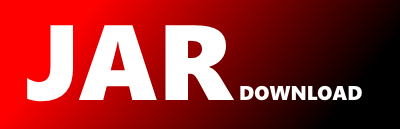
wechat.protobuf.GetContactRequest Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: wechat.proto
package wechat.protobuf;
/**
* Protobuf type {@code wechat_proto.GetContactRequest}
*/
public final class GetContactRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wechat_proto.GetContactRequest)
GetContactRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetContactRequest.newBuilder() to construct.
private GetContactRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetContactRequest() {
userNameList_ = java.util.Collections.emptyList();
antispamTicket_ = java.util.Collections.emptyList();
fromChatRoom_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetContactRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetContactRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
wechat.protobuf.BaseRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = baseRequest_.toBuilder();
}
baseRequest_ = input.readMessage(wechat.protobuf.BaseRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(baseRequest_);
baseRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
bitField0_ |= 0x00000002;
userNum_ = input.readUInt32();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
userNameList_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
userNameList_.add(
input.readMessage(wechat.protobuf.SKBuiltinString.PARSER, extensionRegistry));
break;
}
case 32: {
bitField0_ |= 0x00000004;
antispamTicketNum_ = input.readUInt32();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
antispamTicket_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
antispamTicket_.add(
input.readMessage(wechat.protobuf.SKBuiltinString.PARSER, extensionRegistry));
break;
}
case 48: {
bitField0_ |= 0x00000008;
fromChatRoomNum_ = input.readUInt32();
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
fromChatRoom_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
fromChatRoom_.add(
input.readMessage(wechat.protobuf.SKBuiltinString.PARSER, extensionRegistry));
break;
}
case 64: {
bitField0_ |= 0x00000010;
getContactScene_ = input.readUInt32();
break;
}
case 74: {
wechat.protobuf.SKBuiltinString.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) != 0)) {
subBuilder = chatRoomAccessVerifyTicket_.toBuilder();
}
chatRoomAccessVerifyTicket_ = input.readMessage(wechat.protobuf.SKBuiltinString.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(chatRoomAccessVerifyTicket_);
chatRoomAccessVerifyTicket_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
userNameList_ = java.util.Collections.unmodifiableList(userNameList_);
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
antispamTicket_ = java.util.Collections.unmodifiableList(antispamTicket_);
}
if (((mutable_bitField0_ & 0x00000040) != 0)) {
fromChatRoom_ = java.util.Collections.unmodifiableList(fromChatRoom_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.Wechat.internal_static_wechat_proto_GetContactRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.Wechat.internal_static_wechat_proto_GetContactRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.GetContactRequest.class, wechat.protobuf.GetContactRequest.Builder.class);
}
private int bitField0_;
public static final int BASEREQUEST_FIELD_NUMBER = 1;
private wechat.protobuf.BaseRequest baseRequest_;
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
* @return Whether the baseRequest field is set.
*/
@java.lang.Override
public boolean hasBaseRequest() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
* @return The baseRequest.
*/
@java.lang.Override
public wechat.protobuf.BaseRequest getBaseRequest() {
return baseRequest_ == null ? wechat.protobuf.BaseRequest.getDefaultInstance() : baseRequest_;
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
@java.lang.Override
public wechat.protobuf.BaseRequestOrBuilder getBaseRequestOrBuilder() {
return baseRequest_ == null ? wechat.protobuf.BaseRequest.getDefaultInstance() : baseRequest_;
}
public static final int USERNUM_FIELD_NUMBER = 2;
private int userNum_;
/**
* optional uint32 userNum = 2;
* @return Whether the userNum field is set.
*/
@java.lang.Override
public boolean hasUserNum() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional uint32 userNum = 2;
* @return The userNum.
*/
@java.lang.Override
public int getUserNum() {
return userNum_;
}
public static final int USERNAMELIST_FIELD_NUMBER = 3;
private java.util.List userNameList_;
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
@java.lang.Override
public java.util.List getUserNameListList() {
return userNameList_;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
@java.lang.Override
public java.util.List extends wechat.protobuf.SKBuiltinStringOrBuilder>
getUserNameListOrBuilderList() {
return userNameList_;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
@java.lang.Override
public int getUserNameListCount() {
return userNameList_.size();
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinString getUserNameList(int index) {
return userNameList_.get(index);
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinStringOrBuilder getUserNameListOrBuilder(
int index) {
return userNameList_.get(index);
}
public static final int ANTISPAMTICKETNUM_FIELD_NUMBER = 4;
private int antispamTicketNum_;
/**
* optional uint32 antispamTicketNum = 4;
* @return Whether the antispamTicketNum field is set.
*/
@java.lang.Override
public boolean hasAntispamTicketNum() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional uint32 antispamTicketNum = 4;
* @return The antispamTicketNum.
*/
@java.lang.Override
public int getAntispamTicketNum() {
return antispamTicketNum_;
}
public static final int ANTISPAMTICKET_FIELD_NUMBER = 5;
private java.util.List antispamTicket_;
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
@java.lang.Override
public java.util.List getAntispamTicketList() {
return antispamTicket_;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
@java.lang.Override
public java.util.List extends wechat.protobuf.SKBuiltinStringOrBuilder>
getAntispamTicketOrBuilderList() {
return antispamTicket_;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
@java.lang.Override
public int getAntispamTicketCount() {
return antispamTicket_.size();
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinString getAntispamTicket(int index) {
return antispamTicket_.get(index);
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinStringOrBuilder getAntispamTicketOrBuilder(
int index) {
return antispamTicket_.get(index);
}
public static final int FROMCHATROOMNUM_FIELD_NUMBER = 6;
private int fromChatRoomNum_;
/**
* optional uint32 fromChatRoomNum = 6;
* @return Whether the fromChatRoomNum field is set.
*/
@java.lang.Override
public boolean hasFromChatRoomNum() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional uint32 fromChatRoomNum = 6;
* @return The fromChatRoomNum.
*/
@java.lang.Override
public int getFromChatRoomNum() {
return fromChatRoomNum_;
}
public static final int FROMCHATROOM_FIELD_NUMBER = 7;
private java.util.List fromChatRoom_;
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
@java.lang.Override
public java.util.List getFromChatRoomList() {
return fromChatRoom_;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
@java.lang.Override
public java.util.List extends wechat.protobuf.SKBuiltinStringOrBuilder>
getFromChatRoomOrBuilderList() {
return fromChatRoom_;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
@java.lang.Override
public int getFromChatRoomCount() {
return fromChatRoom_.size();
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinString getFromChatRoom(int index) {
return fromChatRoom_.get(index);
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinStringOrBuilder getFromChatRoomOrBuilder(
int index) {
return fromChatRoom_.get(index);
}
public static final int GETCONTACTSCENE_FIELD_NUMBER = 8;
private int getContactScene_;
/**
* optional uint32 getContactScene = 8;
* @return Whether the getContactScene field is set.
*/
@java.lang.Override
public boolean hasGetContactScene() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional uint32 getContactScene = 8;
* @return The getContactScene.
*/
@java.lang.Override
public int getGetContactScene() {
return getContactScene_;
}
public static final int CHATROOMACCESSVERIFYTICKET_FIELD_NUMBER = 9;
private wechat.protobuf.SKBuiltinString chatRoomAccessVerifyTicket_;
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
* @return Whether the chatRoomAccessVerifyTicket field is set.
*/
@java.lang.Override
public boolean hasChatRoomAccessVerifyTicket() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
* @return The chatRoomAccessVerifyTicket.
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinString getChatRoomAccessVerifyTicket() {
return chatRoomAccessVerifyTicket_ == null ? wechat.protobuf.SKBuiltinString.getDefaultInstance() : chatRoomAccessVerifyTicket_;
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
@java.lang.Override
public wechat.protobuf.SKBuiltinStringOrBuilder getChatRoomAccessVerifyTicketOrBuilder() {
return chatRoomAccessVerifyTicket_ == null ? wechat.protobuf.SKBuiltinString.getDefaultInstance() : chatRoomAccessVerifyTicket_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getBaseRequest());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeUInt32(2, userNum_);
}
for (int i = 0; i < userNameList_.size(); i++) {
output.writeMessage(3, userNameList_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeUInt32(4, antispamTicketNum_);
}
for (int i = 0; i < antispamTicket_.size(); i++) {
output.writeMessage(5, antispamTicket_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeUInt32(6, fromChatRoomNum_);
}
for (int i = 0; i < fromChatRoom_.size(); i++) {
output.writeMessage(7, fromChatRoom_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeUInt32(8, getContactScene_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(9, getChatRoomAccessVerifyTicket());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getBaseRequest());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, userNum_);
}
for (int i = 0; i < userNameList_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, userNameList_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, antispamTicketNum_);
}
for (int i = 0; i < antispamTicket_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, antispamTicket_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, fromChatRoomNum_);
}
for (int i = 0; i < fromChatRoom_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, fromChatRoom_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(8, getContactScene_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getChatRoomAccessVerifyTicket());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof wechat.protobuf.GetContactRequest)) {
return super.equals(obj);
}
wechat.protobuf.GetContactRequest other = (wechat.protobuf.GetContactRequest) obj;
if (hasBaseRequest() != other.hasBaseRequest()) return false;
if (hasBaseRequest()) {
if (!getBaseRequest()
.equals(other.getBaseRequest())) return false;
}
if (hasUserNum() != other.hasUserNum()) return false;
if (hasUserNum()) {
if (getUserNum()
!= other.getUserNum()) return false;
}
if (!getUserNameListList()
.equals(other.getUserNameListList())) return false;
if (hasAntispamTicketNum() != other.hasAntispamTicketNum()) return false;
if (hasAntispamTicketNum()) {
if (getAntispamTicketNum()
!= other.getAntispamTicketNum()) return false;
}
if (!getAntispamTicketList()
.equals(other.getAntispamTicketList())) return false;
if (hasFromChatRoomNum() != other.hasFromChatRoomNum()) return false;
if (hasFromChatRoomNum()) {
if (getFromChatRoomNum()
!= other.getFromChatRoomNum()) return false;
}
if (!getFromChatRoomList()
.equals(other.getFromChatRoomList())) return false;
if (hasGetContactScene() != other.hasGetContactScene()) return false;
if (hasGetContactScene()) {
if (getGetContactScene()
!= other.getGetContactScene()) return false;
}
if (hasChatRoomAccessVerifyTicket() != other.hasChatRoomAccessVerifyTicket()) return false;
if (hasChatRoomAccessVerifyTicket()) {
if (!getChatRoomAccessVerifyTicket()
.equals(other.getChatRoomAccessVerifyTicket())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasBaseRequest()) {
hash = (37 * hash) + BASEREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getBaseRequest().hashCode();
}
if (hasUserNum()) {
hash = (37 * hash) + USERNUM_FIELD_NUMBER;
hash = (53 * hash) + getUserNum();
}
if (getUserNameListCount() > 0) {
hash = (37 * hash) + USERNAMELIST_FIELD_NUMBER;
hash = (53 * hash) + getUserNameListList().hashCode();
}
if (hasAntispamTicketNum()) {
hash = (37 * hash) + ANTISPAMTICKETNUM_FIELD_NUMBER;
hash = (53 * hash) + getAntispamTicketNum();
}
if (getAntispamTicketCount() > 0) {
hash = (37 * hash) + ANTISPAMTICKET_FIELD_NUMBER;
hash = (53 * hash) + getAntispamTicketList().hashCode();
}
if (hasFromChatRoomNum()) {
hash = (37 * hash) + FROMCHATROOMNUM_FIELD_NUMBER;
hash = (53 * hash) + getFromChatRoomNum();
}
if (getFromChatRoomCount() > 0) {
hash = (37 * hash) + FROMCHATROOM_FIELD_NUMBER;
hash = (53 * hash) + getFromChatRoomList().hashCode();
}
if (hasGetContactScene()) {
hash = (37 * hash) + GETCONTACTSCENE_FIELD_NUMBER;
hash = (53 * hash) + getGetContactScene();
}
if (hasChatRoomAccessVerifyTicket()) {
hash = (37 * hash) + CHATROOMACCESSVERIFYTICKET_FIELD_NUMBER;
hash = (53 * hash) + getChatRoomAccessVerifyTicket().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static wechat.protobuf.GetContactRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.GetContactRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.GetContactRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.GetContactRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.GetContactRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static wechat.protobuf.GetContactRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static wechat.protobuf.GetContactRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.GetContactRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.GetContactRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static wechat.protobuf.GetContactRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static wechat.protobuf.GetContactRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static wechat.protobuf.GetContactRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(wechat.protobuf.GetContactRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wechat_proto.GetContactRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wechat_proto.GetContactRequest)
wechat.protobuf.GetContactRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return wechat.protobuf.Wechat.internal_static_wechat_proto_GetContactRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return wechat.protobuf.Wechat.internal_static_wechat_proto_GetContactRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
wechat.protobuf.GetContactRequest.class, wechat.protobuf.GetContactRequest.Builder.class);
}
// Construct using wechat.protobuf.GetContactRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getBaseRequestFieldBuilder();
getUserNameListFieldBuilder();
getAntispamTicketFieldBuilder();
getFromChatRoomFieldBuilder();
getChatRoomAccessVerifyTicketFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (baseRequestBuilder_ == null) {
baseRequest_ = null;
} else {
baseRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
userNum_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
if (userNameListBuilder_ == null) {
userNameList_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
userNameListBuilder_.clear();
}
antispamTicketNum_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
if (antispamTicketBuilder_ == null) {
antispamTicket_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
antispamTicketBuilder_.clear();
}
fromChatRoomNum_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
if (fromChatRoomBuilder_ == null) {
fromChatRoom_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
fromChatRoomBuilder_.clear();
}
getContactScene_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
if (chatRoomAccessVerifyTicketBuilder_ == null) {
chatRoomAccessVerifyTicket_ = null;
} else {
chatRoomAccessVerifyTicketBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return wechat.protobuf.Wechat.internal_static_wechat_proto_GetContactRequest_descriptor;
}
@java.lang.Override
public wechat.protobuf.GetContactRequest getDefaultInstanceForType() {
return wechat.protobuf.GetContactRequest.getDefaultInstance();
}
@java.lang.Override
public wechat.protobuf.GetContactRequest build() {
wechat.protobuf.GetContactRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public wechat.protobuf.GetContactRequest buildPartial() {
wechat.protobuf.GetContactRequest result = new wechat.protobuf.GetContactRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (baseRequestBuilder_ == null) {
result.baseRequest_ = baseRequest_;
} else {
result.baseRequest_ = baseRequestBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.userNum_ = userNum_;
to_bitField0_ |= 0x00000002;
}
if (userNameListBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
userNameList_ = java.util.Collections.unmodifiableList(userNameList_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.userNameList_ = userNameList_;
} else {
result.userNameList_ = userNameListBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.antispamTicketNum_ = antispamTicketNum_;
to_bitField0_ |= 0x00000004;
}
if (antispamTicketBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
antispamTicket_ = java.util.Collections.unmodifiableList(antispamTicket_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.antispamTicket_ = antispamTicket_;
} else {
result.antispamTicket_ = antispamTicketBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.fromChatRoomNum_ = fromChatRoomNum_;
to_bitField0_ |= 0x00000008;
}
if (fromChatRoomBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
fromChatRoom_ = java.util.Collections.unmodifiableList(fromChatRoom_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.fromChatRoom_ = fromChatRoom_;
} else {
result.fromChatRoom_ = fromChatRoomBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.getContactScene_ = getContactScene_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
if (chatRoomAccessVerifyTicketBuilder_ == null) {
result.chatRoomAccessVerifyTicket_ = chatRoomAccessVerifyTicket_;
} else {
result.chatRoomAccessVerifyTicket_ = chatRoomAccessVerifyTicketBuilder_.build();
}
to_bitField0_ |= 0x00000020;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof wechat.protobuf.GetContactRequest) {
return mergeFrom((wechat.protobuf.GetContactRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(wechat.protobuf.GetContactRequest other) {
if (other == wechat.protobuf.GetContactRequest.getDefaultInstance()) return this;
if (other.hasBaseRequest()) {
mergeBaseRequest(other.getBaseRequest());
}
if (other.hasUserNum()) {
setUserNum(other.getUserNum());
}
if (userNameListBuilder_ == null) {
if (!other.userNameList_.isEmpty()) {
if (userNameList_.isEmpty()) {
userNameList_ = other.userNameList_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureUserNameListIsMutable();
userNameList_.addAll(other.userNameList_);
}
onChanged();
}
} else {
if (!other.userNameList_.isEmpty()) {
if (userNameListBuilder_.isEmpty()) {
userNameListBuilder_.dispose();
userNameListBuilder_ = null;
userNameList_ = other.userNameList_;
bitField0_ = (bitField0_ & ~0x00000004);
userNameListBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUserNameListFieldBuilder() : null;
} else {
userNameListBuilder_.addAllMessages(other.userNameList_);
}
}
}
if (other.hasAntispamTicketNum()) {
setAntispamTicketNum(other.getAntispamTicketNum());
}
if (antispamTicketBuilder_ == null) {
if (!other.antispamTicket_.isEmpty()) {
if (antispamTicket_.isEmpty()) {
antispamTicket_ = other.antispamTicket_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureAntispamTicketIsMutable();
antispamTicket_.addAll(other.antispamTicket_);
}
onChanged();
}
} else {
if (!other.antispamTicket_.isEmpty()) {
if (antispamTicketBuilder_.isEmpty()) {
antispamTicketBuilder_.dispose();
antispamTicketBuilder_ = null;
antispamTicket_ = other.antispamTicket_;
bitField0_ = (bitField0_ & ~0x00000010);
antispamTicketBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAntispamTicketFieldBuilder() : null;
} else {
antispamTicketBuilder_.addAllMessages(other.antispamTicket_);
}
}
}
if (other.hasFromChatRoomNum()) {
setFromChatRoomNum(other.getFromChatRoomNum());
}
if (fromChatRoomBuilder_ == null) {
if (!other.fromChatRoom_.isEmpty()) {
if (fromChatRoom_.isEmpty()) {
fromChatRoom_ = other.fromChatRoom_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureFromChatRoomIsMutable();
fromChatRoom_.addAll(other.fromChatRoom_);
}
onChanged();
}
} else {
if (!other.fromChatRoom_.isEmpty()) {
if (fromChatRoomBuilder_.isEmpty()) {
fromChatRoomBuilder_.dispose();
fromChatRoomBuilder_ = null;
fromChatRoom_ = other.fromChatRoom_;
bitField0_ = (bitField0_ & ~0x00000040);
fromChatRoomBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFromChatRoomFieldBuilder() : null;
} else {
fromChatRoomBuilder_.addAllMessages(other.fromChatRoom_);
}
}
}
if (other.hasGetContactScene()) {
setGetContactScene(other.getGetContactScene());
}
if (other.hasChatRoomAccessVerifyTicket()) {
mergeChatRoomAccessVerifyTicket(other.getChatRoomAccessVerifyTicket());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
wechat.protobuf.GetContactRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (wechat.protobuf.GetContactRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private wechat.protobuf.BaseRequest baseRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.BaseRequest, wechat.protobuf.BaseRequest.Builder, wechat.protobuf.BaseRequestOrBuilder> baseRequestBuilder_;
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
* @return Whether the baseRequest field is set.
*/
public boolean hasBaseRequest() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
* @return The baseRequest.
*/
public wechat.protobuf.BaseRequest getBaseRequest() {
if (baseRequestBuilder_ == null) {
return baseRequest_ == null ? wechat.protobuf.BaseRequest.getDefaultInstance() : baseRequest_;
} else {
return baseRequestBuilder_.getMessage();
}
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
public Builder setBaseRequest(wechat.protobuf.BaseRequest value) {
if (baseRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
baseRequest_ = value;
onChanged();
} else {
baseRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
public Builder setBaseRequest(
wechat.protobuf.BaseRequest.Builder builderForValue) {
if (baseRequestBuilder_ == null) {
baseRequest_ = builderForValue.build();
onChanged();
} else {
baseRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
public Builder mergeBaseRequest(wechat.protobuf.BaseRequest value) {
if (baseRequestBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
baseRequest_ != null &&
baseRequest_ != wechat.protobuf.BaseRequest.getDefaultInstance()) {
baseRequest_ =
wechat.protobuf.BaseRequest.newBuilder(baseRequest_).mergeFrom(value).buildPartial();
} else {
baseRequest_ = value;
}
onChanged();
} else {
baseRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
public Builder clearBaseRequest() {
if (baseRequestBuilder_ == null) {
baseRequest_ = null;
onChanged();
} else {
baseRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
public wechat.protobuf.BaseRequest.Builder getBaseRequestBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getBaseRequestFieldBuilder().getBuilder();
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
public wechat.protobuf.BaseRequestOrBuilder getBaseRequestOrBuilder() {
if (baseRequestBuilder_ != null) {
return baseRequestBuilder_.getMessageOrBuilder();
} else {
return baseRequest_ == null ?
wechat.protobuf.BaseRequest.getDefaultInstance() : baseRequest_;
}
}
/**
* optional .wechat_proto.BaseRequest baseRequest = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.BaseRequest, wechat.protobuf.BaseRequest.Builder, wechat.protobuf.BaseRequestOrBuilder>
getBaseRequestFieldBuilder() {
if (baseRequestBuilder_ == null) {
baseRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.BaseRequest, wechat.protobuf.BaseRequest.Builder, wechat.protobuf.BaseRequestOrBuilder>(
getBaseRequest(),
getParentForChildren(),
isClean());
baseRequest_ = null;
}
return baseRequestBuilder_;
}
private int userNum_ ;
/**
* optional uint32 userNum = 2;
* @return Whether the userNum field is set.
*/
@java.lang.Override
public boolean hasUserNum() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional uint32 userNum = 2;
* @return The userNum.
*/
@java.lang.Override
public int getUserNum() {
return userNum_;
}
/**
* optional uint32 userNum = 2;
* @param value The userNum to set.
* @return This builder for chaining.
*/
public Builder setUserNum(int value) {
bitField0_ |= 0x00000002;
userNum_ = value;
onChanged();
return this;
}
/**
* optional uint32 userNum = 2;
* @return This builder for chaining.
*/
public Builder clearUserNum() {
bitField0_ = (bitField0_ & ~0x00000002);
userNum_ = 0;
onChanged();
return this;
}
private java.util.List userNameList_ =
java.util.Collections.emptyList();
private void ensureUserNameListIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
userNameList_ = new java.util.ArrayList(userNameList_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder> userNameListBuilder_;
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public java.util.List getUserNameListList() {
if (userNameListBuilder_ == null) {
return java.util.Collections.unmodifiableList(userNameList_);
} else {
return userNameListBuilder_.getMessageList();
}
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public int getUserNameListCount() {
if (userNameListBuilder_ == null) {
return userNameList_.size();
} else {
return userNameListBuilder_.getCount();
}
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public wechat.protobuf.SKBuiltinString getUserNameList(int index) {
if (userNameListBuilder_ == null) {
return userNameList_.get(index);
} else {
return userNameListBuilder_.getMessage(index);
}
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder setUserNameList(
int index, wechat.protobuf.SKBuiltinString value) {
if (userNameListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserNameListIsMutable();
userNameList_.set(index, value);
onChanged();
} else {
userNameListBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder setUserNameList(
int index, wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (userNameListBuilder_ == null) {
ensureUserNameListIsMutable();
userNameList_.set(index, builderForValue.build());
onChanged();
} else {
userNameListBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder addUserNameList(wechat.protobuf.SKBuiltinString value) {
if (userNameListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserNameListIsMutable();
userNameList_.add(value);
onChanged();
} else {
userNameListBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder addUserNameList(
int index, wechat.protobuf.SKBuiltinString value) {
if (userNameListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserNameListIsMutable();
userNameList_.add(index, value);
onChanged();
} else {
userNameListBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder addUserNameList(
wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (userNameListBuilder_ == null) {
ensureUserNameListIsMutable();
userNameList_.add(builderForValue.build());
onChanged();
} else {
userNameListBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder addUserNameList(
int index, wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (userNameListBuilder_ == null) {
ensureUserNameListIsMutable();
userNameList_.add(index, builderForValue.build());
onChanged();
} else {
userNameListBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder addAllUserNameList(
java.lang.Iterable extends wechat.protobuf.SKBuiltinString> values) {
if (userNameListBuilder_ == null) {
ensureUserNameListIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, userNameList_);
onChanged();
} else {
userNameListBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder clearUserNameList() {
if (userNameListBuilder_ == null) {
userNameList_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
userNameListBuilder_.clear();
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public Builder removeUserNameList(int index) {
if (userNameListBuilder_ == null) {
ensureUserNameListIsMutable();
userNameList_.remove(index);
onChanged();
} else {
userNameListBuilder_.remove(index);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public wechat.protobuf.SKBuiltinString.Builder getUserNameListBuilder(
int index) {
return getUserNameListFieldBuilder().getBuilder(index);
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public wechat.protobuf.SKBuiltinStringOrBuilder getUserNameListOrBuilder(
int index) {
if (userNameListBuilder_ == null) {
return userNameList_.get(index); } else {
return userNameListBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public java.util.List extends wechat.protobuf.SKBuiltinStringOrBuilder>
getUserNameListOrBuilderList() {
if (userNameListBuilder_ != null) {
return userNameListBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(userNameList_);
}
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public wechat.protobuf.SKBuiltinString.Builder addUserNameListBuilder() {
return getUserNameListFieldBuilder().addBuilder(
wechat.protobuf.SKBuiltinString.getDefaultInstance());
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public wechat.protobuf.SKBuiltinString.Builder addUserNameListBuilder(
int index) {
return getUserNameListFieldBuilder().addBuilder(
index, wechat.protobuf.SKBuiltinString.getDefaultInstance());
}
/**
* repeated .wechat_proto.SKBuiltinString userNameList = 3;
*/
public java.util.List
getUserNameListBuilderList() {
return getUserNameListFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>
getUserNameListFieldBuilder() {
if (userNameListBuilder_ == null) {
userNameListBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>(
userNameList_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
userNameList_ = null;
}
return userNameListBuilder_;
}
private int antispamTicketNum_ ;
/**
* optional uint32 antispamTicketNum = 4;
* @return Whether the antispamTicketNum field is set.
*/
@java.lang.Override
public boolean hasAntispamTicketNum() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional uint32 antispamTicketNum = 4;
* @return The antispamTicketNum.
*/
@java.lang.Override
public int getAntispamTicketNum() {
return antispamTicketNum_;
}
/**
* optional uint32 antispamTicketNum = 4;
* @param value The antispamTicketNum to set.
* @return This builder for chaining.
*/
public Builder setAntispamTicketNum(int value) {
bitField0_ |= 0x00000008;
antispamTicketNum_ = value;
onChanged();
return this;
}
/**
* optional uint32 antispamTicketNum = 4;
* @return This builder for chaining.
*/
public Builder clearAntispamTicketNum() {
bitField0_ = (bitField0_ & ~0x00000008);
antispamTicketNum_ = 0;
onChanged();
return this;
}
private java.util.List antispamTicket_ =
java.util.Collections.emptyList();
private void ensureAntispamTicketIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
antispamTicket_ = new java.util.ArrayList(antispamTicket_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder> antispamTicketBuilder_;
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public java.util.List getAntispamTicketList() {
if (antispamTicketBuilder_ == null) {
return java.util.Collections.unmodifiableList(antispamTicket_);
} else {
return antispamTicketBuilder_.getMessageList();
}
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public int getAntispamTicketCount() {
if (antispamTicketBuilder_ == null) {
return antispamTicket_.size();
} else {
return antispamTicketBuilder_.getCount();
}
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public wechat.protobuf.SKBuiltinString getAntispamTicket(int index) {
if (antispamTicketBuilder_ == null) {
return antispamTicket_.get(index);
} else {
return antispamTicketBuilder_.getMessage(index);
}
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder setAntispamTicket(
int index, wechat.protobuf.SKBuiltinString value) {
if (antispamTicketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAntispamTicketIsMutable();
antispamTicket_.set(index, value);
onChanged();
} else {
antispamTicketBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder setAntispamTicket(
int index, wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (antispamTicketBuilder_ == null) {
ensureAntispamTicketIsMutable();
antispamTicket_.set(index, builderForValue.build());
onChanged();
} else {
antispamTicketBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder addAntispamTicket(wechat.protobuf.SKBuiltinString value) {
if (antispamTicketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAntispamTicketIsMutable();
antispamTicket_.add(value);
onChanged();
} else {
antispamTicketBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder addAntispamTicket(
int index, wechat.protobuf.SKBuiltinString value) {
if (antispamTicketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAntispamTicketIsMutable();
antispamTicket_.add(index, value);
onChanged();
} else {
antispamTicketBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder addAntispamTicket(
wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (antispamTicketBuilder_ == null) {
ensureAntispamTicketIsMutable();
antispamTicket_.add(builderForValue.build());
onChanged();
} else {
antispamTicketBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder addAntispamTicket(
int index, wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (antispamTicketBuilder_ == null) {
ensureAntispamTicketIsMutable();
antispamTicket_.add(index, builderForValue.build());
onChanged();
} else {
antispamTicketBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder addAllAntispamTicket(
java.lang.Iterable extends wechat.protobuf.SKBuiltinString> values) {
if (antispamTicketBuilder_ == null) {
ensureAntispamTicketIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, antispamTicket_);
onChanged();
} else {
antispamTicketBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder clearAntispamTicket() {
if (antispamTicketBuilder_ == null) {
antispamTicket_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
antispamTicketBuilder_.clear();
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public Builder removeAntispamTicket(int index) {
if (antispamTicketBuilder_ == null) {
ensureAntispamTicketIsMutable();
antispamTicket_.remove(index);
onChanged();
} else {
antispamTicketBuilder_.remove(index);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public wechat.protobuf.SKBuiltinString.Builder getAntispamTicketBuilder(
int index) {
return getAntispamTicketFieldBuilder().getBuilder(index);
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public wechat.protobuf.SKBuiltinStringOrBuilder getAntispamTicketOrBuilder(
int index) {
if (antispamTicketBuilder_ == null) {
return antispamTicket_.get(index); } else {
return antispamTicketBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public java.util.List extends wechat.protobuf.SKBuiltinStringOrBuilder>
getAntispamTicketOrBuilderList() {
if (antispamTicketBuilder_ != null) {
return antispamTicketBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(antispamTicket_);
}
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public wechat.protobuf.SKBuiltinString.Builder addAntispamTicketBuilder() {
return getAntispamTicketFieldBuilder().addBuilder(
wechat.protobuf.SKBuiltinString.getDefaultInstance());
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public wechat.protobuf.SKBuiltinString.Builder addAntispamTicketBuilder(
int index) {
return getAntispamTicketFieldBuilder().addBuilder(
index, wechat.protobuf.SKBuiltinString.getDefaultInstance());
}
/**
* repeated .wechat_proto.SKBuiltinString antispamTicket = 5;
*/
public java.util.List
getAntispamTicketBuilderList() {
return getAntispamTicketFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>
getAntispamTicketFieldBuilder() {
if (antispamTicketBuilder_ == null) {
antispamTicketBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>(
antispamTicket_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
antispamTicket_ = null;
}
return antispamTicketBuilder_;
}
private int fromChatRoomNum_ ;
/**
* optional uint32 fromChatRoomNum = 6;
* @return Whether the fromChatRoomNum field is set.
*/
@java.lang.Override
public boolean hasFromChatRoomNum() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional uint32 fromChatRoomNum = 6;
* @return The fromChatRoomNum.
*/
@java.lang.Override
public int getFromChatRoomNum() {
return fromChatRoomNum_;
}
/**
* optional uint32 fromChatRoomNum = 6;
* @param value The fromChatRoomNum to set.
* @return This builder for chaining.
*/
public Builder setFromChatRoomNum(int value) {
bitField0_ |= 0x00000020;
fromChatRoomNum_ = value;
onChanged();
return this;
}
/**
* optional uint32 fromChatRoomNum = 6;
* @return This builder for chaining.
*/
public Builder clearFromChatRoomNum() {
bitField0_ = (bitField0_ & ~0x00000020);
fromChatRoomNum_ = 0;
onChanged();
return this;
}
private java.util.List fromChatRoom_ =
java.util.Collections.emptyList();
private void ensureFromChatRoomIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
fromChatRoom_ = new java.util.ArrayList(fromChatRoom_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder> fromChatRoomBuilder_;
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public java.util.List getFromChatRoomList() {
if (fromChatRoomBuilder_ == null) {
return java.util.Collections.unmodifiableList(fromChatRoom_);
} else {
return fromChatRoomBuilder_.getMessageList();
}
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public int getFromChatRoomCount() {
if (fromChatRoomBuilder_ == null) {
return fromChatRoom_.size();
} else {
return fromChatRoomBuilder_.getCount();
}
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public wechat.protobuf.SKBuiltinString getFromChatRoom(int index) {
if (fromChatRoomBuilder_ == null) {
return fromChatRoom_.get(index);
} else {
return fromChatRoomBuilder_.getMessage(index);
}
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder setFromChatRoom(
int index, wechat.protobuf.SKBuiltinString value) {
if (fromChatRoomBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFromChatRoomIsMutable();
fromChatRoom_.set(index, value);
onChanged();
} else {
fromChatRoomBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder setFromChatRoom(
int index, wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (fromChatRoomBuilder_ == null) {
ensureFromChatRoomIsMutable();
fromChatRoom_.set(index, builderForValue.build());
onChanged();
} else {
fromChatRoomBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder addFromChatRoom(wechat.protobuf.SKBuiltinString value) {
if (fromChatRoomBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFromChatRoomIsMutable();
fromChatRoom_.add(value);
onChanged();
} else {
fromChatRoomBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder addFromChatRoom(
int index, wechat.protobuf.SKBuiltinString value) {
if (fromChatRoomBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFromChatRoomIsMutable();
fromChatRoom_.add(index, value);
onChanged();
} else {
fromChatRoomBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder addFromChatRoom(
wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (fromChatRoomBuilder_ == null) {
ensureFromChatRoomIsMutable();
fromChatRoom_.add(builderForValue.build());
onChanged();
} else {
fromChatRoomBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder addFromChatRoom(
int index, wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (fromChatRoomBuilder_ == null) {
ensureFromChatRoomIsMutable();
fromChatRoom_.add(index, builderForValue.build());
onChanged();
} else {
fromChatRoomBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder addAllFromChatRoom(
java.lang.Iterable extends wechat.protobuf.SKBuiltinString> values) {
if (fromChatRoomBuilder_ == null) {
ensureFromChatRoomIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fromChatRoom_);
onChanged();
} else {
fromChatRoomBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder clearFromChatRoom() {
if (fromChatRoomBuilder_ == null) {
fromChatRoom_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
fromChatRoomBuilder_.clear();
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public Builder removeFromChatRoom(int index) {
if (fromChatRoomBuilder_ == null) {
ensureFromChatRoomIsMutable();
fromChatRoom_.remove(index);
onChanged();
} else {
fromChatRoomBuilder_.remove(index);
}
return this;
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public wechat.protobuf.SKBuiltinString.Builder getFromChatRoomBuilder(
int index) {
return getFromChatRoomFieldBuilder().getBuilder(index);
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public wechat.protobuf.SKBuiltinStringOrBuilder getFromChatRoomOrBuilder(
int index) {
if (fromChatRoomBuilder_ == null) {
return fromChatRoom_.get(index); } else {
return fromChatRoomBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public java.util.List extends wechat.protobuf.SKBuiltinStringOrBuilder>
getFromChatRoomOrBuilderList() {
if (fromChatRoomBuilder_ != null) {
return fromChatRoomBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fromChatRoom_);
}
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public wechat.protobuf.SKBuiltinString.Builder addFromChatRoomBuilder() {
return getFromChatRoomFieldBuilder().addBuilder(
wechat.protobuf.SKBuiltinString.getDefaultInstance());
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public wechat.protobuf.SKBuiltinString.Builder addFromChatRoomBuilder(
int index) {
return getFromChatRoomFieldBuilder().addBuilder(
index, wechat.protobuf.SKBuiltinString.getDefaultInstance());
}
/**
* repeated .wechat_proto.SKBuiltinString fromChatRoom = 7;
*/
public java.util.List
getFromChatRoomBuilderList() {
return getFromChatRoomFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>
getFromChatRoomFieldBuilder() {
if (fromChatRoomBuilder_ == null) {
fromChatRoomBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>(
fromChatRoom_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
fromChatRoom_ = null;
}
return fromChatRoomBuilder_;
}
private int getContactScene_ ;
/**
* optional uint32 getContactScene = 8;
* @return Whether the getContactScene field is set.
*/
@java.lang.Override
public boolean hasGetContactScene() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional uint32 getContactScene = 8;
* @return The getContactScene.
*/
@java.lang.Override
public int getGetContactScene() {
return getContactScene_;
}
/**
* optional uint32 getContactScene = 8;
* @param value The getContactScene to set.
* @return This builder for chaining.
*/
public Builder setGetContactScene(int value) {
bitField0_ |= 0x00000080;
getContactScene_ = value;
onChanged();
return this;
}
/**
* optional uint32 getContactScene = 8;
* @return This builder for chaining.
*/
public Builder clearGetContactScene() {
bitField0_ = (bitField0_ & ~0x00000080);
getContactScene_ = 0;
onChanged();
return this;
}
private wechat.protobuf.SKBuiltinString chatRoomAccessVerifyTicket_;
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder> chatRoomAccessVerifyTicketBuilder_;
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
* @return Whether the chatRoomAccessVerifyTicket field is set.
*/
public boolean hasChatRoomAccessVerifyTicket() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
* @return The chatRoomAccessVerifyTicket.
*/
public wechat.protobuf.SKBuiltinString getChatRoomAccessVerifyTicket() {
if (chatRoomAccessVerifyTicketBuilder_ == null) {
return chatRoomAccessVerifyTicket_ == null ? wechat.protobuf.SKBuiltinString.getDefaultInstance() : chatRoomAccessVerifyTicket_;
} else {
return chatRoomAccessVerifyTicketBuilder_.getMessage();
}
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
public Builder setChatRoomAccessVerifyTicket(wechat.protobuf.SKBuiltinString value) {
if (chatRoomAccessVerifyTicketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
chatRoomAccessVerifyTicket_ = value;
onChanged();
} else {
chatRoomAccessVerifyTicketBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
public Builder setChatRoomAccessVerifyTicket(
wechat.protobuf.SKBuiltinString.Builder builderForValue) {
if (chatRoomAccessVerifyTicketBuilder_ == null) {
chatRoomAccessVerifyTicket_ = builderForValue.build();
onChanged();
} else {
chatRoomAccessVerifyTicketBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
public Builder mergeChatRoomAccessVerifyTicket(wechat.protobuf.SKBuiltinString value) {
if (chatRoomAccessVerifyTicketBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0) &&
chatRoomAccessVerifyTicket_ != null &&
chatRoomAccessVerifyTicket_ != wechat.protobuf.SKBuiltinString.getDefaultInstance()) {
chatRoomAccessVerifyTicket_ =
wechat.protobuf.SKBuiltinString.newBuilder(chatRoomAccessVerifyTicket_).mergeFrom(value).buildPartial();
} else {
chatRoomAccessVerifyTicket_ = value;
}
onChanged();
} else {
chatRoomAccessVerifyTicketBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
public Builder clearChatRoomAccessVerifyTicket() {
if (chatRoomAccessVerifyTicketBuilder_ == null) {
chatRoomAccessVerifyTicket_ = null;
onChanged();
} else {
chatRoomAccessVerifyTicketBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
public wechat.protobuf.SKBuiltinString.Builder getChatRoomAccessVerifyTicketBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getChatRoomAccessVerifyTicketFieldBuilder().getBuilder();
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
public wechat.protobuf.SKBuiltinStringOrBuilder getChatRoomAccessVerifyTicketOrBuilder() {
if (chatRoomAccessVerifyTicketBuilder_ != null) {
return chatRoomAccessVerifyTicketBuilder_.getMessageOrBuilder();
} else {
return chatRoomAccessVerifyTicket_ == null ?
wechat.protobuf.SKBuiltinString.getDefaultInstance() : chatRoomAccessVerifyTicket_;
}
}
/**
* optional .wechat_proto.SKBuiltinString chatRoomAccessVerifyTicket = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>
getChatRoomAccessVerifyTicketFieldBuilder() {
if (chatRoomAccessVerifyTicketBuilder_ == null) {
chatRoomAccessVerifyTicketBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
wechat.protobuf.SKBuiltinString, wechat.protobuf.SKBuiltinString.Builder, wechat.protobuf.SKBuiltinStringOrBuilder>(
getChatRoomAccessVerifyTicket(),
getParentForChildren(),
isClean());
chatRoomAccessVerifyTicket_ = null;
}
return chatRoomAccessVerifyTicketBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wechat_proto.GetContactRequest)
}
// @@protoc_insertion_point(class_scope:wechat_proto.GetContactRequest)
private static final wechat.protobuf.GetContactRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new wechat.protobuf.GetContactRequest();
}
public static wechat.protobuf.GetContactRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetContactRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetContactRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public wechat.protobuf.GetContactRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy