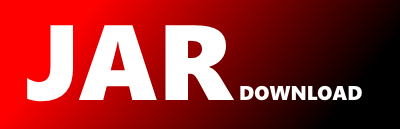
nirvana.support.services.NetworkUtilsSupport.scala Maven / Gradle / Ivy
// Copyright 2014 Jun Tsai. All rights reserved.
// site: http://www.ganshane.com
package nirvana.support.services
import java.net.{UnknownHostException, ServerSocket, InetAddress}
import java.io.IOException
/**
* 针对network的操作类
*/
trait NetworkUtilsSupport {
this:LoggerSupport =>
def hostname: Option[String] = {
var hostname: Option[String] = None
try {
hostname = Some(InetAddress.getLocalHost.getCanonicalHostName)
}
catch {
case e: UnknownHostException => {
logger.error("local_hostname", e)
}
}
return hostname
}
def ip: Option[String] = {
var ip: Option[String] = None
try {
ip = Some(InetAddress.getLocalHost.getHostAddress)
}
catch {
case e: UnknownHostException => {
logger.error("local_hostname", e)
}
}
return ip
}
sealed abstract class PortStatus
case object PortAvailable extends PortStatus
case object PortUnavailable extends PortStatus
/**
* Check whether the port is available to binding
*
* @param port
*/
def tryPort(port: Int): PortStatus= {
var status:PortStatus = PortUnavailable
try{
val socket: ServerSocket = new ServerSocket(port)
val rtn: Int = socket.getLocalPort
socket.close
if(rtn >0)
status = PortAvailable
}catch{
case e:IOException =>
logger.warn("try port "+e.getMessage)
//do nothing
}
return status
}
/**
* get one available port
*
*/
def getAvailablePort: PortStatus= {
return availablePort(0)
}
/**
* Check whether the port is available to binding
*
* @param prefered
* @return -1 means not available, others means available
*/
def availablePort(prefered: Int): PortStatus = {
try {
return tryPort(prefered)
}catch {
case e: IOException => {
}
}
PortUnavailable
}
def host2Ip(host: String): String = {
var address: InetAddress = null
try {
address = InetAddress.getByName(host)
}
catch {
case e: UnknownHostException => {
logger.warn("NetWorkUtil can't transfer hostname(" + host + ") to ip, return hostname", e)
return host
}
}
return address.getHostAddress
}
def ip2Host(ip: String): String = {
var address: InetAddress = null
try {
address = InetAddress.getByName(ip)
}
catch {
case e: UnknownHostException => {
logger.warn("NetWorkUtil can't transfer ip(" + ip + ") to hostname, return ip", e)
return ip
}
}
return address.getHostName
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy