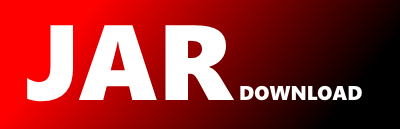
nirvana.support.services.NirvanaUtils.scala Maven / Gradle / Ivy
// Copyright 2014 Jun Tsai. All rights reserved.
// site: http://www.ganshane.com
package nirvana.support.services
import java.io._
import java.util.UUID
import java.util.concurrent.{ExecutorService, TimeUnit}
import org.apache.commons.io.input.ClassLoaderObjectInputStream
/**
* nirvana utils
*/
object NirvanaUtils
extends LoggerSupport
with NetworkUtilsSupport {
def parseBind(bind: String): (String, Int) = {
val arr = bind.split(":")
(arr(0), arr(1).toInt)
}
def isLessThan(a: Array[Byte], b: Array[Byte]): Boolean = {
val len = math.min(a.length, b.length)
0 until len foreach {
case i =>
if (a.apply(i) < b.apply(i))
return true
}
return false
}
def bitXor(a: Long, b: Long): Long = {
return a ^ b
}
def secureRandomLong: Long = {
return UUID.randomUUID.getLeastSignificantBits
}
def currentTimeInSecs: Int = {
(System.currentTimeMillis() / 1000).toInt
}
def serialize(obj: AnyRef): Array[Byte] = {
try {
val bos: ByteArrayOutputStream = new ByteArrayOutputStream
val oos: ObjectOutputStream = new ObjectOutputStream(bos)
oos.writeObject(obj)
oos.close
return bos.toByteArray
}
catch {
case ioe: IOException => {
throw new RuntimeException(ioe)
}
}
}
def deserialize(serialized: Array[Byte]): AnyRef = {
return deserialize(serialized, Thread.currentThread().getContextClassLoader)
}
def deserialize(serialized: Array[Byte], loader: ClassLoader): AnyRef = {
try {
val bis: ByteArrayInputStream = new ByteArrayInputStream(serialized)
var ret: AnyRef = null
if (loader != null) {
val cis: ClassLoaderObjectInputStream = new ClassLoaderObjectInputStream(loader, bis)
ret = cis.readObject
cis.close
}
else {
val ois: ObjectInputStream = new ObjectInputStream(bis)
ret = ois.readObject
ois.close
}
return ret
}
catch {
case ioe: IOException => {
throw new RuntimeException(ioe)
}
case e: ClassNotFoundException => {
throw new RuntimeException(e)
}
}
}
def shutdownExecutor(executor: ExecutorService, executorName: String) {
if (executor == null)
return
executor.shutdown
try {
if (!executor.awaitTermination(2, TimeUnit.SECONDS)) {
executor.shutdownNow
if (!executor.awaitTermination(2, TimeUnit.SECONDS)) {
logger.warn("executor {} not terminated", executorName)
}
}
}
catch {
case ie: InterruptedException => {
executor.shutdownNow
Thread.currentThread.interrupt
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy