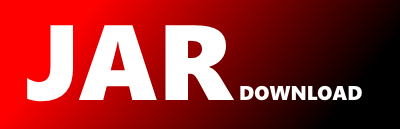
atom.ws.rs.ContextRestService Maven / Gradle / Ivy
/*
* Copyright © 2015 Geeoz, and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* The Research Projects is dual-licensed under the GNU General Public
* License, version 2.0 (GPLv2) and the Geeoz Commercial License.
*
* Solely for non-commercial purposes. A purpose is non-commercial only if
* it is in no manner primarily intended for or directed toward commercial
* advantage or private monetary compensation.
*
* This Geeoz Software is supplied to you by Geeoz in consideration of your
* agreement to the following terms, and your use, installation, modification
* or redistribution of this Geeoz Software constitutes acceptance of these
* terms. If you do not agree with these terms, please do not use, install,
* modify or redistribute this Geeoz Software.
*
* Neither the name, trademarks, service marks or logos of Geeoz may be used
* to endorse or promote products derived from the Geeoz Software without
* specific prior written permission from Geeoz.
*
* The Geeoz Software is provided by Geeoz on an "AS IS" basis. GEEOZ MAKES NO
* WARRANTIES, EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION THE IMPLIED
* WARRANTIES OF NON-INFRINGEMENT, MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE, REGARDING THE GEEOZ SOFTWARE OR ITS USE AND OPERATION ALONE OR IN
* COMBINATION WITH YOUR PRODUCTS.
*
* IN NO EVENT SHALL GEEOZ BE LIABLE FOR ANY SPECIAL, INDIRECT, INCIDENTAL
* OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) ARISING IN ANY WAY OUT OF THE USE, REPRODUCTION, MODIFICATION
* AND/OR DISTRIBUTION OF THE GEEOZ SOFTWARE, HOWEVER CAUSED AND WHETHER UNDER
* THEORY OF CONTRACT, TORT (INCLUDING NEGLIGENCE), STRICT LIABILITY OR
* OTHERWISE, EVEN IF GEEOZ HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* A copy of the GNU General Public License is included in the distribution in
* the file LICENSE and at
*
* http://www.gnu.org/licenses/gpl-2.0.html
*
* If you are using the Research Projects for commercial purposes, we
* encourage you to visit
*
* http://products.geeoz.com/license
*
* for more details.
*
* This software or hardware and documentation may provide access to
* or information on content, products, and services from third parties.
* Geeoz and its affiliates are not responsible for and expressly disclaim
* all warranties of any kind with respect to third-party content, products,
* and services. Geeoz and its affiliates will not be responsible for any loss,
* costs, or damages incurred due to your access to or use of third-party
* content, products, or services. If a third-party content exists, the
* additional copyright notices and license terms applicable to portions of the
* software are set forth in the THIRD_PARTY_LICENSE_README file.
*
* Please contact Geeoz or visit www.geeoz.com if you need additional
* information or have any questions.
*/
package atom.ws.rs;
import atom.app.ActivityThread;
import atom.app.ClientNotifier;
import atom.beans.ChangeSet;
import atom.ws.model.AbstractContextService;
import atom.ws.model.AbstractMessage;
import atom.ws.model.ChangeSetFactory;
import atom.ws.model.ChangeSetMessage;
import atom.ws.model.ClientRequestContext;
import atom.ws.model.ContextService;
import atom.ws.model.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import java.util.List;
import java.util.Queue;
import java.util.concurrent.LinkedBlockingQueue;
/**
* Default implementation of the web context service.
*
* @author Serge Voloshyn
* @version 1.3 3/1/15
*/
@Path("/context")
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public final class ContextRestService extends AbstractContextService {
/**
* Name of the session attribute containing messages for client.
*/
private static final String MESSAGES_FOR_CLIENT = "MESSAGES_FOR_CLIENT_";
/**
* Get new message for the client.
*
* @param httpRequest current http request
* @param clientId client identifier
* @return new message for the client
*/
@POST
@Path("/get-message/{clientId:.+}")
public AbstractMessage getMessages(
@Context final HttpServletRequest httpRequest,
@PathParam("clientId") final String clientId) {
return getMessagesQueue(httpRequest.getSession(true), clientId).poll();
}
/**
* Send message to server.
*
* @param httpRequest current http request
* @param httpResponse current http response
* @param message message to send
*/
@POST
@Path("/send-message")
public void sendMessage(
@Context final HttpServletRequest httpRequest,
@Context final HttpServletResponse httpResponse,
final AbstractMessage message) {
final ClientRequestContext requestContext
= new ClientRequestContext(
httpRequest.getSession(true),
httpRequest.getLocale(),
message.getCookies());
new Thread(() -> {
setClientNotifier(message.getClientId(),
requestContext,
new RestClientNotifier(message.getClientId(),
requestContext.getSession()));
processMessage(message,
requestContext,
new ServiceCallbackImpl(requestContext));
}).start();
}
/**
* Get messages queue from the client's session.
*
* @param session current client session
* @param clientId client identifier
* @return existing messages queue or new queue if not exists yet
*/
@SuppressWarnings("unchecked")
private static Queue getMessagesQueue(
final HttpSession session, final String clientId) {
Queue messageQueue
= (Queue) session
.getAttribute(MESSAGES_FOR_CLIENT.concat(clientId));
if (messageQueue == null) {
synchronized (session) {
messageQueue
= (Queue) session
.getAttribute(MESSAGES_FOR_CLIENT.concat(clientId));
if (messageQueue == null) {
messageQueue = new LinkedBlockingQueue<>();
session.setAttribute(MESSAGES_FOR_CLIENT.concat(clientId),
messageQueue);
}
}
}
return messageQueue;
}
/**
* Publish change set message for client.
*
* @param clientId client identifier
* @param changeSets change sets list
* @param theCookies new cookies
* @param session client http session
* @param requestedByClient whether changeSet was created
* after client request
*/
private static void publishChangeSetForClient(
final String clientId,
final List changeSets,
final Cookie[] theCookies,
final HttpSession session,
final boolean requestedByClient) {
final ChangeSetMessage responseMessage = new ChangeSetMessage();
responseMessage.setClientId(clientId);
responseMessage.setChangeSet(changeSets);
responseMessage.setCookies(theCookies);
responseMessage.setRequestedByClient(requestedByClient);
if (!getMessagesQueue(session, clientId).offer(responseMessage)) {
System.err.println(String
.format("Web messaging queue is full for client id '%s'",
clientId));
}
}
/**
* Service callback implementation.
*/
private static final class ServiceCallbackImpl implements ServiceCallback {
/**
* Request context.
*/
private final ClientRequestContext context;
/**
* Default constructor.
*
* @param aContext request context
*/
public ServiceCallbackImpl(final ClientRequestContext aContext) {
context = aContext;
}
@Override
public void onFinish(final String clientId,
final List changeSet) {
publishChangeSetForClient(clientId, changeSet,
context.getResponseCookies(), context.getSession(),
true);
}
}
/**
* REST client notifier.
*/
private static final class RestClientNotifier implements ClientNotifier {
/**
* Client identifier.
*/
private final String clientId;
/**
* Client http session.
*/
private final HttpSession session;
/**
* Default constructor.
*
* @param aClientId client identifier
* @param aSession client http session
*/
private RestClientNotifier(final String aClientId,
final HttpSession aSession) {
clientId = aClientId;
session = aSession;
}
@Override
public void onViewChange(final ActivityThread thread) {
ContextService.checkChangedActivities(thread);
final List changeSets = ChangeSetFactory
.prepareChangeSet(getClientLayers(thread), thread);
publishChangeSetForClient(
clientId, changeSets, null, session, false);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy