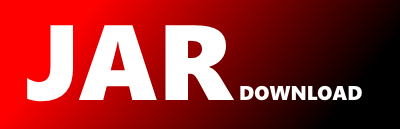
atom.app.Activity Maven / Gradle / Ivy
/*
* Copyright © 2014 Geeoz, and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* The Research Projects is dual-licensed under the GNU General Public
* License, version 2.0 (GPLv2) and the Geeoz Commercial License.
*
* Solely for non-commercial purposes. A purpose is non-commercial only if
* it is in no manner primarily intended for or directed toward commercial
* advantage or private monetary compensation.
*
* This Geeoz Software is supplied to you by Geeoz in consideration of your
* agreement to the following terms, and your use, installation, modification
* or redistribution of this Geeoz Software constitutes acceptance of these
* terms. If you do not agree with these terms, please do not use, install,
* modify or redistribute this Geeoz Software.
*
* Neither the name, trademarks, service marks or logos of Geeoz may be used
* to endorse or promote products derived from the Geeoz Software without
* specific prior written permission from Geeoz.
*
* The Geeoz Software is provided by Geeoz on an "AS IS" basis. GEEOZ MAKES NO
* WARRANTIES, EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION THE IMPLIED
* WARRANTIES OF NON-INFRINGEMENT, MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE, REGARDING THE GEEOZ SOFTWARE OR ITS USE AND OPERATION ALONE OR IN
* COMBINATION WITH YOUR PRODUCTS.
*
* IN NO EVENT SHALL GEEOZ BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL
* OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) ARISING IN ANY WAY OUT OF THE USE, REPRODUCTION, MODIFICATION
* AND/OR DISTRIBUTION OF THE GEEOZ SOFTWARE, HOWEVER CAUSED AND WHETHER UNDER
* THEORY OF CONTRACT, TORT (INCLUDING NEGLIGENCE), STRICT LIABILITY OR
* OTHERWISE, EVEN IF GEEOZ HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* A copy of the GNU General Public License is included in the distribution in
* the file LICENSE and at
*
* http://www.gnu.org/licenses/gpl-2.0.html
*
* If you are using the Research Projects for commercial purposes, we
* encourage you to visit
*
* http://products.geeoz.com/license
*
* for more details.
*
* This software or hardware and documentation may provide access to
* or information on content, products, and services from third parties.
* Geeoz and its affiliates are not responsible for and expressly disclaim
* all warranties of any kind with respect to third-party content, products,
* and services. Geeoz and its affiliates will not be responsible for any loss,
* costs, or damages incurred due to your access to or use of third-party
* content, products, or services. If a third-party content exists, the
* additional copyright notices and license terms applicable to portions of the
* software are set forth in the THIRD_PARTY_LICENSE_README file.
*
* Please contact Geeoz or visit www.geeoz.com if you need additional
* information or have any questions.
*/
package atom.app;
import atom.content.Context;
import atom.content.Intent;
import atom.view.Filter;
import atom.view.View;
import atom.view.ViewGroup;
import atom.view.Window;
import java.beans.PropertyChangeEvent;
import java.util.List;
import java.util.Objects;
/**
* An activity is a single, focused thing that the user can do. Almost all
* activities interact with the user, so the Activity class takes care of
* creating a window for you in which you can place your UI with
* {@link #setContentView}. While activities are often presented to the user
* as full-screen windows.
*
* @author Alex Voloshyn
* @author Serge Voloshyn
* @version 1.10 6/23/14
*/
public class Activity extends Context {
/**
* Use serialVersionUID from JDK 1.0.2 for interoperability.
*/
private static final long serialVersionUID = -8316661669183224489L;
/**
* Intent that created this activity.
*/
private Intent intent;
/**
* Parent activity that initialize current activity creation.
*/
private Activity parent;
/**
* Current {@link atom.view.Window} for the activity.
*/
private Window window = new Window();
/**
* Retrieve an intent that created this activity.
*
* @return an intent that created this activity
*/
public final Intent getIntent() {
return intent;
}
/**
* Sets an intent that created this activity.
*
* @param anIntent an intent that created this activity
*/
public final void setIntent(final Intent anIntent) {
intent = anIntent;
}
/**
* Retrieve an activity that initialize current activity creation.
*
* @return an activity that initialize current activity creation
*/
public final Activity getParent() {
return parent;
}
/**
* Sets activity that initialize current activity creation.
*
* @param activity an activity that initialize current activity creation
*/
public final void setParent(final Activity activity) {
parent = activity;
}
/**
* Provide list of property change events.
*
* @return list of property change events
*/
public final List getChanges() {
return window.getChanges();
}
/**
* Enable tracking changes for the view.
*/
public final void trackChanges() {
window.trackChanges();
}
/**
* Retrieve the current {@link atom.view.Window} for the activity. This can
* be used to directly access parts of the Window API that are not available
* through Activity/Screen.
*
* @return the current window, or null if the activity is not visual
*/
public final Window getWindow() {
return window;
}
/**
* Provide ability to set display after de-serialization.
*
* @param display window to set
*/
public final void setWindow(final Window display) {
window = display;
}
/**
* Finds a view that was identified by the id attribute that was processed
* in {@link #onCreate}.
*
* @param identity id attribute that was processed
* @return the view if found or null otherwise
*/
public final View findViewById(final String identity) {
return window.findViewById(identity);
}
/**
* Return the parent if of the view if it exists, or null.
*
* @param view - child view that will be processing
* @return the parent view if found or null otherwise
*/
public final ViewGroup getParentView(final View view) {
return window.getParentView(view);
}
/**
* Apply changes to view according to existing hierarchy.
*
* @param events property change events that should be applied
*/
public final void applyChanges(final List events) {
window.applyChanges(events);
}
/**
* Call corresponding view attached OnClickListener.
*
* @param identity view identity that fire event
*/
public final void callOnClick(final String identity) {
window.callOnClick(identity);
}
/**
* Called when the activity is starting. This is where most initialization
* should go: calling {@link #setContentView(View)} to inflate the
* activity's UI, using {@link #findViewById(String)} to pragmatically
* interact with widgets in the UI.
*/
protected void onCreate() {
// After extending Activity should override this method to provide
// activity creation.
}
/**
* Perform any final cleanup before an activity is destroyed. This can
* happen either because the activity is finishing on it, or because the
* system is temporarily destroying this instance of the activity to save
* space.
*
* Derived classes must call through to the super class's
* implementation of this method. If they do not, an exception will be
* thrown.
*/
protected void onDestroy() {
// After extending Activity should override this method to provide
// activity destroy.
}
/**
* Adds the stylesheet link from the window.
*
* @param link the stylesheet link to add
*/
public final void addStylesheetLink(final String link) {
window.addLink(link);
}
/**
* Set the activity content to an explicit view. This view is placed
* directly into the activity's view hierarchy. It can itself be a complex
* view hierarchy. When calling this method, the layout parameters of the
* specified view are ignored.
*
* @param view the desired content to display
*/
public final void setContentView(final View view) {
window.setContentView(view);
}
/**
* Set the activity content to an explicit view. This view is placed
* directly into the activity's view hierarchy. It can itself be a complex
* view hierarchy. When calling this method, the layout parameters of the
* specified view are ignored.
*
* @param path path to the XML resource view view
*/
public final void setContentView(final String path) {
setContentView(inflate(path));
}
/**
* Change the title associated with this activity. If this is a top-level
* activity, the title for its window will change. If it is an embedded
* activity, the parent can do whatever it wants with it.
*
* @param title title associated with this activity
*/
public final void setTitle(final String title) {
window.setTitle(title);
}
/**
* Sets resource filter reference.
*
* @param filter a resource filter
*/
public final void setFilter(final Filter filter) {
window.setFilter(filter);
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(final Object object) {
boolean areEquals;
if (this == object) {
areEquals = true;
} else {
if (object instanceof Activity) {
final Activity that = (Activity) object;
areEquals = super.equals(that)
&& Objects.equals(parent, that.parent)
&& Objects.equals(window, that.window)
&& Objects.equals(intent, that.intent);
} else {
areEquals = false;
}
}
return areEquals;
}
/* (non-Javadoc)
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
return Objects.hash(getActivityThread(), parent, window, intent);
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return String.format(
"Activity { intent='%s',"
+ " parent='%s', window='%s' }",
intent, parent, window);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy