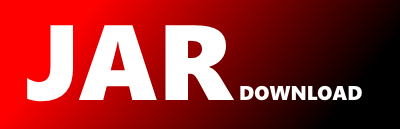
atom.view.View Maven / Gradle / Ivy
/*
* Copyright © 2015 Geeoz, and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* The Research Projects is dual-licensed under the GNU General Public
* License, version 2.0 (GPLv2) and the Geeoz Commercial License.
*
* Solely for non-commercial purposes. A purpose is non-commercial only if
* it is in no manner primarily intended for or directed toward commercial
* advantage or private monetary compensation.
*
* This Geeoz Software is supplied to you by Geeoz in consideration of your
* agreement to the following terms, and your use, installation, modification
* or redistribution of this Geeoz Software constitutes acceptance of these
* terms. If you do not agree with these terms, please do not use, install,
* modify or redistribute this Geeoz Software.
*
* Neither the name, trademarks, service marks or logos of Geeoz may be used
* to endorse or promote products derived from the Geeoz Software without
* specific prior written permission from Geeoz.
*
* The Geeoz Software is provided by Geeoz on an "AS IS" basis. GEEOZ MAKES NO
* WARRANTIES, EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION THE IMPLIED
* WARRANTIES OF NON-INFRINGEMENT, MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE, REGARDING THE GEEOZ SOFTWARE OR ITS USE AND OPERATION ALONE OR IN
* COMBINATION WITH YOUR PRODUCTS.
*
* IN NO EVENT SHALL GEEOZ BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL
* OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) ARISING IN ANY WAY OUT OF THE USE, REPRODUCTION, MODIFICATION
* AND/OR DISTRIBUTION OF THE GEEOZ SOFTWARE, HOWEVER CAUSED AND WHETHER UNDER
* THEORY OF CONTRACT, TORT (INCLUDING NEGLIGENCE), STRICT LIABILITY OR
* OTHERWISE, EVEN IF GEEOZ HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* A copy of the GNU General Public License is included in the distribution in
* the file LICENSE and at
*
* http://www.gnu.org/licenses/gpl-2.0.html
*
* If you are using the Research Projects for commercial purposes, we
* encourage you to visit
*
* http://products.geeoz.com/license
*
* for more details.
*
* This software or hardware and documentation may provide access to
* or information on content, products, and services from third parties.
* Geeoz and its affiliates are not responsible for and expressly disclaim
* all warranties of any kind with respect to third-party content, products,
* and services. Geeoz and its affiliates will not be responsible for any loss,
* costs, or damages incurred due to your access to or use of third-party
* content, products, or services. If a third-party content exists, the
* additional copyright notices and license terms applicable to portions of the
* software are set forth in the THIRD_PARTY_LICENSE_README file.
*
* Please contact Geeoz or visit www.geeoz.com if you need additional
* information or have any questions.
*/
package atom.view;
import atom.view.constants.ViewConstants;
import atom.view.constraints.AbstractConstraint;
import atom.view.constraints.NotNullConstraint;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElements;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlTransient;
import javax.xml.bind.annotation.XmlType;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* This class represents the basic building block for user interface components.
* View is the base class for widgets, which are used to create
* interactive UI components (buttons, text fields, etc.).
* The {@link ViewGroup} subclass is the base class for layouts, which
* are invisible containers that hold other Views (or other ViewGroup) and
* define their layout properties.
*
* @author Alex Voloshyn
* @author Serge Voloshyn
* @author Vladimir Ovcharov
* @version 1.15 4/18/15
* @see ViewGroup
*/
@XmlRootElement
public class View extends AbstractView implements Cloneable {
/**
* Use serialVersionUID from JDK 1.0.2 for interoperability.
*/
private static final long serialVersionUID = -1559168090601702997L;
/**
* The type of the view to display.
*/
private Element element;
/**
* List of the validation constraints for the view.
*/
private List constraints;
/**
* The space-separated style names.
*/
private String styleName;
/**
* Contains bitset of event types listened by the view.
*/
private int eventBits;
/**
* Listener used to dispatch click events.
*/
private transient Map eventListeners = null;
/**
* Simple constructor to use when creating a view from code.
*/
public View() {
super();
}
/**
* Simple constructor that creates View
with defined
* identifier.
*
* @param identify a string used to identify the view
* @see #setViewId(String)
*/
public View(final String identify) {
super(identify);
}
/**
* Retrieve the type of the view to display.
*
* @return the type of the view to display
*/
@XmlAttribute(name = "element")
public final Element getElement() {
return element;
}
/**
* Sets the type of the view to display.
*
* @param type the type of the view to display
*/
public final void setElement(final Element type) {
element = type;
}
/**
* Adds one more style name or sets it in case current style name is
* null
.
*
* @param style the secondary style name to be added
* @see #setStyleName(String)
*/
public final void addStyleName(final String style) {
if (styleName == null) {
setStyleName(style);
} else {
setStyleName(styleName.concat(" ").concat(style));
}
}
/**
* Gets all of the style names, as a space-separated list.
*
* @return the space-separated style names
*/
@XmlAttribute(name = "style")
@JsonProperty("style")
public final String getStyleName() {
return styleName;
}
/**
* Sets the style name for corresponding view.
*
* @param style the style name to be added
*/
public final void setStyleName(final String style) {
fireChange(ViewConstants.STYLE_NAME, style);
styleName = style;
}
@Override
public final View findViewById(final String identity) {
final String viewId = getViewId();
if (viewId != null && viewId.equals(identity)) {
return this;
}
if (this instanceof Group) {
return ((Group) this).findChildViewById(identity);
}
return null;
}
/**
* Directly call any attached EventListener or OnClickMethod.
*
* @param eventCode an event type
*/
public final void handleEvent(final int eventCode) {
if ((eventCode | eventBits) == eventBits && eventListeners != null) {
final EventType eventType = EventType.byCode(eventCode);
final EventListener eventListener
= eventListeners.get(eventType);
if (eventListener != null) {
eventListener.onEvent(eventType, this);
}
}
}
/**
* Get an events bitset listened by the view.
*
* @return an events bitset listened by the view
*/
@XmlTransient
public final int getEventBits() {
return eventBits;
}
/**
* Sets event bits to listen for this view.
*
* @param anEventBits events bitset
*/
public final void setEventBits(final int anEventBits) {
eventBits = anEventBits;
}
/**
* Register a callback to be invoked when this view is clicked.
*
* @param eventType an event type
* @param listener the callback that will run
*/
public final void setEventListener(final EventType eventType,
final EventListener listener) {
if (listener == null && eventListeners != null) {
eventListeners.remove(eventType);
setEventBits(eventBits ^ eventType.getCode());
} else {
if (eventListeners == null) {
eventListeners = new HashMap<>();
unifyId();
}
eventListeners.put(eventType, listener);
setEventBits(eventBits | eventType.getCode());
}
}
/**
* Add the validation constraints to the view instance.
*
* @param constraint the validation constraints
*/
public final void addConstraint(final AbstractConstraint constraint) {
if (constraints == null) {
constraints = new ArrayList<>();
}
constraints.add(constraint);
}
/**
* Retrieve the validation constraints for the view.
*
* @return the validation constraints
*/
@XmlElements({
@XmlElement(name = "NotNullConstraint",
type = NotNullConstraint.class)
})
@JsonProperty("constraints")
public final List getConstraints() {
return constraints;
}
/**
* Sets the validation constraints for the view.
*
* @param list the validation constraints
*/
public final void setConstraints(final List list) {
constraints = list;
}
/**
* Клонирование экземпляра представления для использования в контроллере
* динамических данных.
*
* @return клонированный экземпляра представления
*/
public View cloneAsTemplate() {
try {
return (View) super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
@Override
public boolean equals(final Object object) {
boolean areEquals;
if (this == object) {
areEquals = true;
} else {
if (object instanceof View) {
final View that = (View) object;
areEquals = Objects.equals(getViewId(), that.getViewId())
&& Objects.equals(getBinaryPath(), that.getBinaryPath())
&& Objects.equals(element, that.element)
&& Objects.equals(constraints, that.constraints)
&& Objects.equals(styleName, that.styleName)
&& Objects.equals(eventBits, that.eventBits);
} else {
areEquals = false;
}
}
return areEquals;
}
@Override
public int hashCode() {
return Objects.hash(getViewId(), element);
}
@Override
public String toString() {
return String.format(
"View { "
+ "viewId='%s', "
+ "binaryPath='%s', "
+ "element='%s', "
+ "constraints='%s', "
+ "options='%s', "
+ "styleName='%s', "
+ "eventBits='%d' }",
getViewId(), getBinaryPath(), element, constraints,
getOptions(), styleName, eventBits
);
}
/**
* The type of the view to display.
*/
@XmlType(namespace = AbstractView.LAYOUT_NAMESPACE)
public static enum Element {
/**
* Sections
*
* Represents the content of an HTML document. There is only one body
* element in a document.
*/
body,
/**
* Defines a section in a document.
*/
section,
/**
* Defines a section that contains only navigation links.
*/
nav,
/**
* Defines self-contained content that could exist independently of
* the rest of the content.
*/
article,
/**
* Defines some content loosely related to the page content. If it is
* removed, the remaining content still makes sense.
*/
aside,
/**
* Heading elements implement six levels of document headings; h1 is
* the most important and h6 is the least. A heading element briefly
* describes the topic of the section it introduces.
*/
h1, h2, h3, h4, h5, h6,
/**
* Defines the header of a page or section. It often contains a logo,
* the title of the Web site, and a navigational table of content.
*/
header,
/**
* Defines the footer for a page or section. It often contains
* a copyright notice, some links to legal information, or addresses
* to give feedback.
*/
footer,
/**
* Defines a section containing contact information.
*/
address,
/**
* Defines the main or important content in the document. There is only
* one main element in the document.
*/
main,
/**
*
Grouping content
*
* Defines a portion that should be displayed as a paragraph.
*/
p,
/**
* Represents a thematic break between paragraphs of a section or
* article or any longer content.
*/
hr,
/**
* Indicates that its content is preformatted and that this format must
* be preserved.
*/
pre,
/**
* Represents a content that is quoted from another source.
*/
blockquote,
/**
* Defines an ordered list of items.
*/
ol,
/**
* Defines an unordered list of items.
*/
ul,
/**
* Defines a item of an enumeration list.
*/
li,
/**
* Defines a definition list, that is, a list of terms and their
* associated definitions.
*/
dl,
/**
* Represents a term defined by the next dd.
*/
dt,
/**
* Represents the definition of the terms immediately listed before it.
*/
dd,
/**
* Represents a figure illustrated as part of the document.
*/
figure,
/**
* Represents the legend of a figure.
*/
figcaption,
/**
* Represents a generic container with no special meaning.
*/
div,
/**
*
Text-level semantics
*
* Represents a hyperlink, linking to another resource.
*/
a,
/**
* Represents emphasized text, like a stress accent.
*/
em,
/**
* Represents especially important text.
*/
strong,
/**
* Represents a side comment, that is, text like a disclaimer or
* a copyright, which is not essential to the comprehension of
* the document.
*/
small,
/**
* Represents content that is no longer accurate or relevant.
*/
s,
/**
* Represents the title of a work.
*/
cite,
/**
* Represents an inline quotation.
*/
q,
/**
* Represents a term whose definition is contained in its nearest
* ancestor content.
*/
dfn,
/**
* Represents an abbreviation or an acronym ; the expansion
* of the abbreviation can be represented in the title attribute.
*/
abbr,
/**
* Associates to its content a machine-readable equivalent. (This
* element is only in the WHATWG version of the HTML standard, and
* not in the W3C version of HTML5).
*/
data,
/**
* Represents a date and time value; the machine-readable equivalent can
* be represented in the datetime attribute.
*/
time,
/**
* Represents computer code.
*/
code,
/**
* Represents the output of a program or a computer.
*/
samp,
/**
* Represents user input, often from the keyboard, but not necessarily;
* it may represent other input, like transcribed voice commands.
*/
kbd,
/**
* Represent a subscript, or a superscript.
*/
sub, sup,
/**
* Represents some text in an alternate voice or mood, or at least of
* different quality, such as a taxonomic designation, a technical term,
* an idiomatic phrase, a thought, or a ship name.
*/
i,
/**
* Represents a text which to which attention is drawn for utilitarian
* purposes. It doesn't convey extra importance and doesn't imply
* an alternate voice.
*/
b,
/**
* Represents a non-textual annoatation for which the conventional
* presentation is underlining, such labeling the text as being misspelt
* or labeling a proper name in Chinese text.
*/
u,
/**
* Represents text highlighted for reference purposes, that is for its
* relevance in another context.
*/
mark,
/**
* Represents content to be marked with ruby annotations, short runs of
* text presented alongside the text. This is often used in conjunction
* with East Asian language where the annotations act as a guide
* for pronunciation, like the Japanese furigana.
*/
ruby,
/**
* Represents the text of a ruby annotation.
*/
rt,
/**
* Represents parenthesis around a ruby annotation, used to display
* the annotation in an alternate way by browsers not supporting
* the standard display for annotations.
*/
rp,
/**
* Represents text that must be isolated from its surrounding for
* bidirectional text formatting. It allows embedding a span of text
* with a different, or unknown, directionality.
*/
bdi,
/**
* Represents the directionality of its children, in order to explicitly
* override the Unicode bidirectional algorithm.
*/
bdo,
/**
* Represents text with no specific meaning. This has to be used when no
* other text-semantic element conveys an adequate meaning, which,
* in this case, is often brought by global attributes like class, lang,
* or dir.
*/
span,
/**
* Represents a line break.
*/
br,
/**
* Represents a line break opportunity, that is a suggested point
* for wrapping text in order to improve readability of text split
* on several lines.
*/
wbr,
/**
*
Embedded content
* Represents an image.
*/
img,
/**
* Represents a nested browsing context, that is an embedded HTML
* document.
*/
iframe,
/**
* Represents a integration point for an external, often non-HTML,
* application or interactive content.
*/
embed,
/**
* Represents an external resource, which is treated as an image,
* an HTML sub-document, or an external resource to be processed
* by a plug-in.
*/
object,
/**
* Defines parameters for use by plug-ins invoked by object elements.
*/
param,
/**
* Represents a video, and its associated audio files and captions,
* with the necessary interface to play it.
*/
video,
/**
* Represents a sound, or an audio stream.
*/
audio,
/**
* Allows authors to specify alternative media resources for media
* elements like video or audio.
*/
source,
/**
* Allows authors to specify timed text track for media elements like
* video or audio.
*/
track,
/**
* Represents a bitmap area that scripts can be used to render graphics,
* like graphs, game graphics, or any visual images on the fly.
*/
canvas,
/**
* In conjunction with area, defines an image map.
*/
map,
/**
* In conjunction with map, defines an image map.
*/
area,
/**
* Defines an embedded vectorial image.
*/
svg,
/**
* Defines a mathematical formula.
*/
math,
/**
* Tabular data
* Represents data with more than one dimension.
*/
table,
/**
* Represents the title of a table.
*/
caption,
/**
* Represents a set of one or more columns of a table.
*/
colgroup,
/**
* Represents a column of a table.
*/
col,
/**
* Represents the block of rows that describes the concrete data
* of a table.
*/
tbody,
/**
* Represents the block of rows that describes the column labels
* of a table.
*/
thead,
/**
* Represents the block of rows that describes the column summaries
* of a table.
*/
tfoot,
/**
* Represents a row of cells in a table.
*/
tr,
/**
* Represents a data cell in a table.
*/
td,
/**
* Represents a header cell in a table.
*/
th,
/**
* Forms
* Represents a form, consisting of controls, that can be submitted
* to a server for processing.
*/
form,
/**
* Represents a set of controls.
*/
fieldset,
/**
* Represents the caption for a fieldset.
*/
legend,
/**
* Represents the caption of a form control.
*/
label,
/**
* Represents a typed data field allowing the user to edit the data.
*/
input,
/**
* Represents a button.
*/
button,
/**
* Represents a control allowing selection among a set of options.
*/
select,
/**
* Represents a set of predefined options for other controls.
*/
datalist,
/**
* Represents a set of options, logically grouped.
*/
optgroup,
/**
* Represents an option in a select element, or a suggestion of
* a datalist element.
*/
option,
/**
* Represents a multiline text edit control.
*/
textarea,
/**
* Represents a key-pair generator control.
*/
keygen,
/**
* Represents the result of a calculation.
*/
output,
/**
* Represents the completion progress of a task.
*/
progress,
/**
* Represents a scalar measurement (or a fractional value), within
* a known range.
*/
meter,
/**
* Interactive elements
* Represents a widget from which the user can obtain additional
* information or controls.
*/
details,
/**
* Represents a summary, caption, or legend for a given details.
*/
summary,
/**
* Represents a command that the user can invoke.
*/
menuitem,
/**
* Represents a list of commands.
*/
menu
}
}