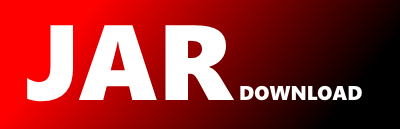
atom.content.Intent Maven / Gradle / Ivy
/*
* Copyright © 2013 Geeoz, and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* The Research Projects is dual-licensed under the GNU General Public
* License, version 2.0 (GPLv2) and the Geeoz Commercial License.
*
* Solely for non-commercial purposes. A purpose is non-commercial only if
* it is in no manner primarily intended for or directed toward commercial
* advantage or private monetary compensation.
*
* This Geeoz Software is supplied to you by Geeoz in consideration of your
* agreement to the following terms, and your use, installation, modification
* or redistribution of this Geeoz Software constitutes acceptance of these
* terms. If you do not agree with these terms, please do not use, install,
* modify or redistribute this Geeoz Software.
*
* Neither the name, trademarks, service marks or logos of Geeoz may be used
* to endorse or promote products derived from the Geeoz Software without
* specific prior written permission from Geeoz.
*
* The Geeoz Software is provided by Geeoz on an "AS IS" basis. GEEOZ MAKES NO
* WARRANTIES, EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION THE IMPLIED
* WARRANTIES OF NON-INFRINGEMENT, MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE, REGARDING THE GEEOZ SOFTWARE OR ITS USE AND OPERATION ALONE OR IN
* COMBINATION WITH YOUR PRODUCTS.
*
* IN NO EVENT SHALL GEEOZ BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL
* OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) ARISING IN ANY WAY OUT OF THE USE, REPRODUCTION, MODIFICATION
* AND/OR DISTRIBUTION OF THE GEEOZ SOFTWARE, HOWEVER CAUSED AND WHETHER UNDER
* THEORY OF CONTRACT, TORT (INCLUDING NEGLIGENCE), STRICT LIABILITY OR
* OTHERWISE, EVEN IF GEEOZ HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* A copy of the GNU General Public License is included in the distribution in
* the file LICENSE and at
*
* http://www.gnu.org/licenses/gpl-2.0.html
*
* If you are using the Research Projects for commercial purposes, we
* encourage you to visit
*
* http://products.geeoz.com/license
*
* for more details.
*
* This software or hardware and documentation may provide access to
* or information on content, products, and services from third parties.
* Geeoz and its affiliates are not responsible for and expressly disclaim
* all warranties of any kind with respect to third-party content, products,
* and services. Geeoz and its affiliates will not be responsible for any loss,
* costs, or damages incurred due to your access to or use of third-party
* content, products, or services. If a third-party content exists, the
* additional copyright notices and license terms applicable to portions of the
* software are set forth in the THIRD_PARTY_LICENSE_README file.
*
* Please contact Geeoz or visit www.geeoz.com if you need additional
* information or have any questions.
*/
package atom.content;
import javax.xml.bind.annotation.XmlTransient;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Objects;
/**
* Provide testing of the abstract description of an operation to be performed.
*
* Standard Activity Actions
*
* These are the current standard actions that Intent defines for launching
* activities (usually through {@link Context#startActivity}. The most
* important, and by far most frequently used, are {@link #ACTION_MAIN},
* {@link #ACTION_MAIN} and {@link #ACTION_EDIT}.
*
*
* - {@link #ACTION_MAIN}
*
- {@link #ACTION_VIEW}
*
- {@link #ACTION_EDIT}
*
*
* Standard Categories
*
* These are the current standard categories that can be used to further
* clarify an Intent via {@link #addCategory}.
*
*
* - {@link #CATEGORY_HOME}
*
*
* Standard Layouts
*
* These are the current standard layouts.
*
*
* - {@link #LAYER_DEFAULT}
*
- {@link #LAYER_MAX_COUNT}
*
*
*
* Standard Extra Data
*
* These are the current standard fields that can be used as extra data via
* {@link #putExtra}.
*
*
* - {@link #EXTRA_BCC}
*
- {@link #EXTRA_CC}
*
- {@link #EXTRA_EMAIL}
*
- {@link #EXTRA_INTENT}
*
- {@link #EXTRA_LOCALE}
*
- {@link #EXTRA_STREAM}
*
- {@link #EXTRA_SUBJECT}
*
- {@link #EXTRA_TEXT}
*
- {@link #EXTRA_TITLE}
*
*
* @author Alex Voloshyn
* @version 1.11 11/2/13
*/
public final class Intent implements Serializable {
/**
* Use serialVersionUID from JDK 1.0.2 for interoperability.
*/
private static final long serialVersionUID = -558232427900033086L;
/**
* Activity Action: Edit the requested activity category.
* Input: nothing
* Output: nothing
*/
public static final String ACTION_EDIT = "edit";
/**
* Activity Action: Start as a main entry point, does not expect to receive
* data.
* Input: nothing
* Output: nothing
*/
public static final String ACTION_MAIN = "main";
/**
* Activity Action: View the requested activity category.
* Input: nothing
* Output: nothing
*/
public static final String ACTION_VIEW = "view";
/**
* This is the home activity, that is the first activity that is displayed
* when the application context initialized.
*/
public static final String CATEGORY_HOME = "home";
/**
* A String[] holding e-mail addresses that should be delivered to.
*/
public static final String EXTRA_EMAIL = "email";
/**
* A String[] holding e-mail addresses that should be carbon copied.
*/
public static final String EXTRA_CC = "cc";
/**
* A String[] holding e-mail addresses that should be blind carbon copied.
*/
public static final String EXTRA_BCC = "bcc";
/**
* A constant string holding the desired locale.
*/
public static final String EXTRA_LOCALE = "locale";
/**
* A constant string holding the desired subject line of a message.
*/
public static final String EXTRA_SUBJECT = "subject";
/**
* Maximum layers count, default layer number is five (5).
*/
public static final int LAYER_MAX_COUNT = 10;
/**
* Default activity layer index.
*/
public static final int LAYER_DEFAULT = 5;
/**
* An extra data for processing.
*/
private transient Map extras = null;
/**
* An action name to perform.
*/
private String action;
/**
* Action list that matched for corresponding activity.
*/
private List categories;
/**
* The data this intent is operating on.
*/
private String data;
/**
* (Usually optional) Explicitly set the component to handle the intent.
* If left with the default value of null, the system will determine the
* appropriate class to use based on the other fields (action, data, type,
* categories) in the Intent. If this class is defined, the specified class
* will always be used regardless of the other fields. You should only set
* this value when you know you absolutely want a specific class to be used;
* otherwise it is better to let the system find the appropriate class so
* that you will respect the installed applications and user preferences.
*/
private ComponentId componentId;
/**
* Create an empty intent.
*/
public Intent() {
super();
}
/**
* Constructor for new action and one category.
*
* @param anAction an action name, such as ACTION_MAIN
* @param category the desired category, this can be either one of the
* predefined Intent categories, or a custom category in
* your own namespace
*/
public Intent(final String anAction, final String category) {
super();
setAction(anAction);
addCategory(category);
}
/**
* Retrieve the general action to be performed, such as
* {@link #ACTION_MAIN}.
*
* @return an action to perform
*/
public String getAction() {
return action;
}
/**
* Set the general action to be performed.
*
* @param anAction an action name, such as ACTION_MAIN
*/
public void setAction(final String anAction) {
if (anAction == null) {
action = null;
} else {
action = anAction.intern();
}
}
/**
* Add a new category to the intent. Categories provide additional detail
* about the action the intent is perform. When resolving an intent, only
* activities that provide all of the requested categories will be
* used.
*
* @param category the desired category, this can be either one of the
* predefined Intent categories, or a custom category in
* your own namespace
*/
public void addCategory(final String category) {
if (category != null) {
if (categories == null) {
setCategories(new ArrayList());
}
categories.add(category.intern());
}
}
/**
* Return the list of all categories in the intent. If there are no
* categories, returns null
.
*
* @return the set of categories you can examine. Do not modify!
*/
public List getCategories() {
return categories;
}
/**
* Sets the list of all categories in the intent.
*
* @param set the set of categories you can examine
*/
public void setCategories(final List set) {
categories = set;
}
/**
* Retrieve data this intent is operating on. This URI specifies the name of
* the data; often it uses the content: scheme, specifying data in a content
* provider. Other schemes may be handled by specific activities, such as
* http: by the web browser.
*
* @return the URI of the data this intent is targeting or null
*/
public String getData() {
return data;
}
/**
* Set the data this intent is operating on.
*
* @param uri the URI of the data this intent is now targeting
*/
public void setData(final String uri) {
data = uri;
}
/**
* Retrieve the concrete component associated with the intent. When
* receiving an intent, this is the component that was found to best handle
* it (that is, yourself) and will always be non-null; in all other cases it
* will be null unless explicitly set.
*
* @return the identity of the application component to handle the intent
* @see atom.content.pm.PackageManager#resolveActivity(Intent)
*/
public ComponentId getComponentId() {
return componentId;
}
/**
* (Usually optional) Explicitly set the component to handle the intent.
* If left with the default value of null, the system will determine the
* appropriate class to use based on the other fields (action, data, type,
* categories) in the Intent. If this class is defined, the specified class
* will always be used regardless of the other fields. You should only set
* this value when you know you absolutely want a specific class to be used;
* otherwise it is better to let the system find the appropriate class so
* that you will respect the installed applications and user preferences.
*
* @param aComponentId the identity of the application component to handle
* the intent, or null to let the system find one for
* you
* @see atom.content.pm.PackageManager#resolveActivity(Intent)
*/
public void setComponentId(final ComponentId aComponentId) {
componentId = aComponentId;
}
/**
* Retrieves a map of extended data from the intent.
*
* @return the map of all extras previously added with putExtra()
*/
public Map getExtras() {
return extras;
}
/**
* Sets a map of extended data from the intent.
*
* @param map the map of all extras previously added with putExtra()
*/
@SuppressWarnings("unused") // Only for deserialization cases
protected void setExtras(final Map map) {
extras = map;
}
/**
* Returns the value associated with the given key, or defaultValue if
* no mapping of the desired type exists for the given key.
*
* @param key a String, or null
* @param defaultValue default value to return
* @return the value or default value
*/
@XmlTransient
public Locale getLocaleExtra(final String key, final Locale defaultValue) {
final Object locale = getObjectExtra(key, defaultValue);
if (locale == null || !(locale instanceof Locale)) {
return defaultValue;
} else {
return (Locale) locale;
}
}
/**
* Returns the value associated with the given key, or defaultValue if
* no mapping of the desired type exists for the given key.
*
* @param key a String, or null
* @param defaultValue default value to return
* @return the value or default value
*/
@XmlTransient
public String[] getStringArrayExtra(final String key,
final String[] defaultValue) {
final Object array = getObjectExtra(key, defaultValue);
if (array == null || !(array instanceof String[])) {
return defaultValue;
} else {
return (String[]) array;
}
}
/**
* Returns the value associated with the given key, or defaultValue if
* no mapping of the desired type exists for the given key.
*
* @param key a String, or null
* @param defaultValue default value to return
* @return the value or default value
*/
@XmlTransient
public Object getObjectExtra(final String key, final Object defaultValue) {
if (extras == null) {
return null;
}
final Object value = extras.get(key);
if (value == null) {
return defaultValue;
}
return value;
}
/**
* Inserts an Object value into the mapping, replacing any existing value
* for the given key. Either key or value may be null.
*
* @param key a String, or null
* @param value an Object, or null
*/
public void putExtra(final String key, final Object value) {
if (extras == null) {
extras = new HashMap<>();
}
extras.put(key, value);
}
/**
* Inserts an Locale value into the mapping, replacing any existing value
* for the given key. Either key or value may be null.
*
* @param key a String, or null
* @param value an Locale, or null
*/
public void putExtra(final String key, final Locale value) {
putExtra(key, (Object) value);
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(final Object object) {
boolean areEquals;
if (this == object) {
areEquals = true;
} else {
if (object instanceof Intent) {
final Intent that = (Intent) object;
areEquals = Objects.equals(action, that.action)
&& Objects.equals(categories, that.categories)
&& Objects.equals(data, that.data)
&& Objects.equals(componentId, that.componentId);
} else {
areEquals = false;
}
}
return areEquals;
}
/* (non-Javadoc)
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
return Objects.hash(action, categories, data, componentId);
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return String.format("Intent { "
+ "action='%s', categories='%s', data='%s', componentId='%s' }",
action, categories, data, componentId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy