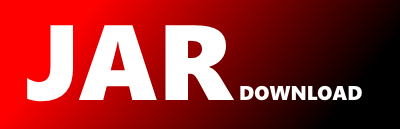
nirvana.support.services.SystemEnvDetectorSupport.scala Maven / Gradle / Ivy
// Copyright 2014 Jun Tsai. All rights reserved.
// site: http://www.ganshane.com
package nirvana.support.services
import org.slf4j.Logger
import java.util;
import java.net.{UnknownHostException, InetAddress}
import java.lang.management.ManagementFactory
/**
* detect system information
*/
trait SystemEnvDetectorSupport {
protected def detectAndPrintSystemEnv(logger:Logger){
val l = new util.ArrayList[Entry]();
try {
put(l,"host.name",InetAddress.getLocalHost.getCanonicalHostName)
} catch {
case e:UnknownHostException =>
put(l,"host.name", "")
}
put(l, "java.version",
System.getProperty("java.version", ""));
put(l, "java.vendor",
System.getProperty("java.vendor", ""));
put(l, "java.home",
System.getProperty("java.home", ""));
put(l, "java.class.path",
System.getProperty("java.class.path", ""));
put(l, "java.library.path",
System.getProperty("java.library.path", ""));
put(l, "java.io.tmpdir",
System.getProperty("java.io.tmpdir", ""));
put(l, "java.compiler",
System.getProperty("java.compiler", ""));
put(l, "os.name",
System.getProperty("os.name", ""));
put(l, "os.arch",
System.getProperty("os.arch", ""));
put(l, "os.version",
System.getProperty("os.version", ""));
put(l, "user.name",
System.getProperty("user.name", ""));
put(l, "user.home",
System.getProperty("user.home", ""));
put(l, "user.dir",
System.getProperty("user.dir", ""));
val it = l.iterator()
while(it.hasNext){
val entry = it.next()
logger.info("{}={}",entry.k,entry.v)
}
try {
val runtimemxBean = ManagementFactory.getRuntimeMXBean
val arguments = runtimemxBean.getInputArguments.toArray.mkString(" ")
logger.info("args={}",arguments)
}catch{
case e:Throwable =>
}
}
private def put(l:util.ArrayList[Entry],k:String,v:String){
l.add(Entry(k,v))
}
case class Entry(k:String,v:String)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy