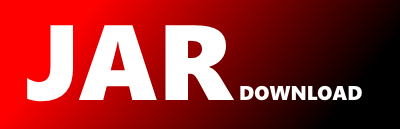
atom.content.pm.PackageInfo Maven / Gradle / Ivy
/*
* Copyright © 2014 Geeoz, and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* The Research Projects is dual-licensed under the GNU General Public
* License, version 2.0 (GPLv2) and the Geeoz Commercial License.
*
* Solely for non-commercial purposes. A purpose is non-commercial only if
* it is in no manner primarily intended for or directed toward commercial
* advantage or private monetary compensation.
*
* This Geeoz Software is supplied to you by Geeoz in consideration of your
* agreement to the following terms, and your use, installation, modification
* or redistribution of this Geeoz Software constitutes acceptance of these
* terms. If you do not agree with these terms, please do not use, install,
* modify or redistribute this Geeoz Software.
*
* Neither the name, trademarks, service marks or logos of Geeoz may be used
* to endorse or promote products derived from the Geeoz Software without
* specific prior written permission from Geeoz.
*
* The Geeoz Software is provided by Geeoz on an "AS IS" basis. GEEOZ MAKES NO
* WARRANTIES, EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION THE IMPLIED
* WARRANTIES OF NON-INFRINGEMENT, MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE, REGARDING THE GEEOZ SOFTWARE OR ITS USE AND OPERATION ALONE OR IN
* COMBINATION WITH YOUR PRODUCTS.
*
* IN NO EVENT SHALL GEEOZ BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL
* OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) ARISING IN ANY WAY OUT OF THE USE, REPRODUCTION, MODIFICATION
* AND/OR DISTRIBUTION OF THE GEEOZ SOFTWARE, HOWEVER CAUSED AND WHETHER UNDER
* THEORY OF CONTRACT, TORT (INCLUDING NEGLIGENCE), STRICT LIABILITY OR
* OTHERWISE, EVEN IF GEEOZ HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* A copy of the GNU General Public License is included in the distribution in
* the file LICENSE and at
*
* http://www.gnu.org/licenses/gpl-2.0.html
*
* If you are using the Research Projects for commercial purposes, we
* encourage you to visit
*
* http://products.geeoz.com/license
*
* for more details.
*
* This software or hardware and documentation may provide access to
* or information on content, products, and services from third parties.
* Geeoz and its affiliates are not responsible for and expressly disclaim
* all warranties of any kind with respect to third-party content, products,
* and services. Geeoz and its affiliates will not be responsible for any loss,
* costs, or damages incurred due to your access to or use of third-party
* content, products, or services. If a third-party content exists, the
* additional copyright notices and license terms applicable to portions of the
* software are set forth in the THIRD_PARTY_LICENSE_README file.
*
* Please contact Geeoz or visit www.geeoz.com if you need additional
* information or have any questions.
*/
package atom.content.pm;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Overall information about the contents of a package. This corresponds to all
* of the information collected from descriptor.
*
* @author Alex Voloshyn
* @version 1.9 4/20/14
*/
@XmlType(namespace = AbstractInfo.MANIFEST_NAMESPACE)
public final class PackageInfo {
/**
* Name of the package that this item is in.
*/
private String name;
/**
* An integer value that represents the version of the application code,
* relative to other versions.
*/
private int versionCode;
/**
* List of all activities in this package.
*/
private final List activities = new ArrayList<>();
/**
* List of all notifiers in this package.
*/
private final List notifiers = new ArrayList<>();
/**
* Initial package preferences.
*/
private String initialPreferences;
/**
* To help set-up a simple, out-of-the-box repository pre-populated with
* some content, system provides a way to configure such content using a xml
* format. This content can be imported either in a specific workspace, or
* imported by default in all predefined or new workspaces.
*/
private String initialContent;
/**
* To help to register data type definitions, to prepare repository
* structure, system provides a way to configure such data type definitions
* in CND format. This data type will imported into the application's
* corresponding workspace.
*/
private String dataTypes;
/**
* Retrieve the name of the package that this item is in.
*
* @return the name of the package that this item is in
*/
@XmlAttribute(name = "package", required = true)
public String getName() {
return name;
}
/**
* Set the name of the package that this item is in.
*
* @param pkgName the name of the package that this item is in
*/
public void setName(final String pkgName) {
name = pkgName;
}
/**
* Retrieve an integer value that represents the version of the code.
*
* @return an integer value that represents the version of the code
*/
@XmlAttribute(name = "versionCode")
public int getVersionCode() {
return versionCode;
}
/**
* Sets an integer value that represents the version of the code.
*
* @param code version of the application code
*/
public void setVersionCode(final int code) {
versionCode = code;
}
/**
* Add new activity information the activity info list for this package.
*
* @param activityInfo an activity information for this package
*/
public void addActivityInfo(final ActivityInfo activityInfo) {
activityInfo.setPackageInfo(this);
activities.add(activityInfo);
}
/**
* Returns list of all activities in this package.
*
* @return list of all activities in this package
*/
@XmlElement(name = "activity",
namespace = AbstractInfo.MANIFEST_NAMESPACE)
public List getActivities() {
return activities;
}
/**
* Add new activity information the activity info list for this package.
*
* @param notifierInfo an notifier information for this package
*/
public void addNotifierInfo(final NotifierInfo notifierInfo) {
notifierInfo.setPackageInfo(this);
notifiers.add(notifierInfo);
}
/**
* Returns list of all notifiers in this package.
*
* @return list of all notifiers in this package
*/
@XmlElement(name = "notifier",
namespace = AbstractInfo.MANIFEST_NAMESPACE)
public List getNotifiers() {
return notifiers;
}
/**
* Retrieve an initial preferences path.
*
* @return an initial preferences path
*/
public String getInitialPreferences() {
return initialPreferences;
}
/**
* Sets an initial preferences path.
*
* @param path an initial preferences path
*/
@XmlAttribute(name = "initial-preferences")
public void setInitialPreferences(final String path) {
initialPreferences = path;
}
/**
* Retrieve an initial content data path.
*
* @return an initial content data path
*/
@XmlAttribute(name = "initial-content")
public String getInitialContent() {
return initialContent;
}
/**
* Sets an initial content data path.
*
* @param path an initial content data path
*/
public void setInitialContent(final String path) {
initialContent = path;
}
/**
* Retrieve a data types definitions path.
*
* @return a data types definitions path
*/
@XmlAttribute(name = "data-types")
public String getDataTypes() {
return dataTypes;
}
/**
* Sets a data type definitions path.
*
* @param path a data types definitions path
*/
public void setDataTypes(final String path) {
dataTypes = path;
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(final Object object) {
boolean areEquals;
if (this == object) {
areEquals = true;
} else {
if (object instanceof PackageInfo) {
final PackageInfo that = (PackageInfo) object;
areEquals = Objects.equals(name, that.name)
&& Objects.equals(versionCode, that.versionCode)
&& Objects.equals(initialPreferences,
that.initialPreferences)
&& Objects.equals(initialContent, that.initialContent)
&& Objects.equals(versionCode, that.versionCode)
&& Objects.equals(activities, that.activities)
&& Objects.equals(notifiers, that.notifiers);
} else {
areEquals = false;
}
}
return areEquals;
}
/* (non-Javadoc)
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
return Objects.hash(name, versionCode);
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return String.format(
"PackageInfo { "
+ "package='%s', "
+ "versionCode='%d', "
+ "activities='%s', "
+ "notifiers='%s', "
+ "preferences='%s', "
+ "content='%s', "
+ "data-types='%s' "
+ "}",
name, versionCode, activities, notifiers, initialPreferences,
initialContent, dataTypes);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy