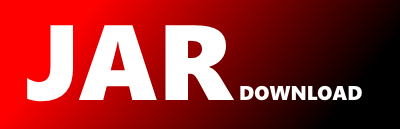
com.garmin.fit.SegmentPointMesg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fit Show documentation
Show all versions of fit Show documentation
The Official Garmin FIT SDK
The newest version!
/////////////////////////////////////////////////////////////////////////////////////////////
// Copyright 2024 Garmin International, Inc.
// Licensed under the Flexible and Interoperable Data Transfer (FIT) Protocol License; you
// may not use this file except in compliance with the Flexible and Interoperable Data
// Transfer (FIT) Protocol License.
/////////////////////////////////////////////////////////////////////////////////////////////
// ****WARNING**** This file is auto-generated! Do NOT edit this file.
// Profile Version = 21.158.0Release
// Tag = production/release/21.158.0-0-gc9428aa
/////////////////////////////////////////////////////////////////////////////////////////////
package com.garmin.fit;
public class SegmentPointMesg extends Mesg {
public static final int MessageIndexFieldNum = 254;
public static final int PositionLatFieldNum = 1;
public static final int PositionLongFieldNum = 2;
public static final int DistanceFieldNum = 3;
public static final int AltitudeFieldNum = 4;
public static final int LeaderTimeFieldNum = 5;
public static final int EnhancedAltitudeFieldNum = 6;
protected static final Mesg segmentPointMesg;
static {int field_index = 0;
// segment_point
segmentPointMesg = new Mesg("segment_point", MesgNum.SEGMENT_POINT);
segmentPointMesg.addField(new Field("message_index", MessageIndexFieldNum, 132, 1, 0, "", false, Profile.Type.MESSAGE_INDEX));
field_index++;
segmentPointMesg.addField(new Field("position_lat", PositionLatFieldNum, 133, 1, 0, "semicircles", false, Profile.Type.SINT32));
field_index++;
segmentPointMesg.addField(new Field("position_long", PositionLongFieldNum, 133, 1, 0, "semicircles", false, Profile.Type.SINT32));
field_index++;
segmentPointMesg.addField(new Field("distance", DistanceFieldNum, 134, 100, 0, "m", false, Profile.Type.UINT32));
field_index++;
segmentPointMesg.addField(new Field("altitude", AltitudeFieldNum, 132, 5, 500, "m", false, Profile.Type.UINT16));
segmentPointMesg.fields.get(field_index).components.add(new FieldComponent(6, false, 16, 5, 500)); // enhanced_altitude
field_index++;
segmentPointMesg.addField(new Field("leader_time", LeaderTimeFieldNum, 134, 1000, 0, "s", false, Profile.Type.UINT32));
field_index++;
segmentPointMesg.addField(new Field("enhanced_altitude", EnhancedAltitudeFieldNum, 134, 5, 500, "m", false, Profile.Type.UINT32));
field_index++;
}
public SegmentPointMesg() {
super(Factory.createMesg(MesgNum.SEGMENT_POINT));
}
public SegmentPointMesg(final Mesg mesg) {
super(mesg);
}
/**
* Get message_index field
*
* @return message_index
*/
public Integer getMessageIndex() {
return getFieldIntegerValue(254, 0, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Set message_index field
*
* @param messageIndex The new messageIndex value to be set
*/
public void setMessageIndex(Integer messageIndex) {
setFieldValue(254, 0, messageIndex, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Get position_lat field
* Units: semicircles
*
* @return position_lat
*/
public Integer getPositionLat() {
return getFieldIntegerValue(1, 0, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Set position_lat field
* Units: semicircles
*
* @param positionLat The new positionLat value to be set
*/
public void setPositionLat(Integer positionLat) {
setFieldValue(1, 0, positionLat, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Get position_long field
* Units: semicircles
*
* @return position_long
*/
public Integer getPositionLong() {
return getFieldIntegerValue(2, 0, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Set position_long field
* Units: semicircles
*
* @param positionLong The new positionLong value to be set
*/
public void setPositionLong(Integer positionLong) {
setFieldValue(2, 0, positionLong, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Get distance field
* Units: m
* Comment: Accumulated distance along the segment at the described point
*
* @return distance
*/
public Float getDistance() {
return getFieldFloatValue(3, 0, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Set distance field
* Units: m
* Comment: Accumulated distance along the segment at the described point
*
* @param distance The new distance value to be set
*/
public void setDistance(Float distance) {
setFieldValue(3, 0, distance, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Get altitude field
* Units: m
* Comment: Accumulated altitude along the segment at the described point
*
* @return altitude
*/
public Float getAltitude() {
return getFieldFloatValue(4, 0, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Set altitude field
* Units: m
* Comment: Accumulated altitude along the segment at the described point
*
* @param altitude The new altitude value to be set
*/
public void setAltitude(Float altitude) {
setFieldValue(4, 0, altitude, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
public Float[] getLeaderTime() {
return getFieldFloatValues(5, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* @return number of leader_time
*/
public int getNumLeaderTime() {
return getNumFieldValues(5, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Get leader_time field
* Units: s
* Comment: Accumualted time each leader board member required to reach the described point. This value is zero for all leader board members at the starting point of the segment.
*
* @param index of leader_time
* @return leader_time
*/
public Float getLeaderTime(int index) {
return getFieldFloatValue(5, index, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Set leader_time field
* Units: s
* Comment: Accumualted time each leader board member required to reach the described point. This value is zero for all leader board members at the starting point of the segment.
*
* @param index of leader_time
* @param leaderTime The new leaderTime value to be set
*/
public void setLeaderTime(int index, Float leaderTime) {
setFieldValue(5, index, leaderTime, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Get enhanced_altitude field
* Units: m
* Comment: Accumulated altitude along the segment at the described point
*
* @return enhanced_altitude
*/
public Float getEnhancedAltitude() {
return getFieldFloatValue(6, 0, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
/**
* Set enhanced_altitude field
* Units: m
* Comment: Accumulated altitude along the segment at the described point
*
* @param enhancedAltitude The new enhancedAltitude value to be set
*/
public void setEnhancedAltitude(Float enhancedAltitude) {
setFieldValue(6, 0, enhancedAltitude, Fit.SUBFIELD_INDEX_MAIN_FIELD);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy