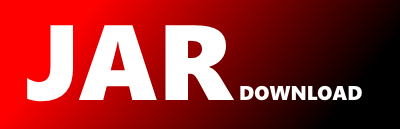
atom.widget.Slider Maven / Gradle / Ivy
/*
* Copyright © 2014 Geeoz, and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* The Research Projects is dual-licensed under the GNU General Public
* License, version 2.0 (GPLv2) and the Geeoz Commercial License.
*
* Solely for non-commercial purposes. A purpose is non-commercial only if
* it is in no manner primarily intended for or directed toward commercial
* advantage or private monetary compensation.
*
* This Geeoz Software is supplied to you by Geeoz in consideration of your
* agreement to the following terms, and your use, installation, modification
* or redistribution of this Geeoz Software constitutes acceptance of these
* terms. If you do not agree with these terms, please do not use, install,
* modify or redistribute this Geeoz Software.
*
* Neither the name, trademarks, service marks or logos of Geeoz may be used
* to endorse or promote products derived from the Geeoz Software without
* specific prior written permission from Geeoz.
*
* The Geeoz Software is provided by Geeoz on an "AS IS" basis. GEEOZ MAKES NO
* WARRANTIES, EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION THE IMPLIED
* WARRANTIES OF NON-INFRINGEMENT, MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE, REGARDING THE GEEOZ SOFTWARE OR ITS USE AND OPERATION ALONE OR IN
* COMBINATION WITH YOUR PRODUCTS.
*
* IN NO EVENT SHALL GEEOZ BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL
* OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) ARISING IN ANY WAY OUT OF THE USE, REPRODUCTION, MODIFICATION
* AND/OR DISTRIBUTION OF THE GEEOZ SOFTWARE, HOWEVER CAUSED AND WHETHER UNDER
* THEORY OF CONTRACT, TORT (INCLUDING NEGLIGENCE), STRICT LIABILITY OR
* OTHERWISE, EVEN IF GEEOZ HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* A copy of the GNU General Public License is included in the distribution in
* the file LICENSE and at
*
* http://www.gnu.org/licenses/gpl-2.0.html
*
* If you are using the Research Projects for commercial purposes, we
* encourage you to visit
*
* http://products.geeoz.com/license
*
* for more details.
*
* This software or hardware and documentation may provide access to
* or information on content, products, and services from third parties.
* Geeoz and its affiliates are not responsible for and expressly disclaim
* all warranties of any kind with respect to third-party content, products,
* and services. Geeoz and its affiliates will not be responsible for any loss,
* costs, or damages incurred due to your access to or use of third-party
* content, products, or services. If a third-party content exists, the
* additional copyright notices and license terms applicable to portions of the
* software are set forth in the THIRD_PARTY_LICENSE_README file.
*
* Please contact Geeoz or visit www.geeoz.com if you need additional
* information or have any questions.
*/
package atom.widget;
import atom.view.AbstractView;
import atom.view.View;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import java.util.Objects;
/**
* Slider is used to set value from min allowed to max allowed or select
* a range of values(from and to) rom min allowed to max allowed.
* In case single mode - slider provide to set value from min allowed to
* max allowed.
* In case range mode - slider provide to a range of values(from and to)
* from min allowed to max allowed.
*
* @author Alex Voloshyn
* @author Eugene Shevchenko
* @version 1.1 4/20/14
*/
@XmlType(namespace = AbstractView.LAYOUT_NAMESPACE)
@XmlRootElement(name = "Slider", namespace = AbstractView.LAYOUT_NAMESPACE)
public final class Slider extends View {
/**
* Serial version UID for serialization.
*/
private static final long serialVersionUID = 5915414318901124642L;
/**
* Default range mode minimum value.
*/
public static final Float DEFAULT_MIN_VALUE = 0f;
/**
* Default range mode maximum value.
*/
public static final Float DEFAULT_MAX_VALUE = 10f;
/**
* Default slider step value.
*/
public static final Float DEFAULT_STEP_VALUE = 1f;
/**
* The programmatic name of the value property.
* It will be used in case if slider initialized in rangeMode mode.
*
* @see atom.widget.Slider#setRangeMode(boolean).
*/
public static final String RANGE_VALUE_NAME = "value";
/**
* The programmatic name of the valueFrom property.
* It will be used if slider is initialized to range mode.
*
* @see atom.widget.Slider#setRangeMode(boolean).
*/
public static final String RANGE_VALUE_FROM_NAME = "valueFrom";
/**
* The programmatic name of the valueTo property.
* It will be used if slider is initialized to range mode.
*
* @see atom.widget.Slider#setRangeMode(boolean).
*/
public static final String RANGE_VALUE_TO_NAME = "valueTo";
/**
* Minimum possible value. Default value 0.
*/
private Float min = DEFAULT_MIN_VALUE;
/**
* Maximum possible value. Default value 10.
*/
private Float max = DEFAULT_MAX_VALUE;
/**
* Increment step. Default value 1.
*/
private Float step = DEFAULT_STEP_VALUE;
/**
* Set the orientation. Accepts 'vertical' or 'horizontal'.
*
* Default valule is 'horizontal'.
*/
private Orientation orientation = Orientation.horizontal;
/**
* Whether to slide the handle smoothly when the user clicks on
* the slider track. The name of a speed, such as "fast" or "slow".
*
* Default value is 'fast'.
*/
private Animate animate = Animate.fast;
/**
* Single slider value.
* It will be used if slider is initialized to single mode.
*
* @see atom.widget.Slider#setRangeMode(boolean).
*/
private Float value;
/**
* Range slider valueFrom.
* It will be used if slider is initialized to range mode.
*
* @see atom.widget.Slider#setRangeMode(boolean).
*/
private Float valueFrom;
/**
* Range slider valueTo.
* It will be used if slider is initialized to range mode.
*
* @see atom.widget.Slider#setRangeMode(boolean).
*/
private Float valueTo;
/**
* Single/Range mode switcher.
* In case rangeMode mode - value will be used as value.
* In case range mode - valueFrom and valueTo will be used as values.
*/
private boolean rangeMode = false;
/**
* Indicator to show/hide selected value.
*/
private boolean showSelected = true;
/**
* Default constructor.
*/
public Slider() {
super();
}
/**
* Creates instance with a defined id.
*
* @param viewId view identity
*/
public Slider(final String viewId) {
super(viewId);
}
/**
* Get minimum possible slider value.
*
* @return minimum possible slider value.
*/
@XmlAttribute(name = "min")
public Float getMin() {
return min;
}
/**
* Initialize minimum possible slider value.
*
* @param minimum minimum possible slider value.
*/
public void setMin(final Float minimum) {
min = minimum;
}
/**
* Get maximum possible slider value.
*
* @return maximum possible slider value.
*/
@XmlAttribute(name = "max")
public Float getMax() {
return max;
}
/**
* Initialize maximum possible slider value.
*
* @param maximum maximum possible slider value.
*/
public void setMax(final Float maximum) {
max = maximum;
}
/**
* Get incrementation step value.
*
* @return incrementation step value.
*/
@XmlAttribute(name = "step")
public Float getStep() {
return step;
}
/**
* Initialize incrementation step slider value.
*
* @param increment incrementation step slider value.
*/
public void setStep(final Float increment) {
step = increment;
}
/**
* Get slider orientation value.
*
* @return slider orientation value. Accepts 'vertical' or 'horizontal'.
* @see atom.widget.Slider.Orientation
*/
@XmlAttribute(name = "orientation")
public Orientation getOrientation() {
return orientation;
}
/**
* Initialize slider orientation.
*
* @param newOrientation slider orientation value. Accepts 'vertical'
* or 'horizontal'.
* @see atom.widget.Slider.Orientation
*/
public void setOrientation(final Orientation newOrientation) {
orientation = newOrientation;
}
/**
* Get slider animation value.
*
* @return slider animation value. Accepts 'fast' or 'slow'.
* @see atom.widget.Slider.Animate
*/
@XmlAttribute(name = "animate")
public Animate getAnimate() {
return animate;
}
/**
* Initialize slider animation.
*
* @param newAnimate slider animation value. Accepts 'fast' or 'slow'.
* @see atom.widget.Slider.Animate
*/
public void setAnimate(final Animate newAnimate) {
animate = newAnimate;
}
/**
* Get single slider value.
* It should be used in case slider is initialized to single mode.
*
* @return single slider value.
*/
@XmlAttribute(name = "value")
public Float getValue() {
return value;
}
/**
* Initialize single slider value.
* It should be used in case slider is initialized to single mode.
*
* @param newValue new single value
* @see atom.widget.Slider#setRangeMode(boolean)
*/
public void setValue(final Float newValue) {
value = newValue;
fireChange(RANGE_VALUE_NAME, newValue);
}
/**
* Get range slider valueFrom.
* It should be used in case slider is initialized to range mode.
*
* @return range slider valueFrom.
*/
@XmlAttribute(name = "valueFrom")
public Float getValueFrom() {
return valueFrom;
}
/**
* Initialize range slider valueFrom.
* It should be used in case slider is initialized to range mode.
*
* @param newValueFrom new range from value
* @see atom.widget.Slider#setRangeMode(boolean)
*/
public void setValueFrom(final Float newValueFrom) {
valueFrom = newValueFrom;
fireChange(RANGE_VALUE_FROM_NAME, newValueFrom);
}
/**
* Get range slider valueTo.
* It should be used in case slider is initialized to range mode.
*
* @return range slider valueTo.
*/
@XmlAttribute(name = "valueTo")
public Float getValueTo() {
return valueTo;
}
/**
* Initialize range slider valueFrom.
* It should be used in case slider is initialized to range mode.
*
* @param newValueTo new range to value
* @see atom.widget.Slider#setRangeMode(boolean)
*/
public void setValueTo(final Float newValueTo) {
valueTo = newValueTo;
fireChange(RANGE_VALUE_TO_NAME, newValueTo);
}
/**
* Single/Range mode indicator.
* In case rangeMode mode - Slider#getValue() will be used as value.
* In case range mode - Slider#getValueFrom() and Slider#getValueTo()
* will be used as values.
*
* @return range mode state
*/
@XmlAttribute(name = "rangeMode")
public boolean isRangeMode() {
return rangeMode;
}
/**
* Single/Range mode switcher.
*
* @param isRange set to true to initialize range mode
*/
public void setRangeMode(final boolean isRange) {
rangeMode = isRange;
}
/**
* Get show/hide selected value indicator.
*
* @return show/hide selected value indicator.
*/
@XmlAttribute(name = "showSelected")
public boolean isShowSelected() {
return showSelected;
}
/**
* Initialize show/hide selected value indicator.
*
* @param show show/hide selected value indicator
*/
public void setShowSelected(final boolean show) {
showSelected = show;
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(final Object object) {
boolean areEquals;
if (this == object) {
areEquals = true;
} else {
if (object instanceof Slider) {
final Slider that = (Slider) object;
final boolean areValuesEqual;
if (isRangeMode()) {
areValuesEqual =
Objects.equals(valueFrom, that.valueFrom)
&& Objects.equals(valueTo, that.valueTo);
} else {
areValuesEqual = Objects.equals(value, that.value);
}
areEquals = super.equals(that) && areValuesEqual
&& Objects.equals(rangeMode, that.rangeMode)
&& Objects.equals(min, that.min)
&& Objects.equals(max, that.max)
&& Objects.equals(step, that.step)
&& Objects.equals(orientation, that.orientation)
&& Objects.equals(animate, that.animate)
&& Objects.equals(showSelected, that.showSelected);
} else {
areEquals = false;
}
}
return areEquals;
}
/**
* Create string from values. In case slider is initialized in single mode
* it will be {value}, in case range mode it will be {valueFrom, valueTo}.
* This method is used in:
*
* @return corresponded value or value range
* @see atom.widget.Slider#hashCode() and
* @see atom.widget.Slider#toString()
*/
public String getValuesStr() {
StringBuilder values = new StringBuilder("{");
if (isRangeMode()) {
if (valueFrom != null) {
values.append(valueFrom.toString());
}
values.append(", ");
if (valueTo != null) {
values.append(valueTo.toString());
}
} else {
if (value != null) {
values.append(value.toString());
}
}
values.append("}");
return values.toString();
}
/* (non-Javadoc)
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
return Objects.hash(
getViewId(),
getValuesStr(),
getMin(),
getMax(),
getStep(),
getOrientation(),
getAnimate(),
isRangeMode(),
isShowSelected());
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return String.format(
"Slider { "
+ "viewId='%s', "
+ "constraints='%s', "
+ "styleName='%s', "
+ "clickable='%b', "
+ "element='%s', "
+ "values='%s', "
+ "min='%f', "
+ "max='%f', "
+ "step='%f', "
+ "orientation='%s', "
+ "animate='%s', "
+ "rangeMode='%b', "
+ "showSelected='%b' "
+ "}",
getViewId(),
getConstraints(),
getStyleName(),
isClickable(),
getElement(),
getValuesStr(),
getMin(),
getMax(),
getStep(),
getOrientation().name(),
getAnimate().name(),
isRangeMode(),
isShowSelected()
);
}
/**
* Enum to select orientation of slider. Accepts 'vertical' or 'horizontal'
* values.
*/
@XmlType(namespace = AbstractView.LAYOUT_NAMESPACE)
public static enum Orientation {
/**
* Horizontal and vertical orientations.
*/
horizontal, vertical
}
/**
* Enum to select animation of slider. Accepts 'fast' or 'slow' values.
*/
@XmlType(namespace = AbstractView.LAYOUT_NAMESPACE)
public static enum Animate {
/**
* Fast and slow animation speed.
*/
fast, slow
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy