining.
*/
public Builder setDeviceBrand(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
deviceBrand_ = value;
onChanged();
return this;
}
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @return This builder for chaining.
*/
public Builder clearDeviceBrand() {
bitField0_ = (bitField0_ & ~0x00000080);
deviceBrand_ = getDefaultInstance().getDeviceBrand();
onChanged();
return this;
}
/**
*
*`json:"devicebrand"`
*
*
* optional string DeviceBrand = 8;
* @param value The bytes for deviceBrand to set.
* @return This builder for chaining.
*/
public Builder setDeviceBrandBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
deviceBrand_ = value;
onChanged();
return this;
}
private java.lang.Object realCountry_ = "";
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return Whether the realCountry field is set.
*/
public boolean hasRealCountry() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return The realCountry.
*/
public java.lang.String getRealCountry() {
java.lang.Object ref = realCountry_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
realCountry_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return The bytes for realCountry.
*/
public com.google.protobuf.ByteString
getRealCountryBytes() {
java.lang.Object ref = realCountry_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
realCountry_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @param value The realCountry to set.
* @return This builder for chaining.
*/
public Builder setRealCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
realCountry_ = value;
onChanged();
return this;
}
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @return This builder for chaining.
*/
public Builder clearRealCountry() {
bitField0_ = (bitField0_ & ~0x00000100);
realCountry_ = getDefaultInstance().getRealCountry();
onChanged();
return this;
}
/**
*
*`json:"realcountry"`
*
*
* optional string RealCountry = 9;
* @param value The bytes for realCountry to set.
* @return This builder for chaining.
*/
public Builder setRealCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
realCountry_ = value;
onChanged();
return this;
}
private java.lang.Object iphoneVer_ = "";
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return Whether the iphoneVer field is set.
*/
public boolean hasIphoneVer() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return The iphoneVer.
*/
public java.lang.String getIphoneVer() {
java.lang.Object ref = iphoneVer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
iphoneVer_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return The bytes for iphoneVer.
*/
public com.google.protobuf.ByteString
getIphoneVerBytes() {
java.lang.Object ref = iphoneVer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
iphoneVer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @param value The iphoneVer to set.
* @return This builder for chaining.
*/
public Builder setIphoneVer(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
iphoneVer_ = value;
onChanged();
return this;
}
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @return This builder for chaining.
*/
public Builder clearIphoneVer() {
bitField0_ = (bitField0_ & ~0x00000200);
iphoneVer_ = getDefaultInstance().getIphoneVer();
onChanged();
return this;
}
/**
*
*`json:"iphonever"`
*
*
* optional string IphoneVer = 10;
* @param value The bytes for iphoneVer to set.
* @return This builder for chaining.
*/
public Builder setIphoneVerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
iphoneVer_ = value;
onChanged();
return this;
}
private java.lang.Object bundleID_ = "";
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return Whether the bundleID field is set.
*/
public boolean hasBundleID() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return The bundleID.
*/
public java.lang.String getBundleID() {
java.lang.Object ref = bundleID_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
bundleID_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return The bytes for bundleID.
*/
public com.google.protobuf.ByteString
getBundleIDBytes() {
java.lang.Object ref = bundleID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bundleID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @param value The bundleID to set.
* @return This builder for chaining.
*/
public Builder setBundleID(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
bundleID_ = value;
onChanged();
return this;
}
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @return This builder for chaining.
*/
public Builder clearBundleID() {
bitField0_ = (bitField0_ & ~0x00000400);
bundleID_ = getDefaultInstance().getBundleID();
onChanged();
return this;
}
/**
*
*`json:"boudleid"`
*
*
* optional string BundleID = 11;
* @param value The bytes for bundleID to set.
* @return This builder for chaining.
*/
public Builder setBundleIDBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
bundleID_ = value;
onChanged();
return this;
}
private java.lang.Object osType_ = "";
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return Whether the osType field is set.
*/
public boolean hasOsType() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return The osType.
*/
public java.lang.String getOsType() {
java.lang.Object ref = osType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osType_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return The bytes for osType.
*/
public com.google.protobuf.ByteString
getOsTypeBytes() {
java.lang.Object ref = osType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @param value The osType to set.
* @return This builder for chaining.
*/
public Builder setOsType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
osType_ = value;
onChanged();
return this;
}
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @return This builder for chaining.
*/
public Builder clearOsType() {
bitField0_ = (bitField0_ & ~0x00000800);
osType_ = getDefaultInstance().getOsType();
onChanged();
return this;
}
/**
*
*`json:"ostype"`
*
*
* optional string OsType = 12;
* @param value The bytes for osType to set.
* @return This builder for chaining.
*/
public Builder setOsTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
osType_ = value;
onChanged();
return this;
}
private java.lang.Object adSource_ = "";
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return Whether the adSource field is set.
*/
public boolean hasAdSource() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return The adSource.
*/
public java.lang.String getAdSource() {
java.lang.Object ref = adSource_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
adSource_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return The bytes for adSource.
*/
public com.google.protobuf.ByteString
getAdSourceBytes() {
java.lang.Object ref = adSource_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adSource_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @param value The adSource to set.
* @return This builder for chaining.
*/
public Builder setAdSource(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
adSource_ = value;
onChanged();
return this;
}
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @return This builder for chaining.
*/
public Builder clearAdSource() {
bitField0_ = (bitField0_ & ~0x00001000);
adSource_ = getDefaultInstance().getAdSource();
onChanged();
return this;
}
/**
*
*`json:"adsource"`
*
*
* optional string AdSource = 13;
* @param value The bytes for adSource to set.
* @return This builder for chaining.
*/
public Builder setAdSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
adSource_ = value;
onChanged();
return this;
}
private java.lang.Object osTypeNumber_ = "";
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return Whether the osTypeNumber field is set.
*/
public boolean hasOsTypeNumber() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return The osTypeNumber.
*/
public java.lang.String getOsTypeNumber() {
java.lang.Object ref = osTypeNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osTypeNumber_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return The bytes for osTypeNumber.
*/
public com.google.protobuf.ByteString
getOsTypeNumberBytes() {
java.lang.Object ref = osTypeNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osTypeNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @param value The osTypeNumber to set.
* @return This builder for chaining.
*/
public Builder setOsTypeNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
osTypeNumber_ = value;
onChanged();
return this;
}
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @return This builder for chaining.
*/
public Builder clearOsTypeNumber() {
bitField0_ = (bitField0_ & ~0x00002000);
osTypeNumber_ = getDefaultInstance().getOsTypeNumber();
onChanged();
return this;
}
/**
*
*`json:"ostypenumber"`
*
*
* optional string OsTypeNumber = 14;
* @param value The bytes for osTypeNumber to set.
* @return This builder for chaining.
*/
public Builder setOsTypeNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
osTypeNumber_ = value;
onChanged();
return this;
}
private int coreCount_ ;
/**
* optional uint32 CoreCount = 15;
* @return Whether the coreCount field is set.
*/
@java.lang.Override
public boolean hasCoreCount() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
* optional uint32 CoreCount = 15;
* @return The coreCount.
*/
@java.lang.Override
public int getCoreCount() {
return coreCount_;
}
/**
* optional uint32 CoreCount = 15;
* @param value The coreCount to set.
* @return This builder for chaining.
*/
public Builder setCoreCount(int value) {
bitField0_ |= 0x00004000;
coreCount_ = value;
onChanged();
return this;
}
/**
* optional uint32 CoreCount = 15;
* @return This builder for chaining.
*/
public Builder clearCoreCount() {
bitField0_ = (bitField0_ & ~0x00004000);
coreCount_ = 0;
onChanged();
return this;
}
private java.lang.Object carrierName_ = "";
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return Whether the carrierName field is set.
*/
public boolean hasCarrierName() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return The carrierName.
*/
public java.lang.String getCarrierName() {
java.lang.Object ref = carrierName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
carrierName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return The bytes for carrierName.
*/
public com.google.protobuf.ByteString
getCarrierNameBytes() {
java.lang.Object ref = carrierName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
carrierName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @param value The carrierName to set.
* @return This builder for chaining.
*/
public Builder setCarrierName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
carrierName_ = value;
onChanged();
return this;
}
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @return This builder for chaining.
*/
public Builder clearCarrierName() {
bitField0_ = (bitField0_ & ~0x00008000);
carrierName_ = getDefaultInstance().getCarrierName();
onChanged();
return this;
}
/**
*
*`json:"carriername"`
*
*
* optional string CarrierName = 16;
* @param value The bytes for carrierName to set.
* @return This builder for chaining.
*/
public Builder setCarrierNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
carrierName_ = value;
onChanged();
return this;
}
private java.lang.Object softTypeXML_ = "";
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return Whether the softTypeXML field is set.
*/
public boolean hasSoftTypeXML() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return The softTypeXML.
*/
public java.lang.String getSoftTypeXML() {
java.lang.Object ref = softTypeXML_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
softTypeXML_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return The bytes for softTypeXML.
*/
public com.google.protobuf.ByteString
getSoftTypeXMLBytes() {
java.lang.Object ref = softTypeXML_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
softTypeXML_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @param value The softTypeXML to set.
* @return This builder for chaining.
*/
public Builder setSoftTypeXML(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
softTypeXML_ = value;
onChanged();
return this;
}
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @return This builder for chaining.
*/
public Builder clearSoftTypeXML() {
bitField0_ = (bitField0_ & ~0x00010000);
softTypeXML_ = getDefaultInstance().getSoftTypeXML();
onChanged();
return this;
}
/**
*
*`json:"softtypexml"`
*
*
* optional string SoftTypeXML = 17;
* @param value The bytes for softTypeXML to set.
* @return This builder for chaining.
*/
public Builder setSoftTypeXMLBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
softTypeXML_ = value;
onChanged();
return this;
}
private java.lang.Object clientCheckDataXML_ = "";
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return Whether the clientCheckDataXML field is set.
*/
public boolean hasClientCheckDataXML() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return The clientCheckDataXML.
*/
public java.lang.String getClientCheckDataXML() {
java.lang.Object ref = clientCheckDataXML_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientCheckDataXML_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return The bytes for clientCheckDataXML.
*/
public com.google.protobuf.ByteString
getClientCheckDataXMLBytes() {
java.lang.Object ref = clientCheckDataXML_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientCheckDataXML_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @param value The clientCheckDataXML to set.
* @return This builder for chaining.
*/
public Builder setClientCheckDataXML(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
clientCheckDataXML_ = value;
onChanged();
return this;
}
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @return This builder for chaining.
*/
public Builder clearClientCheckDataXML() {
bitField0_ = (bitField0_ & ~0x00020000);
clientCheckDataXML_ = getDefaultInstance().getClientCheckDataXML();
onChanged();
return this;
}
/**
*
*`json:"clientcheckdataxml"`
*
*
* optional string ClientCheckDataXML = 18;
* @param value The bytes for clientCheckDataXML to set.
* @return This builder for chaining.
*/
public Builder setClientCheckDataXMLBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
clientCheckDataXML_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.DeviceInfo)
}
// @@protoc_insertion_point(class_scope:rock.DeviceInfo)
private static final rock.protobuf.Rock.DeviceInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.DeviceInfo();
}
public static rock.protobuf.Rock.DeviceInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeviceInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DeviceInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.DeviceInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UserInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.UserInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return Whether the uUID field is set.
*/
boolean hasUUID();
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return The uUID.
*/
java.lang.String getUUID();
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return The bytes for uUID.
*/
com.google.protobuf.ByteString
getUUIDBytes();
/**
* optional uint32 Uin = 2;
* @return Whether the uin field is set.
*/
boolean hasUin();
/**
* optional uint32 Uin = 2;
* @return The uin.
*/
int getUin();
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return Whether the wxid field is set.
*/
boolean hasWxid();
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return The wxid.
*/
java.lang.String getWxid();
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return The bytes for wxid.
*/
com.google.protobuf.ByteString
getWxidBytes();
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return Whether the nickName field is set.
*/
boolean hasNickName();
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return The nickName.
*/
java.lang.String getNickName();
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return The bytes for nickName.
*/
com.google.protobuf.ByteString
getNickNameBytes();
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return Whether the headURL field is set.
*/
boolean hasHeadURL();
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return The headURL.
*/
java.lang.String getHeadURL();
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return The bytes for headURL.
*/
com.google.protobuf.ByteString
getHeadURLBytes();
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @return Whether the session field is set.
*/
boolean hasSession();
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @return The session.
*/
com.google.protobuf.ByteString getSession();
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @return Whether the aesKey field is set.
*/
boolean hasAesKey();
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @return The aesKey.
*/
com.google.protobuf.ByteString getAesKey();
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return Whether the shortHost field is set.
*/
boolean hasShortHost();
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return The shortHost.
*/
java.lang.String getShortHost();
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return The bytes for shortHost.
*/
com.google.protobuf.ByteString
getShortHostBytes();
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return Whether the longHost field is set.
*/
boolean hasLongHost();
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return The longHost.
*/
java.lang.String getLongHost();
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return The bytes for longHost.
*/
com.google.protobuf.ByteString
getLongHostBytes();
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @return Whether the ecPublicKey field is set.
*/
boolean hasEcPublicKey();
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @return The ecPublicKey.
*/
com.google.protobuf.ByteString getEcPublicKey();
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @return Whether the ecPrivateKey field is set.
*/
boolean hasEcPrivateKey();
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @return The ecPrivateKey.
*/
com.google.protobuf.ByteString getEcPrivateKey();
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @return Whether the checkSumKey field is set.
*/
boolean hasCheckSumKey();
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @return The checkSumKey.
*/
com.google.protobuf.ByteString getCheckSumKey();
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @return Whether the autoAuthKey field is set.
*/
boolean hasAutoAuthKey();
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @return The autoAuthKey.
*/
com.google.protobuf.ByteString getAutoAuthKey();
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @return Whether the syncKey field is set.
*/
boolean hasSyncKey();
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @return The syncKey.
*/
com.google.protobuf.ByteString getSyncKey();
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @return Whether the favSyncKey field is set.
*/
boolean hasFavSyncKey();
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @return The favSyncKey.
*/
com.google.protobuf.ByteString getFavSyncKey();
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @return Whether the snsSyncKey field is set.
*/
boolean hasSnsSyncKey();
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @return The snsSyncKey.
*/
com.google.protobuf.ByteString getSnsSyncKey();
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @return Whether the hBAesKey field is set.
*/
boolean hasHBAesKey();
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @return The hBAesKey.
*/
com.google.protobuf.ByteString getHBAesKey();
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return Whether the hBAesKeyEncrypted field is set.
*/
boolean hasHBAesKeyEncrypted();
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return The hBAesKeyEncrypted.
*/
java.lang.String getHBAesKeyEncrypted();
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return The bytes for hBAesKeyEncrypted.
*/
com.google.protobuf.ByteString
getHBAesKeyEncryptedBytes();
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
* @return Whether the dNSInfo field is set.
*/
boolean hasDNSInfo();
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
* @return The dNSInfo.
*/
wechat.protobuf.Wechat.CDNDnsInfo getDNSInfo();
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getDNSInfoOrBuilder();
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
* @return Whether the sNSDnsInfo field is set.
*/
boolean hasSNSDnsInfo();
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
* @return The sNSDnsInfo.
*/
wechat.protobuf.Wechat.CDNDnsInfo getSNSDnsInfo();
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getSNSDnsInfoOrBuilder();
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
* @return Whether the aPPDnsInfo field is set.
*/
boolean hasAPPDnsInfo();
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
* @return The aPPDnsInfo.
*/
wechat.protobuf.Wechat.CDNDnsInfo getAPPDnsInfo();
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getAPPDnsInfoOrBuilder();
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
* @return Whether the fAKEDnsInfo field is set.
*/
boolean hasFAKEDnsInfo();
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
* @return The fAKEDnsInfo.
*/
wechat.protobuf.Wechat.CDNDnsInfo getFAKEDnsInfo();
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getFAKEDnsInfoOrBuilder();
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
* @return Whether the deviceInfo field is set.
*/
boolean hasDeviceInfo();
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
* @return The deviceInfo.
*/
rock.protobuf.Rock.DeviceInfo getDeviceInfo();
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
rock.protobuf.Rock.DeviceInfoOrBuilder getDeviceInfoOrBuilder();
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @return Whether the balanceVersion field is set.
*/
boolean hasBalanceVersion();
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @return The balanceVersion.
*/
int getBalanceVersion();
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
* @return Whether the wifiInfo field is set.
*/
boolean hasWifiInfo();
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
* @return The wifiInfo.
*/
rock.protobuf.Rock.WifiInfo getWifiInfo();
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
rock.protobuf.Rock.WifiInfoOrBuilder getWifiInfoOrBuilder();
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
* @return Whether the mMInfo field is set.
*/
boolean hasMMInfo();
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
* @return The mMInfo.
*/
rock.protobuf.Rock.MMInfo getMMInfo();
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
rock.protobuf.Rock.MMInfoOrBuilder getMMInfoOrBuilder();
}
/**
*
*UserInfo 用户信息
*
*
* Protobuf type {@code rock.UserInfo}
*/
public static final class UserInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.UserInfo)
UserInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use UserInfo.newBuilder() to construct.
private UserInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserInfo() {
uUID_ = "";
wxid_ = "";
nickName_ = "";
headURL_ = "";
session_ = com.google.protobuf.ByteString.EMPTY;
aesKey_ = com.google.protobuf.ByteString.EMPTY;
shortHost_ = "";
longHost_ = "";
ecPublicKey_ = com.google.protobuf.ByteString.EMPTY;
ecPrivateKey_ = com.google.protobuf.ByteString.EMPTY;
checkSumKey_ = com.google.protobuf.ByteString.EMPTY;
autoAuthKey_ = com.google.protobuf.ByteString.EMPTY;
syncKey_ = com.google.protobuf.ByteString.EMPTY;
favSyncKey_ = com.google.protobuf.ByteString.EMPTY;
snsSyncKey_ = com.google.protobuf.ByteString.EMPTY;
hBAesKey_ = com.google.protobuf.ByteString.EMPTY;
hBAesKeyEncrypted_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new UserInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UserInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
uUID_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
uin_ = input.readUInt32();
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
wxid_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
nickName_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
headURL_ = bs;
break;
}
case 50: {
bitField0_ |= 0x00000020;
session_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
aesKey_ = input.readBytes();
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000080;
shortHost_ = bs;
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
longHost_ = bs;
break;
}
case 82: {
bitField0_ |= 0x00000200;
ecPublicKey_ = input.readBytes();
break;
}
case 90: {
bitField0_ |= 0x00000400;
ecPrivateKey_ = input.readBytes();
break;
}
case 98: {
bitField0_ |= 0x00000800;
checkSumKey_ = input.readBytes();
break;
}
case 106: {
bitField0_ |= 0x00001000;
autoAuthKey_ = input.readBytes();
break;
}
case 114: {
bitField0_ |= 0x00002000;
syncKey_ = input.readBytes();
break;
}
case 122: {
bitField0_ |= 0x00004000;
favSyncKey_ = input.readBytes();
break;
}
case 130: {
bitField0_ |= 0x00008000;
snsSyncKey_ = input.readBytes();
break;
}
case 138: {
bitField0_ |= 0x00010000;
hBAesKey_ = input.readBytes();
break;
}
case 146: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00020000;
hBAesKeyEncrypted_ = bs;
break;
}
case 154: {
wechat.protobuf.Wechat.CDNDnsInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00040000) != 0)) {
subBuilder = dNSInfo_.toBuilder();
}
dNSInfo_ = input.readMessage(wechat.protobuf.Wechat.CDNDnsInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(dNSInfo_);
dNSInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00040000;
break;
}
case 162: {
wechat.protobuf.Wechat.CDNDnsInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00080000) != 0)) {
subBuilder = sNSDnsInfo_.toBuilder();
}
sNSDnsInfo_ = input.readMessage(wechat.protobuf.Wechat.CDNDnsInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(sNSDnsInfo_);
sNSDnsInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00080000;
break;
}
case 170: {
wechat.protobuf.Wechat.CDNDnsInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00100000) != 0)) {
subBuilder = aPPDnsInfo_.toBuilder();
}
aPPDnsInfo_ = input.readMessage(wechat.protobuf.Wechat.CDNDnsInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(aPPDnsInfo_);
aPPDnsInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00100000;
break;
}
case 178: {
wechat.protobuf.Wechat.CDNDnsInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00200000) != 0)) {
subBuilder = fAKEDnsInfo_.toBuilder();
}
fAKEDnsInfo_ = input.readMessage(wechat.protobuf.Wechat.CDNDnsInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(fAKEDnsInfo_);
fAKEDnsInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00200000;
break;
}
case 186: {
rock.protobuf.Rock.DeviceInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00400000) != 0)) {
subBuilder = deviceInfo_.toBuilder();
}
deviceInfo_ = input.readMessage(rock.protobuf.Rock.DeviceInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(deviceInfo_);
deviceInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00400000;
break;
}
case 192: {
bitField0_ |= 0x00800000;
balanceVersion_ = input.readUInt32();
break;
}
case 202: {
rock.protobuf.Rock.WifiInfo.Builder subBuilder = null;
if (((bitField0_ & 0x01000000) != 0)) {
subBuilder = wifiInfo_.toBuilder();
}
wifiInfo_ = input.readMessage(rock.protobuf.Rock.WifiInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(wifiInfo_);
wifiInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x01000000;
break;
}
case 210: {
rock.protobuf.Rock.MMInfo.Builder subBuilder = null;
if (((bitField0_ & 0x02000000) != 0)) {
subBuilder = mMInfo_.toBuilder();
}
mMInfo_ = input.readMessage(rock.protobuf.Rock.MMInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(mMInfo_);
mMInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x02000000;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_UserInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_UserInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.UserInfo.class, rock.protobuf.Rock.UserInfo.Builder.class);
}
private int bitField0_;
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uUID_;
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return Whether the uUID field is set.
*/
@java.lang.Override
public boolean hasUUID() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return The uUID.
*/
@java.lang.Override
public java.lang.String getUUID() {
java.lang.Object ref = uUID_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uUID_ = s;
}
return s;
}
}
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return The bytes for uUID.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUUIDBytes() {
java.lang.Object ref = uUID_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uUID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UIN_FIELD_NUMBER = 2;
private int uin_;
/**
* optional uint32 Uin = 2;
* @return Whether the uin field is set.
*/
@java.lang.Override
public boolean hasUin() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional uint32 Uin = 2;
* @return The uin.
*/
@java.lang.Override
public int getUin() {
return uin_;
}
public static final int WXID_FIELD_NUMBER = 3;
private volatile java.lang.Object wxid_;
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return Whether the wxid field is set.
*/
@java.lang.Override
public boolean hasWxid() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return The wxid.
*/
@java.lang.Override
public java.lang.String getWxid() {
java.lang.Object ref = wxid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
wxid_ = s;
}
return s;
}
}
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return The bytes for wxid.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWxidBytes() {
java.lang.Object ref = wxid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
wxid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NICKNAME_FIELD_NUMBER = 4;
private volatile java.lang.Object nickName_;
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return Whether the nickName field is set.
*/
@java.lang.Override
public boolean hasNickName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return The nickName.
*/
@java.lang.Override
public java.lang.String getNickName() {
java.lang.Object ref = nickName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nickName_ = s;
}
return s;
}
}
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return The bytes for nickName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNickNameBytes() {
java.lang.Object ref = nickName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nickName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HEADURL_FIELD_NUMBER = 5;
private volatile java.lang.Object headURL_;
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return Whether the headURL field is set.
*/
@java.lang.Override
public boolean hasHeadURL() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return The headURL.
*/
@java.lang.Override
public java.lang.String getHeadURL() {
java.lang.Object ref = headURL_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
headURL_ = s;
}
return s;
}
}
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return The bytes for headURL.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHeadURLBytes() {
java.lang.Object ref = headURL_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
headURL_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString session_;
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @return Whether the session field is set.
*/
@java.lang.Override
public boolean hasSession() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @return The session.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSession() {
return session_;
}
public static final int AESKEY_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString aesKey_;
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @return Whether the aesKey field is set.
*/
@java.lang.Override
public boolean hasAesKey() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @return The aesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAesKey() {
return aesKey_;
}
public static final int SHORTHOST_FIELD_NUMBER = 8;
private volatile java.lang.Object shortHost_;
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return Whether the shortHost field is set.
*/
@java.lang.Override
public boolean hasShortHost() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return The shortHost.
*/
@java.lang.Override
public java.lang.String getShortHost() {
java.lang.Object ref = shortHost_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortHost_ = s;
}
return s;
}
}
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return The bytes for shortHost.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getShortHostBytes() {
java.lang.Object ref = shortHost_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LONGHOST_FIELD_NUMBER = 9;
private volatile java.lang.Object longHost_;
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return Whether the longHost field is set.
*/
@java.lang.Override
public boolean hasLongHost() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return The longHost.
*/
@java.lang.Override
public java.lang.String getLongHost() {
java.lang.Object ref = longHost_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
longHost_ = s;
}
return s;
}
}
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return The bytes for longHost.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLongHostBytes() {
java.lang.Object ref = longHost_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ECPUBLICKEY_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString ecPublicKey_;
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @return Whether the ecPublicKey field is set.
*/
@java.lang.Override
public boolean hasEcPublicKey() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @return The ecPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEcPublicKey() {
return ecPublicKey_;
}
public static final int ECPRIVATEKEY_FIELD_NUMBER = 11;
private com.google.protobuf.ByteString ecPrivateKey_;
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @return Whether the ecPrivateKey field is set.
*/
@java.lang.Override
public boolean hasEcPrivateKey() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @return The ecPrivateKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEcPrivateKey() {
return ecPrivateKey_;
}
public static final int CHECKSUMKEY_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString checkSumKey_;
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @return Whether the checkSumKey field is set.
*/
@java.lang.Override
public boolean hasCheckSumKey() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @return The checkSumKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCheckSumKey() {
return checkSumKey_;
}
public static final int AUTOAUTHKEY_FIELD_NUMBER = 13;
private com.google.protobuf.ByteString autoAuthKey_;
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @return Whether the autoAuthKey field is set.
*/
@java.lang.Override
public boolean hasAutoAuthKey() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @return The autoAuthKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAutoAuthKey() {
return autoAuthKey_;
}
public static final int SYNCKEY_FIELD_NUMBER = 14;
private com.google.protobuf.ByteString syncKey_;
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @return Whether the syncKey field is set.
*/
@java.lang.Override
public boolean hasSyncKey() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @return The syncKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSyncKey() {
return syncKey_;
}
public static final int FAVSYNCKEY_FIELD_NUMBER = 15;
private com.google.protobuf.ByteString favSyncKey_;
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @return Whether the favSyncKey field is set.
*/
@java.lang.Override
public boolean hasFavSyncKey() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @return The favSyncKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFavSyncKey() {
return favSyncKey_;
}
public static final int SNSSYNCKEY_FIELD_NUMBER = 16;
private com.google.protobuf.ByteString snsSyncKey_;
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @return Whether the snsSyncKey field is set.
*/
@java.lang.Override
public boolean hasSnsSyncKey() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @return The snsSyncKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSnsSyncKey() {
return snsSyncKey_;
}
public static final int HBAESKEY_FIELD_NUMBER = 17;
private com.google.protobuf.ByteString hBAesKey_;
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @return Whether the hBAesKey field is set.
*/
@java.lang.Override
public boolean hasHBAesKey() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @return The hBAesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHBAesKey() {
return hBAesKey_;
}
public static final int HBAESKEYENCRYPTED_FIELD_NUMBER = 18;
private volatile java.lang.Object hBAesKeyEncrypted_;
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return Whether the hBAesKeyEncrypted field is set.
*/
@java.lang.Override
public boolean hasHBAesKeyEncrypted() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return The hBAesKeyEncrypted.
*/
@java.lang.Override
public java.lang.String getHBAesKeyEncrypted() {
java.lang.Object ref = hBAesKeyEncrypted_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hBAesKeyEncrypted_ = s;
}
return s;
}
}
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return The bytes for hBAesKeyEncrypted.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHBAesKeyEncryptedBytes() {
java.lang.Object ref = hBAesKeyEncrypted_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hBAesKeyEncrypted_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DNSINFO_FIELD_NUMBER = 19;
private wechat.protobuf.Wechat.CDNDnsInfo dNSInfo_;
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
* @return Whether the dNSInfo field is set.
*/
@java.lang.Override
public boolean hasDNSInfo() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
* @return The dNSInfo.
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfo getDNSInfo() {
return dNSInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : dNSInfo_;
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getDNSInfoOrBuilder() {
return dNSInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : dNSInfo_;
}
public static final int SNSDNSINFO_FIELD_NUMBER = 20;
private wechat.protobuf.Wechat.CDNDnsInfo sNSDnsInfo_;
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
* @return Whether the sNSDnsInfo field is set.
*/
@java.lang.Override
public boolean hasSNSDnsInfo() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
* @return The sNSDnsInfo.
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfo getSNSDnsInfo() {
return sNSDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : sNSDnsInfo_;
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getSNSDnsInfoOrBuilder() {
return sNSDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : sNSDnsInfo_;
}
public static final int APPDNSINFO_FIELD_NUMBER = 21;
private wechat.protobuf.Wechat.CDNDnsInfo aPPDnsInfo_;
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
* @return Whether the aPPDnsInfo field is set.
*/
@java.lang.Override
public boolean hasAPPDnsInfo() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
* @return The aPPDnsInfo.
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfo getAPPDnsInfo() {
return aPPDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : aPPDnsInfo_;
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getAPPDnsInfoOrBuilder() {
return aPPDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : aPPDnsInfo_;
}
public static final int FAKEDNSINFO_FIELD_NUMBER = 22;
private wechat.protobuf.Wechat.CDNDnsInfo fAKEDnsInfo_;
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
* @return Whether the fAKEDnsInfo field is set.
*/
@java.lang.Override
public boolean hasFAKEDnsInfo() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
* @return The fAKEDnsInfo.
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfo getFAKEDnsInfo() {
return fAKEDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : fAKEDnsInfo_;
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
@java.lang.Override
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getFAKEDnsInfoOrBuilder() {
return fAKEDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : fAKEDnsInfo_;
}
public static final int DEVICEINFO_FIELD_NUMBER = 23;
private rock.protobuf.Rock.DeviceInfo deviceInfo_;
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
* @return Whether the deviceInfo field is set.
*/
@java.lang.Override
public boolean hasDeviceInfo() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
* @return The deviceInfo.
*/
@java.lang.Override
public rock.protobuf.Rock.DeviceInfo getDeviceInfo() {
return deviceInfo_ == null ? rock.protobuf.Rock.DeviceInfo.getDefaultInstance() : deviceInfo_;
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
@java.lang.Override
public rock.protobuf.Rock.DeviceInfoOrBuilder getDeviceInfoOrBuilder() {
return deviceInfo_ == null ? rock.protobuf.Rock.DeviceInfo.getDefaultInstance() : deviceInfo_;
}
public static final int BALANCEVERSION_FIELD_NUMBER = 24;
private int balanceVersion_;
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @return Whether the balanceVersion field is set.
*/
@java.lang.Override
public boolean hasBalanceVersion() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @return The balanceVersion.
*/
@java.lang.Override
public int getBalanceVersion() {
return balanceVersion_;
}
public static final int WIFIINFO_FIELD_NUMBER = 25;
private rock.protobuf.Rock.WifiInfo wifiInfo_;
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
* @return Whether the wifiInfo field is set.
*/
@java.lang.Override
public boolean hasWifiInfo() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
* @return The wifiInfo.
*/
@java.lang.Override
public rock.protobuf.Rock.WifiInfo getWifiInfo() {
return wifiInfo_ == null ? rock.protobuf.Rock.WifiInfo.getDefaultInstance() : wifiInfo_;
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
@java.lang.Override
public rock.protobuf.Rock.WifiInfoOrBuilder getWifiInfoOrBuilder() {
return wifiInfo_ == null ? rock.protobuf.Rock.WifiInfo.getDefaultInstance() : wifiInfo_;
}
public static final int MMINFO_FIELD_NUMBER = 26;
private rock.protobuf.Rock.MMInfo mMInfo_;
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
* @return Whether the mMInfo field is set.
*/
@java.lang.Override
public boolean hasMMInfo() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
* @return The mMInfo.
*/
@java.lang.Override
public rock.protobuf.Rock.MMInfo getMMInfo() {
return mMInfo_ == null ? rock.protobuf.Rock.MMInfo.getDefaultInstance() : mMInfo_;
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
@java.lang.Override
public rock.protobuf.Rock.MMInfoOrBuilder getMMInfoOrBuilder() {
return mMInfo_ == null ? rock.protobuf.Rock.MMInfo.getDefaultInstance() : mMInfo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uUID_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeUInt32(2, uin_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, wxid_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, nickName_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, headURL_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBytes(6, session_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBytes(7, aesKey_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, shortHost_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, longHost_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeBytes(10, ecPublicKey_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeBytes(11, ecPrivateKey_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeBytes(12, checkSumKey_);
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeBytes(13, autoAuthKey_);
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeBytes(14, syncKey_);
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeBytes(15, favSyncKey_);
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeBytes(16, snsSyncKey_);
}
if (((bitField0_ & 0x00010000) != 0)) {
output.writeBytes(17, hBAesKey_);
}
if (((bitField0_ & 0x00020000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, hBAesKeyEncrypted_);
}
if (((bitField0_ & 0x00040000) != 0)) {
output.writeMessage(19, getDNSInfo());
}
if (((bitField0_ & 0x00080000) != 0)) {
output.writeMessage(20, getSNSDnsInfo());
}
if (((bitField0_ & 0x00100000) != 0)) {
output.writeMessage(21, getAPPDnsInfo());
}
if (((bitField0_ & 0x00200000) != 0)) {
output.writeMessage(22, getFAKEDnsInfo());
}
if (((bitField0_ & 0x00400000) != 0)) {
output.writeMessage(23, getDeviceInfo());
}
if (((bitField0_ & 0x00800000) != 0)) {
output.writeUInt32(24, balanceVersion_);
}
if (((bitField0_ & 0x01000000) != 0)) {
output.writeMessage(25, getWifiInfo());
}
if (((bitField0_ & 0x02000000) != 0)) {
output.writeMessage(26, getMMInfo());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uUID_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, uin_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, wxid_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, nickName_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, headURL_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, session_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, aesKey_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, shortHost_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, longHost_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, ecPublicKey_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(11, ecPrivateKey_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, checkSumKey_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, autoAuthKey_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(14, syncKey_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(15, favSyncKey_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(16, snsSyncKey_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(17, hBAesKey_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, hBAesKeyEncrypted_);
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, getDNSInfo());
}
if (((bitField0_ & 0x00080000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, getSNSDnsInfo());
}
if (((bitField0_ & 0x00100000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getAPPDnsInfo());
}
if (((bitField0_ & 0x00200000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, getFAKEDnsInfo());
}
if (((bitField0_ & 0x00400000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, getDeviceInfo());
}
if (((bitField0_ & 0x00800000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(24, balanceVersion_);
}
if (((bitField0_ & 0x01000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(25, getWifiInfo());
}
if (((bitField0_ & 0x02000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, getMMInfo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.UserInfo)) {
return super.equals(obj);
}
rock.protobuf.Rock.UserInfo other = (rock.protobuf.Rock.UserInfo) obj;
if (hasUUID() != other.hasUUID()) return false;
if (hasUUID()) {
if (!getUUID()
.equals(other.getUUID())) return false;
}
if (hasUin() != other.hasUin()) return false;
if (hasUin()) {
if (getUin()
!= other.getUin()) return false;
}
if (hasWxid() != other.hasWxid()) return false;
if (hasWxid()) {
if (!getWxid()
.equals(other.getWxid())) return false;
}
if (hasNickName() != other.hasNickName()) return false;
if (hasNickName()) {
if (!getNickName()
.equals(other.getNickName())) return false;
}
if (hasHeadURL() != other.hasHeadURL()) return false;
if (hasHeadURL()) {
if (!getHeadURL()
.equals(other.getHeadURL())) return false;
}
if (hasSession() != other.hasSession()) return false;
if (hasSession()) {
if (!getSession()
.equals(other.getSession())) return false;
}
if (hasAesKey() != other.hasAesKey()) return false;
if (hasAesKey()) {
if (!getAesKey()
.equals(other.getAesKey())) return false;
}
if (hasShortHost() != other.hasShortHost()) return false;
if (hasShortHost()) {
if (!getShortHost()
.equals(other.getShortHost())) return false;
}
if (hasLongHost() != other.hasLongHost()) return false;
if (hasLongHost()) {
if (!getLongHost()
.equals(other.getLongHost())) return false;
}
if (hasEcPublicKey() != other.hasEcPublicKey()) return false;
if (hasEcPublicKey()) {
if (!getEcPublicKey()
.equals(other.getEcPublicKey())) return false;
}
if (hasEcPrivateKey() != other.hasEcPrivateKey()) return false;
if (hasEcPrivateKey()) {
if (!getEcPrivateKey()
.equals(other.getEcPrivateKey())) return false;
}
if (hasCheckSumKey() != other.hasCheckSumKey()) return false;
if (hasCheckSumKey()) {
if (!getCheckSumKey()
.equals(other.getCheckSumKey())) return false;
}
if (hasAutoAuthKey() != other.hasAutoAuthKey()) return false;
if (hasAutoAuthKey()) {
if (!getAutoAuthKey()
.equals(other.getAutoAuthKey())) return false;
}
if (hasSyncKey() != other.hasSyncKey()) return false;
if (hasSyncKey()) {
if (!getSyncKey()
.equals(other.getSyncKey())) return false;
}
if (hasFavSyncKey() != other.hasFavSyncKey()) return false;
if (hasFavSyncKey()) {
if (!getFavSyncKey()
.equals(other.getFavSyncKey())) return false;
}
if (hasSnsSyncKey() != other.hasSnsSyncKey()) return false;
if (hasSnsSyncKey()) {
if (!getSnsSyncKey()
.equals(other.getSnsSyncKey())) return false;
}
if (hasHBAesKey() != other.hasHBAesKey()) return false;
if (hasHBAesKey()) {
if (!getHBAesKey()
.equals(other.getHBAesKey())) return false;
}
if (hasHBAesKeyEncrypted() != other.hasHBAesKeyEncrypted()) return false;
if (hasHBAesKeyEncrypted()) {
if (!getHBAesKeyEncrypted()
.equals(other.getHBAesKeyEncrypted())) return false;
}
if (hasDNSInfo() != other.hasDNSInfo()) return false;
if (hasDNSInfo()) {
if (!getDNSInfo()
.equals(other.getDNSInfo())) return false;
}
if (hasSNSDnsInfo() != other.hasSNSDnsInfo()) return false;
if (hasSNSDnsInfo()) {
if (!getSNSDnsInfo()
.equals(other.getSNSDnsInfo())) return false;
}
if (hasAPPDnsInfo() != other.hasAPPDnsInfo()) return false;
if (hasAPPDnsInfo()) {
if (!getAPPDnsInfo()
.equals(other.getAPPDnsInfo())) return false;
}
if (hasFAKEDnsInfo() != other.hasFAKEDnsInfo()) return false;
if (hasFAKEDnsInfo()) {
if (!getFAKEDnsInfo()
.equals(other.getFAKEDnsInfo())) return false;
}
if (hasDeviceInfo() != other.hasDeviceInfo()) return false;
if (hasDeviceInfo()) {
if (!getDeviceInfo()
.equals(other.getDeviceInfo())) return false;
}
if (hasBalanceVersion() != other.hasBalanceVersion()) return false;
if (hasBalanceVersion()) {
if (getBalanceVersion()
!= other.getBalanceVersion()) return false;
}
if (hasWifiInfo() != other.hasWifiInfo()) return false;
if (hasWifiInfo()) {
if (!getWifiInfo()
.equals(other.getWifiInfo())) return false;
}
if (hasMMInfo() != other.hasMMInfo()) return false;
if (hasMMInfo()) {
if (!getMMInfo()
.equals(other.getMMInfo())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUUID()) {
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUUID().hashCode();
}
if (hasUin()) {
hash = (37 * hash) + UIN_FIELD_NUMBER;
hash = (53 * hash) + getUin();
}
if (hasWxid()) {
hash = (37 * hash) + WXID_FIELD_NUMBER;
hash = (53 * hash) + getWxid().hashCode();
}
if (hasNickName()) {
hash = (37 * hash) + NICKNAME_FIELD_NUMBER;
hash = (53 * hash) + getNickName().hashCode();
}
if (hasHeadURL()) {
hash = (37 * hash) + HEADURL_FIELD_NUMBER;
hash = (53 * hash) + getHeadURL().hashCode();
}
if (hasSession()) {
hash = (37 * hash) + SESSION_FIELD_NUMBER;
hash = (53 * hash) + getSession().hashCode();
}
if (hasAesKey()) {
hash = (37 * hash) + AESKEY_FIELD_NUMBER;
hash = (53 * hash) + getAesKey().hashCode();
}
if (hasShortHost()) {
hash = (37 * hash) + SHORTHOST_FIELD_NUMBER;
hash = (53 * hash) + getShortHost().hashCode();
}
if (hasLongHost()) {
hash = (37 * hash) + LONGHOST_FIELD_NUMBER;
hash = (53 * hash) + getLongHost().hashCode();
}
if (hasEcPublicKey()) {
hash = (37 * hash) + ECPUBLICKEY_FIELD_NUMBER;
hash = (53 * hash) + getEcPublicKey().hashCode();
}
if (hasEcPrivateKey()) {
hash = (37 * hash) + ECPRIVATEKEY_FIELD_NUMBER;
hash = (53 * hash) + getEcPrivateKey().hashCode();
}
if (hasCheckSumKey()) {
hash = (37 * hash) + CHECKSUMKEY_FIELD_NUMBER;
hash = (53 * hash) + getCheckSumKey().hashCode();
}
if (hasAutoAuthKey()) {
hash = (37 * hash) + AUTOAUTHKEY_FIELD_NUMBER;
hash = (53 * hash) + getAutoAuthKey().hashCode();
}
if (hasSyncKey()) {
hash = (37 * hash) + SYNCKEY_FIELD_NUMBER;
hash = (53 * hash) + getSyncKey().hashCode();
}
if (hasFavSyncKey()) {
hash = (37 * hash) + FAVSYNCKEY_FIELD_NUMBER;
hash = (53 * hash) + getFavSyncKey().hashCode();
}
if (hasSnsSyncKey()) {
hash = (37 * hash) + SNSSYNCKEY_FIELD_NUMBER;
hash = (53 * hash) + getSnsSyncKey().hashCode();
}
if (hasHBAesKey()) {
hash = (37 * hash) + HBAESKEY_FIELD_NUMBER;
hash = (53 * hash) + getHBAesKey().hashCode();
}
if (hasHBAesKeyEncrypted()) {
hash = (37 * hash) + HBAESKEYENCRYPTED_FIELD_NUMBER;
hash = (53 * hash) + getHBAesKeyEncrypted().hashCode();
}
if (hasDNSInfo()) {
hash = (37 * hash) + DNSINFO_FIELD_NUMBER;
hash = (53 * hash) + getDNSInfo().hashCode();
}
if (hasSNSDnsInfo()) {
hash = (37 * hash) + SNSDNSINFO_FIELD_NUMBER;
hash = (53 * hash) + getSNSDnsInfo().hashCode();
}
if (hasAPPDnsInfo()) {
hash = (37 * hash) + APPDNSINFO_FIELD_NUMBER;
hash = (53 * hash) + getAPPDnsInfo().hashCode();
}
if (hasFAKEDnsInfo()) {
hash = (37 * hash) + FAKEDNSINFO_FIELD_NUMBER;
hash = (53 * hash) + getFAKEDnsInfo().hashCode();
}
if (hasDeviceInfo()) {
hash = (37 * hash) + DEVICEINFO_FIELD_NUMBER;
hash = (53 * hash) + getDeviceInfo().hashCode();
}
if (hasBalanceVersion()) {
hash = (37 * hash) + BALANCEVERSION_FIELD_NUMBER;
hash = (53 * hash) + getBalanceVersion();
}
if (hasWifiInfo()) {
hash = (37 * hash) + WIFIINFO_FIELD_NUMBER;
hash = (53 * hash) + getWifiInfo().hashCode();
}
if (hasMMInfo()) {
hash = (37 * hash) + MMINFO_FIELD_NUMBER;
hash = (53 * hash) + getMMInfo().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.UserInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.UserInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.UserInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.UserInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.UserInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.UserInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.UserInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.UserInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.UserInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.UserInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.UserInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.UserInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.UserInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*UserInfo 用户信息
*
*
* Protobuf type {@code rock.UserInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.UserInfo)
rock.protobuf.Rock.UserInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_UserInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_UserInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.UserInfo.class, rock.protobuf.Rock.UserInfo.Builder.class);
}
// Construct using rock.protobuf.Rock.UserInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDNSInfoFieldBuilder();
getSNSDnsInfoFieldBuilder();
getAPPDnsInfoFieldBuilder();
getFAKEDnsInfoFieldBuilder();
getDeviceInfoFieldBuilder();
getWifiInfoFieldBuilder();
getMMInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uUID_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
uin_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
wxid_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
nickName_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
headURL_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
session_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
aesKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
shortHost_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
longHost_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
ecPublicKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000200);
ecPrivateKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000400);
checkSumKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000800);
autoAuthKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00001000);
syncKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00002000);
favSyncKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00004000);
snsSyncKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00008000);
hBAesKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00010000);
hBAesKeyEncrypted_ = "";
bitField0_ = (bitField0_ & ~0x00020000);
if (dNSInfoBuilder_ == null) {
dNSInfo_ = null;
} else {
dNSInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00040000);
if (sNSDnsInfoBuilder_ == null) {
sNSDnsInfo_ = null;
} else {
sNSDnsInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00080000);
if (aPPDnsInfoBuilder_ == null) {
aPPDnsInfo_ = null;
} else {
aPPDnsInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00100000);
if (fAKEDnsInfoBuilder_ == null) {
fAKEDnsInfo_ = null;
} else {
fAKEDnsInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00200000);
if (deviceInfoBuilder_ == null) {
deviceInfo_ = null;
} else {
deviceInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00400000);
balanceVersion_ = 0;
bitField0_ = (bitField0_ & ~0x00800000);
if (wifiInfoBuilder_ == null) {
wifiInfo_ = null;
} else {
wifiInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x01000000);
if (mMInfoBuilder_ == null) {
mMInfo_ = null;
} else {
mMInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x02000000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_UserInfo_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.UserInfo getDefaultInstanceForType() {
return rock.protobuf.Rock.UserInfo.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.UserInfo build() {
rock.protobuf.Rock.UserInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.UserInfo buildPartial() {
rock.protobuf.Rock.UserInfo result = new rock.protobuf.Rock.UserInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.uUID_ = uUID_;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.uin_ = uin_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.wxid_ = wxid_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.nickName_ = nickName_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.headURL_ = headURL_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.session_ = session_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.aesKey_ = aesKey_;
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.shortHost_ = shortHost_;
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.longHost_ = longHost_;
if (((from_bitField0_ & 0x00000200) != 0)) {
to_bitField0_ |= 0x00000200;
}
result.ecPublicKey_ = ecPublicKey_;
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000400;
}
result.ecPrivateKey_ = ecPrivateKey_;
if (((from_bitField0_ & 0x00000800) != 0)) {
to_bitField0_ |= 0x00000800;
}
result.checkSumKey_ = checkSumKey_;
if (((from_bitField0_ & 0x00001000) != 0)) {
to_bitField0_ |= 0x00001000;
}
result.autoAuthKey_ = autoAuthKey_;
if (((from_bitField0_ & 0x00002000) != 0)) {
to_bitField0_ |= 0x00002000;
}
result.syncKey_ = syncKey_;
if (((from_bitField0_ & 0x00004000) != 0)) {
to_bitField0_ |= 0x00004000;
}
result.favSyncKey_ = favSyncKey_;
if (((from_bitField0_ & 0x00008000) != 0)) {
to_bitField0_ |= 0x00008000;
}
result.snsSyncKey_ = snsSyncKey_;
if (((from_bitField0_ & 0x00010000) != 0)) {
to_bitField0_ |= 0x00010000;
}
result.hBAesKey_ = hBAesKey_;
if (((from_bitField0_ & 0x00020000) != 0)) {
to_bitField0_ |= 0x00020000;
}
result.hBAesKeyEncrypted_ = hBAesKeyEncrypted_;
if (((from_bitField0_ & 0x00040000) != 0)) {
if (dNSInfoBuilder_ == null) {
result.dNSInfo_ = dNSInfo_;
} else {
result.dNSInfo_ = dNSInfoBuilder_.build();
}
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
if (sNSDnsInfoBuilder_ == null) {
result.sNSDnsInfo_ = sNSDnsInfo_;
} else {
result.sNSDnsInfo_ = sNSDnsInfoBuilder_.build();
}
to_bitField0_ |= 0x00080000;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
if (aPPDnsInfoBuilder_ == null) {
result.aPPDnsInfo_ = aPPDnsInfo_;
} else {
result.aPPDnsInfo_ = aPPDnsInfoBuilder_.build();
}
to_bitField0_ |= 0x00100000;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
if (fAKEDnsInfoBuilder_ == null) {
result.fAKEDnsInfo_ = fAKEDnsInfo_;
} else {
result.fAKEDnsInfo_ = fAKEDnsInfoBuilder_.build();
}
to_bitField0_ |= 0x00200000;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
if (deviceInfoBuilder_ == null) {
result.deviceInfo_ = deviceInfo_;
} else {
result.deviceInfo_ = deviceInfoBuilder_.build();
}
to_bitField0_ |= 0x00400000;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.balanceVersion_ = balanceVersion_;
to_bitField0_ |= 0x00800000;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
if (wifiInfoBuilder_ == null) {
result.wifiInfo_ = wifiInfo_;
} else {
result.wifiInfo_ = wifiInfoBuilder_.build();
}
to_bitField0_ |= 0x01000000;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
if (mMInfoBuilder_ == null) {
result.mMInfo_ = mMInfo_;
} else {
result.mMInfo_ = mMInfoBuilder_.build();
}
to_bitField0_ |= 0x02000000;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.UserInfo) {
return mergeFrom((rock.protobuf.Rock.UserInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.UserInfo other) {
if (other == rock.protobuf.Rock.UserInfo.getDefaultInstance()) return this;
if (other.hasUUID()) {
bitField0_ |= 0x00000001;
uUID_ = other.uUID_;
onChanged();
}
if (other.hasUin()) {
setUin(other.getUin());
}
if (other.hasWxid()) {
bitField0_ |= 0x00000004;
wxid_ = other.wxid_;
onChanged();
}
if (other.hasNickName()) {
bitField0_ |= 0x00000008;
nickName_ = other.nickName_;
onChanged();
}
if (other.hasHeadURL()) {
bitField0_ |= 0x00000010;
headURL_ = other.headURL_;
onChanged();
}
if (other.hasSession()) {
setSession(other.getSession());
}
if (other.hasAesKey()) {
setAesKey(other.getAesKey());
}
if (other.hasShortHost()) {
bitField0_ |= 0x00000080;
shortHost_ = other.shortHost_;
onChanged();
}
if (other.hasLongHost()) {
bitField0_ |= 0x00000100;
longHost_ = other.longHost_;
onChanged();
}
if (other.hasEcPublicKey()) {
setEcPublicKey(other.getEcPublicKey());
}
if (other.hasEcPrivateKey()) {
setEcPrivateKey(other.getEcPrivateKey());
}
if (other.hasCheckSumKey()) {
setCheckSumKey(other.getCheckSumKey());
}
if (other.hasAutoAuthKey()) {
setAutoAuthKey(other.getAutoAuthKey());
}
if (other.hasSyncKey()) {
setSyncKey(other.getSyncKey());
}
if (other.hasFavSyncKey()) {
setFavSyncKey(other.getFavSyncKey());
}
if (other.hasSnsSyncKey()) {
setSnsSyncKey(other.getSnsSyncKey());
}
if (other.hasHBAesKey()) {
setHBAesKey(other.getHBAesKey());
}
if (other.hasHBAesKeyEncrypted()) {
bitField0_ |= 0x00020000;
hBAesKeyEncrypted_ = other.hBAesKeyEncrypted_;
onChanged();
}
if (other.hasDNSInfo()) {
mergeDNSInfo(other.getDNSInfo());
}
if (other.hasSNSDnsInfo()) {
mergeSNSDnsInfo(other.getSNSDnsInfo());
}
if (other.hasAPPDnsInfo()) {
mergeAPPDnsInfo(other.getAPPDnsInfo());
}
if (other.hasFAKEDnsInfo()) {
mergeFAKEDnsInfo(other.getFAKEDnsInfo());
}
if (other.hasDeviceInfo()) {
mergeDeviceInfo(other.getDeviceInfo());
}
if (other.hasBalanceVersion()) {
setBalanceVersion(other.getBalanceVersion());
}
if (other.hasWifiInfo()) {
mergeWifiInfo(other.getWifiInfo());
}
if (other.hasMMInfo()) {
mergeMMInfo(other.getMMInfo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.UserInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.UserInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object uUID_ = "";
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return Whether the uUID field is set.
*/
public boolean hasUUID() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return The uUID.
*/
public java.lang.String getUUID() {
java.lang.Object ref = uUID_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uUID_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return The bytes for uUID.
*/
public com.google.protobuf.ByteString
getUUIDBytes() {
java.lang.Object ref = uUID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uUID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @param value The uUID to set.
* @return This builder for chaining.
*/
public Builder setUUID(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
uUID_ = value;
onChanged();
return this;
}
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @return This builder for chaining.
*/
public Builder clearUUID() {
bitField0_ = (bitField0_ & ~0x00000001);
uUID_ = getDefaultInstance().getUUID();
onChanged();
return this;
}
/**
*
*`json:"uuid"`
*
*
* optional string UUID = 1;
* @param value The bytes for uUID to set.
* @return This builder for chaining.
*/
public Builder setUUIDBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
uUID_ = value;
onChanged();
return this;
}
private int uin_ ;
/**
* optional uint32 Uin = 2;
* @return Whether the uin field is set.
*/
@java.lang.Override
public boolean hasUin() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional uint32 Uin = 2;
* @return The uin.
*/
@java.lang.Override
public int getUin() {
return uin_;
}
/**
* optional uint32 Uin = 2;
* @param value The uin to set.
* @return This builder for chaining.
*/
public Builder setUin(int value) {
bitField0_ |= 0x00000002;
uin_ = value;
onChanged();
return this;
}
/**
* optional uint32 Uin = 2;
* @return This builder for chaining.
*/
public Builder clearUin() {
bitField0_ = (bitField0_ & ~0x00000002);
uin_ = 0;
onChanged();
return this;
}
private java.lang.Object wxid_ = "";
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return Whether the wxid field is set.
*/
public boolean hasWxid() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return The wxid.
*/
public java.lang.String getWxid() {
java.lang.Object ref = wxid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
wxid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return The bytes for wxid.
*/
public com.google.protobuf.ByteString
getWxidBytes() {
java.lang.Object ref = wxid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
wxid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @param value The wxid to set.
* @return This builder for chaining.
*/
public Builder setWxid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
wxid_ = value;
onChanged();
return this;
}
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @return This builder for chaining.
*/
public Builder clearWxid() {
bitField0_ = (bitField0_ & ~0x00000004);
wxid_ = getDefaultInstance().getWxid();
onChanged();
return this;
}
/**
*
*`json:"wxid"`
*
*
* optional string Wxid = 3;
* @param value The bytes for wxid to set.
* @return This builder for chaining.
*/
public Builder setWxidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
wxid_ = value;
onChanged();
return this;
}
private java.lang.Object nickName_ = "";
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return Whether the nickName field is set.
*/
public boolean hasNickName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return The nickName.
*/
public java.lang.String getNickName() {
java.lang.Object ref = nickName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nickName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return The bytes for nickName.
*/
public com.google.protobuf.ByteString
getNickNameBytes() {
java.lang.Object ref = nickName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nickName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @param value The nickName to set.
* @return This builder for chaining.
*/
public Builder setNickName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
nickName_ = value;
onChanged();
return this;
}
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @return This builder for chaining.
*/
public Builder clearNickName() {
bitField0_ = (bitField0_ & ~0x00000008);
nickName_ = getDefaultInstance().getNickName();
onChanged();
return this;
}
/**
*
*`json:"nickname"`
*
*
* optional string NickName = 4;
* @param value The bytes for nickName to set.
* @return This builder for chaining.
*/
public Builder setNickNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
nickName_ = value;
onChanged();
return this;
}
private java.lang.Object headURL_ = "";
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return Whether the headURL field is set.
*/
public boolean hasHeadURL() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return The headURL.
*/
public java.lang.String getHeadURL() {
java.lang.Object ref = headURL_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
headURL_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return The bytes for headURL.
*/
public com.google.protobuf.ByteString
getHeadURLBytes() {
java.lang.Object ref = headURL_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
headURL_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @param value The headURL to set.
* @return This builder for chaining.
*/
public Builder setHeadURL(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
headURL_ = value;
onChanged();
return this;
}
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @return This builder for chaining.
*/
public Builder clearHeadURL() {
bitField0_ = (bitField0_ & ~0x00000010);
headURL_ = getDefaultInstance().getHeadURL();
onChanged();
return this;
}
/**
*
*`json:"headurl"`
*
*
* optional string HeadURL = 5;
* @param value The bytes for headURL to set.
* @return This builder for chaining.
*/
public Builder setHeadURLBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
headURL_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString session_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @return Whether the session field is set.
*/
@java.lang.Override
public boolean hasSession() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @return The session.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSession() {
return session_;
}
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @param value The session to set.
* @return This builder for chaining.
*/
public Builder setSession(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
session_ = value;
onChanged();
return this;
}
/**
*
*`json:"cookie"`
*
*
* optional bytes Session = 6;
* @return This builder for chaining.
*/
public Builder clearSession() {
bitField0_ = (bitField0_ & ~0x00000020);
session_ = getDefaultInstance().getSession();
onChanged();
return this;
}
private com.google.protobuf.ByteString aesKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @return Whether the aesKey field is set.
*/
@java.lang.Override
public boolean hasAesKey() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @return The aesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAesKey() {
return aesKey_;
}
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @param value The aesKey to set.
* @return This builder for chaining.
*/
public Builder setAesKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
aesKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"aeskey"`
*
*
* optional bytes AesKey = 7;
* @return This builder for chaining.
*/
public Builder clearAesKey() {
bitField0_ = (bitField0_ & ~0x00000040);
aesKey_ = getDefaultInstance().getAesKey();
onChanged();
return this;
}
private java.lang.Object shortHost_ = "";
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return Whether the shortHost field is set.
*/
public boolean hasShortHost() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return The shortHost.
*/
public java.lang.String getShortHost() {
java.lang.Object ref = shortHost_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortHost_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return The bytes for shortHost.
*/
public com.google.protobuf.ByteString
getShortHostBytes() {
java.lang.Object ref = shortHost_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @param value The shortHost to set.
* @return This builder for chaining.
*/
public Builder setShortHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
shortHost_ = value;
onChanged();
return this;
}
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @return This builder for chaining.
*/
public Builder clearShortHost() {
bitField0_ = (bitField0_ & ~0x00000080);
shortHost_ = getDefaultInstance().getShortHost();
onChanged();
return this;
}
/**
*
*`json:"shorthost"`
*
*
* optional string ShortHost = 8;
* @param value The bytes for shortHost to set.
* @return This builder for chaining.
*/
public Builder setShortHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
shortHost_ = value;
onChanged();
return this;
}
private java.lang.Object longHost_ = "";
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return Whether the longHost field is set.
*/
public boolean hasLongHost() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return The longHost.
*/
public java.lang.String getLongHost() {
java.lang.Object ref = longHost_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
longHost_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return The bytes for longHost.
*/
public com.google.protobuf.ByteString
getLongHostBytes() {
java.lang.Object ref = longHost_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @param value The longHost to set.
* @return This builder for chaining.
*/
public Builder setLongHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
longHost_ = value;
onChanged();
return this;
}
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @return This builder for chaining.
*/
public Builder clearLongHost() {
bitField0_ = (bitField0_ & ~0x00000100);
longHost_ = getDefaultInstance().getLongHost();
onChanged();
return this;
}
/**
*
*`json:"longhost"`
*
*
* optional string LongHost = 9;
* @param value The bytes for longHost to set.
* @return This builder for chaining.
*/
public Builder setLongHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
longHost_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString ecPublicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @return Whether the ecPublicKey field is set.
*/
@java.lang.Override
public boolean hasEcPublicKey() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @return The ecPublicKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEcPublicKey() {
return ecPublicKey_;
}
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @param value The ecPublicKey to set.
* @return This builder for chaining.
*/
public Builder setEcPublicKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
ecPublicKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"ecpukey"`
*
*
* optional bytes EcPublicKey = 10;
* @return This builder for chaining.
*/
public Builder clearEcPublicKey() {
bitField0_ = (bitField0_ & ~0x00000200);
ecPublicKey_ = getDefaultInstance().getEcPublicKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString ecPrivateKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @return Whether the ecPrivateKey field is set.
*/
@java.lang.Override
public boolean hasEcPrivateKey() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @return The ecPrivateKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEcPrivateKey() {
return ecPrivateKey_;
}
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @param value The ecPrivateKey to set.
* @return This builder for chaining.
*/
public Builder setEcPrivateKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
ecPrivateKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"ecprkey"`
*
*
* optional bytes EcPrivateKey = 11;
* @return This builder for chaining.
*/
public Builder clearEcPrivateKey() {
bitField0_ = (bitField0_ & ~0x00000400);
ecPrivateKey_ = getDefaultInstance().getEcPrivateKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString checkSumKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @return Whether the checkSumKey field is set.
*/
@java.lang.Override
public boolean hasCheckSumKey() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @return The checkSumKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCheckSumKey() {
return checkSumKey_;
}
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @param value The checkSumKey to set.
* @return This builder for chaining.
*/
public Builder setCheckSumKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
checkSumKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"checksumkey"`
*
*
* optional bytes CheckSumKey = 12;
* @return This builder for chaining.
*/
public Builder clearCheckSumKey() {
bitField0_ = (bitField0_ & ~0x00000800);
checkSumKey_ = getDefaultInstance().getCheckSumKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString autoAuthKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @return Whether the autoAuthKey field is set.
*/
@java.lang.Override
public boolean hasAutoAuthKey() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @return The autoAuthKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAutoAuthKey() {
return autoAuthKey_;
}
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @param value The autoAuthKey to set.
* @return This builder for chaining.
*/
public Builder setAutoAuthKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
autoAuthKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"autoauthkey"`
*
*
* optional bytes AutoAuthKey = 13;
* @return This builder for chaining.
*/
public Builder clearAutoAuthKey() {
bitField0_ = (bitField0_ & ~0x00001000);
autoAuthKey_ = getDefaultInstance().getAutoAuthKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString syncKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @return Whether the syncKey field is set.
*/
@java.lang.Override
public boolean hasSyncKey() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @return The syncKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSyncKey() {
return syncKey_;
}
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @param value The syncKey to set.
* @return This builder for chaining.
*/
public Builder setSyncKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
syncKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"synckey"`
*
*
* optional bytes SyncKey = 14;
* @return This builder for chaining.
*/
public Builder clearSyncKey() {
bitField0_ = (bitField0_ & ~0x00002000);
syncKey_ = getDefaultInstance().getSyncKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString favSyncKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @return Whether the favSyncKey field is set.
*/
@java.lang.Override
public boolean hasFavSyncKey() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @return The favSyncKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFavSyncKey() {
return favSyncKey_;
}
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @param value The favSyncKey to set.
* @return This builder for chaining.
*/
public Builder setFavSyncKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
favSyncKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"favsynckey"`
*
*
* optional bytes FavSyncKey = 15;
* @return This builder for chaining.
*/
public Builder clearFavSyncKey() {
bitField0_ = (bitField0_ & ~0x00004000);
favSyncKey_ = getDefaultInstance().getFavSyncKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString snsSyncKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @return Whether the snsSyncKey field is set.
*/
@java.lang.Override
public boolean hasSnsSyncKey() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @return The snsSyncKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSnsSyncKey() {
return snsSyncKey_;
}
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @param value The snsSyncKey to set.
* @return This builder for chaining.
*/
public Builder setSnsSyncKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
snsSyncKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"snssynckey"`
*
*
* optional bytes SnsSyncKey = 16;
* @return This builder for chaining.
*/
public Builder clearSnsSyncKey() {
bitField0_ = (bitField0_ & ~0x00008000);
snsSyncKey_ = getDefaultInstance().getSnsSyncKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString hBAesKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @return Whether the hBAesKey field is set.
*/
@java.lang.Override
public boolean hasHBAesKey() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @return The hBAesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHBAesKey() {
return hBAesKey_;
}
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @param value The hBAesKey to set.
* @return This builder for chaining.
*/
public Builder setHBAesKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
hBAesKey_ = value;
onChanged();
return this;
}
/**
*
*`json:"hbaeskey"`
*
*
* optional bytes HBAesKey = 17;
* @return This builder for chaining.
*/
public Builder clearHBAesKey() {
bitField0_ = (bitField0_ & ~0x00010000);
hBAesKey_ = getDefaultInstance().getHBAesKey();
onChanged();
return this;
}
private java.lang.Object hBAesKeyEncrypted_ = "";
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return Whether the hBAesKeyEncrypted field is set.
*/
public boolean hasHBAesKeyEncrypted() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return The hBAesKeyEncrypted.
*/
public java.lang.String getHBAesKeyEncrypted() {
java.lang.Object ref = hBAesKeyEncrypted_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hBAesKeyEncrypted_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return The bytes for hBAesKeyEncrypted.
*/
public com.google.protobuf.ByteString
getHBAesKeyEncryptedBytes() {
java.lang.Object ref = hBAesKeyEncrypted_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hBAesKeyEncrypted_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @param value The hBAesKeyEncrypted to set.
* @return This builder for chaining.
*/
public Builder setHBAesKeyEncrypted(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
hBAesKeyEncrypted_ = value;
onChanged();
return this;
}
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @return This builder for chaining.
*/
public Builder clearHBAesKeyEncrypted() {
bitField0_ = (bitField0_ & ~0x00020000);
hBAesKeyEncrypted_ = getDefaultInstance().getHBAesKeyEncrypted();
onChanged();
return this;
}
/**
*
*`json:"hbesKeyencrypted"`
*
*
* optional string HBAesKeyEncrypted = 18;
* @param value The bytes for hBAesKeyEncrypted to set.
* @return This builder for chaining.
*/
public Builder setHBAesKeyEncryptedBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
hBAesKeyEncrypted_ = value;
onChanged();
return this;
}
private wechat.protobuf.Wechat.CDNDnsInfo dNSInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder> dNSInfoBuilder_;
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
* @return Whether the dNSInfo field is set.
*/
public boolean hasDNSInfo() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
* @return The dNSInfo.
*/
public wechat.protobuf.Wechat.CDNDnsInfo getDNSInfo() {
if (dNSInfoBuilder_ == null) {
return dNSInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : dNSInfo_;
} else {
return dNSInfoBuilder_.getMessage();
}
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
public Builder setDNSInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (dNSInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dNSInfo_ = value;
onChanged();
} else {
dNSInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00040000;
return this;
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
public Builder setDNSInfo(
wechat.protobuf.Wechat.CDNDnsInfo.Builder builderForValue) {
if (dNSInfoBuilder_ == null) {
dNSInfo_ = builderForValue.build();
onChanged();
} else {
dNSInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00040000;
return this;
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
public Builder mergeDNSInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (dNSInfoBuilder_ == null) {
if (((bitField0_ & 0x00040000) != 0) &&
dNSInfo_ != null &&
dNSInfo_ != wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance()) {
dNSInfo_ =
wechat.protobuf.Wechat.CDNDnsInfo.newBuilder(dNSInfo_).mergeFrom(value).buildPartial();
} else {
dNSInfo_ = value;
}
onChanged();
} else {
dNSInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00040000;
return this;
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
public Builder clearDNSInfo() {
if (dNSInfoBuilder_ == null) {
dNSInfo_ = null;
onChanged();
} else {
dNSInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00040000);
return this;
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
public wechat.protobuf.Wechat.CDNDnsInfo.Builder getDNSInfoBuilder() {
bitField0_ |= 0x00040000;
onChanged();
return getDNSInfoFieldBuilder().getBuilder();
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getDNSInfoOrBuilder() {
if (dNSInfoBuilder_ != null) {
return dNSInfoBuilder_.getMessageOrBuilder();
} else {
return dNSInfo_ == null ?
wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : dNSInfo_;
}
}
/**
*
* CDNDns
*
*
* optional .wechat_proto.CDNDnsInfo DNSInfo = 19;
*/
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>
getDNSInfoFieldBuilder() {
if (dNSInfoBuilder_ == null) {
dNSInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>(
getDNSInfo(),
getParentForChildren(),
isClean());
dNSInfo_ = null;
}
return dNSInfoBuilder_;
}
private wechat.protobuf.Wechat.CDNDnsInfo sNSDnsInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder> sNSDnsInfoBuilder_;
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
* @return Whether the sNSDnsInfo field is set.
*/
public boolean hasSNSDnsInfo() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
* @return The sNSDnsInfo.
*/
public wechat.protobuf.Wechat.CDNDnsInfo getSNSDnsInfo() {
if (sNSDnsInfoBuilder_ == null) {
return sNSDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : sNSDnsInfo_;
} else {
return sNSDnsInfoBuilder_.getMessage();
}
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
public Builder setSNSDnsInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (sNSDnsInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sNSDnsInfo_ = value;
onChanged();
} else {
sNSDnsInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00080000;
return this;
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
public Builder setSNSDnsInfo(
wechat.protobuf.Wechat.CDNDnsInfo.Builder builderForValue) {
if (sNSDnsInfoBuilder_ == null) {
sNSDnsInfo_ = builderForValue.build();
onChanged();
} else {
sNSDnsInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00080000;
return this;
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
public Builder mergeSNSDnsInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (sNSDnsInfoBuilder_ == null) {
if (((bitField0_ & 0x00080000) != 0) &&
sNSDnsInfo_ != null &&
sNSDnsInfo_ != wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance()) {
sNSDnsInfo_ =
wechat.protobuf.Wechat.CDNDnsInfo.newBuilder(sNSDnsInfo_).mergeFrom(value).buildPartial();
} else {
sNSDnsInfo_ = value;
}
onChanged();
} else {
sNSDnsInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00080000;
return this;
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
public Builder clearSNSDnsInfo() {
if (sNSDnsInfoBuilder_ == null) {
sNSDnsInfo_ = null;
onChanged();
} else {
sNSDnsInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00080000);
return this;
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
public wechat.protobuf.Wechat.CDNDnsInfo.Builder getSNSDnsInfoBuilder() {
bitField0_ |= 0x00080000;
onChanged();
return getSNSDnsInfoFieldBuilder().getBuilder();
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getSNSDnsInfoOrBuilder() {
if (sNSDnsInfoBuilder_ != null) {
return sNSDnsInfoBuilder_.getMessageOrBuilder();
} else {
return sNSDnsInfo_ == null ?
wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : sNSDnsInfo_;
}
}
/**
*
*`json:"snsdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo SNSDnsInfo = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>
getSNSDnsInfoFieldBuilder() {
if (sNSDnsInfoBuilder_ == null) {
sNSDnsInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>(
getSNSDnsInfo(),
getParentForChildren(),
isClean());
sNSDnsInfo_ = null;
}
return sNSDnsInfoBuilder_;
}
private wechat.protobuf.Wechat.CDNDnsInfo aPPDnsInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder> aPPDnsInfoBuilder_;
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
* @return Whether the aPPDnsInfo field is set.
*/
public boolean hasAPPDnsInfo() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
* @return The aPPDnsInfo.
*/
public wechat.protobuf.Wechat.CDNDnsInfo getAPPDnsInfo() {
if (aPPDnsInfoBuilder_ == null) {
return aPPDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : aPPDnsInfo_;
} else {
return aPPDnsInfoBuilder_.getMessage();
}
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
public Builder setAPPDnsInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (aPPDnsInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aPPDnsInfo_ = value;
onChanged();
} else {
aPPDnsInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00100000;
return this;
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
public Builder setAPPDnsInfo(
wechat.protobuf.Wechat.CDNDnsInfo.Builder builderForValue) {
if (aPPDnsInfoBuilder_ == null) {
aPPDnsInfo_ = builderForValue.build();
onChanged();
} else {
aPPDnsInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00100000;
return this;
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
public Builder mergeAPPDnsInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (aPPDnsInfoBuilder_ == null) {
if (((bitField0_ & 0x00100000) != 0) &&
aPPDnsInfo_ != null &&
aPPDnsInfo_ != wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance()) {
aPPDnsInfo_ =
wechat.protobuf.Wechat.CDNDnsInfo.newBuilder(aPPDnsInfo_).mergeFrom(value).buildPartial();
} else {
aPPDnsInfo_ = value;
}
onChanged();
} else {
aPPDnsInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00100000;
return this;
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
public Builder clearAPPDnsInfo() {
if (aPPDnsInfoBuilder_ == null) {
aPPDnsInfo_ = null;
onChanged();
} else {
aPPDnsInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00100000);
return this;
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
public wechat.protobuf.Wechat.CDNDnsInfo.Builder getAPPDnsInfoBuilder() {
bitField0_ |= 0x00100000;
onChanged();
return getAPPDnsInfoFieldBuilder().getBuilder();
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getAPPDnsInfoOrBuilder() {
if (aPPDnsInfoBuilder_ != null) {
return aPPDnsInfoBuilder_.getMessageOrBuilder();
} else {
return aPPDnsInfo_ == null ?
wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : aPPDnsInfo_;
}
}
/**
*
*`json:"appdnsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo APPDnsInfo = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>
getAPPDnsInfoFieldBuilder() {
if (aPPDnsInfoBuilder_ == null) {
aPPDnsInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>(
getAPPDnsInfo(),
getParentForChildren(),
isClean());
aPPDnsInfo_ = null;
}
return aPPDnsInfoBuilder_;
}
private wechat.protobuf.Wechat.CDNDnsInfo fAKEDnsInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder> fAKEDnsInfoBuilder_;
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
* @return Whether the fAKEDnsInfo field is set.
*/
public boolean hasFAKEDnsInfo() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
* @return The fAKEDnsInfo.
*/
public wechat.protobuf.Wechat.CDNDnsInfo getFAKEDnsInfo() {
if (fAKEDnsInfoBuilder_ == null) {
return fAKEDnsInfo_ == null ? wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : fAKEDnsInfo_;
} else {
return fAKEDnsInfoBuilder_.getMessage();
}
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
public Builder setFAKEDnsInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (fAKEDnsInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
fAKEDnsInfo_ = value;
onChanged();
} else {
fAKEDnsInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00200000;
return this;
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
public Builder setFAKEDnsInfo(
wechat.protobuf.Wechat.CDNDnsInfo.Builder builderForValue) {
if (fAKEDnsInfoBuilder_ == null) {
fAKEDnsInfo_ = builderForValue.build();
onChanged();
} else {
fAKEDnsInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00200000;
return this;
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
public Builder mergeFAKEDnsInfo(wechat.protobuf.Wechat.CDNDnsInfo value) {
if (fAKEDnsInfoBuilder_ == null) {
if (((bitField0_ & 0x00200000) != 0) &&
fAKEDnsInfo_ != null &&
fAKEDnsInfo_ != wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance()) {
fAKEDnsInfo_ =
wechat.protobuf.Wechat.CDNDnsInfo.newBuilder(fAKEDnsInfo_).mergeFrom(value).buildPartial();
} else {
fAKEDnsInfo_ = value;
}
onChanged();
} else {
fAKEDnsInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00200000;
return this;
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
public Builder clearFAKEDnsInfo() {
if (fAKEDnsInfoBuilder_ == null) {
fAKEDnsInfo_ = null;
onChanged();
} else {
fAKEDnsInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00200000);
return this;
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
public wechat.protobuf.Wechat.CDNDnsInfo.Builder getFAKEDnsInfoBuilder() {
bitField0_ |= 0x00200000;
onChanged();
return getFAKEDnsInfoFieldBuilder().getBuilder();
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
public wechat.protobuf.Wechat.CDNDnsInfoOrBuilder getFAKEDnsInfoOrBuilder() {
if (fAKEDnsInfoBuilder_ != null) {
return fAKEDnsInfoBuilder_.getMessageOrBuilder();
} else {
return fAKEDnsInfo_ == null ?
wechat.protobuf.Wechat.CDNDnsInfo.getDefaultInstance() : fAKEDnsInfo_;
}
}
/**
*
*`json:"fakednsinfo"`
*
*
* optional .wechat_proto.CDNDnsInfo FAKEDnsInfo = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>
getFAKEDnsInfoFieldBuilder() {
if (fAKEDnsInfoBuilder_ == null) {
fAKEDnsInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.Wechat.CDNDnsInfo, wechat.protobuf.Wechat.CDNDnsInfo.Builder, wechat.protobuf.Wechat.CDNDnsInfoOrBuilder>(
getFAKEDnsInfo(),
getParentForChildren(),
isClean());
fAKEDnsInfo_ = null;
}
return fAKEDnsInfoBuilder_;
}
private rock.protobuf.Rock.DeviceInfo deviceInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.DeviceInfo, rock.protobuf.Rock.DeviceInfo.Builder, rock.protobuf.Rock.DeviceInfoOrBuilder> deviceInfoBuilder_;
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
* @return Whether the deviceInfo field is set.
*/
public boolean hasDeviceInfo() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
* @return The deviceInfo.
*/
public rock.protobuf.Rock.DeviceInfo getDeviceInfo() {
if (deviceInfoBuilder_ == null) {
return deviceInfo_ == null ? rock.protobuf.Rock.DeviceInfo.getDefaultInstance() : deviceInfo_;
} else {
return deviceInfoBuilder_.getMessage();
}
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
public Builder setDeviceInfo(rock.protobuf.Rock.DeviceInfo value) {
if (deviceInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deviceInfo_ = value;
onChanged();
} else {
deviceInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00400000;
return this;
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
public Builder setDeviceInfo(
rock.protobuf.Rock.DeviceInfo.Builder builderForValue) {
if (deviceInfoBuilder_ == null) {
deviceInfo_ = builderForValue.build();
onChanged();
} else {
deviceInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00400000;
return this;
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
public Builder mergeDeviceInfo(rock.protobuf.Rock.DeviceInfo value) {
if (deviceInfoBuilder_ == null) {
if (((bitField0_ & 0x00400000) != 0) &&
deviceInfo_ != null &&
deviceInfo_ != rock.protobuf.Rock.DeviceInfo.getDefaultInstance()) {
deviceInfo_ =
rock.protobuf.Rock.DeviceInfo.newBuilder(deviceInfo_).mergeFrom(value).buildPartial();
} else {
deviceInfo_ = value;
}
onChanged();
} else {
deviceInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00400000;
return this;
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
public Builder clearDeviceInfo() {
if (deviceInfoBuilder_ == null) {
deviceInfo_ = null;
onChanged();
} else {
deviceInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00400000);
return this;
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
public rock.protobuf.Rock.DeviceInfo.Builder getDeviceInfoBuilder() {
bitField0_ |= 0x00400000;
onChanged();
return getDeviceInfoFieldBuilder().getBuilder();
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
public rock.protobuf.Rock.DeviceInfoOrBuilder getDeviceInfoOrBuilder() {
if (deviceInfoBuilder_ != null) {
return deviceInfoBuilder_.getMessageOrBuilder();
} else {
return deviceInfo_ == null ?
rock.protobuf.Rock.DeviceInfo.getDefaultInstance() : deviceInfo_;
}
}
/**
*
* 设备信息
*
*
* optional .rock.DeviceInfo DeviceInfo = 23;
*/
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.DeviceInfo, rock.protobuf.Rock.DeviceInfo.Builder, rock.protobuf.Rock.DeviceInfoOrBuilder>
getDeviceInfoFieldBuilder() {
if (deviceInfoBuilder_ == null) {
deviceInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.DeviceInfo, rock.protobuf.Rock.DeviceInfo.Builder, rock.protobuf.Rock.DeviceInfoOrBuilder>(
getDeviceInfo(),
getParentForChildren(),
isClean());
deviceInfo_ = null;
}
return deviceInfoBuilder_;
}
private int balanceVersion_ ;
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @return Whether the balanceVersion field is set.
*/
@java.lang.Override
public boolean hasBalanceVersion() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @return The balanceVersion.
*/
@java.lang.Override
public int getBalanceVersion() {
return balanceVersion_;
}
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @param value The balanceVersion to set.
* @return This builder for chaining.
*/
public Builder setBalanceVersion(int value) {
bitField0_ |= 0x00800000;
balanceVersion_ = value;
onChanged();
return this;
}
/**
*
* uint32
*
*
* optional uint32 BalanceVersion = 24;
* @return This builder for chaining.
*/
public Builder clearBalanceVersion() {
bitField0_ = (bitField0_ & ~0x00800000);
balanceVersion_ = 0;
onChanged();
return this;
}
private rock.protobuf.Rock.WifiInfo wifiInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.WifiInfo, rock.protobuf.Rock.WifiInfo.Builder, rock.protobuf.Rock.WifiInfoOrBuilder> wifiInfoBuilder_;
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
* @return Whether the wifiInfo field is set.
*/
public boolean hasWifiInfo() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
* @return The wifiInfo.
*/
public rock.protobuf.Rock.WifiInfo getWifiInfo() {
if (wifiInfoBuilder_ == null) {
return wifiInfo_ == null ? rock.protobuf.Rock.WifiInfo.getDefaultInstance() : wifiInfo_;
} else {
return wifiInfoBuilder_.getMessage();
}
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
public Builder setWifiInfo(rock.protobuf.Rock.WifiInfo value) {
if (wifiInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
wifiInfo_ = value;
onChanged();
} else {
wifiInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x01000000;
return this;
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
public Builder setWifiInfo(
rock.protobuf.Rock.WifiInfo.Builder builderForValue) {
if (wifiInfoBuilder_ == null) {
wifiInfo_ = builderForValue.build();
onChanged();
} else {
wifiInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x01000000;
return this;
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
public Builder mergeWifiInfo(rock.protobuf.Rock.WifiInfo value) {
if (wifiInfoBuilder_ == null) {
if (((bitField0_ & 0x01000000) != 0) &&
wifiInfo_ != null &&
wifiInfo_ != rock.protobuf.Rock.WifiInfo.getDefaultInstance()) {
wifiInfo_ =
rock.protobuf.Rock.WifiInfo.newBuilder(wifiInfo_).mergeFrom(value).buildPartial();
} else {
wifiInfo_ = value;
}
onChanged();
} else {
wifiInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x01000000;
return this;
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
public Builder clearWifiInfo() {
if (wifiInfoBuilder_ == null) {
wifiInfo_ = null;
onChanged();
} else {
wifiInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x01000000);
return this;
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
public rock.protobuf.Rock.WifiInfo.Builder getWifiInfoBuilder() {
bitField0_ |= 0x01000000;
onChanged();
return getWifiInfoFieldBuilder().getBuilder();
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
public rock.protobuf.Rock.WifiInfoOrBuilder getWifiInfoOrBuilder() {
if (wifiInfoBuilder_ != null) {
return wifiInfoBuilder_.getMessageOrBuilder();
} else {
return wifiInfo_ == null ?
rock.protobuf.Rock.WifiInfo.getDefaultInstance() : wifiInfo_;
}
}
/**
*
* Wifi信息
*
*
* optional .rock.WifiInfo WifiInfo = 25;
*/
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.WifiInfo, rock.protobuf.Rock.WifiInfo.Builder, rock.protobuf.Rock.WifiInfoOrBuilder>
getWifiInfoFieldBuilder() {
if (wifiInfoBuilder_ == null) {
wifiInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.WifiInfo, rock.protobuf.Rock.WifiInfo.Builder, rock.protobuf.Rock.WifiInfoOrBuilder>(
getWifiInfo(),
getParentForChildren(),
isClean());
wifiInfo_ = null;
}
return wifiInfoBuilder_;
}
private rock.protobuf.Rock.MMInfo mMInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.MMInfo, rock.protobuf.Rock.MMInfo.Builder, rock.protobuf.Rock.MMInfoOrBuilder> mMInfoBuilder_;
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
* @return Whether the mMInfo field is set.
*/
public boolean hasMMInfo() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
* @return The mMInfo.
*/
public rock.protobuf.Rock.MMInfo getMMInfo() {
if (mMInfoBuilder_ == null) {
return mMInfo_ == null ? rock.protobuf.Rock.MMInfo.getDefaultInstance() : mMInfo_;
} else {
return mMInfoBuilder_.getMessage();
}
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
public Builder setMMInfo(rock.protobuf.Rock.MMInfo value) {
if (mMInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mMInfo_ = value;
onChanged();
} else {
mMInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x02000000;
return this;
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
public Builder setMMInfo(
rock.protobuf.Rock.MMInfo.Builder builderForValue) {
if (mMInfoBuilder_ == null) {
mMInfo_ = builderForValue.build();
onChanged();
} else {
mMInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x02000000;
return this;
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
public Builder mergeMMInfo(rock.protobuf.Rock.MMInfo value) {
if (mMInfoBuilder_ == null) {
if (((bitField0_ & 0x02000000) != 0) &&
mMInfo_ != null &&
mMInfo_ != rock.protobuf.Rock.MMInfo.getDefaultInstance()) {
mMInfo_ =
rock.protobuf.Rock.MMInfo.newBuilder(mMInfo_).mergeFrom(value).buildPartial();
} else {
mMInfo_ = value;
}
onChanged();
} else {
mMInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x02000000;
return this;
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
public Builder clearMMInfo() {
if (mMInfoBuilder_ == null) {
mMInfo_ = null;
onChanged();
} else {
mMInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x02000000);
return this;
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
public rock.protobuf.Rock.MMInfo.Builder getMMInfoBuilder() {
bitField0_ |= 0x02000000;
onChanged();
return getMMInfoFieldBuilder().getBuilder();
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
public rock.protobuf.Rock.MMInfoOrBuilder getMMInfoOrBuilder() {
if (mMInfoBuilder_ != null) {
return mMInfoBuilder_.getMessageOrBuilder();
} else {
return mMInfo_ == null ?
rock.protobuf.Rock.MMInfo.getDefaultInstance() : mMInfo_;
}
}
/**
*
* MMTLS信息
*
*
* optional .rock.MMInfo MMInfo = 26;
*/
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.MMInfo, rock.protobuf.Rock.MMInfo.Builder, rock.protobuf.Rock.MMInfoOrBuilder>
getMMInfoFieldBuilder() {
if (mMInfoBuilder_ == null) {
mMInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.MMInfo, rock.protobuf.Rock.MMInfo.Builder, rock.protobuf.Rock.MMInfoOrBuilder>(
getMMInfo(),
getParentForChildren(),
isClean());
mMInfo_ = null;
}
return mMInfoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.UserInfo)
}
// @@protoc_insertion_point(class_scope:rock.UserInfo)
private static final rock.protobuf.Rock.UserInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.UserInfo();
}
public static rock.protobuf.Rock.UserInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UserInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.UserInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WifiInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.WifiInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional string Name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string Name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* optional string Name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string WifiBssID = 2;
* @return Whether the wifiBssID field is set.
*/
boolean hasWifiBssID();
/**
* optional string WifiBssID = 2;
* @return The wifiBssID.
*/
java.lang.String getWifiBssID();
/**
* optional string WifiBssID = 2;
* @return The bytes for wifiBssID.
*/
com.google.protobuf.ByteString
getWifiBssIDBytes();
}
/**
*
* WifiInfo WifiInfo
*
*
* Protobuf type {@code rock.WifiInfo}
*/
public static final class WifiInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.WifiInfo)
WifiInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use WifiInfo.newBuilder() to construct.
private WifiInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WifiInfo() {
name_ = "";
wifiBssID_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WifiInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WifiInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
wifiBssID_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_WifiInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_WifiInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.WifiInfo.class, rock.protobuf.Rock.WifiInfo.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string Name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string Name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string Name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WIFIBSSID_FIELD_NUMBER = 2;
private volatile java.lang.Object wifiBssID_;
/**
* optional string WifiBssID = 2;
* @return Whether the wifiBssID field is set.
*/
@java.lang.Override
public boolean hasWifiBssID() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string WifiBssID = 2;
* @return The wifiBssID.
*/
@java.lang.Override
public java.lang.String getWifiBssID() {
java.lang.Object ref = wifiBssID_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
wifiBssID_ = s;
}
return s;
}
}
/**
* optional string WifiBssID = 2;
* @return The bytes for wifiBssID.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWifiBssIDBytes() {
java.lang.Object ref = wifiBssID_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
wifiBssID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, wifiBssID_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, wifiBssID_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.WifiInfo)) {
return super.equals(obj);
}
rock.protobuf.Rock.WifiInfo other = (rock.protobuf.Rock.WifiInfo) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasWifiBssID() != other.hasWifiBssID()) return false;
if (hasWifiBssID()) {
if (!getWifiBssID()
.equals(other.getWifiBssID())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasWifiBssID()) {
hash = (37 * hash) + WIFIBSSID_FIELD_NUMBER;
hash = (53 * hash) + getWifiBssID().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.WifiInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.WifiInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.WifiInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.WifiInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* WifiInfo WifiInfo
*
*
* Protobuf type {@code rock.WifiInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.WifiInfo)
rock.protobuf.Rock.WifiInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_WifiInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_WifiInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.WifiInfo.class, rock.protobuf.Rock.WifiInfo.Builder.class);
}
// Construct using rock.protobuf.Rock.WifiInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
wifiBssID_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_WifiInfo_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.WifiInfo getDefaultInstanceForType() {
return rock.protobuf.Rock.WifiInfo.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.WifiInfo build() {
rock.protobuf.Rock.WifiInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.WifiInfo buildPartial() {
rock.protobuf.Rock.WifiInfo result = new rock.protobuf.Rock.WifiInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.wifiBssID_ = wifiBssID_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.WifiInfo) {
return mergeFrom((rock.protobuf.Rock.WifiInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.WifiInfo other) {
if (other == rock.protobuf.Rock.WifiInfo.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasWifiBssID()) {
bitField0_ |= 0x00000002;
wifiBssID_ = other.wifiBssID_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.WifiInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.WifiInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* optional string Name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string Name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string Name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string Name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string Name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string Name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private java.lang.Object wifiBssID_ = "";
/**
* optional string WifiBssID = 2;
* @return Whether the wifiBssID field is set.
*/
public boolean hasWifiBssID() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string WifiBssID = 2;
* @return The wifiBssID.
*/
public java.lang.String getWifiBssID() {
java.lang.Object ref = wifiBssID_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
wifiBssID_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string WifiBssID = 2;
* @return The bytes for wifiBssID.
*/
public com.google.protobuf.ByteString
getWifiBssIDBytes() {
java.lang.Object ref = wifiBssID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
wifiBssID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string WifiBssID = 2;
* @param value The wifiBssID to set.
* @return This builder for chaining.
*/
public Builder setWifiBssID(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
wifiBssID_ = value;
onChanged();
return this;
}
/**
* optional string WifiBssID = 2;
* @return This builder for chaining.
*/
public Builder clearWifiBssID() {
bitField0_ = (bitField0_ & ~0x00000002);
wifiBssID_ = getDefaultInstance().getWifiBssID();
onChanged();
return this;
}
/**
* optional string WifiBssID = 2;
* @param value The bytes for wifiBssID to set.
* @return This builder for chaining.
*/
public Builder setWifiBssIDBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
wifiBssID_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.WifiInfo)
}
// @@protoc_insertion_point(class_scope:rock.WifiInfo)
private static final rock.protobuf.Rock.WifiInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.WifiInfo();
}
public static rock.protobuf.Rock.WifiInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WifiInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WifiInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.WifiInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MMInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.MMInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return Whether the shortHost field is set.
*/
boolean hasShortHost();
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return The shortHost.
*/
java.lang.String getShortHost();
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return The bytes for shortHost.
*/
com.google.protobuf.ByteString
getShortHostBytes();
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return Whether the shortURL field is set.
*/
boolean hasShortURL();
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return The shortURL.
*/
java.lang.String getShortURL();
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return The bytes for shortURL.
*/
com.google.protobuf.ByteString
getShortURLBytes();
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
java.util.List
getShortPskList();
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
rock.protobuf.Rock.Psk getShortPsk(int index);
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
int getShortPskCount();
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
java.util.List extends rock.protobuf.Rock.PskOrBuilder>
getShortPskOrBuilderList();
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
rock.protobuf.Rock.PskOrBuilder getShortPskOrBuilder(
int index);
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @return Whether the pskAccessKey field is set.
*/
boolean hasPskAccessKey();
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @return The pskAccessKey.
*/
com.google.protobuf.ByteString getPskAccessKey();
/**
* optional string LongHost = 5;
* @return Whether the longHost field is set.
*/
boolean hasLongHost();
/**
* optional string LongHost = 5;
* @return The longHost.
*/
java.lang.String getLongHost();
/**
* optional string LongHost = 5;
* @return The bytes for longHost.
*/
com.google.protobuf.ByteString
getLongHostBytes();
/**
* optional int32 LongPort = 6;
* @return Whether the longPort field is set.
*/
boolean hasLongPort();
/**
* optional int32 LongPort = 6;
* @return The longPort.
*/
int getLongPort();
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
* @return Whether the hkdfKey field is set.
*/
boolean hasHkdfKey();
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
* @return The hkdfKey.
*/
rock.protobuf.Rock.HkdfKey56 getHkdfKey();
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
rock.protobuf.Rock.HkdfKey56OrBuilder getHkdfKeyOrBuilder();
}
/**
*
* MMInfo MMInfo
*
*
* Protobuf type {@code rock.MMInfo}
*/
public static final class MMInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.MMInfo)
MMInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use MMInfo.newBuilder() to construct.
private MMInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MMInfo() {
shortHost_ = "";
shortURL_ = "";
shortPsk_ = java.util.Collections.emptyList();
pskAccessKey_ = com.google.protobuf.ByteString.EMPTY;
longHost_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MMInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MMInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
shortHost_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
shortURL_ = bs;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
shortPsk_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
shortPsk_.add(
input.readMessage(rock.protobuf.Rock.Psk.PARSER, extensionRegistry));
break;
}
case 34: {
bitField0_ |= 0x00000004;
pskAccessKey_ = input.readBytes();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
longHost_ = bs;
break;
}
case 48: {
bitField0_ |= 0x00000010;
longPort_ = input.readInt32();
break;
}
case 58: {
rock.protobuf.Rock.HkdfKey56.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) != 0)) {
subBuilder = hkdfKey_.toBuilder();
}
hkdfKey_ = input.readMessage(rock.protobuf.Rock.HkdfKey56.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(hkdfKey_);
hkdfKey_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
shortPsk_ = java.util.Collections.unmodifiableList(shortPsk_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_MMInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_MMInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.MMInfo.class, rock.protobuf.Rock.MMInfo.Builder.class);
}
private int bitField0_;
public static final int SHORTHOST_FIELD_NUMBER = 1;
private volatile java.lang.Object shortHost_;
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return Whether the shortHost field is set.
*/
@java.lang.Override
public boolean hasShortHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return The shortHost.
*/
@java.lang.Override
public java.lang.String getShortHost() {
java.lang.Object ref = shortHost_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortHost_ = s;
}
return s;
}
}
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return The bytes for shortHost.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getShortHostBytes() {
java.lang.Object ref = shortHost_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHORTURL_FIELD_NUMBER = 2;
private volatile java.lang.Object shortURL_;
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return Whether the shortURL field is set.
*/
@java.lang.Override
public boolean hasShortURL() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return The shortURL.
*/
@java.lang.Override
public java.lang.String getShortURL() {
java.lang.Object ref = shortURL_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortURL_ = s;
}
return s;
}
}
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return The bytes for shortURL.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getShortURLBytes() {
java.lang.Object ref = shortURL_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortURL_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHORTPSK_FIELD_NUMBER = 3;
private java.util.List shortPsk_;
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
@java.lang.Override
public java.util.List getShortPskList() {
return shortPsk_;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
@java.lang.Override
public java.util.List extends rock.protobuf.Rock.PskOrBuilder>
getShortPskOrBuilderList() {
return shortPsk_;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
@java.lang.Override
public int getShortPskCount() {
return shortPsk_.size();
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
@java.lang.Override
public rock.protobuf.Rock.Psk getShortPsk(int index) {
return shortPsk_.get(index);
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
@java.lang.Override
public rock.protobuf.Rock.PskOrBuilder getShortPskOrBuilder(
int index) {
return shortPsk_.get(index);
}
public static final int PSKACCESSKEY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString pskAccessKey_;
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @return Whether the pskAccessKey field is set.
*/
@java.lang.Override
public boolean hasPskAccessKey() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @return The pskAccessKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPskAccessKey() {
return pskAccessKey_;
}
public static final int LONGHOST_FIELD_NUMBER = 5;
private volatile java.lang.Object longHost_;
/**
* optional string LongHost = 5;
* @return Whether the longHost field is set.
*/
@java.lang.Override
public boolean hasLongHost() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string LongHost = 5;
* @return The longHost.
*/
@java.lang.Override
public java.lang.String getLongHost() {
java.lang.Object ref = longHost_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
longHost_ = s;
}
return s;
}
}
/**
* optional string LongHost = 5;
* @return The bytes for longHost.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLongHostBytes() {
java.lang.Object ref = longHost_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LONGPORT_FIELD_NUMBER = 6;
private int longPort_;
/**
* optional int32 LongPort = 6;
* @return Whether the longPort field is set.
*/
@java.lang.Override
public boolean hasLongPort() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional int32 LongPort = 6;
* @return The longPort.
*/
@java.lang.Override
public int getLongPort() {
return longPort_;
}
public static final int HKDFKEY_FIELD_NUMBER = 7;
private rock.protobuf.Rock.HkdfKey56 hkdfKey_;
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
* @return Whether the hkdfKey field is set.
*/
@java.lang.Override
public boolean hasHkdfKey() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
* @return The hkdfKey.
*/
@java.lang.Override
public rock.protobuf.Rock.HkdfKey56 getHkdfKey() {
return hkdfKey_ == null ? rock.protobuf.Rock.HkdfKey56.getDefaultInstance() : hkdfKey_;
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
@java.lang.Override
public rock.protobuf.Rock.HkdfKey56OrBuilder getHkdfKeyOrBuilder() {
return hkdfKey_ == null ? rock.protobuf.Rock.HkdfKey56.getDefaultInstance() : hkdfKey_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, shortHost_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, shortURL_);
}
for (int i = 0; i < shortPsk_.size(); i++) {
output.writeMessage(3, shortPsk_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(4, pskAccessKey_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, longHost_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(6, longPort_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(7, getHkdfKey());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, shortHost_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, shortURL_);
}
for (int i = 0; i < shortPsk_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, shortPsk_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, pskAccessKey_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, longHost_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, longPort_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getHkdfKey());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.MMInfo)) {
return super.equals(obj);
}
rock.protobuf.Rock.MMInfo other = (rock.protobuf.Rock.MMInfo) obj;
if (hasShortHost() != other.hasShortHost()) return false;
if (hasShortHost()) {
if (!getShortHost()
.equals(other.getShortHost())) return false;
}
if (hasShortURL() != other.hasShortURL()) return false;
if (hasShortURL()) {
if (!getShortURL()
.equals(other.getShortURL())) return false;
}
if (!getShortPskList()
.equals(other.getShortPskList())) return false;
if (hasPskAccessKey() != other.hasPskAccessKey()) return false;
if (hasPskAccessKey()) {
if (!getPskAccessKey()
.equals(other.getPskAccessKey())) return false;
}
if (hasLongHost() != other.hasLongHost()) return false;
if (hasLongHost()) {
if (!getLongHost()
.equals(other.getLongHost())) return false;
}
if (hasLongPort() != other.hasLongPort()) return false;
if (hasLongPort()) {
if (getLongPort()
!= other.getLongPort()) return false;
}
if (hasHkdfKey() != other.hasHkdfKey()) return false;
if (hasHkdfKey()) {
if (!getHkdfKey()
.equals(other.getHkdfKey())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasShortHost()) {
hash = (37 * hash) + SHORTHOST_FIELD_NUMBER;
hash = (53 * hash) + getShortHost().hashCode();
}
if (hasShortURL()) {
hash = (37 * hash) + SHORTURL_FIELD_NUMBER;
hash = (53 * hash) + getShortURL().hashCode();
}
if (getShortPskCount() > 0) {
hash = (37 * hash) + SHORTPSK_FIELD_NUMBER;
hash = (53 * hash) + getShortPskList().hashCode();
}
if (hasPskAccessKey()) {
hash = (37 * hash) + PSKACCESSKEY_FIELD_NUMBER;
hash = (53 * hash) + getPskAccessKey().hashCode();
}
if (hasLongHost()) {
hash = (37 * hash) + LONGHOST_FIELD_NUMBER;
hash = (53 * hash) + getLongHost().hashCode();
}
if (hasLongPort()) {
hash = (37 * hash) + LONGPORT_FIELD_NUMBER;
hash = (53 * hash) + getLongPort();
}
if (hasHkdfKey()) {
hash = (37 * hash) + HKDFKEY_FIELD_NUMBER;
hash = (53 * hash) + getHkdfKey().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.MMInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.MMInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.MMInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.MMInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.MMInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.MMInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.MMInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.MMInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.MMInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.MMInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.MMInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.MMInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.MMInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MMInfo MMInfo
*
*
* Protobuf type {@code rock.MMInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.MMInfo)
rock.protobuf.Rock.MMInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_MMInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_MMInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.MMInfo.class, rock.protobuf.Rock.MMInfo.Builder.class);
}
// Construct using rock.protobuf.Rock.MMInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getShortPskFieldBuilder();
getHkdfKeyFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
shortHost_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
shortURL_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
if (shortPskBuilder_ == null) {
shortPsk_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
shortPskBuilder_.clear();
}
pskAccessKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
longHost_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
longPort_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
if (hkdfKeyBuilder_ == null) {
hkdfKey_ = null;
} else {
hkdfKeyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_MMInfo_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.MMInfo getDefaultInstanceForType() {
return rock.protobuf.Rock.MMInfo.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.MMInfo build() {
rock.protobuf.Rock.MMInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.MMInfo buildPartial() {
rock.protobuf.Rock.MMInfo result = new rock.protobuf.Rock.MMInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.shortHost_ = shortHost_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.shortURL_ = shortURL_;
if (shortPskBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
shortPsk_ = java.util.Collections.unmodifiableList(shortPsk_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.shortPsk_ = shortPsk_;
} else {
result.shortPsk_ = shortPskBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.pskAccessKey_ = pskAccessKey_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.longHost_ = longHost_;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.longPort_ = longPort_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
if (hkdfKeyBuilder_ == null) {
result.hkdfKey_ = hkdfKey_;
} else {
result.hkdfKey_ = hkdfKeyBuilder_.build();
}
to_bitField0_ |= 0x00000020;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.MMInfo) {
return mergeFrom((rock.protobuf.Rock.MMInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.MMInfo other) {
if (other == rock.protobuf.Rock.MMInfo.getDefaultInstance()) return this;
if (other.hasShortHost()) {
bitField0_ |= 0x00000001;
shortHost_ = other.shortHost_;
onChanged();
}
if (other.hasShortURL()) {
bitField0_ |= 0x00000002;
shortURL_ = other.shortURL_;
onChanged();
}
if (shortPskBuilder_ == null) {
if (!other.shortPsk_.isEmpty()) {
if (shortPsk_.isEmpty()) {
shortPsk_ = other.shortPsk_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureShortPskIsMutable();
shortPsk_.addAll(other.shortPsk_);
}
onChanged();
}
} else {
if (!other.shortPsk_.isEmpty()) {
if (shortPskBuilder_.isEmpty()) {
shortPskBuilder_.dispose();
shortPskBuilder_ = null;
shortPsk_ = other.shortPsk_;
bitField0_ = (bitField0_ & ~0x00000004);
shortPskBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getShortPskFieldBuilder() : null;
} else {
shortPskBuilder_.addAllMessages(other.shortPsk_);
}
}
}
if (other.hasPskAccessKey()) {
setPskAccessKey(other.getPskAccessKey());
}
if (other.hasLongHost()) {
bitField0_ |= 0x00000010;
longHost_ = other.longHost_;
onChanged();
}
if (other.hasLongPort()) {
setLongPort(other.getLongPort());
}
if (other.hasHkdfKey()) {
mergeHkdfKey(other.getHkdfKey());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.MMInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.MMInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object shortHost_ = "";
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return Whether the shortHost field is set.
*/
public boolean hasShortHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return The shortHost.
*/
public java.lang.String getShortHost() {
java.lang.Object ref = shortHost_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortHost_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return The bytes for shortHost.
*/
public com.google.protobuf.ByteString
getShortHostBytes() {
java.lang.Object ref = shortHost_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @param value The shortHost to set.
* @return This builder for chaining.
*/
public Builder setShortHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
shortHost_ = value;
onChanged();
return this;
}
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @return This builder for chaining.
*/
public Builder clearShortHost() {
bitField0_ = (bitField0_ & ~0x00000001);
shortHost_ = getDefaultInstance().getShortHost();
onChanged();
return this;
}
/**
*
* 短链接 属性
* mmtls 协议host 例如:hkextshort.weixin.qq.com,这个需要保存这数据库
*
*
* optional string ShortHost = 1;
* @param value The bytes for shortHost to set.
* @return This builder for chaining.
*/
public Builder setShortHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
shortHost_ = value;
onChanged();
return this;
}
private java.lang.Object shortURL_ = "";
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return Whether the shortURL field is set.
*/
public boolean hasShortURL() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return The shortURL.
*/
public java.lang.String getShortURL() {
java.lang.Object ref = shortURL_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortURL_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return The bytes for shortURL.
*/
public com.google.protobuf.ByteString
getShortURLBytes() {
java.lang.Object ref = shortURL_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortURL_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @param value The shortURL to set.
* @return This builder for chaining.
*/
public Builder setShortURL(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
shortURL_ = value;
onChanged();
return this;
}
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @return This builder for chaining.
*/
public Builder clearShortURL() {
bitField0_ = (bitField0_ & ~0x00000002);
shortURL_ = getDefaultInstance().getShortURL();
onChanged();
return this;
}
/**
*
* mmtls路径 -- 例如:/mmtls/12345678(随机8位16进制字符串),每次握手都随机一个
*
*
* optional string ShortURL = 2;
* @param value The bytes for shortURL to set.
* @return This builder for chaining.
*/
public Builder setShortURLBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
shortURL_ = value;
onChanged();
return this;
}
private java.util.List shortPsk_ =
java.util.Collections.emptyList();
private void ensureShortPskIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
shortPsk_ = new java.util.ArrayList(shortPsk_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
rock.protobuf.Rock.Psk, rock.protobuf.Rock.Psk.Builder, rock.protobuf.Rock.PskOrBuilder> shortPskBuilder_;
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public java.util.List getShortPskList() {
if (shortPskBuilder_ == null) {
return java.util.Collections.unmodifiableList(shortPsk_);
} else {
return shortPskBuilder_.getMessageList();
}
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public int getShortPskCount() {
if (shortPskBuilder_ == null) {
return shortPsk_.size();
} else {
return shortPskBuilder_.getCount();
}
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public rock.protobuf.Rock.Psk getShortPsk(int index) {
if (shortPskBuilder_ == null) {
return shortPsk_.get(index);
} else {
return shortPskBuilder_.getMessage(index);
}
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder setShortPsk(
int index, rock.protobuf.Rock.Psk value) {
if (shortPskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShortPskIsMutable();
shortPsk_.set(index, value);
onChanged();
} else {
shortPskBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder setShortPsk(
int index, rock.protobuf.Rock.Psk.Builder builderForValue) {
if (shortPskBuilder_ == null) {
ensureShortPskIsMutable();
shortPsk_.set(index, builderForValue.build());
onChanged();
} else {
shortPskBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder addShortPsk(rock.protobuf.Rock.Psk value) {
if (shortPskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShortPskIsMutable();
shortPsk_.add(value);
onChanged();
} else {
shortPskBuilder_.addMessage(value);
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder addShortPsk(
int index, rock.protobuf.Rock.Psk value) {
if (shortPskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShortPskIsMutable();
shortPsk_.add(index, value);
onChanged();
} else {
shortPskBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder addShortPsk(
rock.protobuf.Rock.Psk.Builder builderForValue) {
if (shortPskBuilder_ == null) {
ensureShortPskIsMutable();
shortPsk_.add(builderForValue.build());
onChanged();
} else {
shortPskBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder addShortPsk(
int index, rock.protobuf.Rock.Psk.Builder builderForValue) {
if (shortPskBuilder_ == null) {
ensureShortPskIsMutable();
shortPsk_.add(index, builderForValue.build());
onChanged();
} else {
shortPskBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder addAllShortPsk(
java.lang.Iterable extends rock.protobuf.Rock.Psk> values) {
if (shortPskBuilder_ == null) {
ensureShortPskIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, shortPsk_);
onChanged();
} else {
shortPskBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder clearShortPsk() {
if (shortPskBuilder_ == null) {
shortPsk_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
shortPskBuilder_.clear();
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public Builder removeShortPsk(int index) {
if (shortPskBuilder_ == null) {
ensureShortPskIsMutable();
shortPsk_.remove(index);
onChanged();
} else {
shortPskBuilder_.remove(index);
}
return this;
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public rock.protobuf.Rock.Psk.Builder getShortPskBuilder(
int index) {
return getShortPskFieldBuilder().getBuilder(index);
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public rock.protobuf.Rock.PskOrBuilder getShortPskOrBuilder(
int index) {
if (shortPskBuilder_ == null) {
return shortPsk_.get(index); } else {
return shortPskBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public java.util.List extends rock.protobuf.Rock.PskOrBuilder>
getShortPskOrBuilderList() {
if (shortPskBuilder_ != null) {
return shortPskBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(shortPsk_);
}
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public rock.protobuf.Rock.Psk.Builder addShortPskBuilder() {
return getShortPskFieldBuilder().addBuilder(
rock.protobuf.Rock.Psk.getDefaultInstance());
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public rock.protobuf.Rock.Psk.Builder addShortPskBuilder(
int index) {
return getShortPskFieldBuilder().addBuilder(
index, rock.protobuf.Rock.Psk.getDefaultInstance());
}
/**
*
* 短链接会话票据(服务端返回, 第一次握手不设置), 下一次握手选择其中一个发给服务器, 需要保存到数据库
*
*
* repeated .rock.Psk ShortPsk = 3;
*/
public java.util.List
getShortPskBuilderList() {
return getShortPskFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
rock.protobuf.Rock.Psk, rock.protobuf.Rock.Psk.Builder, rock.protobuf.Rock.PskOrBuilder>
getShortPskFieldBuilder() {
if (shortPskBuilder_ == null) {
shortPskBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
rock.protobuf.Rock.Psk, rock.protobuf.Rock.Psk.Builder, rock.protobuf.Rock.PskOrBuilder>(
shortPsk_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
shortPsk_ = null;
}
return shortPskBuilder_;
}
private com.google.protobuf.ByteString pskAccessKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @return Whether the pskAccessKey field is set.
*/
@java.lang.Override
public boolean hasPskAccessKey() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @return The pskAccessKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPskAccessKey() {
return pskAccessKey_;
}
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @param value The pskAccessKey to set.
* @return This builder for chaining.
*/
public Builder setPskAccessKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
pskAccessKey_ = value;
onChanged();
return this;
}
/**
*
* 握手扩展出来的用于后续加密的Key
*
*
* optional bytes PskAccessKey = 4;
* @return This builder for chaining.
*/
public Builder clearPskAccessKey() {
bitField0_ = (bitField0_ & ~0x00000008);
pskAccessKey_ = getDefaultInstance().getPskAccessKey();
onChanged();
return this;
}
private java.lang.Object longHost_ = "";
/**
* optional string LongHost = 5;
* @return Whether the longHost field is set.
*/
public boolean hasLongHost() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string LongHost = 5;
* @return The longHost.
*/
public java.lang.String getLongHost() {
java.lang.Object ref = longHost_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
longHost_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string LongHost = 5;
* @return The bytes for longHost.
*/
public com.google.protobuf.ByteString
getLongHostBytes() {
java.lang.Object ref = longHost_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string LongHost = 5;
* @param value The longHost to set.
* @return This builder for chaining.
*/
public Builder setLongHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
longHost_ = value;
onChanged();
return this;
}
/**
* optional string LongHost = 5;
* @return This builder for chaining.
*/
public Builder clearLongHost() {
bitField0_ = (bitField0_ & ~0x00000010);
longHost_ = getDefaultInstance().getLongHost();
onChanged();
return this;
}
/**
* optional string LongHost = 5;
* @param value The bytes for longHost to set.
* @return This builder for chaining.
*/
public Builder setLongHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
longHost_ = value;
onChanged();
return this;
}
private int longPort_ ;
/**
* optional int32 LongPort = 6;
* @return Whether the longPort field is set.
*/
@java.lang.Override
public boolean hasLongPort() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional int32 LongPort = 6;
* @return The longPort.
*/
@java.lang.Override
public int getLongPort() {
return longPort_;
}
/**
* optional int32 LongPort = 6;
* @param value The longPort to set.
* @return This builder for chaining.
*/
public Builder setLongPort(int value) {
bitField0_ |= 0x00000020;
longPort_ = value;
onChanged();
return this;
}
/**
* optional int32 LongPort = 6;
* @return This builder for chaining.
*/
public Builder clearLongPort() {
bitField0_ = (bitField0_ & ~0x00000020);
longPort_ = 0;
onChanged();
return this;
}
private rock.protobuf.Rock.HkdfKey56 hkdfKey_;
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.HkdfKey56, rock.protobuf.Rock.HkdfKey56.Builder, rock.protobuf.Rock.HkdfKey56OrBuilder> hkdfKeyBuilder_;
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
* @return Whether the hkdfKey field is set.
*/
public boolean hasHkdfKey() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
* @return The hkdfKey.
*/
public rock.protobuf.Rock.HkdfKey56 getHkdfKey() {
if (hkdfKeyBuilder_ == null) {
return hkdfKey_ == null ? rock.protobuf.Rock.HkdfKey56.getDefaultInstance() : hkdfKey_;
} else {
return hkdfKeyBuilder_.getMessage();
}
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
public Builder setHkdfKey(rock.protobuf.Rock.HkdfKey56 value) {
if (hkdfKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
hkdfKey_ = value;
onChanged();
} else {
hkdfKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
public Builder setHkdfKey(
rock.protobuf.Rock.HkdfKey56.Builder builderForValue) {
if (hkdfKeyBuilder_ == null) {
hkdfKey_ = builderForValue.build();
onChanged();
} else {
hkdfKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
public Builder mergeHkdfKey(rock.protobuf.Rock.HkdfKey56 value) {
if (hkdfKeyBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
hkdfKey_ != null &&
hkdfKey_ != rock.protobuf.Rock.HkdfKey56.getDefaultInstance()) {
hkdfKey_ =
rock.protobuf.Rock.HkdfKey56.newBuilder(hkdfKey_).mergeFrom(value).buildPartial();
} else {
hkdfKey_ = value;
}
onChanged();
} else {
hkdfKeyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
public Builder clearHkdfKey() {
if (hkdfKeyBuilder_ == null) {
hkdfKey_ = null;
onChanged();
} else {
hkdfKeyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
public rock.protobuf.Rock.HkdfKey56.Builder getHkdfKeyBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getHkdfKeyFieldBuilder().getBuilder();
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
public rock.protobuf.Rock.HkdfKey56OrBuilder getHkdfKeyOrBuilder() {
if (hkdfKeyBuilder_ != null) {
return hkdfKeyBuilder_.getMessageOrBuilder();
} else {
return hkdfKey_ == null ?
rock.protobuf.Rock.HkdfKey56.getDefaultInstance() : hkdfKey_;
}
}
/**
* optional .rock.HkdfKey56 hkdfKey = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.HkdfKey56, rock.protobuf.Rock.HkdfKey56.Builder, rock.protobuf.Rock.HkdfKey56OrBuilder>
getHkdfKeyFieldBuilder() {
if (hkdfKeyBuilder_ == null) {
hkdfKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
rock.protobuf.Rock.HkdfKey56, rock.protobuf.Rock.HkdfKey56.Builder, rock.protobuf.Rock.HkdfKey56OrBuilder>(
getHkdfKey(),
getParentForChildren(),
isClean());
hkdfKey_ = null;
}
return hkdfKeyBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.MMInfo)
}
// @@protoc_insertion_point(class_scope:rock.MMInfo)
private static final rock.protobuf.Rock.MMInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.MMInfo();
}
public static rock.protobuf.Rock.MMInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MMInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MMInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.MMInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HkdfKey56OrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.HkdfKey56)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes EncodeAesKey = 1;
* @return Whether the encodeAesKey field is set.
*/
boolean hasEncodeAesKey();
/**
* optional bytes EncodeAesKey = 1;
* @return The encodeAesKey.
*/
com.google.protobuf.ByteString getEncodeAesKey();
/**
* optional bytes EncodeNonce = 2;
* @return Whether the encodeNonce field is set.
*/
boolean hasEncodeNonce();
/**
* optional bytes EncodeNonce = 2;
* @return The encodeNonce.
*/
com.google.protobuf.ByteString getEncodeNonce();
/**
* optional bytes DecodeAesKey = 3;
* @return Whether the decodeAesKey field is set.
*/
boolean hasDecodeAesKey();
/**
* optional bytes DecodeAesKey = 3;
* @return The decodeAesKey.
*/
com.google.protobuf.ByteString getDecodeAesKey();
/**
* optional bytes DecodeNonce = 4;
* @return Whether the decodeNonce field is set.
*/
boolean hasDecodeNonce();
/**
* optional bytes DecodeNonce = 4;
* @return The decodeNonce.
*/
com.google.protobuf.ByteString getDecodeNonce();
}
/**
* Protobuf type {@code rock.HkdfKey56}
*/
public static final class HkdfKey56 extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.HkdfKey56)
HkdfKey56OrBuilder {
private static final long serialVersionUID = 0L;
// Use HkdfKey56.newBuilder() to construct.
private HkdfKey56(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HkdfKey56() {
encodeAesKey_ = com.google.protobuf.ByteString.EMPTY;
encodeNonce_ = com.google.protobuf.ByteString.EMPTY;
decodeAesKey_ = com.google.protobuf.ByteString.EMPTY;
decodeNonce_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HkdfKey56();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HkdfKey56(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
encodeAesKey_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
encodeNonce_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
decodeAesKey_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
decodeNonce_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_HkdfKey56_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_HkdfKey56_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.HkdfKey56.class, rock.protobuf.Rock.HkdfKey56.Builder.class);
}
private int bitField0_;
public static final int ENCODEAESKEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString encodeAesKey_;
/**
* optional bytes EncodeAesKey = 1;
* @return Whether the encodeAesKey field is set.
*/
@java.lang.Override
public boolean hasEncodeAesKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes EncodeAesKey = 1;
* @return The encodeAesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEncodeAesKey() {
return encodeAesKey_;
}
public static final int ENCODENONCE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString encodeNonce_;
/**
* optional bytes EncodeNonce = 2;
* @return Whether the encodeNonce field is set.
*/
@java.lang.Override
public boolean hasEncodeNonce() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes EncodeNonce = 2;
* @return The encodeNonce.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEncodeNonce() {
return encodeNonce_;
}
public static final int DECODEAESKEY_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString decodeAesKey_;
/**
* optional bytes DecodeAesKey = 3;
* @return Whether the decodeAesKey field is set.
*/
@java.lang.Override
public boolean hasDecodeAesKey() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes DecodeAesKey = 3;
* @return The decodeAesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDecodeAesKey() {
return decodeAesKey_;
}
public static final int DECODENONCE_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString decodeNonce_;
/**
* optional bytes DecodeNonce = 4;
* @return Whether the decodeNonce field is set.
*/
@java.lang.Override
public boolean hasDecodeNonce() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bytes DecodeNonce = 4;
* @return The decodeNonce.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDecodeNonce() {
return decodeNonce_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, encodeAesKey_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, encodeNonce_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, decodeAesKey_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBytes(4, decodeNonce_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, encodeAesKey_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, encodeNonce_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, decodeAesKey_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, decodeNonce_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.HkdfKey56)) {
return super.equals(obj);
}
rock.protobuf.Rock.HkdfKey56 other = (rock.protobuf.Rock.HkdfKey56) obj;
if (hasEncodeAesKey() != other.hasEncodeAesKey()) return false;
if (hasEncodeAesKey()) {
if (!getEncodeAesKey()
.equals(other.getEncodeAesKey())) return false;
}
if (hasEncodeNonce() != other.hasEncodeNonce()) return false;
if (hasEncodeNonce()) {
if (!getEncodeNonce()
.equals(other.getEncodeNonce())) return false;
}
if (hasDecodeAesKey() != other.hasDecodeAesKey()) return false;
if (hasDecodeAesKey()) {
if (!getDecodeAesKey()
.equals(other.getDecodeAesKey())) return false;
}
if (hasDecodeNonce() != other.hasDecodeNonce()) return false;
if (hasDecodeNonce()) {
if (!getDecodeNonce()
.equals(other.getDecodeNonce())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasEncodeAesKey()) {
hash = (37 * hash) + ENCODEAESKEY_FIELD_NUMBER;
hash = (53 * hash) + getEncodeAesKey().hashCode();
}
if (hasEncodeNonce()) {
hash = (37 * hash) + ENCODENONCE_FIELD_NUMBER;
hash = (53 * hash) + getEncodeNonce().hashCode();
}
if (hasDecodeAesKey()) {
hash = (37 * hash) + DECODEAESKEY_FIELD_NUMBER;
hash = (53 * hash) + getDecodeAesKey().hashCode();
}
if (hasDecodeNonce()) {
hash = (37 * hash) + DECODENONCE_FIELD_NUMBER;
hash = (53 * hash) + getDecodeNonce().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.HkdfKey56 parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.HkdfKey56 parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.HkdfKey56 parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.HkdfKey56 prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code rock.HkdfKey56}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.HkdfKey56)
rock.protobuf.Rock.HkdfKey56OrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_HkdfKey56_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_HkdfKey56_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.HkdfKey56.class, rock.protobuf.Rock.HkdfKey56.Builder.class);
}
// Construct using rock.protobuf.Rock.HkdfKey56.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
encodeAesKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
encodeNonce_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
decodeAesKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
decodeNonce_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_HkdfKey56_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.HkdfKey56 getDefaultInstanceForType() {
return rock.protobuf.Rock.HkdfKey56.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.HkdfKey56 build() {
rock.protobuf.Rock.HkdfKey56 result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.HkdfKey56 buildPartial() {
rock.protobuf.Rock.HkdfKey56 result = new rock.protobuf.Rock.HkdfKey56(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.encodeAesKey_ = encodeAesKey_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.encodeNonce_ = encodeNonce_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.decodeAesKey_ = decodeAesKey_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.decodeNonce_ = decodeNonce_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.HkdfKey56) {
return mergeFrom((rock.protobuf.Rock.HkdfKey56)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.HkdfKey56 other) {
if (other == rock.protobuf.Rock.HkdfKey56.getDefaultInstance()) return this;
if (other.hasEncodeAesKey()) {
setEncodeAesKey(other.getEncodeAesKey());
}
if (other.hasEncodeNonce()) {
setEncodeNonce(other.getEncodeNonce());
}
if (other.hasDecodeAesKey()) {
setDecodeAesKey(other.getDecodeAesKey());
}
if (other.hasDecodeNonce()) {
setDecodeNonce(other.getDecodeNonce());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.HkdfKey56 parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.HkdfKey56) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString encodeAesKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes EncodeAesKey = 1;
* @return Whether the encodeAesKey field is set.
*/
@java.lang.Override
public boolean hasEncodeAesKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes EncodeAesKey = 1;
* @return The encodeAesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEncodeAesKey() {
return encodeAesKey_;
}
/**
* optional bytes EncodeAesKey = 1;
* @param value The encodeAesKey to set.
* @return This builder for chaining.
*/
public Builder setEncodeAesKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
encodeAesKey_ = value;
onChanged();
return this;
}
/**
* optional bytes EncodeAesKey = 1;
* @return This builder for chaining.
*/
public Builder clearEncodeAesKey() {
bitField0_ = (bitField0_ & ~0x00000001);
encodeAesKey_ = getDefaultInstance().getEncodeAesKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString encodeNonce_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes EncodeNonce = 2;
* @return Whether the encodeNonce field is set.
*/
@java.lang.Override
public boolean hasEncodeNonce() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes EncodeNonce = 2;
* @return The encodeNonce.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEncodeNonce() {
return encodeNonce_;
}
/**
* optional bytes EncodeNonce = 2;
* @param value The encodeNonce to set.
* @return This builder for chaining.
*/
public Builder setEncodeNonce(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
encodeNonce_ = value;
onChanged();
return this;
}
/**
* optional bytes EncodeNonce = 2;
* @return This builder for chaining.
*/
public Builder clearEncodeNonce() {
bitField0_ = (bitField0_ & ~0x00000002);
encodeNonce_ = getDefaultInstance().getEncodeNonce();
onChanged();
return this;
}
private com.google.protobuf.ByteString decodeAesKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes DecodeAesKey = 3;
* @return Whether the decodeAesKey field is set.
*/
@java.lang.Override
public boolean hasDecodeAesKey() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes DecodeAesKey = 3;
* @return The decodeAesKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDecodeAesKey() {
return decodeAesKey_;
}
/**
* optional bytes DecodeAesKey = 3;
* @param value The decodeAesKey to set.
* @return This builder for chaining.
*/
public Builder setDecodeAesKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
decodeAesKey_ = value;
onChanged();
return this;
}
/**
* optional bytes DecodeAesKey = 3;
* @return This builder for chaining.
*/
public Builder clearDecodeAesKey() {
bitField0_ = (bitField0_ & ~0x00000004);
decodeAesKey_ = getDefaultInstance().getDecodeAesKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString decodeNonce_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes DecodeNonce = 4;
* @return Whether the decodeNonce field is set.
*/
@java.lang.Override
public boolean hasDecodeNonce() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bytes DecodeNonce = 4;
* @return The decodeNonce.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDecodeNonce() {
return decodeNonce_;
}
/**
* optional bytes DecodeNonce = 4;
* @param value The decodeNonce to set.
* @return This builder for chaining.
*/
public Builder setDecodeNonce(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
decodeNonce_ = value;
onChanged();
return this;
}
/**
* optional bytes DecodeNonce = 4;
* @return This builder for chaining.
*/
public Builder clearDecodeNonce() {
bitField0_ = (bitField0_ & ~0x00000008);
decodeNonce_ = getDefaultInstance().getDecodeNonce();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.HkdfKey56)
}
// @@protoc_insertion_point(class_scope:rock.HkdfKey56)
private static final rock.protobuf.Rock.HkdfKey56 DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.HkdfKey56();
}
public static rock.protobuf.Rock.HkdfKey56 getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HkdfKey56 parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HkdfKey56(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.HkdfKey56 getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PskOrBuilder extends
// @@protoc_insertion_point(interface_extends:rock.Psk)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 Kind = 1;
* @return Whether the kind field is set.
*/
boolean hasKind();
/**
* optional int32 Kind = 1;
* @return The kind.
*/
int getKind();
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @return Whether the ticketKLifeTimeHint field is set.
*/
boolean hasTicketKLifeTimeHint();
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @return The ticketKLifeTimeHint.
*/
int getTicketKLifeTimeHint();
/**
* optional bytes MacValue = 3;
* @return Whether the macValue field is set.
*/
boolean hasMacValue();
/**
* optional bytes MacValue = 3;
* @return The macValue.
*/
com.google.protobuf.ByteString getMacValue();
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @return Whether the keyVersion field is set.
*/
boolean hasKeyVersion();
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @return The keyVersion.
*/
int getKeyVersion();
/**
* optional bytes Iv = 5;
* @return Whether the iv field is set.
*/
boolean hasIv();
/**
* optional bytes Iv = 5;
* @return The iv.
*/
com.google.protobuf.ByteString getIv();
/**
* optional bytes EncryptedTicket = 6;
* @return Whether the encryptedTicket field is set.
*/
boolean hasEncryptedTicket();
/**
* optional bytes EncryptedTicket = 6;
* @return The encryptedTicket.
*/
com.google.protobuf.ByteString getEncryptedTicket();
}
/**
*
* Psk Psk
*
*
* Protobuf type {@code rock.Psk}
*/
public static final class Psk extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:rock.Psk)
PskOrBuilder {
private static final long serialVersionUID = 0L;
// Use Psk.newBuilder() to construct.
private Psk(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Psk() {
macValue_ = com.google.protobuf.ByteString.EMPTY;
iv_ = com.google.protobuf.ByteString.EMPTY;
encryptedTicket_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Psk();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Psk(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
kind_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
ticketKLifeTimeHint_ = input.readUInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
macValue_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
keyVersion_ = input.readUInt32();
break;
}
case 42: {
bitField0_ |= 0x00000010;
iv_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
encryptedTicket_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_Psk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_Psk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.Psk.class, rock.protobuf.Rock.Psk.Builder.class);
}
private int bitField0_;
public static final int KIND_FIELD_NUMBER = 1;
private int kind_;
/**
* optional int32 Kind = 1;
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 Kind = 1;
* @return The kind.
*/
@java.lang.Override
public int getKind() {
return kind_;
}
public static final int TICKETKLIFETIMEHINT_FIELD_NUMBER = 2;
private int ticketKLifeTimeHint_;
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @return Whether the ticketKLifeTimeHint field is set.
*/
@java.lang.Override
public boolean hasTicketKLifeTimeHint() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @return The ticketKLifeTimeHint.
*/
@java.lang.Override
public int getTicketKLifeTimeHint() {
return ticketKLifeTimeHint_;
}
public static final int MACVALUE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString macValue_;
/**
* optional bytes MacValue = 3;
* @return Whether the macValue field is set.
*/
@java.lang.Override
public boolean hasMacValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes MacValue = 3;
* @return The macValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getMacValue() {
return macValue_;
}
public static final int KEYVERSION_FIELD_NUMBER = 4;
private int keyVersion_;
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @return Whether the keyVersion field is set.
*/
@java.lang.Override
public boolean hasKeyVersion() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @return The keyVersion.
*/
@java.lang.Override
public int getKeyVersion() {
return keyVersion_;
}
public static final int IV_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString iv_;
/**
* optional bytes Iv = 5;
* @return Whether the iv field is set.
*/
@java.lang.Override
public boolean hasIv() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bytes Iv = 5;
* @return The iv.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIv() {
return iv_;
}
public static final int ENCRYPTEDTICKET_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString encryptedTicket_;
/**
* optional bytes EncryptedTicket = 6;
* @return Whether the encryptedTicket field is set.
*/
@java.lang.Override
public boolean hasEncryptedTicket() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional bytes EncryptedTicket = 6;
* @return The encryptedTicket.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEncryptedTicket() {
return encryptedTicket_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, kind_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeUInt32(2, ticketKLifeTimeHint_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, macValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeUInt32(4, keyVersion_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBytes(5, iv_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBytes(6, encryptedTicket_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, kind_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, ticketKLifeTimeHint_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, macValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, keyVersion_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, iv_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, encryptedTicket_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof rock.protobuf.Rock.Psk)) {
return super.equals(obj);
}
rock.protobuf.Rock.Psk other = (rock.protobuf.Rock.Psk) obj;
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (getKind()
!= other.getKind()) return false;
}
if (hasTicketKLifeTimeHint() != other.hasTicketKLifeTimeHint()) return false;
if (hasTicketKLifeTimeHint()) {
if (getTicketKLifeTimeHint()
!= other.getTicketKLifeTimeHint()) return false;
}
if (hasMacValue() != other.hasMacValue()) return false;
if (hasMacValue()) {
if (!getMacValue()
.equals(other.getMacValue())) return false;
}
if (hasKeyVersion() != other.hasKeyVersion()) return false;
if (hasKeyVersion()) {
if (getKeyVersion()
!= other.getKeyVersion()) return false;
}
if (hasIv() != other.hasIv()) return false;
if (hasIv()) {
if (!getIv()
.equals(other.getIv())) return false;
}
if (hasEncryptedTicket() != other.hasEncryptedTicket()) return false;
if (hasEncryptedTicket()) {
if (!getEncryptedTicket()
.equals(other.getEncryptedTicket())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind();
}
if (hasTicketKLifeTimeHint()) {
hash = (37 * hash) + TICKETKLIFETIMEHINT_FIELD_NUMBER;
hash = (53 * hash) + getTicketKLifeTimeHint();
}
if (hasMacValue()) {
hash = (37 * hash) + MACVALUE_FIELD_NUMBER;
hash = (53 * hash) + getMacValue().hashCode();
}
if (hasKeyVersion()) {
hash = (37 * hash) + KEYVERSION_FIELD_NUMBER;
hash = (53 * hash) + getKeyVersion();
}
if (hasIv()) {
hash = (37 * hash) + IV_FIELD_NUMBER;
hash = (53 * hash) + getIv().hashCode();
}
if (hasEncryptedTicket()) {
hash = (37 * hash) + ENCRYPTEDTICKET_FIELD_NUMBER;
hash = (53 * hash) + getEncryptedTicket().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static rock.protobuf.Rock.Psk parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.Psk parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.Psk parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.Psk parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.Psk parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static rock.protobuf.Rock.Psk parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static rock.protobuf.Rock.Psk parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.Psk parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.Psk parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.Psk parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static rock.protobuf.Rock.Psk parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static rock.protobuf.Rock.Psk parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(rock.protobuf.Rock.Psk prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Psk Psk
*
*
* Protobuf type {@code rock.Psk}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:rock.Psk)
rock.protobuf.Rock.PskOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return rock.protobuf.Rock.internal_static_rock_Psk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return rock.protobuf.Rock.internal_static_rock_Psk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
rock.protobuf.Rock.Psk.class, rock.protobuf.Rock.Psk.Builder.class);
}
// Construct using rock.protobuf.Rock.Psk.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
kind_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
ticketKLifeTimeHint_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
macValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
keyVersion_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
iv_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
encryptedTicket_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return rock.protobuf.Rock.internal_static_rock_Psk_descriptor;
}
@java.lang.Override
public rock.protobuf.Rock.Psk getDefaultInstanceForType() {
return rock.protobuf.Rock.Psk.getDefaultInstance();
}
@java.lang.Override
public rock.protobuf.Rock.Psk build() {
rock.protobuf.Rock.Psk result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public rock.protobuf.Rock.Psk buildPartial() {
rock.protobuf.Rock.Psk result = new rock.protobuf.Rock.Psk(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.ticketKLifeTimeHint_ = ticketKLifeTimeHint_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.macValue_ = macValue_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.keyVersion_ = keyVersion_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.iv_ = iv_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.encryptedTicket_ = encryptedTicket_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof rock.protobuf.Rock.Psk) {
return mergeFrom((rock.protobuf.Rock.Psk)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(rock.protobuf.Rock.Psk other) {
if (other == rock.protobuf.Rock.Psk.getDefaultInstance()) return this;
if (other.hasKind()) {
setKind(other.getKind());
}
if (other.hasTicketKLifeTimeHint()) {
setTicketKLifeTimeHint(other.getTicketKLifeTimeHint());
}
if (other.hasMacValue()) {
setMacValue(other.getMacValue());
}
if (other.hasKeyVersion()) {
setKeyVersion(other.getKeyVersion());
}
if (other.hasIv()) {
setIv(other.getIv());
}
if (other.hasEncryptedTicket()) {
setEncryptedTicket(other.getEncryptedTicket());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
rock.protobuf.Rock.Psk parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (rock.protobuf.Rock.Psk) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int kind_ ;
/**
* optional int32 Kind = 1;
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 Kind = 1;
* @return The kind.
*/
@java.lang.Override
public int getKind() {
return kind_;
}
/**
* optional int32 Kind = 1;
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(int value) {
bitField0_ |= 0x00000001;
kind_ = value;
onChanged();
return this;
}
/**
* optional int32 Kind = 1;
* @return This builder for chaining.
*/
public Builder clearKind() {
bitField0_ = (bitField0_ & ~0x00000001);
kind_ = 0;
onChanged();
return this;
}
private int ticketKLifeTimeHint_ ;
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @return Whether the ticketKLifeTimeHint field is set.
*/
@java.lang.Override
public boolean hasTicketKLifeTimeHint() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @return The ticketKLifeTimeHint.
*/
@java.lang.Override
public int getTicketKLifeTimeHint() {
return ticketKLifeTimeHint_;
}
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @param value The ticketKLifeTimeHint to set.
* @return This builder for chaining.
*/
public Builder setTicketKLifeTimeHint(int value) {
bitField0_ |= 0x00000002;
ticketKLifeTimeHint_ = value;
onChanged();
return this;
}
/**
*
* uint32
*
*
* optional uint32 TicketKLifeTimeHint = 2;
* @return This builder for chaining.
*/
public Builder clearTicketKLifeTimeHint() {
bitField0_ = (bitField0_ & ~0x00000002);
ticketKLifeTimeHint_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString macValue_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes MacValue = 3;
* @return Whether the macValue field is set.
*/
@java.lang.Override
public boolean hasMacValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes MacValue = 3;
* @return The macValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getMacValue() {
return macValue_;
}
/**
* optional bytes MacValue = 3;
* @param value The macValue to set.
* @return This builder for chaining.
*/
public Builder setMacValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
macValue_ = value;
onChanged();
return this;
}
/**
* optional bytes MacValue = 3;
* @return This builder for chaining.
*/
public Builder clearMacValue() {
bitField0_ = (bitField0_ & ~0x00000004);
macValue_ = getDefaultInstance().getMacValue();
onChanged();
return this;
}
private int keyVersion_ ;
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @return Whether the keyVersion field is set.
*/
@java.lang.Override
public boolean hasKeyVersion() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @return The keyVersion.
*/
@java.lang.Override
public int getKeyVersion() {
return keyVersion_;
}
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @param value The keyVersion to set.
* @return This builder for chaining.
*/
public Builder setKeyVersion(int value) {
bitField0_ |= 0x00000008;
keyVersion_ = value;
onChanged();
return this;
}
/**
*
* uint32
*
*
* optional uint32 KeyVersion = 4;
* @return This builder for chaining.
*/
public Builder clearKeyVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
keyVersion_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString iv_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes Iv = 5;
* @return Whether the iv field is set.
*/
@java.lang.Override
public boolean hasIv() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bytes Iv = 5;
* @return The iv.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIv() {
return iv_;
}
/**
* optional bytes Iv = 5;
* @param value The iv to set.
* @return This builder for chaining.
*/
public Builder setIv(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
iv_ = value;
onChanged();
return this;
}
/**
* optional bytes Iv = 5;
* @return This builder for chaining.
*/
public Builder clearIv() {
bitField0_ = (bitField0_ & ~0x00000010);
iv_ = getDefaultInstance().getIv();
onChanged();
return this;
}
private com.google.protobuf.ByteString encryptedTicket_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes EncryptedTicket = 6;
* @return Whether the encryptedTicket field is set.
*/
@java.lang.Override
public boolean hasEncryptedTicket() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional bytes EncryptedTicket = 6;
* @return The encryptedTicket.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEncryptedTicket() {
return encryptedTicket_;
}
/**
* optional bytes EncryptedTicket = 6;
* @param value The encryptedTicket to set.
* @return This builder for chaining.
*/
public Builder setEncryptedTicket(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
encryptedTicket_ = value;
onChanged();
return this;
}
/**
* optional bytes EncryptedTicket = 6;
* @return This builder for chaining.
*/
public Builder clearEncryptedTicket() {
bitField0_ = (bitField0_ & ~0x00000020);
encryptedTicket_ = getDefaultInstance().getEncryptedTicket();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:rock.Psk)
}
// @@protoc_insertion_point(class_scope:rock.Psk)
private static final rock.protobuf.Rock.Psk DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new rock.protobuf.Rock.Psk();
}
public static rock.protobuf.Rock.Psk getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Psk parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Psk(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public rock.protobuf.Rock.Psk getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_RockRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_RockRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_RockResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_RockResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_DeviceInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_DeviceInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_UserInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_UserInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_WifiInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_WifiInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_MMInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_MMInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_HkdfKey56_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_HkdfKey56_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_rock_Psk_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_rock_Psk_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\nrock.proto\022\004rock\032\014wechat.proto\"=\n\013Rock" +
"Request\022 \n\010UserInfo\030\001 \002(\0132\016.rock.UserInf" +
"o\022\014\n\004data\030\002 \002(\014\">\n\014RockResponse\022 \n\010UserI" +
"nfo\030\001 \002(\0132\016.rock.UserInfo\022\014\n\004data\030\002 \002(\014\"" +
"\346\002\n\nDeviceInfo\022\017\n\007UUIDOne\030\001 \001(\t\022\017\n\007UUIDT" +
"wo\030\002 \001(\t\022\014\n\004Imei\030\003 \001(\t\022\020\n\010DeviceID\030\004 \001(\014" +
"\022\022\n\nDeviceName\030\005 \001(\t\022\020\n\010TimeZone\030\006 \001(\t\022\020" +
"\n\010Language\030\007 \001(\t\022\023\n\013DeviceBrand\030\010 \001(\t\022\023\n" +
"\013RealCountry\030\t \001(\t\022\021\n\tIphoneVer\030\n \001(\t\022\020\n" +
"\010BundleID\030\013 \001(\t\022\016\n\006OsType\030\014 \001(\t\022\020\n\010AdSou" +
"rce\030\r \001(\t\022\024\n\014OsTypeNumber\030\016 \001(\t\022\021\n\tCoreC" +
"ount\030\017 \001(\r\022\023\n\013CarrierName\030\020 \001(\t\022\023\n\013SoftT" +
"ypeXML\030\021 \001(\t\022\032\n\022ClientCheckDataXML\030\022 \001(\t" +
"\"\213\005\n\010UserInfo\022\014\n\004UUID\030\001 \001(\t\022\013\n\003Uin\030\002 \001(\r" +
"\022\014\n\004Wxid\030\003 \001(\t\022\020\n\010NickName\030\004 \001(\t\022\017\n\007Head" +
"URL\030\005 \001(\t\022\017\n\007Session\030\006 \001(\014\022\016\n\006AesKey\030\007 \001" +
"(\014\022\021\n\tShortHost\030\010 \001(\t\022\020\n\010LongHost\030\t \001(\t\022" +
"\023\n\013EcPublicKey\030\n \001(\014\022\024\n\014EcPrivateKey\030\013 \001" +
"(\014\022\023\n\013CheckSumKey\030\014 \001(\014\022\023\n\013AutoAuthKey\030\r" +
" \001(\014\022\017\n\007SyncKey\030\016 \001(\014\022\022\n\nFavSyncKey\030\017 \001(" +
"\014\022\022\n\nSnsSyncKey\030\020 \001(\014\022\020\n\010HBAesKey\030\021 \001(\014\022" +
"\031\n\021HBAesKeyEncrypted\030\022 \001(\t\022)\n\007DNSInfo\030\023 " +
"\001(\0132\030.wechat_proto.CDNDnsInfo\022,\n\nSNSDnsI" +
"nfo\030\024 \001(\0132\030.wechat_proto.CDNDnsInfo\022,\n\nA" +
"PPDnsInfo\030\025 \001(\0132\030.wechat_proto.CDNDnsInf" +
"o\022-\n\013FAKEDnsInfo\030\026 \001(\0132\030.wechat_proto.CD" +
"NDnsInfo\022$\n\nDeviceInfo\030\027 \001(\0132\020.rock.Devi" +
"ceInfo\022\026\n\016BalanceVersion\030\030 \001(\r\022 \n\010WifiIn" +
"fo\030\031 \001(\0132\016.rock.WifiInfo\022\034\n\006MMInfo\030\032 \001(\013" +
"2\014.rock.MMInfo\"+\n\010WifiInfo\022\014\n\004Name\030\001 \001(\t" +
"\022\021\n\tWifiBssID\030\002 \001(\t\"\246\001\n\006MMInfo\022\021\n\tShortH" +
"ost\030\001 \001(\t\022\020\n\010ShortURL\030\002 \001(\t\022\033\n\010ShortPsk\030" +
"\003 \003(\0132\t.rock.Psk\022\024\n\014PskAccessKey\030\004 \001(\014\022\020" +
"\n\010LongHost\030\005 \001(\t\022\020\n\010LongPort\030\006 \001(\005\022 \n\007hk" +
"dfKey\030\007 \001(\0132\017.rock.HkdfKey56\"a\n\tHkdfKey5" +
"6\022\024\n\014EncodeAesKey\030\001 \001(\014\022\023\n\013EncodeNonce\030\002" +
" \001(\014\022\024\n\014DecodeAesKey\030\003 \001(\014\022\023\n\013DecodeNonc" +
"e\030\004 \001(\014\"{\n\003Psk\022\014\n\004Kind\030\001 \001(\005\022\033\n\023TicketKL" +
"ifeTimeHint\030\002 \001(\r\022\020\n\010MacValue\030\003 \001(\014\022\022\n\nK" +
"eyVersion\030\004 \001(\r\022\n\n\002Iv\030\005 \001(\014\022\027\n\017Encrypted" +
"Ticket\030\006 \001(\014B\017\n\rrock.protobuf"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
wechat.protobuf.Wechat.getDescriptor(),
});
internal_static_rock_RockRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_rock_RockRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_RockRequest_descriptor,
new java.lang.String[] { "UserInfo", "Data", });
internal_static_rock_RockResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_rock_RockResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_RockResponse_descriptor,
new java.lang.String[] { "UserInfo", "Data", });
internal_static_rock_DeviceInfo_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_rock_DeviceInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_DeviceInfo_descriptor,
new java.lang.String[] { "UUIDOne", "UUIDTwo", "Imei", "DeviceID", "DeviceName", "TimeZone", "Language", "DeviceBrand", "RealCountry", "IphoneVer", "BundleID", "OsType", "AdSource", "OsTypeNumber", "CoreCount", "CarrierName", "SoftTypeXML", "ClientCheckDataXML", });
internal_static_rock_UserInfo_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_rock_UserInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_UserInfo_descriptor,
new java.lang.String[] { "UUID", "Uin", "Wxid", "NickName", "HeadURL", "Session", "AesKey", "ShortHost", "LongHost", "EcPublicKey", "EcPrivateKey", "CheckSumKey", "AutoAuthKey", "SyncKey", "FavSyncKey", "SnsSyncKey", "HBAesKey", "HBAesKeyEncrypted", "DNSInfo", "SNSDnsInfo", "APPDnsInfo", "FAKEDnsInfo", "DeviceInfo", "BalanceVersion", "WifiInfo", "MMInfo", });
internal_static_rock_WifiInfo_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_rock_WifiInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_WifiInfo_descriptor,
new java.lang.String[] { "Name", "WifiBssID", });
internal_static_rock_MMInfo_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_rock_MMInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_MMInfo_descriptor,
new java.lang.String[] { "ShortHost", "ShortURL", "ShortPsk", "PskAccessKey", "LongHost", "LongPort", "HkdfKey", });
internal_static_rock_HkdfKey56_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_rock_HkdfKey56_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_HkdfKey56_descriptor,
new java.lang.String[] { "EncodeAesKey", "EncodeNonce", "DecodeAesKey", "DecodeNonce", });
internal_static_rock_Psk_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_rock_Psk_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_rock_Psk_descriptor,
new java.lang.String[] { "Kind", "TicketKLifeTimeHint", "MacValue", "KeyVersion", "Iv", "EncryptedTicket", });
wechat.protobuf.Wechat.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy