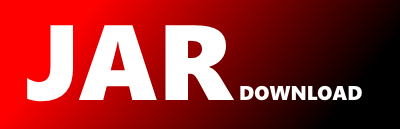
wechat.protobuf.SpamDataBodyMessage Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: SpamDataBodyMessage.proto
package wechat.protobuf;
public final class SpamDataBodyMessage {
private SpamDataBodyMessage() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface FileInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:wechat_proto.FileInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional string filepath = 1;
* @return Whether the filepath field is set.
*/
boolean hasFilepath();
/**
* optional string filepath = 1;
* @return The filepath.
*/
java.lang.String getFilepath();
/**
* optional string filepath = 1;
* @return The bytes for filepath.
*/
com.google.protobuf.ByteString
getFilepathBytes();
/**
* optional string fileuuid = 2;
* @return Whether the fileuuid field is set.
*/
boolean hasFileuuid();
/**
* optional string fileuuid = 2;
* @return The fileuuid.
*/
java.lang.String getFileuuid();
/**
* optional string fileuuid = 2;
* @return The bytes for fileuuid.
*/
com.google.protobuf.ByteString
getFileuuidBytes();
}
/**
* Protobuf type {@code wechat_proto.FileInfo}
*/
public static final class FileInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wechat_proto.FileInfo)
FileInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use FileInfo.newBuilder() to construct.
private FileInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FileInfo() {
filepath_ = "";
fileuuid_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FileInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FileInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
filepath_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
fileuuid_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_FileInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_FileInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.SpamDataBodyMessage.FileInfo.class, wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder.class);
}
private int bitField0_;
public static final int FILEPATH_FIELD_NUMBER = 1;
private volatile java.lang.Object filepath_;
/**
* optional string filepath = 1;
* @return Whether the filepath field is set.
*/
@java.lang.Override
public boolean hasFilepath() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string filepath = 1;
* @return The filepath.
*/
@java.lang.Override
public java.lang.String getFilepath() {
java.lang.Object ref = filepath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
filepath_ = s;
}
return s;
}
}
/**
* optional string filepath = 1;
* @return The bytes for filepath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFilepathBytes() {
java.lang.Object ref = filepath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filepath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILEUUID_FIELD_NUMBER = 2;
private volatile java.lang.Object fileuuid_;
/**
* optional string fileuuid = 2;
* @return Whether the fileuuid field is set.
*/
@java.lang.Override
public boolean hasFileuuid() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string fileuuid = 2;
* @return The fileuuid.
*/
@java.lang.Override
public java.lang.String getFileuuid() {
java.lang.Object ref = fileuuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileuuid_ = s;
}
return s;
}
}
/**
* optional string fileuuid = 2;
* @return The bytes for fileuuid.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFileuuidBytes() {
java.lang.Object ref = fileuuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileuuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, filepath_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, fileuuid_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, filepath_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, fileuuid_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof wechat.protobuf.SpamDataBodyMessage.FileInfo)) {
return super.equals(obj);
}
wechat.protobuf.SpamDataBodyMessage.FileInfo other = (wechat.protobuf.SpamDataBodyMessage.FileInfo) obj;
if (hasFilepath() != other.hasFilepath()) return false;
if (hasFilepath()) {
if (!getFilepath()
.equals(other.getFilepath())) return false;
}
if (hasFileuuid() != other.hasFileuuid()) return false;
if (hasFileuuid()) {
if (!getFileuuid()
.equals(other.getFileuuid())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFilepath()) {
hash = (37 * hash) + FILEPATH_FIELD_NUMBER;
hash = (53 * hash) + getFilepath().hashCode();
}
if (hasFileuuid()) {
hash = (37 * hash) + FILEUUID_FIELD_NUMBER;
hash = (53 * hash) + getFileuuid().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(wechat.protobuf.SpamDataBodyMessage.FileInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wechat_proto.FileInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wechat_proto.FileInfo)
wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_FileInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_FileInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.SpamDataBodyMessage.FileInfo.class, wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder.class);
}
// Construct using wechat.protobuf.SpamDataBodyMessage.FileInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
filepath_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
fileuuid_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_FileInfo_descriptor;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.FileInfo getDefaultInstanceForType() {
return wechat.protobuf.SpamDataBodyMessage.FileInfo.getDefaultInstance();
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.FileInfo build() {
wechat.protobuf.SpamDataBodyMessage.FileInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.FileInfo buildPartial() {
wechat.protobuf.SpamDataBodyMessage.FileInfo result = new wechat.protobuf.SpamDataBodyMessage.FileInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.filepath_ = filepath_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.fileuuid_ = fileuuid_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof wechat.protobuf.SpamDataBodyMessage.FileInfo) {
return mergeFrom((wechat.protobuf.SpamDataBodyMessage.FileInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(wechat.protobuf.SpamDataBodyMessage.FileInfo other) {
if (other == wechat.protobuf.SpamDataBodyMessage.FileInfo.getDefaultInstance()) return this;
if (other.hasFilepath()) {
bitField0_ |= 0x00000001;
filepath_ = other.filepath_;
onChanged();
}
if (other.hasFileuuid()) {
bitField0_ |= 0x00000002;
fileuuid_ = other.fileuuid_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
wechat.protobuf.SpamDataBodyMessage.FileInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (wechat.protobuf.SpamDataBodyMessage.FileInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object filepath_ = "";
/**
* optional string filepath = 1;
* @return Whether the filepath field is set.
*/
public boolean hasFilepath() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string filepath = 1;
* @return The filepath.
*/
public java.lang.String getFilepath() {
java.lang.Object ref = filepath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
filepath_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string filepath = 1;
* @return The bytes for filepath.
*/
public com.google.protobuf.ByteString
getFilepathBytes() {
java.lang.Object ref = filepath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filepath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string filepath = 1;
* @param value The filepath to set.
* @return This builder for chaining.
*/
public Builder setFilepath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
filepath_ = value;
onChanged();
return this;
}
/**
* optional string filepath = 1;
* @return This builder for chaining.
*/
public Builder clearFilepath() {
bitField0_ = (bitField0_ & ~0x00000001);
filepath_ = getDefaultInstance().getFilepath();
onChanged();
return this;
}
/**
* optional string filepath = 1;
* @param value The bytes for filepath to set.
* @return This builder for chaining.
*/
public Builder setFilepathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
filepath_ = value;
onChanged();
return this;
}
private java.lang.Object fileuuid_ = "";
/**
* optional string fileuuid = 2;
* @return Whether the fileuuid field is set.
*/
public boolean hasFileuuid() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string fileuuid = 2;
* @return The fileuuid.
*/
public java.lang.String getFileuuid() {
java.lang.Object ref = fileuuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileuuid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fileuuid = 2;
* @return The bytes for fileuuid.
*/
public com.google.protobuf.ByteString
getFileuuidBytes() {
java.lang.Object ref = fileuuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileuuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fileuuid = 2;
* @param value The fileuuid to set.
* @return This builder for chaining.
*/
public Builder setFileuuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
fileuuid_ = value;
onChanged();
return this;
}
/**
* optional string fileuuid = 2;
* @return This builder for chaining.
*/
public Builder clearFileuuid() {
bitField0_ = (bitField0_ & ~0x00000002);
fileuuid_ = getDefaultInstance().getFileuuid();
onChanged();
return this;
}
/**
* optional string fileuuid = 2;
* @param value The bytes for fileuuid to set.
* @return This builder for chaining.
*/
public Builder setFileuuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
fileuuid_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wechat_proto.FileInfo)
}
// @@protoc_insertion_point(class_scope:wechat_proto.FileInfo)
private static final wechat.protobuf.SpamDataBodyMessage.FileInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new wechat.protobuf.SpamDataBodyMessage.FileInfo();
}
public static wechat.protobuf.SpamDataBodyMessage.FileInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FileInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FileInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.FileInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SpamDataBodyOrBuilder extends
// @@protoc_insertion_point(interface_extends:wechat_proto.SpamDataBody)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 unKnown1 = 1;
* @return Whether the unKnown1 field is set.
*/
boolean hasUnKnown1();
/**
* optional int32 unKnown1 = 1;
* @return The unKnown1.
*/
int getUnKnown1();
/**
* optional int32 timeStamp = 2;
* @return Whether the timeStamp field is set.
*/
boolean hasTimeStamp();
/**
* optional int32 timeStamp = 2;
* @return The timeStamp.
*/
int getTimeStamp();
/**
* optional int32 keyHash = 3;
* @return Whether the keyHash field is set.
*/
boolean hasKeyHash();
/**
* optional int32 keyHash = 3;
* @return The keyHash.
*/
int getKeyHash();
/**
* optional string yes1 = 11;
* @return Whether the yes1 field is set.
*/
boolean hasYes1();
/**
* optional string yes1 = 11;
* @return The yes1.
*/
java.lang.String getYes1();
/**
* optional string yes1 = 11;
* @return The bytes for yes1.
*/
com.google.protobuf.ByteString
getYes1Bytes();
/**
* optional string yes2 = 12;
* @return Whether the yes2 field is set.
*/
boolean hasYes2();
/**
* optional string yes2 = 12;
* @return The yes2.
*/
java.lang.String getYes2();
/**
* optional string yes2 = 12;
* @return The bytes for yes2.
*/
com.google.protobuf.ByteString
getYes2Bytes();
/**
* optional string iosVersion = 13;
* @return Whether the iosVersion field is set.
*/
boolean hasIosVersion();
/**
* optional string iosVersion = 13;
* @return The iosVersion.
*/
java.lang.String getIosVersion();
/**
* optional string iosVersion = 13;
* @return The bytes for iosVersion.
*/
com.google.protobuf.ByteString
getIosVersionBytes();
/**
* optional string deviceType = 14;
* @return Whether the deviceType field is set.
*/
boolean hasDeviceType();
/**
* optional string deviceType = 14;
* @return The deviceType.
*/
java.lang.String getDeviceType();
/**
* optional string deviceType = 14;
* @return The bytes for deviceType.
*/
com.google.protobuf.ByteString
getDeviceTypeBytes();
/**
* optional int32 unKnown2 = 15;
* @return Whether the unKnown2 field is set.
*/
boolean hasUnKnown2();
/**
* optional int32 unKnown2 = 15;
* @return The unKnown2.
*/
int getUnKnown2();
/**
* optional string identifierForVendor = 16;
* @return Whether the identifierForVendor field is set.
*/
boolean hasIdentifierForVendor();
/**
* optional string identifierForVendor = 16;
* @return The identifierForVendor.
*/
java.lang.String getIdentifierForVendor();
/**
* optional string identifierForVendor = 16;
* @return The bytes for identifierForVendor.
*/
com.google.protobuf.ByteString
getIdentifierForVendorBytes();
/**
* optional string advertisingIdentifier = 17;
* @return Whether the advertisingIdentifier field is set.
*/
boolean hasAdvertisingIdentifier();
/**
* optional string advertisingIdentifier = 17;
* @return The advertisingIdentifier.
*/
java.lang.String getAdvertisingIdentifier();
/**
* optional string advertisingIdentifier = 17;
* @return The bytes for advertisingIdentifier.
*/
com.google.protobuf.ByteString
getAdvertisingIdentifierBytes();
/**
* optional string carrier = 18;
* @return Whether the carrier field is set.
*/
boolean hasCarrier();
/**
* optional string carrier = 18;
* @return The carrier.
*/
java.lang.String getCarrier();
/**
* optional string carrier = 18;
* @return The bytes for carrier.
*/
com.google.protobuf.ByteString
getCarrierBytes();
/**
* optional int32 batteryInfo = 19;
* @return Whether the batteryInfo field is set.
*/
boolean hasBatteryInfo();
/**
* optional int32 batteryInfo = 19;
* @return The batteryInfo.
*/
int getBatteryInfo();
/**
* optional string networkName = 20;
* @return Whether the networkName field is set.
*/
boolean hasNetworkName();
/**
* optional string networkName = 20;
* @return The networkName.
*/
java.lang.String getNetworkName();
/**
* optional string networkName = 20;
* @return The bytes for networkName.
*/
com.google.protobuf.ByteString
getNetworkNameBytes();
/**
* optional int32 netType = 21;
* @return Whether the netType field is set.
*/
boolean hasNetType();
/**
* optional int32 netType = 21;
* @return The netType.
*/
int getNetType();
/**
* optional string appBundleId = 22;
* @return Whether the appBundleId field is set.
*/
boolean hasAppBundleId();
/**
* optional string appBundleId = 22;
* @return The appBundleId.
*/
java.lang.String getAppBundleId();
/**
* optional string appBundleId = 22;
* @return The bytes for appBundleId.
*/
com.google.protobuf.ByteString
getAppBundleIdBytes();
/**
* optional string deviceName = 23;
* @return Whether the deviceName field is set.
*/
boolean hasDeviceName();
/**
* optional string deviceName = 23;
* @return The deviceName.
*/
java.lang.String getDeviceName();
/**
* optional string deviceName = 23;
* @return The bytes for deviceName.
*/
com.google.protobuf.ByteString
getDeviceNameBytes();
/**
* optional string userName = 24;
* @return Whether the userName field is set.
*/
boolean hasUserName();
/**
* optional string userName = 24;
* @return The userName.
*/
java.lang.String getUserName();
/**
* optional string userName = 24;
* @return The bytes for userName.
*/
com.google.protobuf.ByteString
getUserNameBytes();
/**
* optional int64 unknown3 = 25;
* @return Whether the unknown3 field is set.
*/
boolean hasUnknown3();
/**
* optional int64 unknown3 = 25;
* @return The unknown3.
*/
long getUnknown3();
/**
* optional int64 unknown4 = 26;
* @return Whether the unknown4 field is set.
*/
boolean hasUnknown4();
/**
* optional int64 unknown4 = 26;
* @return The unknown4.
*/
long getUnknown4();
/**
* optional int32 unknown5 = 27;
* @return Whether the unknown5 field is set.
*/
boolean hasUnknown5();
/**
* optional int32 unknown5 = 27;
* @return The unknown5.
*/
int getUnknown5();
/**
* optional int32 unknown6 = 28;
* @return Whether the unknown6 field is set.
*/
boolean hasUnknown6();
/**
* optional int32 unknown6 = 28;
* @return The unknown6.
*/
int getUnknown6();
/**
* optional string lang = 29;
* @return Whether the lang field is set.
*/
boolean hasLang();
/**
* optional string lang = 29;
* @return The lang.
*/
java.lang.String getLang();
/**
* optional string lang = 29;
* @return The bytes for lang.
*/
com.google.protobuf.ByteString
getLangBytes();
/**
* optional string country = 30;
* @return Whether the country field is set.
*/
boolean hasCountry();
/**
* optional string country = 30;
* @return The country.
*/
java.lang.String getCountry();
/**
* optional string country = 30;
* @return The bytes for country.
*/
com.google.protobuf.ByteString
getCountryBytes();
/**
* optional int32 unknown7 = 31;
* @return Whether the unknown7 field is set.
*/
boolean hasUnknown7();
/**
* optional int32 unknown7 = 31;
* @return The unknown7.
*/
int getUnknown7();
/**
* optional string documentDir = 32;
* @return Whether the documentDir field is set.
*/
boolean hasDocumentDir();
/**
* optional string documentDir = 32;
* @return The documentDir.
*/
java.lang.String getDocumentDir();
/**
* optional string documentDir = 32;
* @return The bytes for documentDir.
*/
com.google.protobuf.ByteString
getDocumentDirBytes();
/**
* optional int32 unknown8 = 33;
* @return Whether the unknown8 field is set.
*/
boolean hasUnknown8();
/**
* optional int32 unknown8 = 33;
* @return The unknown8.
*/
int getUnknown8();
/**
* optional int32 unknown9 = 34;
* @return Whether the unknown9 field is set.
*/
boolean hasUnknown9();
/**
* optional int32 unknown9 = 34;
* @return The unknown9.
*/
int getUnknown9();
/**
* optional string headMD5 = 35;
* @return Whether the headMD5 field is set.
*/
boolean hasHeadMD5();
/**
* optional string headMD5 = 35;
* @return The headMD5.
*/
java.lang.String getHeadMD5();
/**
* optional string headMD5 = 35;
* @return The bytes for headMD5.
*/
com.google.protobuf.ByteString
getHeadMD5Bytes();
/**
* optional string appUUID = 36;
* @return Whether the appUUID field is set.
*/
boolean hasAppUUID();
/**
* optional string appUUID = 36;
* @return The appUUID.
*/
java.lang.String getAppUUID();
/**
* optional string appUUID = 36;
* @return The bytes for appUUID.
*/
com.google.protobuf.ByteString
getAppUUIDBytes();
/**
* optional string syslogUUID = 37;
* @return Whether the syslogUUID field is set.
*/
boolean hasSyslogUUID();
/**
* optional string syslogUUID = 37;
* @return The syslogUUID.
*/
java.lang.String getSyslogUUID();
/**
* optional string syslogUUID = 37;
* @return The bytes for syslogUUID.
*/
com.google.protobuf.ByteString
getSyslogUUIDBytes();
/**
* optional string unknown10 = 38;
* @return Whether the unknown10 field is set.
*/
boolean hasUnknown10();
/**
* optional string unknown10 = 38;
* @return The unknown10.
*/
java.lang.String getUnknown10();
/**
* optional string unknown10 = 38;
* @return The bytes for unknown10.
*/
com.google.protobuf.ByteString
getUnknown10Bytes();
/**
* optional string unknown11 = 39;
* @return Whether the unknown11 field is set.
*/
boolean hasUnknown11();
/**
* optional string unknown11 = 39;
* @return The unknown11.
*/
java.lang.String getUnknown11();
/**
* optional string unknown11 = 39;
* @return The bytes for unknown11.
*/
com.google.protobuf.ByteString
getUnknown11Bytes();
/**
* optional string appName = 40;
* @return Whether the appName field is set.
*/
boolean hasAppName();
/**
* optional string appName = 40;
* @return The appName.
*/
java.lang.String getAppName();
/**
* optional string appName = 40;
* @return The bytes for appName.
*/
com.google.protobuf.ByteString
getAppNameBytes();
/**
* optional string sshPath = 41;
* @return Whether the sshPath field is set.
*/
boolean hasSshPath();
/**
* optional string sshPath = 41;
* @return The sshPath.
*/
java.lang.String getSshPath();
/**
* optional string sshPath = 41;
* @return The bytes for sshPath.
*/
com.google.protobuf.ByteString
getSshPathBytes();
/**
* optional string tempTest = 42;
* @return Whether the tempTest field is set.
*/
boolean hasTempTest();
/**
* optional string tempTest = 42;
* @return The tempTest.
*/
java.lang.String getTempTest();
/**
* optional string tempTest = 42;
* @return The bytes for tempTest.
*/
com.google.protobuf.ByteString
getTempTestBytes();
/**
* optional string devMD5 = 43;
* @return Whether the devMD5 field is set.
*/
boolean hasDevMD5();
/**
* optional string devMD5 = 43;
* @return The devMD5.
*/
java.lang.String getDevMD5();
/**
* optional string devMD5 = 43;
* @return The bytes for devMD5.
*/
com.google.protobuf.ByteString
getDevMD5Bytes();
/**
* optional string devUser = 44;
* @return Whether the devUser field is set.
*/
boolean hasDevUser();
/**
* optional string devUser = 44;
* @return The devUser.
*/
java.lang.String getDevUser();
/**
* optional string devUser = 44;
* @return The bytes for devUser.
*/
com.google.protobuf.ByteString
getDevUserBytes();
/**
* optional string devPrefix = 45;
* @return Whether the devPrefix field is set.
*/
boolean hasDevPrefix();
/**
* optional string devPrefix = 45;
* @return The devPrefix.
*/
java.lang.String getDevPrefix();
/**
* optional string devPrefix = 45;
* @return The bytes for devPrefix.
*/
com.google.protobuf.ByteString
getDevPrefixBytes();
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
java.util.List
getAppFileInfoList();
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
wechat.protobuf.SpamDataBodyMessage.FileInfo getAppFileInfo(int index);
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
int getAppFileInfoCount();
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
java.util.List extends wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder>
getAppFileInfoOrBuilderList();
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder getAppFileInfoOrBuilder(
int index);
/**
* optional string unknown12 = 47;
* @return Whether the unknown12 field is set.
*/
boolean hasUnknown12();
/**
* optional string unknown12 = 47;
* @return The unknown12.
*/
java.lang.String getUnknown12();
/**
* optional string unknown12 = 47;
* @return The bytes for unknown12.
*/
com.google.protobuf.ByteString
getUnknown12Bytes();
/**
* optional int32 isModify = 50;
* @return Whether the isModify field is set.
*/
boolean hasIsModify();
/**
* optional int32 isModify = 50;
* @return The isModify.
*/
int getIsModify();
/**
* optional string modifyMD5 = 51;
* @return Whether the modifyMD5 field is set.
*/
boolean hasModifyMD5();
/**
* optional string modifyMD5 = 51;
* @return The modifyMD5.
*/
java.lang.String getModifyMD5();
/**
* optional string modifyMD5 = 51;
* @return The bytes for modifyMD5.
*/
com.google.protobuf.ByteString
getModifyMD5Bytes();
/**
* optional int64 rqtHash = 52;
* @return Whether the rqtHash field is set.
*/
boolean hasRqtHash();
/**
* optional int64 rqtHash = 52;
* @return The rqtHash.
*/
long getRqtHash();
}
/**
* Protobuf type {@code wechat_proto.SpamDataBody}
*/
public static final class SpamDataBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wechat_proto.SpamDataBody)
SpamDataBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use SpamDataBody.newBuilder() to construct.
private SpamDataBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SpamDataBody() {
yes1_ = "";
yes2_ = "";
iosVersion_ = "";
deviceType_ = "";
identifierForVendor_ = "";
advertisingIdentifier_ = "";
carrier_ = "";
networkName_ = "";
appBundleId_ = "";
deviceName_ = "";
userName_ = "";
lang_ = "";
country_ = "";
documentDir_ = "";
headMD5_ = "";
appUUID_ = "";
syslogUUID_ = "";
unknown10_ = "";
unknown11_ = "";
appName_ = "";
sshPath_ = "";
tempTest_ = "";
devMD5_ = "";
devUser_ = "";
devPrefix_ = "";
appFileInfo_ = java.util.Collections.emptyList();
unknown12_ = "";
modifyMD5_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SpamDataBody();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SpamDataBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
int mutable_bitField1_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
unKnown1_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
timeStamp_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
keyHash_ = input.readInt32();
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
yes1_ = bs;
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
yes2_ = bs;
break;
}
case 106: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
iosVersion_ = bs;
break;
}
case 114: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
deviceType_ = bs;
break;
}
case 120: {
bitField0_ |= 0x00000080;
unKnown2_ = input.readInt32();
break;
}
case 130: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
identifierForVendor_ = bs;
break;
}
case 138: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000200;
advertisingIdentifier_ = bs;
break;
}
case 146: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
carrier_ = bs;
break;
}
case 152: {
bitField0_ |= 0x00000800;
batteryInfo_ = input.readInt32();
break;
}
case 162: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00001000;
networkName_ = bs;
break;
}
case 168: {
bitField0_ |= 0x00002000;
netType_ = input.readInt32();
break;
}
case 178: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00004000;
appBundleId_ = bs;
break;
}
case 186: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00008000;
deviceName_ = bs;
break;
}
case 194: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00010000;
userName_ = bs;
break;
}
case 200: {
bitField0_ |= 0x00020000;
unknown3_ = input.readInt64();
break;
}
case 208: {
bitField0_ |= 0x00040000;
unknown4_ = input.readInt64();
break;
}
case 216: {
bitField0_ |= 0x00080000;
unknown5_ = input.readInt32();
break;
}
case 224: {
bitField0_ |= 0x00100000;
unknown6_ = input.readInt32();
break;
}
case 234: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00200000;
lang_ = bs;
break;
}
case 242: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00400000;
country_ = bs;
break;
}
case 248: {
bitField0_ |= 0x00800000;
unknown7_ = input.readInt32();
break;
}
case 258: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x01000000;
documentDir_ = bs;
break;
}
case 264: {
bitField0_ |= 0x02000000;
unknown8_ = input.readInt32();
break;
}
case 272: {
bitField0_ |= 0x04000000;
unknown9_ = input.readInt32();
break;
}
case 282: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x08000000;
headMD5_ = bs;
break;
}
case 290: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x10000000;
appUUID_ = bs;
break;
}
case 298: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x20000000;
syslogUUID_ = bs;
break;
}
case 306: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x40000000;
unknown10_ = bs;
break;
}
case 314: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x80000000;
unknown11_ = bs;
break;
}
case 322: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000001;
appName_ = bs;
break;
}
case 330: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000002;
sshPath_ = bs;
break;
}
case 338: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000004;
tempTest_ = bs;
break;
}
case 346: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000008;
devMD5_ = bs;
break;
}
case 354: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000010;
devUser_ = bs;
break;
}
case 362: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000020;
devPrefix_ = bs;
break;
}
case 370: {
if (!((mutable_bitField1_ & 0x00000040) != 0)) {
appFileInfo_ = new java.util.ArrayList();
mutable_bitField1_ |= 0x00000040;
}
appFileInfo_.add(
input.readMessage(wechat.protobuf.SpamDataBodyMessage.FileInfo.PARSER, extensionRegistry));
break;
}
case 378: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000040;
unknown12_ = bs;
break;
}
case 400: {
bitField1_ |= 0x00000080;
isModify_ = input.readInt32();
break;
}
case 410: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField1_ |= 0x00000100;
modifyMD5_ = bs;
break;
}
case 416: {
bitField1_ |= 0x00000200;
rqtHash_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField1_ & 0x00000040) != 0)) {
appFileInfo_ = java.util.Collections.unmodifiableList(appFileInfo_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_SpamDataBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_SpamDataBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.SpamDataBodyMessage.SpamDataBody.class, wechat.protobuf.SpamDataBodyMessage.SpamDataBody.Builder.class);
}
private int bitField0_;
private int bitField1_;
public static final int UNKNOWN1_FIELD_NUMBER = 1;
private int unKnown1_;
/**
* optional int32 unKnown1 = 1;
* @return Whether the unKnown1 field is set.
*/
@java.lang.Override
public boolean hasUnKnown1() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 unKnown1 = 1;
* @return The unKnown1.
*/
@java.lang.Override
public int getUnKnown1() {
return unKnown1_;
}
public static final int TIMESTAMP_FIELD_NUMBER = 2;
private int timeStamp_;
/**
* optional int32 timeStamp = 2;
* @return Whether the timeStamp field is set.
*/
@java.lang.Override
public boolean hasTimeStamp() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 timeStamp = 2;
* @return The timeStamp.
*/
@java.lang.Override
public int getTimeStamp() {
return timeStamp_;
}
public static final int KEYHASH_FIELD_NUMBER = 3;
private int keyHash_;
/**
* optional int32 keyHash = 3;
* @return Whether the keyHash field is set.
*/
@java.lang.Override
public boolean hasKeyHash() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int32 keyHash = 3;
* @return The keyHash.
*/
@java.lang.Override
public int getKeyHash() {
return keyHash_;
}
public static final int YES1_FIELD_NUMBER = 11;
private volatile java.lang.Object yes1_;
/**
* optional string yes1 = 11;
* @return Whether the yes1 field is set.
*/
@java.lang.Override
public boolean hasYes1() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string yes1 = 11;
* @return The yes1.
*/
@java.lang.Override
public java.lang.String getYes1() {
java.lang.Object ref = yes1_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
yes1_ = s;
}
return s;
}
}
/**
* optional string yes1 = 11;
* @return The bytes for yes1.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getYes1Bytes() {
java.lang.Object ref = yes1_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
yes1_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int YES2_FIELD_NUMBER = 12;
private volatile java.lang.Object yes2_;
/**
* optional string yes2 = 12;
* @return Whether the yes2 field is set.
*/
@java.lang.Override
public boolean hasYes2() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string yes2 = 12;
* @return The yes2.
*/
@java.lang.Override
public java.lang.String getYes2() {
java.lang.Object ref = yes2_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
yes2_ = s;
}
return s;
}
}
/**
* optional string yes2 = 12;
* @return The bytes for yes2.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getYes2Bytes() {
java.lang.Object ref = yes2_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
yes2_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IOSVERSION_FIELD_NUMBER = 13;
private volatile java.lang.Object iosVersion_;
/**
* optional string iosVersion = 13;
* @return Whether the iosVersion field is set.
*/
@java.lang.Override
public boolean hasIosVersion() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string iosVersion = 13;
* @return The iosVersion.
*/
@java.lang.Override
public java.lang.String getIosVersion() {
java.lang.Object ref = iosVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
iosVersion_ = s;
}
return s;
}
}
/**
* optional string iosVersion = 13;
* @return The bytes for iosVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIosVersionBytes() {
java.lang.Object ref = iosVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
iosVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVICETYPE_FIELD_NUMBER = 14;
private volatile java.lang.Object deviceType_;
/**
* optional string deviceType = 14;
* @return Whether the deviceType field is set.
*/
@java.lang.Override
public boolean hasDeviceType() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional string deviceType = 14;
* @return The deviceType.
*/
@java.lang.Override
public java.lang.String getDeviceType() {
java.lang.Object ref = deviceType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceType_ = s;
}
return s;
}
}
/**
* optional string deviceType = 14;
* @return The bytes for deviceType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDeviceTypeBytes() {
java.lang.Object ref = deviceType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNKNOWN2_FIELD_NUMBER = 15;
private int unKnown2_;
/**
* optional int32 unKnown2 = 15;
* @return Whether the unKnown2 field is set.
*/
@java.lang.Override
public boolean hasUnKnown2() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional int32 unKnown2 = 15;
* @return The unKnown2.
*/
@java.lang.Override
public int getUnKnown2() {
return unKnown2_;
}
public static final int IDENTIFIERFORVENDOR_FIELD_NUMBER = 16;
private volatile java.lang.Object identifierForVendor_;
/**
* optional string identifierForVendor = 16;
* @return Whether the identifierForVendor field is set.
*/
@java.lang.Override
public boolean hasIdentifierForVendor() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional string identifierForVendor = 16;
* @return The identifierForVendor.
*/
@java.lang.Override
public java.lang.String getIdentifierForVendor() {
java.lang.Object ref = identifierForVendor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
identifierForVendor_ = s;
}
return s;
}
}
/**
* optional string identifierForVendor = 16;
* @return The bytes for identifierForVendor.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdentifierForVendorBytes() {
java.lang.Object ref = identifierForVendor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identifierForVendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADVERTISINGIDENTIFIER_FIELD_NUMBER = 17;
private volatile java.lang.Object advertisingIdentifier_;
/**
* optional string advertisingIdentifier = 17;
* @return Whether the advertisingIdentifier field is set.
*/
@java.lang.Override
public boolean hasAdvertisingIdentifier() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional string advertisingIdentifier = 17;
* @return The advertisingIdentifier.
*/
@java.lang.Override
public java.lang.String getAdvertisingIdentifier() {
java.lang.Object ref = advertisingIdentifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
advertisingIdentifier_ = s;
}
return s;
}
}
/**
* optional string advertisingIdentifier = 17;
* @return The bytes for advertisingIdentifier.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdvertisingIdentifierBytes() {
java.lang.Object ref = advertisingIdentifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
advertisingIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CARRIER_FIELD_NUMBER = 18;
private volatile java.lang.Object carrier_;
/**
* optional string carrier = 18;
* @return Whether the carrier field is set.
*/
@java.lang.Override
public boolean hasCarrier() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional string carrier = 18;
* @return The carrier.
*/
@java.lang.Override
public java.lang.String getCarrier() {
java.lang.Object ref = carrier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
carrier_ = s;
}
return s;
}
}
/**
* optional string carrier = 18;
* @return The bytes for carrier.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCarrierBytes() {
java.lang.Object ref = carrier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
carrier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BATTERYINFO_FIELD_NUMBER = 19;
private int batteryInfo_;
/**
* optional int32 batteryInfo = 19;
* @return Whether the batteryInfo field is set.
*/
@java.lang.Override
public boolean hasBatteryInfo() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
* optional int32 batteryInfo = 19;
* @return The batteryInfo.
*/
@java.lang.Override
public int getBatteryInfo() {
return batteryInfo_;
}
public static final int NETWORKNAME_FIELD_NUMBER = 20;
private volatile java.lang.Object networkName_;
/**
* optional string networkName = 20;
* @return Whether the networkName field is set.
*/
@java.lang.Override
public boolean hasNetworkName() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
* optional string networkName = 20;
* @return The networkName.
*/
@java.lang.Override
public java.lang.String getNetworkName() {
java.lang.Object ref = networkName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
networkName_ = s;
}
return s;
}
}
/**
* optional string networkName = 20;
* @return The bytes for networkName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNetworkNameBytes() {
java.lang.Object ref = networkName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
networkName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETTYPE_FIELD_NUMBER = 21;
private int netType_;
/**
* optional int32 netType = 21;
* @return Whether the netType field is set.
*/
@java.lang.Override
public boolean hasNetType() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
* optional int32 netType = 21;
* @return The netType.
*/
@java.lang.Override
public int getNetType() {
return netType_;
}
public static final int APPBUNDLEID_FIELD_NUMBER = 22;
private volatile java.lang.Object appBundleId_;
/**
* optional string appBundleId = 22;
* @return Whether the appBundleId field is set.
*/
@java.lang.Override
public boolean hasAppBundleId() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
* optional string appBundleId = 22;
* @return The appBundleId.
*/
@java.lang.Override
public java.lang.String getAppBundleId() {
java.lang.Object ref = appBundleId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appBundleId_ = s;
}
return s;
}
}
/**
* optional string appBundleId = 22;
* @return The bytes for appBundleId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAppBundleIdBytes() {
java.lang.Object ref = appBundleId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appBundleId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVICENAME_FIELD_NUMBER = 23;
private volatile java.lang.Object deviceName_;
/**
* optional string deviceName = 23;
* @return Whether the deviceName field is set.
*/
@java.lang.Override
public boolean hasDeviceName() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
* optional string deviceName = 23;
* @return The deviceName.
*/
@java.lang.Override
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceName_ = s;
}
return s;
}
}
/**
* optional string deviceName = 23;
* @return The bytes for deviceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USERNAME_FIELD_NUMBER = 24;
private volatile java.lang.Object userName_;
/**
* optional string userName = 24;
* @return Whether the userName field is set.
*/
@java.lang.Override
public boolean hasUserName() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
* optional string userName = 24;
* @return The userName.
*/
@java.lang.Override
public java.lang.String getUserName() {
java.lang.Object ref = userName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userName_ = s;
}
return s;
}
}
/**
* optional string userName = 24;
* @return The bytes for userName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserNameBytes() {
java.lang.Object ref = userName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNKNOWN3_FIELD_NUMBER = 25;
private long unknown3_;
/**
* optional int64 unknown3 = 25;
* @return Whether the unknown3 field is set.
*/
@java.lang.Override
public boolean hasUnknown3() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
* optional int64 unknown3 = 25;
* @return The unknown3.
*/
@java.lang.Override
public long getUnknown3() {
return unknown3_;
}
public static final int UNKNOWN4_FIELD_NUMBER = 26;
private long unknown4_;
/**
* optional int64 unknown4 = 26;
* @return Whether the unknown4 field is set.
*/
@java.lang.Override
public boolean hasUnknown4() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
* optional int64 unknown4 = 26;
* @return The unknown4.
*/
@java.lang.Override
public long getUnknown4() {
return unknown4_;
}
public static final int UNKNOWN5_FIELD_NUMBER = 27;
private int unknown5_;
/**
* optional int32 unknown5 = 27;
* @return Whether the unknown5 field is set.
*/
@java.lang.Override
public boolean hasUnknown5() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
* optional int32 unknown5 = 27;
* @return The unknown5.
*/
@java.lang.Override
public int getUnknown5() {
return unknown5_;
}
public static final int UNKNOWN6_FIELD_NUMBER = 28;
private int unknown6_;
/**
* optional int32 unknown6 = 28;
* @return Whether the unknown6 field is set.
*/
@java.lang.Override
public boolean hasUnknown6() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
* optional int32 unknown6 = 28;
* @return The unknown6.
*/
@java.lang.Override
public int getUnknown6() {
return unknown6_;
}
public static final int LANG_FIELD_NUMBER = 29;
private volatile java.lang.Object lang_;
/**
* optional string lang = 29;
* @return Whether the lang field is set.
*/
@java.lang.Override
public boolean hasLang() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
* optional string lang = 29;
* @return The lang.
*/
@java.lang.Override
public java.lang.String getLang() {
java.lang.Object ref = lang_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
lang_ = s;
}
return s;
}
}
/**
* optional string lang = 29;
* @return The bytes for lang.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLangBytes() {
java.lang.Object ref = lang_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lang_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COUNTRY_FIELD_NUMBER = 30;
private volatile java.lang.Object country_;
/**
* optional string country = 30;
* @return Whether the country field is set.
*/
@java.lang.Override
public boolean hasCountry() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
* optional string country = 30;
* @return The country.
*/
@java.lang.Override
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
}
}
/**
* optional string country = 30;
* @return The bytes for country.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNKNOWN7_FIELD_NUMBER = 31;
private int unknown7_;
/**
* optional int32 unknown7 = 31;
* @return Whether the unknown7 field is set.
*/
@java.lang.Override
public boolean hasUnknown7() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
* optional int32 unknown7 = 31;
* @return The unknown7.
*/
@java.lang.Override
public int getUnknown7() {
return unknown7_;
}
public static final int DOCUMENTDIR_FIELD_NUMBER = 32;
private volatile java.lang.Object documentDir_;
/**
* optional string documentDir = 32;
* @return Whether the documentDir field is set.
*/
@java.lang.Override
public boolean hasDocumentDir() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
* optional string documentDir = 32;
* @return The documentDir.
*/
@java.lang.Override
public java.lang.String getDocumentDir() {
java.lang.Object ref = documentDir_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
documentDir_ = s;
}
return s;
}
}
/**
* optional string documentDir = 32;
* @return The bytes for documentDir.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDocumentDirBytes() {
java.lang.Object ref = documentDir_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
documentDir_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNKNOWN8_FIELD_NUMBER = 33;
private int unknown8_;
/**
* optional int32 unknown8 = 33;
* @return Whether the unknown8 field is set.
*/
@java.lang.Override
public boolean hasUnknown8() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
* optional int32 unknown8 = 33;
* @return The unknown8.
*/
@java.lang.Override
public int getUnknown8() {
return unknown8_;
}
public static final int UNKNOWN9_FIELD_NUMBER = 34;
private int unknown9_;
/**
* optional int32 unknown9 = 34;
* @return Whether the unknown9 field is set.
*/
@java.lang.Override
public boolean hasUnknown9() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
* optional int32 unknown9 = 34;
* @return The unknown9.
*/
@java.lang.Override
public int getUnknown9() {
return unknown9_;
}
public static final int HEADMD5_FIELD_NUMBER = 35;
private volatile java.lang.Object headMD5_;
/**
* optional string headMD5 = 35;
* @return Whether the headMD5 field is set.
*/
@java.lang.Override
public boolean hasHeadMD5() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
* optional string headMD5 = 35;
* @return The headMD5.
*/
@java.lang.Override
public java.lang.String getHeadMD5() {
java.lang.Object ref = headMD5_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
headMD5_ = s;
}
return s;
}
}
/**
* optional string headMD5 = 35;
* @return The bytes for headMD5.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHeadMD5Bytes() {
java.lang.Object ref = headMD5_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
headMD5_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPUUID_FIELD_NUMBER = 36;
private volatile java.lang.Object appUUID_;
/**
* optional string appUUID = 36;
* @return Whether the appUUID field is set.
*/
@java.lang.Override
public boolean hasAppUUID() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
* optional string appUUID = 36;
* @return The appUUID.
*/
@java.lang.Override
public java.lang.String getAppUUID() {
java.lang.Object ref = appUUID_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appUUID_ = s;
}
return s;
}
}
/**
* optional string appUUID = 36;
* @return The bytes for appUUID.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAppUUIDBytes() {
java.lang.Object ref = appUUID_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appUUID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SYSLOGUUID_FIELD_NUMBER = 37;
private volatile java.lang.Object syslogUUID_;
/**
* optional string syslogUUID = 37;
* @return Whether the syslogUUID field is set.
*/
@java.lang.Override
public boolean hasSyslogUUID() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
* optional string syslogUUID = 37;
* @return The syslogUUID.
*/
@java.lang.Override
public java.lang.String getSyslogUUID() {
java.lang.Object ref = syslogUUID_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
syslogUUID_ = s;
}
return s;
}
}
/**
* optional string syslogUUID = 37;
* @return The bytes for syslogUUID.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSyslogUUIDBytes() {
java.lang.Object ref = syslogUUID_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
syslogUUID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNKNOWN10_FIELD_NUMBER = 38;
private volatile java.lang.Object unknown10_;
/**
* optional string unknown10 = 38;
* @return Whether the unknown10 field is set.
*/
@java.lang.Override
public boolean hasUnknown10() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
* optional string unknown10 = 38;
* @return The unknown10.
*/
@java.lang.Override
public java.lang.String getUnknown10() {
java.lang.Object ref = unknown10_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unknown10_ = s;
}
return s;
}
}
/**
* optional string unknown10 = 38;
* @return The bytes for unknown10.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUnknown10Bytes() {
java.lang.Object ref = unknown10_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unknown10_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNKNOWN11_FIELD_NUMBER = 39;
private volatile java.lang.Object unknown11_;
/**
* optional string unknown11 = 39;
* @return Whether the unknown11 field is set.
*/
@java.lang.Override
public boolean hasUnknown11() {
return ((bitField0_ & 0x80000000) != 0);
}
/**
* optional string unknown11 = 39;
* @return The unknown11.
*/
@java.lang.Override
public java.lang.String getUnknown11() {
java.lang.Object ref = unknown11_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unknown11_ = s;
}
return s;
}
}
/**
* optional string unknown11 = 39;
* @return The bytes for unknown11.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUnknown11Bytes() {
java.lang.Object ref = unknown11_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unknown11_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPNAME_FIELD_NUMBER = 40;
private volatile java.lang.Object appName_;
/**
* optional string appName = 40;
* @return Whether the appName field is set.
*/
@java.lang.Override
public boolean hasAppName() {
return ((bitField1_ & 0x00000001) != 0);
}
/**
* optional string appName = 40;
* @return The appName.
*/
@java.lang.Override
public java.lang.String getAppName() {
java.lang.Object ref = appName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appName_ = s;
}
return s;
}
}
/**
* optional string appName = 40;
* @return The bytes for appName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAppNameBytes() {
java.lang.Object ref = appName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SSHPATH_FIELD_NUMBER = 41;
private volatile java.lang.Object sshPath_;
/**
* optional string sshPath = 41;
* @return Whether the sshPath field is set.
*/
@java.lang.Override
public boolean hasSshPath() {
return ((bitField1_ & 0x00000002) != 0);
}
/**
* optional string sshPath = 41;
* @return The sshPath.
*/
@java.lang.Override
public java.lang.String getSshPath() {
java.lang.Object ref = sshPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sshPath_ = s;
}
return s;
}
}
/**
* optional string sshPath = 41;
* @return The bytes for sshPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSshPathBytes() {
java.lang.Object ref = sshPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sshPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TEMPTEST_FIELD_NUMBER = 42;
private volatile java.lang.Object tempTest_;
/**
* optional string tempTest = 42;
* @return Whether the tempTest field is set.
*/
@java.lang.Override
public boolean hasTempTest() {
return ((bitField1_ & 0x00000004) != 0);
}
/**
* optional string tempTest = 42;
* @return The tempTest.
*/
@java.lang.Override
public java.lang.String getTempTest() {
java.lang.Object ref = tempTest_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tempTest_ = s;
}
return s;
}
}
/**
* optional string tempTest = 42;
* @return The bytes for tempTest.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTempTestBytes() {
java.lang.Object ref = tempTest_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tempTest_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVMD5_FIELD_NUMBER = 43;
private volatile java.lang.Object devMD5_;
/**
* optional string devMD5 = 43;
* @return Whether the devMD5 field is set.
*/
@java.lang.Override
public boolean hasDevMD5() {
return ((bitField1_ & 0x00000008) != 0);
}
/**
* optional string devMD5 = 43;
* @return The devMD5.
*/
@java.lang.Override
public java.lang.String getDevMD5() {
java.lang.Object ref = devMD5_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
devMD5_ = s;
}
return s;
}
}
/**
* optional string devMD5 = 43;
* @return The bytes for devMD5.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDevMD5Bytes() {
java.lang.Object ref = devMD5_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
devMD5_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVUSER_FIELD_NUMBER = 44;
private volatile java.lang.Object devUser_;
/**
* optional string devUser = 44;
* @return Whether the devUser field is set.
*/
@java.lang.Override
public boolean hasDevUser() {
return ((bitField1_ & 0x00000010) != 0);
}
/**
* optional string devUser = 44;
* @return The devUser.
*/
@java.lang.Override
public java.lang.String getDevUser() {
java.lang.Object ref = devUser_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
devUser_ = s;
}
return s;
}
}
/**
* optional string devUser = 44;
* @return The bytes for devUser.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDevUserBytes() {
java.lang.Object ref = devUser_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
devUser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVPREFIX_FIELD_NUMBER = 45;
private volatile java.lang.Object devPrefix_;
/**
* optional string devPrefix = 45;
* @return Whether the devPrefix field is set.
*/
@java.lang.Override
public boolean hasDevPrefix() {
return ((bitField1_ & 0x00000020) != 0);
}
/**
* optional string devPrefix = 45;
* @return The devPrefix.
*/
@java.lang.Override
public java.lang.String getDevPrefix() {
java.lang.Object ref = devPrefix_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
devPrefix_ = s;
}
return s;
}
}
/**
* optional string devPrefix = 45;
* @return The bytes for devPrefix.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDevPrefixBytes() {
java.lang.Object ref = devPrefix_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
devPrefix_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPFILEINFO_FIELD_NUMBER = 46;
private java.util.List appFileInfo_;
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
@java.lang.Override
public java.util.List getAppFileInfoList() {
return appFileInfo_;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
@java.lang.Override
public java.util.List extends wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder>
getAppFileInfoOrBuilderList() {
return appFileInfo_;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
@java.lang.Override
public int getAppFileInfoCount() {
return appFileInfo_.size();
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.FileInfo getAppFileInfo(int index) {
return appFileInfo_.get(index);
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder getAppFileInfoOrBuilder(
int index) {
return appFileInfo_.get(index);
}
public static final int UNKNOWN12_FIELD_NUMBER = 47;
private volatile java.lang.Object unknown12_;
/**
* optional string unknown12 = 47;
* @return Whether the unknown12 field is set.
*/
@java.lang.Override
public boolean hasUnknown12() {
return ((bitField1_ & 0x00000040) != 0);
}
/**
* optional string unknown12 = 47;
* @return The unknown12.
*/
@java.lang.Override
public java.lang.String getUnknown12() {
java.lang.Object ref = unknown12_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unknown12_ = s;
}
return s;
}
}
/**
* optional string unknown12 = 47;
* @return The bytes for unknown12.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUnknown12Bytes() {
java.lang.Object ref = unknown12_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unknown12_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ISMODIFY_FIELD_NUMBER = 50;
private int isModify_;
/**
* optional int32 isModify = 50;
* @return Whether the isModify field is set.
*/
@java.lang.Override
public boolean hasIsModify() {
return ((bitField1_ & 0x00000080) != 0);
}
/**
* optional int32 isModify = 50;
* @return The isModify.
*/
@java.lang.Override
public int getIsModify() {
return isModify_;
}
public static final int MODIFYMD5_FIELD_NUMBER = 51;
private volatile java.lang.Object modifyMD5_;
/**
* optional string modifyMD5 = 51;
* @return Whether the modifyMD5 field is set.
*/
@java.lang.Override
public boolean hasModifyMD5() {
return ((bitField1_ & 0x00000100) != 0);
}
/**
* optional string modifyMD5 = 51;
* @return The modifyMD5.
*/
@java.lang.Override
public java.lang.String getModifyMD5() {
java.lang.Object ref = modifyMD5_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
modifyMD5_ = s;
}
return s;
}
}
/**
* optional string modifyMD5 = 51;
* @return The bytes for modifyMD5.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getModifyMD5Bytes() {
java.lang.Object ref = modifyMD5_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
modifyMD5_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RQTHASH_FIELD_NUMBER = 52;
private long rqtHash_;
/**
* optional int64 rqtHash = 52;
* @return Whether the rqtHash field is set.
*/
@java.lang.Override
public boolean hasRqtHash() {
return ((bitField1_ & 0x00000200) != 0);
}
/**
* optional int64 rqtHash = 52;
* @return The rqtHash.
*/
@java.lang.Override
public long getRqtHash() {
return rqtHash_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, unKnown1_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, timeStamp_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(3, keyHash_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, yes1_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, yes2_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, iosVersion_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, deviceType_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt32(15, unKnown2_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, identifierForVendor_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, advertisingIdentifier_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, carrier_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeInt32(19, batteryInfo_);
}
if (((bitField0_ & 0x00001000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, networkName_);
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeInt32(21, netType_);
}
if (((bitField0_ & 0x00004000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 22, appBundleId_);
}
if (((bitField0_ & 0x00008000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 23, deviceName_);
}
if (((bitField0_ & 0x00010000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 24, userName_);
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeInt64(25, unknown3_);
}
if (((bitField0_ & 0x00040000) != 0)) {
output.writeInt64(26, unknown4_);
}
if (((bitField0_ & 0x00080000) != 0)) {
output.writeInt32(27, unknown5_);
}
if (((bitField0_ & 0x00100000) != 0)) {
output.writeInt32(28, unknown6_);
}
if (((bitField0_ & 0x00200000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, lang_);
}
if (((bitField0_ & 0x00400000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, country_);
}
if (((bitField0_ & 0x00800000) != 0)) {
output.writeInt32(31, unknown7_);
}
if (((bitField0_ & 0x01000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 32, documentDir_);
}
if (((bitField0_ & 0x02000000) != 0)) {
output.writeInt32(33, unknown8_);
}
if (((bitField0_ & 0x04000000) != 0)) {
output.writeInt32(34, unknown9_);
}
if (((bitField0_ & 0x08000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 35, headMD5_);
}
if (((bitField0_ & 0x10000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 36, appUUID_);
}
if (((bitField0_ & 0x20000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 37, syslogUUID_);
}
if (((bitField0_ & 0x40000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 38, unknown10_);
}
if (((bitField0_ & 0x80000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 39, unknown11_);
}
if (((bitField1_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 40, appName_);
}
if (((bitField1_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 41, sshPath_);
}
if (((bitField1_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 42, tempTest_);
}
if (((bitField1_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 43, devMD5_);
}
if (((bitField1_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 44, devUser_);
}
if (((bitField1_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 45, devPrefix_);
}
for (int i = 0; i < appFileInfo_.size(); i++) {
output.writeMessage(46, appFileInfo_.get(i));
}
if (((bitField1_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 47, unknown12_);
}
if (((bitField1_ & 0x00000080) != 0)) {
output.writeInt32(50, isModify_);
}
if (((bitField1_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 51, modifyMD5_);
}
if (((bitField1_ & 0x00000200) != 0)) {
output.writeInt64(52, rqtHash_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, unKnown1_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, timeStamp_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, keyHash_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, yes1_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, yes2_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, iosVersion_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, deviceType_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(15, unKnown2_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, identifierForVendor_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, advertisingIdentifier_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, carrier_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(19, batteryInfo_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, networkName_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(21, netType_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(22, appBundleId_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(23, deviceName_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(24, userName_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(25, unknown3_);
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(26, unknown4_);
}
if (((bitField0_ & 0x00080000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(27, unknown5_);
}
if (((bitField0_ & 0x00100000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(28, unknown6_);
}
if (((bitField0_ & 0x00200000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(29, lang_);
}
if (((bitField0_ & 0x00400000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, country_);
}
if (((bitField0_ & 0x00800000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(31, unknown7_);
}
if (((bitField0_ & 0x01000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(32, documentDir_);
}
if (((bitField0_ & 0x02000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(33, unknown8_);
}
if (((bitField0_ & 0x04000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(34, unknown9_);
}
if (((bitField0_ & 0x08000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(35, headMD5_);
}
if (((bitField0_ & 0x10000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(36, appUUID_);
}
if (((bitField0_ & 0x20000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(37, syslogUUID_);
}
if (((bitField0_ & 0x40000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(38, unknown10_);
}
if (((bitField0_ & 0x80000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(39, unknown11_);
}
if (((bitField1_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(40, appName_);
}
if (((bitField1_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(41, sshPath_);
}
if (((bitField1_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(42, tempTest_);
}
if (((bitField1_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(43, devMD5_);
}
if (((bitField1_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(44, devUser_);
}
if (((bitField1_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(45, devPrefix_);
}
for (int i = 0; i < appFileInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(46, appFileInfo_.get(i));
}
if (((bitField1_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(47, unknown12_);
}
if (((bitField1_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(50, isModify_);
}
if (((bitField1_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(51, modifyMD5_);
}
if (((bitField1_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(52, rqtHash_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof wechat.protobuf.SpamDataBodyMessage.SpamDataBody)) {
return super.equals(obj);
}
wechat.protobuf.SpamDataBodyMessage.SpamDataBody other = (wechat.protobuf.SpamDataBodyMessage.SpamDataBody) obj;
if (hasUnKnown1() != other.hasUnKnown1()) return false;
if (hasUnKnown1()) {
if (getUnKnown1()
!= other.getUnKnown1()) return false;
}
if (hasTimeStamp() != other.hasTimeStamp()) return false;
if (hasTimeStamp()) {
if (getTimeStamp()
!= other.getTimeStamp()) return false;
}
if (hasKeyHash() != other.hasKeyHash()) return false;
if (hasKeyHash()) {
if (getKeyHash()
!= other.getKeyHash()) return false;
}
if (hasYes1() != other.hasYes1()) return false;
if (hasYes1()) {
if (!getYes1()
.equals(other.getYes1())) return false;
}
if (hasYes2() != other.hasYes2()) return false;
if (hasYes2()) {
if (!getYes2()
.equals(other.getYes2())) return false;
}
if (hasIosVersion() != other.hasIosVersion()) return false;
if (hasIosVersion()) {
if (!getIosVersion()
.equals(other.getIosVersion())) return false;
}
if (hasDeviceType() != other.hasDeviceType()) return false;
if (hasDeviceType()) {
if (!getDeviceType()
.equals(other.getDeviceType())) return false;
}
if (hasUnKnown2() != other.hasUnKnown2()) return false;
if (hasUnKnown2()) {
if (getUnKnown2()
!= other.getUnKnown2()) return false;
}
if (hasIdentifierForVendor() != other.hasIdentifierForVendor()) return false;
if (hasIdentifierForVendor()) {
if (!getIdentifierForVendor()
.equals(other.getIdentifierForVendor())) return false;
}
if (hasAdvertisingIdentifier() != other.hasAdvertisingIdentifier()) return false;
if (hasAdvertisingIdentifier()) {
if (!getAdvertisingIdentifier()
.equals(other.getAdvertisingIdentifier())) return false;
}
if (hasCarrier() != other.hasCarrier()) return false;
if (hasCarrier()) {
if (!getCarrier()
.equals(other.getCarrier())) return false;
}
if (hasBatteryInfo() != other.hasBatteryInfo()) return false;
if (hasBatteryInfo()) {
if (getBatteryInfo()
!= other.getBatteryInfo()) return false;
}
if (hasNetworkName() != other.hasNetworkName()) return false;
if (hasNetworkName()) {
if (!getNetworkName()
.equals(other.getNetworkName())) return false;
}
if (hasNetType() != other.hasNetType()) return false;
if (hasNetType()) {
if (getNetType()
!= other.getNetType()) return false;
}
if (hasAppBundleId() != other.hasAppBundleId()) return false;
if (hasAppBundleId()) {
if (!getAppBundleId()
.equals(other.getAppBundleId())) return false;
}
if (hasDeviceName() != other.hasDeviceName()) return false;
if (hasDeviceName()) {
if (!getDeviceName()
.equals(other.getDeviceName())) return false;
}
if (hasUserName() != other.hasUserName()) return false;
if (hasUserName()) {
if (!getUserName()
.equals(other.getUserName())) return false;
}
if (hasUnknown3() != other.hasUnknown3()) return false;
if (hasUnknown3()) {
if (getUnknown3()
!= other.getUnknown3()) return false;
}
if (hasUnknown4() != other.hasUnknown4()) return false;
if (hasUnknown4()) {
if (getUnknown4()
!= other.getUnknown4()) return false;
}
if (hasUnknown5() != other.hasUnknown5()) return false;
if (hasUnknown5()) {
if (getUnknown5()
!= other.getUnknown5()) return false;
}
if (hasUnknown6() != other.hasUnknown6()) return false;
if (hasUnknown6()) {
if (getUnknown6()
!= other.getUnknown6()) return false;
}
if (hasLang() != other.hasLang()) return false;
if (hasLang()) {
if (!getLang()
.equals(other.getLang())) return false;
}
if (hasCountry() != other.hasCountry()) return false;
if (hasCountry()) {
if (!getCountry()
.equals(other.getCountry())) return false;
}
if (hasUnknown7() != other.hasUnknown7()) return false;
if (hasUnknown7()) {
if (getUnknown7()
!= other.getUnknown7()) return false;
}
if (hasDocumentDir() != other.hasDocumentDir()) return false;
if (hasDocumentDir()) {
if (!getDocumentDir()
.equals(other.getDocumentDir())) return false;
}
if (hasUnknown8() != other.hasUnknown8()) return false;
if (hasUnknown8()) {
if (getUnknown8()
!= other.getUnknown8()) return false;
}
if (hasUnknown9() != other.hasUnknown9()) return false;
if (hasUnknown9()) {
if (getUnknown9()
!= other.getUnknown9()) return false;
}
if (hasHeadMD5() != other.hasHeadMD5()) return false;
if (hasHeadMD5()) {
if (!getHeadMD5()
.equals(other.getHeadMD5())) return false;
}
if (hasAppUUID() != other.hasAppUUID()) return false;
if (hasAppUUID()) {
if (!getAppUUID()
.equals(other.getAppUUID())) return false;
}
if (hasSyslogUUID() != other.hasSyslogUUID()) return false;
if (hasSyslogUUID()) {
if (!getSyslogUUID()
.equals(other.getSyslogUUID())) return false;
}
if (hasUnknown10() != other.hasUnknown10()) return false;
if (hasUnknown10()) {
if (!getUnknown10()
.equals(other.getUnknown10())) return false;
}
if (hasUnknown11() != other.hasUnknown11()) return false;
if (hasUnknown11()) {
if (!getUnknown11()
.equals(other.getUnknown11())) return false;
}
if (hasAppName() != other.hasAppName()) return false;
if (hasAppName()) {
if (!getAppName()
.equals(other.getAppName())) return false;
}
if (hasSshPath() != other.hasSshPath()) return false;
if (hasSshPath()) {
if (!getSshPath()
.equals(other.getSshPath())) return false;
}
if (hasTempTest() != other.hasTempTest()) return false;
if (hasTempTest()) {
if (!getTempTest()
.equals(other.getTempTest())) return false;
}
if (hasDevMD5() != other.hasDevMD5()) return false;
if (hasDevMD5()) {
if (!getDevMD5()
.equals(other.getDevMD5())) return false;
}
if (hasDevUser() != other.hasDevUser()) return false;
if (hasDevUser()) {
if (!getDevUser()
.equals(other.getDevUser())) return false;
}
if (hasDevPrefix() != other.hasDevPrefix()) return false;
if (hasDevPrefix()) {
if (!getDevPrefix()
.equals(other.getDevPrefix())) return false;
}
if (!getAppFileInfoList()
.equals(other.getAppFileInfoList())) return false;
if (hasUnknown12() != other.hasUnknown12()) return false;
if (hasUnknown12()) {
if (!getUnknown12()
.equals(other.getUnknown12())) return false;
}
if (hasIsModify() != other.hasIsModify()) return false;
if (hasIsModify()) {
if (getIsModify()
!= other.getIsModify()) return false;
}
if (hasModifyMD5() != other.hasModifyMD5()) return false;
if (hasModifyMD5()) {
if (!getModifyMD5()
.equals(other.getModifyMD5())) return false;
}
if (hasRqtHash() != other.hasRqtHash()) return false;
if (hasRqtHash()) {
if (getRqtHash()
!= other.getRqtHash()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUnKnown1()) {
hash = (37 * hash) + UNKNOWN1_FIELD_NUMBER;
hash = (53 * hash) + getUnKnown1();
}
if (hasTimeStamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getTimeStamp();
}
if (hasKeyHash()) {
hash = (37 * hash) + KEYHASH_FIELD_NUMBER;
hash = (53 * hash) + getKeyHash();
}
if (hasYes1()) {
hash = (37 * hash) + YES1_FIELD_NUMBER;
hash = (53 * hash) + getYes1().hashCode();
}
if (hasYes2()) {
hash = (37 * hash) + YES2_FIELD_NUMBER;
hash = (53 * hash) + getYes2().hashCode();
}
if (hasIosVersion()) {
hash = (37 * hash) + IOSVERSION_FIELD_NUMBER;
hash = (53 * hash) + getIosVersion().hashCode();
}
if (hasDeviceType()) {
hash = (37 * hash) + DEVICETYPE_FIELD_NUMBER;
hash = (53 * hash) + getDeviceType().hashCode();
}
if (hasUnKnown2()) {
hash = (37 * hash) + UNKNOWN2_FIELD_NUMBER;
hash = (53 * hash) + getUnKnown2();
}
if (hasIdentifierForVendor()) {
hash = (37 * hash) + IDENTIFIERFORVENDOR_FIELD_NUMBER;
hash = (53 * hash) + getIdentifierForVendor().hashCode();
}
if (hasAdvertisingIdentifier()) {
hash = (37 * hash) + ADVERTISINGIDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getAdvertisingIdentifier().hashCode();
}
if (hasCarrier()) {
hash = (37 * hash) + CARRIER_FIELD_NUMBER;
hash = (53 * hash) + getCarrier().hashCode();
}
if (hasBatteryInfo()) {
hash = (37 * hash) + BATTERYINFO_FIELD_NUMBER;
hash = (53 * hash) + getBatteryInfo();
}
if (hasNetworkName()) {
hash = (37 * hash) + NETWORKNAME_FIELD_NUMBER;
hash = (53 * hash) + getNetworkName().hashCode();
}
if (hasNetType()) {
hash = (37 * hash) + NETTYPE_FIELD_NUMBER;
hash = (53 * hash) + getNetType();
}
if (hasAppBundleId()) {
hash = (37 * hash) + APPBUNDLEID_FIELD_NUMBER;
hash = (53 * hash) + getAppBundleId().hashCode();
}
if (hasDeviceName()) {
hash = (37 * hash) + DEVICENAME_FIELD_NUMBER;
hash = (53 * hash) + getDeviceName().hashCode();
}
if (hasUserName()) {
hash = (37 * hash) + USERNAME_FIELD_NUMBER;
hash = (53 * hash) + getUserName().hashCode();
}
if (hasUnknown3()) {
hash = (37 * hash) + UNKNOWN3_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUnknown3());
}
if (hasUnknown4()) {
hash = (37 * hash) + UNKNOWN4_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUnknown4());
}
if (hasUnknown5()) {
hash = (37 * hash) + UNKNOWN5_FIELD_NUMBER;
hash = (53 * hash) + getUnknown5();
}
if (hasUnknown6()) {
hash = (37 * hash) + UNKNOWN6_FIELD_NUMBER;
hash = (53 * hash) + getUnknown6();
}
if (hasLang()) {
hash = (37 * hash) + LANG_FIELD_NUMBER;
hash = (53 * hash) + getLang().hashCode();
}
if (hasCountry()) {
hash = (37 * hash) + COUNTRY_FIELD_NUMBER;
hash = (53 * hash) + getCountry().hashCode();
}
if (hasUnknown7()) {
hash = (37 * hash) + UNKNOWN7_FIELD_NUMBER;
hash = (53 * hash) + getUnknown7();
}
if (hasDocumentDir()) {
hash = (37 * hash) + DOCUMENTDIR_FIELD_NUMBER;
hash = (53 * hash) + getDocumentDir().hashCode();
}
if (hasUnknown8()) {
hash = (37 * hash) + UNKNOWN8_FIELD_NUMBER;
hash = (53 * hash) + getUnknown8();
}
if (hasUnknown9()) {
hash = (37 * hash) + UNKNOWN9_FIELD_NUMBER;
hash = (53 * hash) + getUnknown9();
}
if (hasHeadMD5()) {
hash = (37 * hash) + HEADMD5_FIELD_NUMBER;
hash = (53 * hash) + getHeadMD5().hashCode();
}
if (hasAppUUID()) {
hash = (37 * hash) + APPUUID_FIELD_NUMBER;
hash = (53 * hash) + getAppUUID().hashCode();
}
if (hasSyslogUUID()) {
hash = (37 * hash) + SYSLOGUUID_FIELD_NUMBER;
hash = (53 * hash) + getSyslogUUID().hashCode();
}
if (hasUnknown10()) {
hash = (37 * hash) + UNKNOWN10_FIELD_NUMBER;
hash = (53 * hash) + getUnknown10().hashCode();
}
if (hasUnknown11()) {
hash = (37 * hash) + UNKNOWN11_FIELD_NUMBER;
hash = (53 * hash) + getUnknown11().hashCode();
}
if (hasAppName()) {
hash = (37 * hash) + APPNAME_FIELD_NUMBER;
hash = (53 * hash) + getAppName().hashCode();
}
if (hasSshPath()) {
hash = (37 * hash) + SSHPATH_FIELD_NUMBER;
hash = (53 * hash) + getSshPath().hashCode();
}
if (hasTempTest()) {
hash = (37 * hash) + TEMPTEST_FIELD_NUMBER;
hash = (53 * hash) + getTempTest().hashCode();
}
if (hasDevMD5()) {
hash = (37 * hash) + DEVMD5_FIELD_NUMBER;
hash = (53 * hash) + getDevMD5().hashCode();
}
if (hasDevUser()) {
hash = (37 * hash) + DEVUSER_FIELD_NUMBER;
hash = (53 * hash) + getDevUser().hashCode();
}
if (hasDevPrefix()) {
hash = (37 * hash) + DEVPREFIX_FIELD_NUMBER;
hash = (53 * hash) + getDevPrefix().hashCode();
}
if (getAppFileInfoCount() > 0) {
hash = (37 * hash) + APPFILEINFO_FIELD_NUMBER;
hash = (53 * hash) + getAppFileInfoList().hashCode();
}
if (hasUnknown12()) {
hash = (37 * hash) + UNKNOWN12_FIELD_NUMBER;
hash = (53 * hash) + getUnknown12().hashCode();
}
if (hasIsModify()) {
hash = (37 * hash) + ISMODIFY_FIELD_NUMBER;
hash = (53 * hash) + getIsModify();
}
if (hasModifyMD5()) {
hash = (37 * hash) + MODIFYMD5_FIELD_NUMBER;
hash = (53 * hash) + getModifyMD5().hashCode();
}
if (hasRqtHash()) {
hash = (37 * hash) + RQTHASH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRqtHash());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(wechat.protobuf.SpamDataBodyMessage.SpamDataBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wechat_proto.SpamDataBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wechat_proto.SpamDataBody)
wechat.protobuf.SpamDataBodyMessage.SpamDataBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_SpamDataBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_SpamDataBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.SpamDataBodyMessage.SpamDataBody.class, wechat.protobuf.SpamDataBodyMessage.SpamDataBody.Builder.class);
}
// Construct using wechat.protobuf.SpamDataBodyMessage.SpamDataBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAppFileInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
unKnown1_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
timeStamp_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
keyHash_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
yes1_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
yes2_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
iosVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
deviceType_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
unKnown2_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
identifierForVendor_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
advertisingIdentifier_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
carrier_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
batteryInfo_ = 0;
bitField0_ = (bitField0_ & ~0x00000800);
networkName_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
netType_ = 0;
bitField0_ = (bitField0_ & ~0x00002000);
appBundleId_ = "";
bitField0_ = (bitField0_ & ~0x00004000);
deviceName_ = "";
bitField0_ = (bitField0_ & ~0x00008000);
userName_ = "";
bitField0_ = (bitField0_ & ~0x00010000);
unknown3_ = 0L;
bitField0_ = (bitField0_ & ~0x00020000);
unknown4_ = 0L;
bitField0_ = (bitField0_ & ~0x00040000);
unknown5_ = 0;
bitField0_ = (bitField0_ & ~0x00080000);
unknown6_ = 0;
bitField0_ = (bitField0_ & ~0x00100000);
lang_ = "";
bitField0_ = (bitField0_ & ~0x00200000);
country_ = "";
bitField0_ = (bitField0_ & ~0x00400000);
unknown7_ = 0;
bitField0_ = (bitField0_ & ~0x00800000);
documentDir_ = "";
bitField0_ = (bitField0_ & ~0x01000000);
unknown8_ = 0;
bitField0_ = (bitField0_ & ~0x02000000);
unknown9_ = 0;
bitField0_ = (bitField0_ & ~0x04000000);
headMD5_ = "";
bitField0_ = (bitField0_ & ~0x08000000);
appUUID_ = "";
bitField0_ = (bitField0_ & ~0x10000000);
syslogUUID_ = "";
bitField0_ = (bitField0_ & ~0x20000000);
unknown10_ = "";
bitField0_ = (bitField0_ & ~0x40000000);
unknown11_ = "";
bitField0_ = (bitField0_ & ~0x80000000);
appName_ = "";
bitField1_ = (bitField1_ & ~0x00000001);
sshPath_ = "";
bitField1_ = (bitField1_ & ~0x00000002);
tempTest_ = "";
bitField1_ = (bitField1_ & ~0x00000004);
devMD5_ = "";
bitField1_ = (bitField1_ & ~0x00000008);
devUser_ = "";
bitField1_ = (bitField1_ & ~0x00000010);
devPrefix_ = "";
bitField1_ = (bitField1_ & ~0x00000020);
if (appFileInfoBuilder_ == null) {
appFileInfo_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000040);
} else {
appFileInfoBuilder_.clear();
}
unknown12_ = "";
bitField1_ = (bitField1_ & ~0x00000080);
isModify_ = 0;
bitField1_ = (bitField1_ & ~0x00000100);
modifyMD5_ = "";
bitField1_ = (bitField1_ & ~0x00000200);
rqtHash_ = 0L;
bitField1_ = (bitField1_ & ~0x00000400);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_SpamDataBody_descriptor;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.SpamDataBody getDefaultInstanceForType() {
return wechat.protobuf.SpamDataBodyMessage.SpamDataBody.getDefaultInstance();
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.SpamDataBody build() {
wechat.protobuf.SpamDataBodyMessage.SpamDataBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.SpamDataBody buildPartial() {
wechat.protobuf.SpamDataBodyMessage.SpamDataBody result = new wechat.protobuf.SpamDataBodyMessage.SpamDataBody(this);
int from_bitField0_ = bitField0_;
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
int to_bitField1_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.unKnown1_ = unKnown1_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.timeStamp_ = timeStamp_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.keyHash_ = keyHash_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.yes1_ = yes1_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.yes2_ = yes2_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.iosVersion_ = iosVersion_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.deviceType_ = deviceType_;
if (((from_bitField0_ & 0x00000080) != 0)) {
result.unKnown2_ = unKnown2_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.identifierForVendor_ = identifierForVendor_;
if (((from_bitField0_ & 0x00000200) != 0)) {
to_bitField0_ |= 0x00000200;
}
result.advertisingIdentifier_ = advertisingIdentifier_;
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000400;
}
result.carrier_ = carrier_;
if (((from_bitField0_ & 0x00000800) != 0)) {
result.batteryInfo_ = batteryInfo_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
to_bitField0_ |= 0x00001000;
}
result.networkName_ = networkName_;
if (((from_bitField0_ & 0x00002000) != 0)) {
result.netType_ = netType_;
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
to_bitField0_ |= 0x00004000;
}
result.appBundleId_ = appBundleId_;
if (((from_bitField0_ & 0x00008000) != 0)) {
to_bitField0_ |= 0x00008000;
}
result.deviceName_ = deviceName_;
if (((from_bitField0_ & 0x00010000) != 0)) {
to_bitField0_ |= 0x00010000;
}
result.userName_ = userName_;
if (((from_bitField0_ & 0x00020000) != 0)) {
result.unknown3_ = unknown3_;
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.unknown4_ = unknown4_;
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.unknown5_ = unknown5_;
to_bitField0_ |= 0x00080000;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.unknown6_ = unknown6_;
to_bitField0_ |= 0x00100000;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
to_bitField0_ |= 0x00200000;
}
result.lang_ = lang_;
if (((from_bitField0_ & 0x00400000) != 0)) {
to_bitField0_ |= 0x00400000;
}
result.country_ = country_;
if (((from_bitField0_ & 0x00800000) != 0)) {
result.unknown7_ = unknown7_;
to_bitField0_ |= 0x00800000;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
to_bitField0_ |= 0x01000000;
}
result.documentDir_ = documentDir_;
if (((from_bitField0_ & 0x02000000) != 0)) {
result.unknown8_ = unknown8_;
to_bitField0_ |= 0x02000000;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.unknown9_ = unknown9_;
to_bitField0_ |= 0x04000000;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
to_bitField0_ |= 0x08000000;
}
result.headMD5_ = headMD5_;
if (((from_bitField0_ & 0x10000000) != 0)) {
to_bitField0_ |= 0x10000000;
}
result.appUUID_ = appUUID_;
if (((from_bitField0_ & 0x20000000) != 0)) {
to_bitField0_ |= 0x20000000;
}
result.syslogUUID_ = syslogUUID_;
if (((from_bitField0_ & 0x40000000) != 0)) {
to_bitField0_ |= 0x40000000;
}
result.unknown10_ = unknown10_;
if (((from_bitField0_ & 0x80000000) != 0)) {
to_bitField0_ |= 0x80000000;
}
result.unknown11_ = unknown11_;
if (((from_bitField1_ & 0x00000001) != 0)) {
to_bitField1_ |= 0x00000001;
}
result.appName_ = appName_;
if (((from_bitField1_ & 0x00000002) != 0)) {
to_bitField1_ |= 0x00000002;
}
result.sshPath_ = sshPath_;
if (((from_bitField1_ & 0x00000004) != 0)) {
to_bitField1_ |= 0x00000004;
}
result.tempTest_ = tempTest_;
if (((from_bitField1_ & 0x00000008) != 0)) {
to_bitField1_ |= 0x00000008;
}
result.devMD5_ = devMD5_;
if (((from_bitField1_ & 0x00000010) != 0)) {
to_bitField1_ |= 0x00000010;
}
result.devUser_ = devUser_;
if (((from_bitField1_ & 0x00000020) != 0)) {
to_bitField1_ |= 0x00000020;
}
result.devPrefix_ = devPrefix_;
if (appFileInfoBuilder_ == null) {
if (((bitField1_ & 0x00000040) != 0)) {
appFileInfo_ = java.util.Collections.unmodifiableList(appFileInfo_);
bitField1_ = (bitField1_ & ~0x00000040);
}
result.appFileInfo_ = appFileInfo_;
} else {
result.appFileInfo_ = appFileInfoBuilder_.build();
}
if (((from_bitField1_ & 0x00000080) != 0)) {
to_bitField1_ |= 0x00000040;
}
result.unknown12_ = unknown12_;
if (((from_bitField1_ & 0x00000100) != 0)) {
result.isModify_ = isModify_;
to_bitField1_ |= 0x00000080;
}
if (((from_bitField1_ & 0x00000200) != 0)) {
to_bitField1_ |= 0x00000100;
}
result.modifyMD5_ = modifyMD5_;
if (((from_bitField1_ & 0x00000400) != 0)) {
result.rqtHash_ = rqtHash_;
to_bitField1_ |= 0x00000200;
}
result.bitField0_ = to_bitField0_;
result.bitField1_ = to_bitField1_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof wechat.protobuf.SpamDataBodyMessage.SpamDataBody) {
return mergeFrom((wechat.protobuf.SpamDataBodyMessage.SpamDataBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(wechat.protobuf.SpamDataBodyMessage.SpamDataBody other) {
if (other == wechat.protobuf.SpamDataBodyMessage.SpamDataBody.getDefaultInstance()) return this;
if (other.hasUnKnown1()) {
setUnKnown1(other.getUnKnown1());
}
if (other.hasTimeStamp()) {
setTimeStamp(other.getTimeStamp());
}
if (other.hasKeyHash()) {
setKeyHash(other.getKeyHash());
}
if (other.hasYes1()) {
bitField0_ |= 0x00000008;
yes1_ = other.yes1_;
onChanged();
}
if (other.hasYes2()) {
bitField0_ |= 0x00000010;
yes2_ = other.yes2_;
onChanged();
}
if (other.hasIosVersion()) {
bitField0_ |= 0x00000020;
iosVersion_ = other.iosVersion_;
onChanged();
}
if (other.hasDeviceType()) {
bitField0_ |= 0x00000040;
deviceType_ = other.deviceType_;
onChanged();
}
if (other.hasUnKnown2()) {
setUnKnown2(other.getUnKnown2());
}
if (other.hasIdentifierForVendor()) {
bitField0_ |= 0x00000100;
identifierForVendor_ = other.identifierForVendor_;
onChanged();
}
if (other.hasAdvertisingIdentifier()) {
bitField0_ |= 0x00000200;
advertisingIdentifier_ = other.advertisingIdentifier_;
onChanged();
}
if (other.hasCarrier()) {
bitField0_ |= 0x00000400;
carrier_ = other.carrier_;
onChanged();
}
if (other.hasBatteryInfo()) {
setBatteryInfo(other.getBatteryInfo());
}
if (other.hasNetworkName()) {
bitField0_ |= 0x00001000;
networkName_ = other.networkName_;
onChanged();
}
if (other.hasNetType()) {
setNetType(other.getNetType());
}
if (other.hasAppBundleId()) {
bitField0_ |= 0x00004000;
appBundleId_ = other.appBundleId_;
onChanged();
}
if (other.hasDeviceName()) {
bitField0_ |= 0x00008000;
deviceName_ = other.deviceName_;
onChanged();
}
if (other.hasUserName()) {
bitField0_ |= 0x00010000;
userName_ = other.userName_;
onChanged();
}
if (other.hasUnknown3()) {
setUnknown3(other.getUnknown3());
}
if (other.hasUnknown4()) {
setUnknown4(other.getUnknown4());
}
if (other.hasUnknown5()) {
setUnknown5(other.getUnknown5());
}
if (other.hasUnknown6()) {
setUnknown6(other.getUnknown6());
}
if (other.hasLang()) {
bitField0_ |= 0x00200000;
lang_ = other.lang_;
onChanged();
}
if (other.hasCountry()) {
bitField0_ |= 0x00400000;
country_ = other.country_;
onChanged();
}
if (other.hasUnknown7()) {
setUnknown7(other.getUnknown7());
}
if (other.hasDocumentDir()) {
bitField0_ |= 0x01000000;
documentDir_ = other.documentDir_;
onChanged();
}
if (other.hasUnknown8()) {
setUnknown8(other.getUnknown8());
}
if (other.hasUnknown9()) {
setUnknown9(other.getUnknown9());
}
if (other.hasHeadMD5()) {
bitField0_ |= 0x08000000;
headMD5_ = other.headMD5_;
onChanged();
}
if (other.hasAppUUID()) {
bitField0_ |= 0x10000000;
appUUID_ = other.appUUID_;
onChanged();
}
if (other.hasSyslogUUID()) {
bitField0_ |= 0x20000000;
syslogUUID_ = other.syslogUUID_;
onChanged();
}
if (other.hasUnknown10()) {
bitField0_ |= 0x40000000;
unknown10_ = other.unknown10_;
onChanged();
}
if (other.hasUnknown11()) {
bitField0_ |= 0x80000000;
unknown11_ = other.unknown11_;
onChanged();
}
if (other.hasAppName()) {
bitField1_ |= 0x00000001;
appName_ = other.appName_;
onChanged();
}
if (other.hasSshPath()) {
bitField1_ |= 0x00000002;
sshPath_ = other.sshPath_;
onChanged();
}
if (other.hasTempTest()) {
bitField1_ |= 0x00000004;
tempTest_ = other.tempTest_;
onChanged();
}
if (other.hasDevMD5()) {
bitField1_ |= 0x00000008;
devMD5_ = other.devMD5_;
onChanged();
}
if (other.hasDevUser()) {
bitField1_ |= 0x00000010;
devUser_ = other.devUser_;
onChanged();
}
if (other.hasDevPrefix()) {
bitField1_ |= 0x00000020;
devPrefix_ = other.devPrefix_;
onChanged();
}
if (appFileInfoBuilder_ == null) {
if (!other.appFileInfo_.isEmpty()) {
if (appFileInfo_.isEmpty()) {
appFileInfo_ = other.appFileInfo_;
bitField1_ = (bitField1_ & ~0x00000040);
} else {
ensureAppFileInfoIsMutable();
appFileInfo_.addAll(other.appFileInfo_);
}
onChanged();
}
} else {
if (!other.appFileInfo_.isEmpty()) {
if (appFileInfoBuilder_.isEmpty()) {
appFileInfoBuilder_.dispose();
appFileInfoBuilder_ = null;
appFileInfo_ = other.appFileInfo_;
bitField1_ = (bitField1_ & ~0x00000040);
appFileInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAppFileInfoFieldBuilder() : null;
} else {
appFileInfoBuilder_.addAllMessages(other.appFileInfo_);
}
}
}
if (other.hasUnknown12()) {
bitField1_ |= 0x00000080;
unknown12_ = other.unknown12_;
onChanged();
}
if (other.hasIsModify()) {
setIsModify(other.getIsModify());
}
if (other.hasModifyMD5()) {
bitField1_ |= 0x00000200;
modifyMD5_ = other.modifyMD5_;
onChanged();
}
if (other.hasRqtHash()) {
setRqtHash(other.getRqtHash());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
wechat.protobuf.SpamDataBodyMessage.SpamDataBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (wechat.protobuf.SpamDataBodyMessage.SpamDataBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int bitField1_;
private int unKnown1_ ;
/**
* optional int32 unKnown1 = 1;
* @return Whether the unKnown1 field is set.
*/
@java.lang.Override
public boolean hasUnKnown1() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 unKnown1 = 1;
* @return The unKnown1.
*/
@java.lang.Override
public int getUnKnown1() {
return unKnown1_;
}
/**
* optional int32 unKnown1 = 1;
* @param value The unKnown1 to set.
* @return This builder for chaining.
*/
public Builder setUnKnown1(int value) {
bitField0_ |= 0x00000001;
unKnown1_ = value;
onChanged();
return this;
}
/**
* optional int32 unKnown1 = 1;
* @return This builder for chaining.
*/
public Builder clearUnKnown1() {
bitField0_ = (bitField0_ & ~0x00000001);
unKnown1_ = 0;
onChanged();
return this;
}
private int timeStamp_ ;
/**
* optional int32 timeStamp = 2;
* @return Whether the timeStamp field is set.
*/
@java.lang.Override
public boolean hasTimeStamp() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 timeStamp = 2;
* @return The timeStamp.
*/
@java.lang.Override
public int getTimeStamp() {
return timeStamp_;
}
/**
* optional int32 timeStamp = 2;
* @param value The timeStamp to set.
* @return This builder for chaining.
*/
public Builder setTimeStamp(int value) {
bitField0_ |= 0x00000002;
timeStamp_ = value;
onChanged();
return this;
}
/**
* optional int32 timeStamp = 2;
* @return This builder for chaining.
*/
public Builder clearTimeStamp() {
bitField0_ = (bitField0_ & ~0x00000002);
timeStamp_ = 0;
onChanged();
return this;
}
private int keyHash_ ;
/**
* optional int32 keyHash = 3;
* @return Whether the keyHash field is set.
*/
@java.lang.Override
public boolean hasKeyHash() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int32 keyHash = 3;
* @return The keyHash.
*/
@java.lang.Override
public int getKeyHash() {
return keyHash_;
}
/**
* optional int32 keyHash = 3;
* @param value The keyHash to set.
* @return This builder for chaining.
*/
public Builder setKeyHash(int value) {
bitField0_ |= 0x00000004;
keyHash_ = value;
onChanged();
return this;
}
/**
* optional int32 keyHash = 3;
* @return This builder for chaining.
*/
public Builder clearKeyHash() {
bitField0_ = (bitField0_ & ~0x00000004);
keyHash_ = 0;
onChanged();
return this;
}
private java.lang.Object yes1_ = "";
/**
* optional string yes1 = 11;
* @return Whether the yes1 field is set.
*/
public boolean hasYes1() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string yes1 = 11;
* @return The yes1.
*/
public java.lang.String getYes1() {
java.lang.Object ref = yes1_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
yes1_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string yes1 = 11;
* @return The bytes for yes1.
*/
public com.google.protobuf.ByteString
getYes1Bytes() {
java.lang.Object ref = yes1_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
yes1_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string yes1 = 11;
* @param value The yes1 to set.
* @return This builder for chaining.
*/
public Builder setYes1(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
yes1_ = value;
onChanged();
return this;
}
/**
* optional string yes1 = 11;
* @return This builder for chaining.
*/
public Builder clearYes1() {
bitField0_ = (bitField0_ & ~0x00000008);
yes1_ = getDefaultInstance().getYes1();
onChanged();
return this;
}
/**
* optional string yes1 = 11;
* @param value The bytes for yes1 to set.
* @return This builder for chaining.
*/
public Builder setYes1Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
yes1_ = value;
onChanged();
return this;
}
private java.lang.Object yes2_ = "";
/**
* optional string yes2 = 12;
* @return Whether the yes2 field is set.
*/
public boolean hasYes2() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string yes2 = 12;
* @return The yes2.
*/
public java.lang.String getYes2() {
java.lang.Object ref = yes2_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
yes2_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string yes2 = 12;
* @return The bytes for yes2.
*/
public com.google.protobuf.ByteString
getYes2Bytes() {
java.lang.Object ref = yes2_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
yes2_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string yes2 = 12;
* @param value The yes2 to set.
* @return This builder for chaining.
*/
public Builder setYes2(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
yes2_ = value;
onChanged();
return this;
}
/**
* optional string yes2 = 12;
* @return This builder for chaining.
*/
public Builder clearYes2() {
bitField0_ = (bitField0_ & ~0x00000010);
yes2_ = getDefaultInstance().getYes2();
onChanged();
return this;
}
/**
* optional string yes2 = 12;
* @param value The bytes for yes2 to set.
* @return This builder for chaining.
*/
public Builder setYes2Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
yes2_ = value;
onChanged();
return this;
}
private java.lang.Object iosVersion_ = "";
/**
* optional string iosVersion = 13;
* @return Whether the iosVersion field is set.
*/
public boolean hasIosVersion() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string iosVersion = 13;
* @return The iosVersion.
*/
public java.lang.String getIosVersion() {
java.lang.Object ref = iosVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
iosVersion_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string iosVersion = 13;
* @return The bytes for iosVersion.
*/
public com.google.protobuf.ByteString
getIosVersionBytes() {
java.lang.Object ref = iosVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
iosVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string iosVersion = 13;
* @param value The iosVersion to set.
* @return This builder for chaining.
*/
public Builder setIosVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
iosVersion_ = value;
onChanged();
return this;
}
/**
* optional string iosVersion = 13;
* @return This builder for chaining.
*/
public Builder clearIosVersion() {
bitField0_ = (bitField0_ & ~0x00000020);
iosVersion_ = getDefaultInstance().getIosVersion();
onChanged();
return this;
}
/**
* optional string iosVersion = 13;
* @param value The bytes for iosVersion to set.
* @return This builder for chaining.
*/
public Builder setIosVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
iosVersion_ = value;
onChanged();
return this;
}
private java.lang.Object deviceType_ = "";
/**
* optional string deviceType = 14;
* @return Whether the deviceType field is set.
*/
public boolean hasDeviceType() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional string deviceType = 14;
* @return The deviceType.
*/
public java.lang.String getDeviceType() {
java.lang.Object ref = deviceType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceType_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string deviceType = 14;
* @return The bytes for deviceType.
*/
public com.google.protobuf.ByteString
getDeviceTypeBytes() {
java.lang.Object ref = deviceType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string deviceType = 14;
* @param value The deviceType to set.
* @return This builder for chaining.
*/
public Builder setDeviceType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
deviceType_ = value;
onChanged();
return this;
}
/**
* optional string deviceType = 14;
* @return This builder for chaining.
*/
public Builder clearDeviceType() {
bitField0_ = (bitField0_ & ~0x00000040);
deviceType_ = getDefaultInstance().getDeviceType();
onChanged();
return this;
}
/**
* optional string deviceType = 14;
* @param value The bytes for deviceType to set.
* @return This builder for chaining.
*/
public Builder setDeviceTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
deviceType_ = value;
onChanged();
return this;
}
private int unKnown2_ ;
/**
* optional int32 unKnown2 = 15;
* @return Whether the unKnown2 field is set.
*/
@java.lang.Override
public boolean hasUnKnown2() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional int32 unKnown2 = 15;
* @return The unKnown2.
*/
@java.lang.Override
public int getUnKnown2() {
return unKnown2_;
}
/**
* optional int32 unKnown2 = 15;
* @param value The unKnown2 to set.
* @return This builder for chaining.
*/
public Builder setUnKnown2(int value) {
bitField0_ |= 0x00000080;
unKnown2_ = value;
onChanged();
return this;
}
/**
* optional int32 unKnown2 = 15;
* @return This builder for chaining.
*/
public Builder clearUnKnown2() {
bitField0_ = (bitField0_ & ~0x00000080);
unKnown2_ = 0;
onChanged();
return this;
}
private java.lang.Object identifierForVendor_ = "";
/**
* optional string identifierForVendor = 16;
* @return Whether the identifierForVendor field is set.
*/
public boolean hasIdentifierForVendor() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional string identifierForVendor = 16;
* @return The identifierForVendor.
*/
public java.lang.String getIdentifierForVendor() {
java.lang.Object ref = identifierForVendor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
identifierForVendor_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string identifierForVendor = 16;
* @return The bytes for identifierForVendor.
*/
public com.google.protobuf.ByteString
getIdentifierForVendorBytes() {
java.lang.Object ref = identifierForVendor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identifierForVendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string identifierForVendor = 16;
* @param value The identifierForVendor to set.
* @return This builder for chaining.
*/
public Builder setIdentifierForVendor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
identifierForVendor_ = value;
onChanged();
return this;
}
/**
* optional string identifierForVendor = 16;
* @return This builder for chaining.
*/
public Builder clearIdentifierForVendor() {
bitField0_ = (bitField0_ & ~0x00000100);
identifierForVendor_ = getDefaultInstance().getIdentifierForVendor();
onChanged();
return this;
}
/**
* optional string identifierForVendor = 16;
* @param value The bytes for identifierForVendor to set.
* @return This builder for chaining.
*/
public Builder setIdentifierForVendorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
identifierForVendor_ = value;
onChanged();
return this;
}
private java.lang.Object advertisingIdentifier_ = "";
/**
* optional string advertisingIdentifier = 17;
* @return Whether the advertisingIdentifier field is set.
*/
public boolean hasAdvertisingIdentifier() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional string advertisingIdentifier = 17;
* @return The advertisingIdentifier.
*/
public java.lang.String getAdvertisingIdentifier() {
java.lang.Object ref = advertisingIdentifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
advertisingIdentifier_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string advertisingIdentifier = 17;
* @return The bytes for advertisingIdentifier.
*/
public com.google.protobuf.ByteString
getAdvertisingIdentifierBytes() {
java.lang.Object ref = advertisingIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
advertisingIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string advertisingIdentifier = 17;
* @param value The advertisingIdentifier to set.
* @return This builder for chaining.
*/
public Builder setAdvertisingIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
advertisingIdentifier_ = value;
onChanged();
return this;
}
/**
* optional string advertisingIdentifier = 17;
* @return This builder for chaining.
*/
public Builder clearAdvertisingIdentifier() {
bitField0_ = (bitField0_ & ~0x00000200);
advertisingIdentifier_ = getDefaultInstance().getAdvertisingIdentifier();
onChanged();
return this;
}
/**
* optional string advertisingIdentifier = 17;
* @param value The bytes for advertisingIdentifier to set.
* @return This builder for chaining.
*/
public Builder setAdvertisingIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
advertisingIdentifier_ = value;
onChanged();
return this;
}
private java.lang.Object carrier_ = "";
/**
* optional string carrier = 18;
* @return Whether the carrier field is set.
*/
public boolean hasCarrier() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional string carrier = 18;
* @return The carrier.
*/
public java.lang.String getCarrier() {
java.lang.Object ref = carrier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
carrier_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string carrier = 18;
* @return The bytes for carrier.
*/
public com.google.protobuf.ByteString
getCarrierBytes() {
java.lang.Object ref = carrier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
carrier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string carrier = 18;
* @param value The carrier to set.
* @return This builder for chaining.
*/
public Builder setCarrier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
carrier_ = value;
onChanged();
return this;
}
/**
* optional string carrier = 18;
* @return This builder for chaining.
*/
public Builder clearCarrier() {
bitField0_ = (bitField0_ & ~0x00000400);
carrier_ = getDefaultInstance().getCarrier();
onChanged();
return this;
}
/**
* optional string carrier = 18;
* @param value The bytes for carrier to set.
* @return This builder for chaining.
*/
public Builder setCarrierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
carrier_ = value;
onChanged();
return this;
}
private int batteryInfo_ ;
/**
* optional int32 batteryInfo = 19;
* @return Whether the batteryInfo field is set.
*/
@java.lang.Override
public boolean hasBatteryInfo() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
* optional int32 batteryInfo = 19;
* @return The batteryInfo.
*/
@java.lang.Override
public int getBatteryInfo() {
return batteryInfo_;
}
/**
* optional int32 batteryInfo = 19;
* @param value The batteryInfo to set.
* @return This builder for chaining.
*/
public Builder setBatteryInfo(int value) {
bitField0_ |= 0x00000800;
batteryInfo_ = value;
onChanged();
return this;
}
/**
* optional int32 batteryInfo = 19;
* @return This builder for chaining.
*/
public Builder clearBatteryInfo() {
bitField0_ = (bitField0_ & ~0x00000800);
batteryInfo_ = 0;
onChanged();
return this;
}
private java.lang.Object networkName_ = "";
/**
* optional string networkName = 20;
* @return Whether the networkName field is set.
*/
public boolean hasNetworkName() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
* optional string networkName = 20;
* @return The networkName.
*/
public java.lang.String getNetworkName() {
java.lang.Object ref = networkName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
networkName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string networkName = 20;
* @return The bytes for networkName.
*/
public com.google.protobuf.ByteString
getNetworkNameBytes() {
java.lang.Object ref = networkName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
networkName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string networkName = 20;
* @param value The networkName to set.
* @return This builder for chaining.
*/
public Builder setNetworkName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
networkName_ = value;
onChanged();
return this;
}
/**
* optional string networkName = 20;
* @return This builder for chaining.
*/
public Builder clearNetworkName() {
bitField0_ = (bitField0_ & ~0x00001000);
networkName_ = getDefaultInstance().getNetworkName();
onChanged();
return this;
}
/**
* optional string networkName = 20;
* @param value The bytes for networkName to set.
* @return This builder for chaining.
*/
public Builder setNetworkNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
networkName_ = value;
onChanged();
return this;
}
private int netType_ ;
/**
* optional int32 netType = 21;
* @return Whether the netType field is set.
*/
@java.lang.Override
public boolean hasNetType() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
* optional int32 netType = 21;
* @return The netType.
*/
@java.lang.Override
public int getNetType() {
return netType_;
}
/**
* optional int32 netType = 21;
* @param value The netType to set.
* @return This builder for chaining.
*/
public Builder setNetType(int value) {
bitField0_ |= 0x00002000;
netType_ = value;
onChanged();
return this;
}
/**
* optional int32 netType = 21;
* @return This builder for chaining.
*/
public Builder clearNetType() {
bitField0_ = (bitField0_ & ~0x00002000);
netType_ = 0;
onChanged();
return this;
}
private java.lang.Object appBundleId_ = "";
/**
* optional string appBundleId = 22;
* @return Whether the appBundleId field is set.
*/
public boolean hasAppBundleId() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
* optional string appBundleId = 22;
* @return The appBundleId.
*/
public java.lang.String getAppBundleId() {
java.lang.Object ref = appBundleId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appBundleId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string appBundleId = 22;
* @return The bytes for appBundleId.
*/
public com.google.protobuf.ByteString
getAppBundleIdBytes() {
java.lang.Object ref = appBundleId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appBundleId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string appBundleId = 22;
* @param value The appBundleId to set.
* @return This builder for chaining.
*/
public Builder setAppBundleId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
appBundleId_ = value;
onChanged();
return this;
}
/**
* optional string appBundleId = 22;
* @return This builder for chaining.
*/
public Builder clearAppBundleId() {
bitField0_ = (bitField0_ & ~0x00004000);
appBundleId_ = getDefaultInstance().getAppBundleId();
onChanged();
return this;
}
/**
* optional string appBundleId = 22;
* @param value The bytes for appBundleId to set.
* @return This builder for chaining.
*/
public Builder setAppBundleIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
appBundleId_ = value;
onChanged();
return this;
}
private java.lang.Object deviceName_ = "";
/**
* optional string deviceName = 23;
* @return Whether the deviceName field is set.
*/
public boolean hasDeviceName() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
* optional string deviceName = 23;
* @return The deviceName.
*/
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string deviceName = 23;
* @return The bytes for deviceName.
*/
public com.google.protobuf.ByteString
getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string deviceName = 23;
* @param value The deviceName to set.
* @return This builder for chaining.
*/
public Builder setDeviceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
deviceName_ = value;
onChanged();
return this;
}
/**
* optional string deviceName = 23;
* @return This builder for chaining.
*/
public Builder clearDeviceName() {
bitField0_ = (bitField0_ & ~0x00008000);
deviceName_ = getDefaultInstance().getDeviceName();
onChanged();
return this;
}
/**
* optional string deviceName = 23;
* @param value The bytes for deviceName to set.
* @return This builder for chaining.
*/
public Builder setDeviceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
deviceName_ = value;
onChanged();
return this;
}
private java.lang.Object userName_ = "";
/**
* optional string userName = 24;
* @return Whether the userName field is set.
*/
public boolean hasUserName() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
* optional string userName = 24;
* @return The userName.
*/
public java.lang.String getUserName() {
java.lang.Object ref = userName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string userName = 24;
* @return The bytes for userName.
*/
public com.google.protobuf.ByteString
getUserNameBytes() {
java.lang.Object ref = userName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string userName = 24;
* @param value The userName to set.
* @return This builder for chaining.
*/
public Builder setUserName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
userName_ = value;
onChanged();
return this;
}
/**
* optional string userName = 24;
* @return This builder for chaining.
*/
public Builder clearUserName() {
bitField0_ = (bitField0_ & ~0x00010000);
userName_ = getDefaultInstance().getUserName();
onChanged();
return this;
}
/**
* optional string userName = 24;
* @param value The bytes for userName to set.
* @return This builder for chaining.
*/
public Builder setUserNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
userName_ = value;
onChanged();
return this;
}
private long unknown3_ ;
/**
* optional int64 unknown3 = 25;
* @return Whether the unknown3 field is set.
*/
@java.lang.Override
public boolean hasUnknown3() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
* optional int64 unknown3 = 25;
* @return The unknown3.
*/
@java.lang.Override
public long getUnknown3() {
return unknown3_;
}
/**
* optional int64 unknown3 = 25;
* @param value The unknown3 to set.
* @return This builder for chaining.
*/
public Builder setUnknown3(long value) {
bitField0_ |= 0x00020000;
unknown3_ = value;
onChanged();
return this;
}
/**
* optional int64 unknown3 = 25;
* @return This builder for chaining.
*/
public Builder clearUnknown3() {
bitField0_ = (bitField0_ & ~0x00020000);
unknown3_ = 0L;
onChanged();
return this;
}
private long unknown4_ ;
/**
* optional int64 unknown4 = 26;
* @return Whether the unknown4 field is set.
*/
@java.lang.Override
public boolean hasUnknown4() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
* optional int64 unknown4 = 26;
* @return The unknown4.
*/
@java.lang.Override
public long getUnknown4() {
return unknown4_;
}
/**
* optional int64 unknown4 = 26;
* @param value The unknown4 to set.
* @return This builder for chaining.
*/
public Builder setUnknown4(long value) {
bitField0_ |= 0x00040000;
unknown4_ = value;
onChanged();
return this;
}
/**
* optional int64 unknown4 = 26;
* @return This builder for chaining.
*/
public Builder clearUnknown4() {
bitField0_ = (bitField0_ & ~0x00040000);
unknown4_ = 0L;
onChanged();
return this;
}
private int unknown5_ ;
/**
* optional int32 unknown5 = 27;
* @return Whether the unknown5 field is set.
*/
@java.lang.Override
public boolean hasUnknown5() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
* optional int32 unknown5 = 27;
* @return The unknown5.
*/
@java.lang.Override
public int getUnknown5() {
return unknown5_;
}
/**
* optional int32 unknown5 = 27;
* @param value The unknown5 to set.
* @return This builder for chaining.
*/
public Builder setUnknown5(int value) {
bitField0_ |= 0x00080000;
unknown5_ = value;
onChanged();
return this;
}
/**
* optional int32 unknown5 = 27;
* @return This builder for chaining.
*/
public Builder clearUnknown5() {
bitField0_ = (bitField0_ & ~0x00080000);
unknown5_ = 0;
onChanged();
return this;
}
private int unknown6_ ;
/**
* optional int32 unknown6 = 28;
* @return Whether the unknown6 field is set.
*/
@java.lang.Override
public boolean hasUnknown6() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
* optional int32 unknown6 = 28;
* @return The unknown6.
*/
@java.lang.Override
public int getUnknown6() {
return unknown6_;
}
/**
* optional int32 unknown6 = 28;
* @param value The unknown6 to set.
* @return This builder for chaining.
*/
public Builder setUnknown6(int value) {
bitField0_ |= 0x00100000;
unknown6_ = value;
onChanged();
return this;
}
/**
* optional int32 unknown6 = 28;
* @return This builder for chaining.
*/
public Builder clearUnknown6() {
bitField0_ = (bitField0_ & ~0x00100000);
unknown6_ = 0;
onChanged();
return this;
}
private java.lang.Object lang_ = "";
/**
* optional string lang = 29;
* @return Whether the lang field is set.
*/
public boolean hasLang() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
* optional string lang = 29;
* @return The lang.
*/
public java.lang.String getLang() {
java.lang.Object ref = lang_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
lang_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string lang = 29;
* @return The bytes for lang.
*/
public com.google.protobuf.ByteString
getLangBytes() {
java.lang.Object ref = lang_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lang_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string lang = 29;
* @param value The lang to set.
* @return This builder for chaining.
*/
public Builder setLang(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00200000;
lang_ = value;
onChanged();
return this;
}
/**
* optional string lang = 29;
* @return This builder for chaining.
*/
public Builder clearLang() {
bitField0_ = (bitField0_ & ~0x00200000);
lang_ = getDefaultInstance().getLang();
onChanged();
return this;
}
/**
* optional string lang = 29;
* @param value The bytes for lang to set.
* @return This builder for chaining.
*/
public Builder setLangBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00200000;
lang_ = value;
onChanged();
return this;
}
private java.lang.Object country_ = "";
/**
* optional string country = 30;
* @return Whether the country field is set.
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
* optional string country = 30;
* @return The country.
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string country = 30;
* @return The bytes for country.
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string country = 30;
* @param value The country to set.
* @return This builder for chaining.
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00400000;
country_ = value;
onChanged();
return this;
}
/**
* optional string country = 30;
* @return This builder for chaining.
*/
public Builder clearCountry() {
bitField0_ = (bitField0_ & ~0x00400000);
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* optional string country = 30;
* @param value The bytes for country to set.
* @return This builder for chaining.
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00400000;
country_ = value;
onChanged();
return this;
}
private int unknown7_ ;
/**
* optional int32 unknown7 = 31;
* @return Whether the unknown7 field is set.
*/
@java.lang.Override
public boolean hasUnknown7() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
* optional int32 unknown7 = 31;
* @return The unknown7.
*/
@java.lang.Override
public int getUnknown7() {
return unknown7_;
}
/**
* optional int32 unknown7 = 31;
* @param value The unknown7 to set.
* @return This builder for chaining.
*/
public Builder setUnknown7(int value) {
bitField0_ |= 0x00800000;
unknown7_ = value;
onChanged();
return this;
}
/**
* optional int32 unknown7 = 31;
* @return This builder for chaining.
*/
public Builder clearUnknown7() {
bitField0_ = (bitField0_ & ~0x00800000);
unknown7_ = 0;
onChanged();
return this;
}
private java.lang.Object documentDir_ = "";
/**
* optional string documentDir = 32;
* @return Whether the documentDir field is set.
*/
public boolean hasDocumentDir() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
* optional string documentDir = 32;
* @return The documentDir.
*/
public java.lang.String getDocumentDir() {
java.lang.Object ref = documentDir_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
documentDir_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string documentDir = 32;
* @return The bytes for documentDir.
*/
public com.google.protobuf.ByteString
getDocumentDirBytes() {
java.lang.Object ref = documentDir_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
documentDir_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string documentDir = 32;
* @param value The documentDir to set.
* @return This builder for chaining.
*/
public Builder setDocumentDir(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x01000000;
documentDir_ = value;
onChanged();
return this;
}
/**
* optional string documentDir = 32;
* @return This builder for chaining.
*/
public Builder clearDocumentDir() {
bitField0_ = (bitField0_ & ~0x01000000);
documentDir_ = getDefaultInstance().getDocumentDir();
onChanged();
return this;
}
/**
* optional string documentDir = 32;
* @param value The bytes for documentDir to set.
* @return This builder for chaining.
*/
public Builder setDocumentDirBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x01000000;
documentDir_ = value;
onChanged();
return this;
}
private int unknown8_ ;
/**
* optional int32 unknown8 = 33;
* @return Whether the unknown8 field is set.
*/
@java.lang.Override
public boolean hasUnknown8() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
* optional int32 unknown8 = 33;
* @return The unknown8.
*/
@java.lang.Override
public int getUnknown8() {
return unknown8_;
}
/**
* optional int32 unknown8 = 33;
* @param value The unknown8 to set.
* @return This builder for chaining.
*/
public Builder setUnknown8(int value) {
bitField0_ |= 0x02000000;
unknown8_ = value;
onChanged();
return this;
}
/**
* optional int32 unknown8 = 33;
* @return This builder for chaining.
*/
public Builder clearUnknown8() {
bitField0_ = (bitField0_ & ~0x02000000);
unknown8_ = 0;
onChanged();
return this;
}
private int unknown9_ ;
/**
* optional int32 unknown9 = 34;
* @return Whether the unknown9 field is set.
*/
@java.lang.Override
public boolean hasUnknown9() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
* optional int32 unknown9 = 34;
* @return The unknown9.
*/
@java.lang.Override
public int getUnknown9() {
return unknown9_;
}
/**
* optional int32 unknown9 = 34;
* @param value The unknown9 to set.
* @return This builder for chaining.
*/
public Builder setUnknown9(int value) {
bitField0_ |= 0x04000000;
unknown9_ = value;
onChanged();
return this;
}
/**
* optional int32 unknown9 = 34;
* @return This builder for chaining.
*/
public Builder clearUnknown9() {
bitField0_ = (bitField0_ & ~0x04000000);
unknown9_ = 0;
onChanged();
return this;
}
private java.lang.Object headMD5_ = "";
/**
* optional string headMD5 = 35;
* @return Whether the headMD5 field is set.
*/
public boolean hasHeadMD5() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
* optional string headMD5 = 35;
* @return The headMD5.
*/
public java.lang.String getHeadMD5() {
java.lang.Object ref = headMD5_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
headMD5_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string headMD5 = 35;
* @return The bytes for headMD5.
*/
public com.google.protobuf.ByteString
getHeadMD5Bytes() {
java.lang.Object ref = headMD5_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
headMD5_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string headMD5 = 35;
* @param value The headMD5 to set.
* @return This builder for chaining.
*/
public Builder setHeadMD5(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x08000000;
headMD5_ = value;
onChanged();
return this;
}
/**
* optional string headMD5 = 35;
* @return This builder for chaining.
*/
public Builder clearHeadMD5() {
bitField0_ = (bitField0_ & ~0x08000000);
headMD5_ = getDefaultInstance().getHeadMD5();
onChanged();
return this;
}
/**
* optional string headMD5 = 35;
* @param value The bytes for headMD5 to set.
* @return This builder for chaining.
*/
public Builder setHeadMD5Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x08000000;
headMD5_ = value;
onChanged();
return this;
}
private java.lang.Object appUUID_ = "";
/**
* optional string appUUID = 36;
* @return Whether the appUUID field is set.
*/
public boolean hasAppUUID() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
* optional string appUUID = 36;
* @return The appUUID.
*/
public java.lang.String getAppUUID() {
java.lang.Object ref = appUUID_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appUUID_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string appUUID = 36;
* @return The bytes for appUUID.
*/
public com.google.protobuf.ByteString
getAppUUIDBytes() {
java.lang.Object ref = appUUID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appUUID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string appUUID = 36;
* @param value The appUUID to set.
* @return This builder for chaining.
*/
public Builder setAppUUID(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x10000000;
appUUID_ = value;
onChanged();
return this;
}
/**
* optional string appUUID = 36;
* @return This builder for chaining.
*/
public Builder clearAppUUID() {
bitField0_ = (bitField0_ & ~0x10000000);
appUUID_ = getDefaultInstance().getAppUUID();
onChanged();
return this;
}
/**
* optional string appUUID = 36;
* @param value The bytes for appUUID to set.
* @return This builder for chaining.
*/
public Builder setAppUUIDBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x10000000;
appUUID_ = value;
onChanged();
return this;
}
private java.lang.Object syslogUUID_ = "";
/**
* optional string syslogUUID = 37;
* @return Whether the syslogUUID field is set.
*/
public boolean hasSyslogUUID() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
* optional string syslogUUID = 37;
* @return The syslogUUID.
*/
public java.lang.String getSyslogUUID() {
java.lang.Object ref = syslogUUID_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
syslogUUID_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string syslogUUID = 37;
* @return The bytes for syslogUUID.
*/
public com.google.protobuf.ByteString
getSyslogUUIDBytes() {
java.lang.Object ref = syslogUUID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
syslogUUID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string syslogUUID = 37;
* @param value The syslogUUID to set.
* @return This builder for chaining.
*/
public Builder setSyslogUUID(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x20000000;
syslogUUID_ = value;
onChanged();
return this;
}
/**
* optional string syslogUUID = 37;
* @return This builder for chaining.
*/
public Builder clearSyslogUUID() {
bitField0_ = (bitField0_ & ~0x20000000);
syslogUUID_ = getDefaultInstance().getSyslogUUID();
onChanged();
return this;
}
/**
* optional string syslogUUID = 37;
* @param value The bytes for syslogUUID to set.
* @return This builder for chaining.
*/
public Builder setSyslogUUIDBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x20000000;
syslogUUID_ = value;
onChanged();
return this;
}
private java.lang.Object unknown10_ = "";
/**
* optional string unknown10 = 38;
* @return Whether the unknown10 field is set.
*/
public boolean hasUnknown10() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
* optional string unknown10 = 38;
* @return The unknown10.
*/
public java.lang.String getUnknown10() {
java.lang.Object ref = unknown10_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unknown10_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string unknown10 = 38;
* @return The bytes for unknown10.
*/
public com.google.protobuf.ByteString
getUnknown10Bytes() {
java.lang.Object ref = unknown10_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unknown10_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string unknown10 = 38;
* @param value The unknown10 to set.
* @return This builder for chaining.
*/
public Builder setUnknown10(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x40000000;
unknown10_ = value;
onChanged();
return this;
}
/**
* optional string unknown10 = 38;
* @return This builder for chaining.
*/
public Builder clearUnknown10() {
bitField0_ = (bitField0_ & ~0x40000000);
unknown10_ = getDefaultInstance().getUnknown10();
onChanged();
return this;
}
/**
* optional string unknown10 = 38;
* @param value The bytes for unknown10 to set.
* @return This builder for chaining.
*/
public Builder setUnknown10Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x40000000;
unknown10_ = value;
onChanged();
return this;
}
private java.lang.Object unknown11_ = "";
/**
* optional string unknown11 = 39;
* @return Whether the unknown11 field is set.
*/
public boolean hasUnknown11() {
return ((bitField0_ & 0x80000000) != 0);
}
/**
* optional string unknown11 = 39;
* @return The unknown11.
*/
public java.lang.String getUnknown11() {
java.lang.Object ref = unknown11_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unknown11_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string unknown11 = 39;
* @return The bytes for unknown11.
*/
public com.google.protobuf.ByteString
getUnknown11Bytes() {
java.lang.Object ref = unknown11_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unknown11_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string unknown11 = 39;
* @param value The unknown11 to set.
* @return This builder for chaining.
*/
public Builder setUnknown11(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x80000000;
unknown11_ = value;
onChanged();
return this;
}
/**
* optional string unknown11 = 39;
* @return This builder for chaining.
*/
public Builder clearUnknown11() {
bitField0_ = (bitField0_ & ~0x80000000);
unknown11_ = getDefaultInstance().getUnknown11();
onChanged();
return this;
}
/**
* optional string unknown11 = 39;
* @param value The bytes for unknown11 to set.
* @return This builder for chaining.
*/
public Builder setUnknown11Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x80000000;
unknown11_ = value;
onChanged();
return this;
}
private java.lang.Object appName_ = "";
/**
* optional string appName = 40;
* @return Whether the appName field is set.
*/
public boolean hasAppName() {
return ((bitField1_ & 0x00000001) != 0);
}
/**
* optional string appName = 40;
* @return The appName.
*/
public java.lang.String getAppName() {
java.lang.Object ref = appName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string appName = 40;
* @return The bytes for appName.
*/
public com.google.protobuf.ByteString
getAppNameBytes() {
java.lang.Object ref = appName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string appName = 40;
* @param value The appName to set.
* @return This builder for chaining.
*/
public Builder setAppName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000001;
appName_ = value;
onChanged();
return this;
}
/**
* optional string appName = 40;
* @return This builder for chaining.
*/
public Builder clearAppName() {
bitField1_ = (bitField1_ & ~0x00000001);
appName_ = getDefaultInstance().getAppName();
onChanged();
return this;
}
/**
* optional string appName = 40;
* @param value The bytes for appName to set.
* @return This builder for chaining.
*/
public Builder setAppNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000001;
appName_ = value;
onChanged();
return this;
}
private java.lang.Object sshPath_ = "";
/**
* optional string sshPath = 41;
* @return Whether the sshPath field is set.
*/
public boolean hasSshPath() {
return ((bitField1_ & 0x00000002) != 0);
}
/**
* optional string sshPath = 41;
* @return The sshPath.
*/
public java.lang.String getSshPath() {
java.lang.Object ref = sshPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sshPath_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string sshPath = 41;
* @return The bytes for sshPath.
*/
public com.google.protobuf.ByteString
getSshPathBytes() {
java.lang.Object ref = sshPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sshPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string sshPath = 41;
* @param value The sshPath to set.
* @return This builder for chaining.
*/
public Builder setSshPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000002;
sshPath_ = value;
onChanged();
return this;
}
/**
* optional string sshPath = 41;
* @return This builder for chaining.
*/
public Builder clearSshPath() {
bitField1_ = (bitField1_ & ~0x00000002);
sshPath_ = getDefaultInstance().getSshPath();
onChanged();
return this;
}
/**
* optional string sshPath = 41;
* @param value The bytes for sshPath to set.
* @return This builder for chaining.
*/
public Builder setSshPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000002;
sshPath_ = value;
onChanged();
return this;
}
private java.lang.Object tempTest_ = "";
/**
* optional string tempTest = 42;
* @return Whether the tempTest field is set.
*/
public boolean hasTempTest() {
return ((bitField1_ & 0x00000004) != 0);
}
/**
* optional string tempTest = 42;
* @return The tempTest.
*/
public java.lang.String getTempTest() {
java.lang.Object ref = tempTest_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tempTest_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string tempTest = 42;
* @return The bytes for tempTest.
*/
public com.google.protobuf.ByteString
getTempTestBytes() {
java.lang.Object ref = tempTest_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tempTest_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string tempTest = 42;
* @param value The tempTest to set.
* @return This builder for chaining.
*/
public Builder setTempTest(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000004;
tempTest_ = value;
onChanged();
return this;
}
/**
* optional string tempTest = 42;
* @return This builder for chaining.
*/
public Builder clearTempTest() {
bitField1_ = (bitField1_ & ~0x00000004);
tempTest_ = getDefaultInstance().getTempTest();
onChanged();
return this;
}
/**
* optional string tempTest = 42;
* @param value The bytes for tempTest to set.
* @return This builder for chaining.
*/
public Builder setTempTestBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000004;
tempTest_ = value;
onChanged();
return this;
}
private java.lang.Object devMD5_ = "";
/**
* optional string devMD5 = 43;
* @return Whether the devMD5 field is set.
*/
public boolean hasDevMD5() {
return ((bitField1_ & 0x00000008) != 0);
}
/**
* optional string devMD5 = 43;
* @return The devMD5.
*/
public java.lang.String getDevMD5() {
java.lang.Object ref = devMD5_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
devMD5_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string devMD5 = 43;
* @return The bytes for devMD5.
*/
public com.google.protobuf.ByteString
getDevMD5Bytes() {
java.lang.Object ref = devMD5_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
devMD5_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string devMD5 = 43;
* @param value The devMD5 to set.
* @return This builder for chaining.
*/
public Builder setDevMD5(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000008;
devMD5_ = value;
onChanged();
return this;
}
/**
* optional string devMD5 = 43;
* @return This builder for chaining.
*/
public Builder clearDevMD5() {
bitField1_ = (bitField1_ & ~0x00000008);
devMD5_ = getDefaultInstance().getDevMD5();
onChanged();
return this;
}
/**
* optional string devMD5 = 43;
* @param value The bytes for devMD5 to set.
* @return This builder for chaining.
*/
public Builder setDevMD5Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000008;
devMD5_ = value;
onChanged();
return this;
}
private java.lang.Object devUser_ = "";
/**
* optional string devUser = 44;
* @return Whether the devUser field is set.
*/
public boolean hasDevUser() {
return ((bitField1_ & 0x00000010) != 0);
}
/**
* optional string devUser = 44;
* @return The devUser.
*/
public java.lang.String getDevUser() {
java.lang.Object ref = devUser_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
devUser_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string devUser = 44;
* @return The bytes for devUser.
*/
public com.google.protobuf.ByteString
getDevUserBytes() {
java.lang.Object ref = devUser_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
devUser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string devUser = 44;
* @param value The devUser to set.
* @return This builder for chaining.
*/
public Builder setDevUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000010;
devUser_ = value;
onChanged();
return this;
}
/**
* optional string devUser = 44;
* @return This builder for chaining.
*/
public Builder clearDevUser() {
bitField1_ = (bitField1_ & ~0x00000010);
devUser_ = getDefaultInstance().getDevUser();
onChanged();
return this;
}
/**
* optional string devUser = 44;
* @param value The bytes for devUser to set.
* @return This builder for chaining.
*/
public Builder setDevUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000010;
devUser_ = value;
onChanged();
return this;
}
private java.lang.Object devPrefix_ = "";
/**
* optional string devPrefix = 45;
* @return Whether the devPrefix field is set.
*/
public boolean hasDevPrefix() {
return ((bitField1_ & 0x00000020) != 0);
}
/**
* optional string devPrefix = 45;
* @return The devPrefix.
*/
public java.lang.String getDevPrefix() {
java.lang.Object ref = devPrefix_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
devPrefix_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string devPrefix = 45;
* @return The bytes for devPrefix.
*/
public com.google.protobuf.ByteString
getDevPrefixBytes() {
java.lang.Object ref = devPrefix_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
devPrefix_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string devPrefix = 45;
* @param value The devPrefix to set.
* @return This builder for chaining.
*/
public Builder setDevPrefix(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000020;
devPrefix_ = value;
onChanged();
return this;
}
/**
* optional string devPrefix = 45;
* @return This builder for chaining.
*/
public Builder clearDevPrefix() {
bitField1_ = (bitField1_ & ~0x00000020);
devPrefix_ = getDefaultInstance().getDevPrefix();
onChanged();
return this;
}
/**
* optional string devPrefix = 45;
* @param value The bytes for devPrefix to set.
* @return This builder for chaining.
*/
public Builder setDevPrefixBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000020;
devPrefix_ = value;
onChanged();
return this;
}
private java.util.List appFileInfo_ =
java.util.Collections.emptyList();
private void ensureAppFileInfoIsMutable() {
if (!((bitField1_ & 0x00000040) != 0)) {
appFileInfo_ = new java.util.ArrayList(appFileInfo_);
bitField1_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SpamDataBodyMessage.FileInfo, wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder, wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder> appFileInfoBuilder_;
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public java.util.List getAppFileInfoList() {
if (appFileInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(appFileInfo_);
} else {
return appFileInfoBuilder_.getMessageList();
}
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public int getAppFileInfoCount() {
if (appFileInfoBuilder_ == null) {
return appFileInfo_.size();
} else {
return appFileInfoBuilder_.getCount();
}
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public wechat.protobuf.SpamDataBodyMessage.FileInfo getAppFileInfo(int index) {
if (appFileInfoBuilder_ == null) {
return appFileInfo_.get(index);
} else {
return appFileInfoBuilder_.getMessage(index);
}
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder setAppFileInfo(
int index, wechat.protobuf.SpamDataBodyMessage.FileInfo value) {
if (appFileInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAppFileInfoIsMutable();
appFileInfo_.set(index, value);
onChanged();
} else {
appFileInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder setAppFileInfo(
int index, wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder builderForValue) {
if (appFileInfoBuilder_ == null) {
ensureAppFileInfoIsMutable();
appFileInfo_.set(index, builderForValue.build());
onChanged();
} else {
appFileInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder addAppFileInfo(wechat.protobuf.SpamDataBodyMessage.FileInfo value) {
if (appFileInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAppFileInfoIsMutable();
appFileInfo_.add(value);
onChanged();
} else {
appFileInfoBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder addAppFileInfo(
int index, wechat.protobuf.SpamDataBodyMessage.FileInfo value) {
if (appFileInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAppFileInfoIsMutable();
appFileInfo_.add(index, value);
onChanged();
} else {
appFileInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder addAppFileInfo(
wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder builderForValue) {
if (appFileInfoBuilder_ == null) {
ensureAppFileInfoIsMutable();
appFileInfo_.add(builderForValue.build());
onChanged();
} else {
appFileInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder addAppFileInfo(
int index, wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder builderForValue) {
if (appFileInfoBuilder_ == null) {
ensureAppFileInfoIsMutable();
appFileInfo_.add(index, builderForValue.build());
onChanged();
} else {
appFileInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder addAllAppFileInfo(
java.lang.Iterable extends wechat.protobuf.SpamDataBodyMessage.FileInfo> values) {
if (appFileInfoBuilder_ == null) {
ensureAppFileInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, appFileInfo_);
onChanged();
} else {
appFileInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder clearAppFileInfo() {
if (appFileInfoBuilder_ == null) {
appFileInfo_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000040);
onChanged();
} else {
appFileInfoBuilder_.clear();
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public Builder removeAppFileInfo(int index) {
if (appFileInfoBuilder_ == null) {
ensureAppFileInfoIsMutable();
appFileInfo_.remove(index);
onChanged();
} else {
appFileInfoBuilder_.remove(index);
}
return this;
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder getAppFileInfoBuilder(
int index) {
return getAppFileInfoFieldBuilder().getBuilder(index);
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder getAppFileInfoOrBuilder(
int index) {
if (appFileInfoBuilder_ == null) {
return appFileInfo_.get(index); } else {
return appFileInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public java.util.List extends wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder>
getAppFileInfoOrBuilderList() {
if (appFileInfoBuilder_ != null) {
return appFileInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(appFileInfo_);
}
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder addAppFileInfoBuilder() {
return getAppFileInfoFieldBuilder().addBuilder(
wechat.protobuf.SpamDataBodyMessage.FileInfo.getDefaultInstance());
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder addAppFileInfoBuilder(
int index) {
return getAppFileInfoFieldBuilder().addBuilder(
index, wechat.protobuf.SpamDataBodyMessage.FileInfo.getDefaultInstance());
}
/**
* repeated .wechat_proto.FileInfo appFileInfo = 46;
*/
public java.util.List
getAppFileInfoBuilderList() {
return getAppFileInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SpamDataBodyMessage.FileInfo, wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder, wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder>
getAppFileInfoFieldBuilder() {
if (appFileInfoBuilder_ == null) {
appFileInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SpamDataBodyMessage.FileInfo, wechat.protobuf.SpamDataBodyMessage.FileInfo.Builder, wechat.protobuf.SpamDataBodyMessage.FileInfoOrBuilder>(
appFileInfo_,
((bitField1_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
appFileInfo_ = null;
}
return appFileInfoBuilder_;
}
private java.lang.Object unknown12_ = "";
/**
* optional string unknown12 = 47;
* @return Whether the unknown12 field is set.
*/
public boolean hasUnknown12() {
return ((bitField1_ & 0x00000080) != 0);
}
/**
* optional string unknown12 = 47;
* @return The unknown12.
*/
public java.lang.String getUnknown12() {
java.lang.Object ref = unknown12_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unknown12_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string unknown12 = 47;
* @return The bytes for unknown12.
*/
public com.google.protobuf.ByteString
getUnknown12Bytes() {
java.lang.Object ref = unknown12_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unknown12_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string unknown12 = 47;
* @param value The unknown12 to set.
* @return This builder for chaining.
*/
public Builder setUnknown12(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000080;
unknown12_ = value;
onChanged();
return this;
}
/**
* optional string unknown12 = 47;
* @return This builder for chaining.
*/
public Builder clearUnknown12() {
bitField1_ = (bitField1_ & ~0x00000080);
unknown12_ = getDefaultInstance().getUnknown12();
onChanged();
return this;
}
/**
* optional string unknown12 = 47;
* @param value The bytes for unknown12 to set.
* @return This builder for chaining.
*/
public Builder setUnknown12Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000080;
unknown12_ = value;
onChanged();
return this;
}
private int isModify_ ;
/**
* optional int32 isModify = 50;
* @return Whether the isModify field is set.
*/
@java.lang.Override
public boolean hasIsModify() {
return ((bitField1_ & 0x00000100) != 0);
}
/**
* optional int32 isModify = 50;
* @return The isModify.
*/
@java.lang.Override
public int getIsModify() {
return isModify_;
}
/**
* optional int32 isModify = 50;
* @param value The isModify to set.
* @return This builder for chaining.
*/
public Builder setIsModify(int value) {
bitField1_ |= 0x00000100;
isModify_ = value;
onChanged();
return this;
}
/**
* optional int32 isModify = 50;
* @return This builder for chaining.
*/
public Builder clearIsModify() {
bitField1_ = (bitField1_ & ~0x00000100);
isModify_ = 0;
onChanged();
return this;
}
private java.lang.Object modifyMD5_ = "";
/**
* optional string modifyMD5 = 51;
* @return Whether the modifyMD5 field is set.
*/
public boolean hasModifyMD5() {
return ((bitField1_ & 0x00000200) != 0);
}
/**
* optional string modifyMD5 = 51;
* @return The modifyMD5.
*/
public java.lang.String getModifyMD5() {
java.lang.Object ref = modifyMD5_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
modifyMD5_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string modifyMD5 = 51;
* @return The bytes for modifyMD5.
*/
public com.google.protobuf.ByteString
getModifyMD5Bytes() {
java.lang.Object ref = modifyMD5_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
modifyMD5_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string modifyMD5 = 51;
* @param value The modifyMD5 to set.
* @return This builder for chaining.
*/
public Builder setModifyMD5(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000200;
modifyMD5_ = value;
onChanged();
return this;
}
/**
* optional string modifyMD5 = 51;
* @return This builder for chaining.
*/
public Builder clearModifyMD5() {
bitField1_ = (bitField1_ & ~0x00000200);
modifyMD5_ = getDefaultInstance().getModifyMD5();
onChanged();
return this;
}
/**
* optional string modifyMD5 = 51;
* @param value The bytes for modifyMD5 to set.
* @return This builder for chaining.
*/
public Builder setModifyMD5Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000200;
modifyMD5_ = value;
onChanged();
return this;
}
private long rqtHash_ ;
/**
* optional int64 rqtHash = 52;
* @return Whether the rqtHash field is set.
*/
@java.lang.Override
public boolean hasRqtHash() {
return ((bitField1_ & 0x00000400) != 0);
}
/**
* optional int64 rqtHash = 52;
* @return The rqtHash.
*/
@java.lang.Override
public long getRqtHash() {
return rqtHash_;
}
/**
* optional int64 rqtHash = 52;
* @param value The rqtHash to set.
* @return This builder for chaining.
*/
public Builder setRqtHash(long value) {
bitField1_ |= 0x00000400;
rqtHash_ = value;
onChanged();
return this;
}
/**
* optional int64 rqtHash = 52;
* @return This builder for chaining.
*/
public Builder clearRqtHash() {
bitField1_ = (bitField1_ & ~0x00000400);
rqtHash_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wechat_proto.SpamDataBody)
}
// @@protoc_insertion_point(class_scope:wechat_proto.SpamDataBody)
private static final wechat.protobuf.SpamDataBodyMessage.SpamDataBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new wechat.protobuf.SpamDataBodyMessage.SpamDataBody();
}
public static wechat.protobuf.SpamDataBodyMessage.SpamDataBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SpamDataBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SpamDataBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.SpamDataBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NewClientCheckDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:wechat_proto.NewClientCheckData)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 c32cdata = 1;
* @return Whether the c32cdata field is set.
*/
boolean hasC32Cdata();
/**
* optional int64 c32cdata = 1;
* @return The c32cdata.
*/
long getC32Cdata();
/**
* optional int64 timeStamp = 2;
* @return Whether the timeStamp field is set.
*/
boolean hasTimeStamp();
/**
* optional int64 timeStamp = 2;
* @return The timeStamp.
*/
long getTimeStamp();
/**
* optional bytes databody = 3;
* @return Whether the databody field is set.
*/
boolean hasDatabody();
/**
* optional bytes databody = 3;
* @return The databody.
*/
com.google.protobuf.ByteString getDatabody();
}
/**
* Protobuf type {@code wechat_proto.NewClientCheckData}
*/
public static final class NewClientCheckData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wechat_proto.NewClientCheckData)
NewClientCheckDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use NewClientCheckData.newBuilder() to construct.
private NewClientCheckData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NewClientCheckData() {
databody_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new NewClientCheckData();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NewClientCheckData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
c32Cdata_ = input.readInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
timeStamp_ = input.readInt64();
break;
}
case 26: {
bitField0_ |= 0x00000004;
databody_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_NewClientCheckData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_NewClientCheckData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.SpamDataBodyMessage.NewClientCheckData.class, wechat.protobuf.SpamDataBodyMessage.NewClientCheckData.Builder.class);
}
private int bitField0_;
public static final int C32CDATA_FIELD_NUMBER = 1;
private long c32Cdata_;
/**
* optional int64 c32cdata = 1;
* @return Whether the c32cdata field is set.
*/
@java.lang.Override
public boolean hasC32Cdata() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 c32cdata = 1;
* @return The c32cdata.
*/
@java.lang.Override
public long getC32Cdata() {
return c32Cdata_;
}
public static final int TIMESTAMP_FIELD_NUMBER = 2;
private long timeStamp_;
/**
* optional int64 timeStamp = 2;
* @return Whether the timeStamp field is set.
*/
@java.lang.Override
public boolean hasTimeStamp() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 timeStamp = 2;
* @return The timeStamp.
*/
@java.lang.Override
public long getTimeStamp() {
return timeStamp_;
}
public static final int DATABODY_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString databody_;
/**
* optional bytes databody = 3;
* @return Whether the databody field is set.
*/
@java.lang.Override
public boolean hasDatabody() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes databody = 3;
* @return The databody.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDatabody() {
return databody_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, c32Cdata_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, timeStamp_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, databody_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, c32Cdata_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, timeStamp_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, databody_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof wechat.protobuf.SpamDataBodyMessage.NewClientCheckData)) {
return super.equals(obj);
}
wechat.protobuf.SpamDataBodyMessage.NewClientCheckData other = (wechat.protobuf.SpamDataBodyMessage.NewClientCheckData) obj;
if (hasC32Cdata() != other.hasC32Cdata()) return false;
if (hasC32Cdata()) {
if (getC32Cdata()
!= other.getC32Cdata()) return false;
}
if (hasTimeStamp() != other.hasTimeStamp()) return false;
if (hasTimeStamp()) {
if (getTimeStamp()
!= other.getTimeStamp()) return false;
}
if (hasDatabody() != other.hasDatabody()) return false;
if (hasDatabody()) {
if (!getDatabody()
.equals(other.getDatabody())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasC32Cdata()) {
hash = (37 * hash) + C32CDATA_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getC32Cdata());
}
if (hasTimeStamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeStamp());
}
if (hasDatabody()) {
hash = (37 * hash) + DATABODY_FIELD_NUMBER;
hash = (53 * hash) + getDatabody().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(wechat.protobuf.SpamDataBodyMessage.NewClientCheckData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wechat_proto.NewClientCheckData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wechat_proto.NewClientCheckData)
wechat.protobuf.SpamDataBodyMessage.NewClientCheckDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_NewClientCheckData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_NewClientCheckData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.SpamDataBodyMessage.NewClientCheckData.class, wechat.protobuf.SpamDataBodyMessage.NewClientCheckData.Builder.class);
}
// Construct using wechat.protobuf.SpamDataBodyMessage.NewClientCheckData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
c32Cdata_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
timeStamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
databody_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return wechat.protobuf.SpamDataBodyMessage.internal_static_wechat_proto_NewClientCheckData_descriptor;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.NewClientCheckData getDefaultInstanceForType() {
return wechat.protobuf.SpamDataBodyMessage.NewClientCheckData.getDefaultInstance();
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.NewClientCheckData build() {
wechat.protobuf.SpamDataBodyMessage.NewClientCheckData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.NewClientCheckData buildPartial() {
wechat.protobuf.SpamDataBodyMessage.NewClientCheckData result = new wechat.protobuf.SpamDataBodyMessage.NewClientCheckData(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.c32Cdata_ = c32Cdata_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.timeStamp_ = timeStamp_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.databody_ = databody_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof wechat.protobuf.SpamDataBodyMessage.NewClientCheckData) {
return mergeFrom((wechat.protobuf.SpamDataBodyMessage.NewClientCheckData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(wechat.protobuf.SpamDataBodyMessage.NewClientCheckData other) {
if (other == wechat.protobuf.SpamDataBodyMessage.NewClientCheckData.getDefaultInstance()) return this;
if (other.hasC32Cdata()) {
setC32Cdata(other.getC32Cdata());
}
if (other.hasTimeStamp()) {
setTimeStamp(other.getTimeStamp());
}
if (other.hasDatabody()) {
setDatabody(other.getDatabody());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
wechat.protobuf.SpamDataBodyMessage.NewClientCheckData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (wechat.protobuf.SpamDataBodyMessage.NewClientCheckData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long c32Cdata_ ;
/**
* optional int64 c32cdata = 1;
* @return Whether the c32cdata field is set.
*/
@java.lang.Override
public boolean hasC32Cdata() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 c32cdata = 1;
* @return The c32cdata.
*/
@java.lang.Override
public long getC32Cdata() {
return c32Cdata_;
}
/**
* optional int64 c32cdata = 1;
* @param value The c32cdata to set.
* @return This builder for chaining.
*/
public Builder setC32Cdata(long value) {
bitField0_ |= 0x00000001;
c32Cdata_ = value;
onChanged();
return this;
}
/**
* optional int64 c32cdata = 1;
* @return This builder for chaining.
*/
public Builder clearC32Cdata() {
bitField0_ = (bitField0_ & ~0x00000001);
c32Cdata_ = 0L;
onChanged();
return this;
}
private long timeStamp_ ;
/**
* optional int64 timeStamp = 2;
* @return Whether the timeStamp field is set.
*/
@java.lang.Override
public boolean hasTimeStamp() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 timeStamp = 2;
* @return The timeStamp.
*/
@java.lang.Override
public long getTimeStamp() {
return timeStamp_;
}
/**
* optional int64 timeStamp = 2;
* @param value The timeStamp to set.
* @return This builder for chaining.
*/
public Builder setTimeStamp(long value) {
bitField0_ |= 0x00000002;
timeStamp_ = value;
onChanged();
return this;
}
/**
* optional int64 timeStamp = 2;
* @return This builder for chaining.
*/
public Builder clearTimeStamp() {
bitField0_ = (bitField0_ & ~0x00000002);
timeStamp_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString databody_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes databody = 3;
* @return Whether the databody field is set.
*/
@java.lang.Override
public boolean hasDatabody() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes databody = 3;
* @return The databody.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDatabody() {
return databody_;
}
/**
* optional bytes databody = 3;
* @param value The databody to set.
* @return This builder for chaining.
*/
public Builder setDatabody(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
databody_ = value;
onChanged();
return this;
}
/**
* optional bytes databody = 3;
* @return This builder for chaining.
*/
public Builder clearDatabody() {
bitField0_ = (bitField0_ & ~0x00000004);
databody_ = getDefaultInstance().getDatabody();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wechat_proto.NewClientCheckData)
}
// @@protoc_insertion_point(class_scope:wechat_proto.NewClientCheckData)
private static final wechat.protobuf.SpamDataBodyMessage.NewClientCheckData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new wechat.protobuf.SpamDataBodyMessage.NewClientCheckData();
}
public static wechat.protobuf.SpamDataBodyMessage.NewClientCheckData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NewClientCheckData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NewClientCheckData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public wechat.protobuf.SpamDataBodyMessage.NewClientCheckData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_wechat_proto_FileInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_wechat_proto_FileInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_wechat_proto_SpamDataBody_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_wechat_proto_SpamDataBody_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_wechat_proto_NewClientCheckData_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_wechat_proto_NewClientCheckData_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\031SpamDataBodyMessage.proto\022\014wechat_prot" +
"o\".\n\010FileInfo\022\020\n\010filepath\030\001 \001(\t\022\020\n\010fileu" +
"uid\030\002 \001(\t\"\311\006\n\014SpamDataBody\022\020\n\010unKnown1\030\001" +
" \001(\005\022\021\n\ttimeStamp\030\002 \001(\005\022\017\n\007keyHash\030\003 \001(\005" +
"\022\014\n\004yes1\030\013 \001(\t\022\014\n\004yes2\030\014 \001(\t\022\022\n\niosVersi" +
"on\030\r \001(\t\022\022\n\ndeviceType\030\016 \001(\t\022\020\n\010unKnown2" +
"\030\017 \001(\005\022\033\n\023identifierForVendor\030\020 \001(\t\022\035\n\025a" +
"dvertisingIdentifier\030\021 \001(\t\022\017\n\007carrier\030\022 " +
"\001(\t\022\023\n\013batteryInfo\030\023 \001(\005\022\023\n\013networkName\030" +
"\024 \001(\t\022\017\n\007netType\030\025 \001(\005\022\023\n\013appBundleId\030\026 " +
"\001(\t\022\022\n\ndeviceName\030\027 \001(\t\022\020\n\010userName\030\030 \001(" +
"\t\022\020\n\010unknown3\030\031 \001(\003\022\020\n\010unknown4\030\032 \001(\003\022\020\n" +
"\010unknown5\030\033 \001(\005\022\020\n\010unknown6\030\034 \001(\005\022\014\n\004lan" +
"g\030\035 \001(\t\022\017\n\007country\030\036 \001(\t\022\020\n\010unknown7\030\037 \001" +
"(\005\022\023\n\013documentDir\030 \001(\t\022\020\n\010unknown8\030! \001(" +
"\005\022\020\n\010unknown9\030\" \001(\005\022\017\n\007headMD5\030# \001(\t\022\017\n\007" +
"appUUID\030$ \001(\t\022\022\n\nsyslogUUID\030% \001(\t\022\021\n\tunk" +
"nown10\030& \001(\t\022\021\n\tunknown11\030\' \001(\t\022\017\n\007appNa" +
"me\030( \001(\t\022\017\n\007sshPath\030) \001(\t\022\020\n\010tempTest\030* " +
"\001(\t\022\016\n\006devMD5\030+ \001(\t\022\017\n\007devUser\030, \001(\t\022\021\n\t" +
"devPrefix\030- \001(\t\022+\n\013appFileInfo\030. \003(\0132\026.w" +
"echat_proto.FileInfo\022\021\n\tunknown12\030/ \001(\t\022" +
"\020\n\010isModify\0302 \001(\005\022\021\n\tmodifyMD5\0303 \001(\t\022\017\n\007" +
"rqtHash\0304 \001(\003\"K\n\022NewClientCheckData\022\020\n\010c" +
"32cdata\030\001 \001(\003\022\021\n\ttimeStamp\030\002 \001(\003\022\020\n\010data" +
"body\030\003 \001(\014B\034\n\017wechat.protobufZ\t../wechat"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_wechat_proto_FileInfo_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_wechat_proto_FileInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_wechat_proto_FileInfo_descriptor,
new java.lang.String[] { "Filepath", "Fileuuid", });
internal_static_wechat_proto_SpamDataBody_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_wechat_proto_SpamDataBody_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_wechat_proto_SpamDataBody_descriptor,
new java.lang.String[] { "UnKnown1", "TimeStamp", "KeyHash", "Yes1", "Yes2", "IosVersion", "DeviceType", "UnKnown2", "IdentifierForVendor", "AdvertisingIdentifier", "Carrier", "BatteryInfo", "NetworkName", "NetType", "AppBundleId", "DeviceName", "UserName", "Unknown3", "Unknown4", "Unknown5", "Unknown6", "Lang", "Country", "Unknown7", "DocumentDir", "Unknown8", "Unknown9", "HeadMD5", "AppUUID", "SyslogUUID", "Unknown10", "Unknown11", "AppName", "SshPath", "TempTest", "DevMD5", "DevUser", "DevPrefix", "AppFileInfo", "Unknown12", "IsModify", "ModifyMD5", "RqtHash", });
internal_static_wechat_proto_NewClientCheckData_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_wechat_proto_NewClientCheckData_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_wechat_proto_NewClientCheckData_descriptor,
new java.lang.String[] { "C32Cdata", "TimeStamp", "Databody", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy