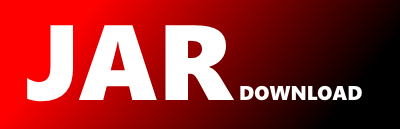
com.ean.wsapi.hotel.v3.HotelConfirmation Maven / Gradle / Ivy
package com.ean.wsapi.hotel.v3;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for HotelConfirmation complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="HotelConfirmation">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="supplierId" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="chainCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="creditCardType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="arrivalDate" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="departureDate" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="confirmationNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="cancellationNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="RateInfo" type="{http://v3.hotel.wsapi.ean.com/}HotelRateInfo" minOccurs="0"/>
* <element name="RateInfos" type="{http://v3.hotel.wsapi.ean.com/}RateInfos" minOccurs="0"/>
* <element name="numberOfAdults" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="numberOfChildren" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="cancellationPolicy" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="affiliateConfirmationId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="smokingPreference" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="supplierPropertyId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="roomTypeCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="roomType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="rateCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="rateType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="rateDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="roomDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="status" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="nonRefundable" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="locale" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="CouponInformation" type="{http://v3.hotel.wsapi.ean.com/}CouponInformation" minOccurs="0"/>
* <element name="ReservationGuest" type="{http://v3.hotel.wsapi.ean.com/}ReservationGuest" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Hotel" type="{http://v3.hotel.wsapi.ean.com/}Hotel" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ConfirmationExtras" type="{http://v3.hotel.wsapi.ean.com/}ConfirmationExtras" minOccurs="0"/>
* <element name="nights" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "HotelConfirmation", propOrder = {
"supplierId",
"chainCode",
"creditCardType",
"arrivalDate",
"departureDate",
"confirmationNumber",
"cancellationNumber",
"rateInfo",
"rateInfos",
"numberOfAdults",
"numberOfChildren",
"cancellationPolicy",
"affiliateConfirmationId",
"smokingPreference",
"supplierPropertyId",
"roomTypeCode",
"roomType",
"rateCode",
"rateType",
"rateDescription",
"roomDescription",
"status",
"nonRefundable",
"locale",
"couponInformation",
"reservationGuest",
"hotel",
"confirmationExtras",
"nights"
})
public class HotelConfirmation {
protected long supplierId;
protected String chainCode;
protected String creditCardType;
protected String arrivalDate;
protected String departureDate;
protected String confirmationNumber;
protected String cancellationNumber;
@XmlElement(name = "RateInfo")
protected HotelRateInfo rateInfo;
@XmlElement(name = "RateInfos")
protected RateInfos rateInfos;
protected int numberOfAdults;
protected int numberOfChildren;
protected String cancellationPolicy;
protected String affiliateConfirmationId;
protected String smokingPreference;
protected String supplierPropertyId;
protected String roomTypeCode;
protected String roomType;
protected String rateCode;
protected String rateType;
protected String rateDescription;
protected String roomDescription;
protected String status;
protected Boolean nonRefundable;
protected String locale;
@XmlElement(name = "CouponInformation")
protected CouponInformation couponInformation;
@XmlElement(name = "ReservationGuest")
protected List reservationGuest;
@XmlElement(name = "Hotel")
protected List hotel;
@XmlElement(name = "ConfirmationExtras")
protected ConfirmationExtras confirmationExtras;
protected Integer nights;
/**
* Gets the value of the supplierId property.
*
*/
public long getSupplierId() {
return supplierId;
}
/**
* Sets the value of the supplierId property.
*
*/
public void setSupplierId(long value) {
this.supplierId = value;
}
/**
* Gets the value of the chainCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getChainCode() {
return chainCode;
}
/**
* Sets the value of the chainCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setChainCode(String value) {
this.chainCode = value;
}
/**
* Gets the value of the creditCardType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCreditCardType() {
return creditCardType;
}
/**
* Sets the value of the creditCardType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCreditCardType(String value) {
this.creditCardType = value;
}
/**
* Gets the value of the arrivalDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getArrivalDate() {
return arrivalDate;
}
/**
* Sets the value of the arrivalDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setArrivalDate(String value) {
this.arrivalDate = value;
}
/**
* Gets the value of the departureDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDepartureDate() {
return departureDate;
}
/**
* Sets the value of the departureDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDepartureDate(String value) {
this.departureDate = value;
}
/**
* Gets the value of the confirmationNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getConfirmationNumber() {
return confirmationNumber;
}
/**
* Sets the value of the confirmationNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setConfirmationNumber(String value) {
this.confirmationNumber = value;
}
/**
* Gets the value of the cancellationNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCancellationNumber() {
return cancellationNumber;
}
/**
* Sets the value of the cancellationNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCancellationNumber(String value) {
this.cancellationNumber = value;
}
/**
* Gets the value of the rateInfo property.
*
* @return
* possible object is
* {@link HotelRateInfo }
*
*/
public HotelRateInfo getRateInfo() {
return rateInfo;
}
/**
* Sets the value of the rateInfo property.
*
* @param value
* allowed object is
* {@link HotelRateInfo }
*
*/
public void setRateInfo(HotelRateInfo value) {
this.rateInfo = value;
}
/**
* Gets the value of the rateInfos property.
*
* @return
* possible object is
* {@link RateInfos }
*
*/
public RateInfos getRateInfos() {
return rateInfos;
}
/**
* Sets the value of the rateInfos property.
*
* @param value
* allowed object is
* {@link RateInfos }
*
*/
public void setRateInfos(RateInfos value) {
this.rateInfos = value;
}
/**
* Gets the value of the numberOfAdults property.
*
*/
public int getNumberOfAdults() {
return numberOfAdults;
}
/**
* Sets the value of the numberOfAdults property.
*
*/
public void setNumberOfAdults(int value) {
this.numberOfAdults = value;
}
/**
* Gets the value of the numberOfChildren property.
*
*/
public int getNumberOfChildren() {
return numberOfChildren;
}
/**
* Sets the value of the numberOfChildren property.
*
*/
public void setNumberOfChildren(int value) {
this.numberOfChildren = value;
}
/**
* Gets the value of the cancellationPolicy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCancellationPolicy() {
return cancellationPolicy;
}
/**
* Sets the value of the cancellationPolicy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCancellationPolicy(String value) {
this.cancellationPolicy = value;
}
/**
* Gets the value of the affiliateConfirmationId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAffiliateConfirmationId() {
return affiliateConfirmationId;
}
/**
* Sets the value of the affiliateConfirmationId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAffiliateConfirmationId(String value) {
this.affiliateConfirmationId = value;
}
/**
* Gets the value of the smokingPreference property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSmokingPreference() {
return smokingPreference;
}
/**
* Sets the value of the smokingPreference property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSmokingPreference(String value) {
this.smokingPreference = value;
}
/**
* Gets the value of the supplierPropertyId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSupplierPropertyId() {
return supplierPropertyId;
}
/**
* Sets the value of the supplierPropertyId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSupplierPropertyId(String value) {
this.supplierPropertyId = value;
}
/**
* Gets the value of the roomTypeCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoomTypeCode() {
return roomTypeCode;
}
/**
* Sets the value of the roomTypeCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRoomTypeCode(String value) {
this.roomTypeCode = value;
}
/**
* Gets the value of the roomType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoomType() {
return roomType;
}
/**
* Sets the value of the roomType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRoomType(String value) {
this.roomType = value;
}
/**
* Gets the value of the rateCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRateCode() {
return rateCode;
}
/**
* Sets the value of the rateCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRateCode(String value) {
this.rateCode = value;
}
/**
* Gets the value of the rateType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRateType() {
return rateType;
}
/**
* Sets the value of the rateType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRateType(String value) {
this.rateType = value;
}
/**
* Gets the value of the rateDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRateDescription() {
return rateDescription;
}
/**
* Sets the value of the rateDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRateDescription(String value) {
this.rateDescription = value;
}
/**
* Gets the value of the roomDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoomDescription() {
return roomDescription;
}
/**
* Sets the value of the roomDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRoomDescription(String value) {
this.roomDescription = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStatus(String value) {
this.status = value;
}
/**
* Gets the value of the nonRefundable property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isNonRefundable() {
return nonRefundable;
}
/**
* Sets the value of the nonRefundable property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setNonRefundable(Boolean value) {
this.nonRefundable = value;
}
/**
* Gets the value of the locale property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLocale() {
return locale;
}
/**
* Sets the value of the locale property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLocale(String value) {
this.locale = value;
}
/**
* Gets the value of the couponInformation property.
*
* @return
* possible object is
* {@link CouponInformation }
*
*/
public CouponInformation getCouponInformation() {
return couponInformation;
}
/**
* Sets the value of the couponInformation property.
*
* @param value
* allowed object is
* {@link CouponInformation }
*
*/
public void setCouponInformation(CouponInformation value) {
this.couponInformation = value;
}
/**
* Gets the value of the reservationGuest property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the reservationGuest property.
*
*
* For example, to add a new item, do as follows:
*
* getReservationGuest().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ReservationGuest }
*
*
*/
public List getReservationGuest() {
if (reservationGuest == null) {
reservationGuest = new ArrayList();
}
return this.reservationGuest;
}
/**
* Gets the value of the hotel property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the hotel property.
*
*
* For example, to add a new item, do as follows:
*
* getHotel().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Hotel }
*
*
*/
public List getHotel() {
if (hotel == null) {
hotel = new ArrayList();
}
return this.hotel;
}
/**
* Gets the value of the confirmationExtras property.
*
* @return
* possible object is
* {@link ConfirmationExtras }
*
*/
public ConfirmationExtras getConfirmationExtras() {
return confirmationExtras;
}
/**
* Sets the value of the confirmationExtras property.
*
* @param value
* allowed object is
* {@link ConfirmationExtras }
*
*/
public void setConfirmationExtras(ConfirmationExtras value) {
this.confirmationExtras = value;
}
/**
* Gets the value of the nights property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getNights() {
return nights;
}
/**
* Sets the value of the nights property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setNights(Integer value) {
this.nights = value;
}
}