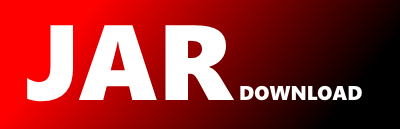
com.ean.wsapi.hotel.v3.HotelDetails Maven / Gradle / Ivy
package com.ean.wsapi.hotel.v3;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for HotelDetails complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="HotelDetails">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="nativeCurrencyCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="numberOfRooms" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="numberOfFloors" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="checkInTime" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="checkOutTime" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="extraPersonCharge" type="{http://www.w3.org/2001/XMLSchema}float" minOccurs="0"/>
* <element name="propertyInformation" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="areaInformation" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="propertyDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="hotelPolicy" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="guaranteePolicy" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="depositPolicy" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="guaranteeCreditCardsAccepted" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="depositCreditCardsAccepted" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="roomInformation" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="drivingDirections" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="checkInInstructions" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="nationalRatingsDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="knowBeforeYouGoDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="roomFeesDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="mandatoryFeesDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="renovationsDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="locationDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="diningDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="amenitiesDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="businessAmenitiesDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="roomDetailDescription" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "HotelDetails", propOrder = {
"nativeCurrencyCode",
"numberOfRooms",
"numberOfFloors",
"checkInTime",
"checkOutTime",
"extraPersonCharge",
"propertyInformation",
"areaInformation",
"propertyDescription",
"hotelPolicy",
"guaranteePolicy",
"depositPolicy",
"guaranteeCreditCardsAccepted",
"depositCreditCardsAccepted",
"roomInformation",
"drivingDirections",
"checkInInstructions",
"nationalRatingsDescription",
"knowBeforeYouGoDescription",
"roomFeesDescription",
"mandatoryFeesDescription",
"renovationsDescription",
"locationDescription",
"diningDescription",
"amenitiesDescription",
"businessAmenitiesDescription",
"roomDetailDescription"
})
public class HotelDetails {
protected String nativeCurrencyCode;
protected Integer numberOfRooms;
protected Integer numberOfFloors;
protected String checkInTime;
protected String checkOutTime;
protected Float extraPersonCharge;
protected String propertyInformation;
protected String areaInformation;
protected String propertyDescription;
protected String hotelPolicy;
protected String guaranteePolicy;
protected String depositPolicy;
protected String guaranteeCreditCardsAccepted;
protected String depositCreditCardsAccepted;
protected String roomInformation;
protected String drivingDirections;
protected String checkInInstructions;
protected String nationalRatingsDescription;
protected String knowBeforeYouGoDescription;
protected String roomFeesDescription;
protected String mandatoryFeesDescription;
protected String renovationsDescription;
protected String locationDescription;
protected String diningDescription;
protected String amenitiesDescription;
protected String businessAmenitiesDescription;
protected String roomDetailDescription;
/**
* Gets the value of the nativeCurrencyCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNativeCurrencyCode() {
return nativeCurrencyCode;
}
/**
* Sets the value of the nativeCurrencyCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNativeCurrencyCode(String value) {
this.nativeCurrencyCode = value;
}
/**
* Gets the value of the numberOfRooms property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getNumberOfRooms() {
return numberOfRooms;
}
/**
* Sets the value of the numberOfRooms property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setNumberOfRooms(Integer value) {
this.numberOfRooms = value;
}
/**
* Gets the value of the numberOfFloors property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getNumberOfFloors() {
return numberOfFloors;
}
/**
* Sets the value of the numberOfFloors property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setNumberOfFloors(Integer value) {
this.numberOfFloors = value;
}
/**
* Gets the value of the checkInTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCheckInTime() {
return checkInTime;
}
/**
* Sets the value of the checkInTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCheckInTime(String value) {
this.checkInTime = value;
}
/**
* Gets the value of the checkOutTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCheckOutTime() {
return checkOutTime;
}
/**
* Sets the value of the checkOutTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCheckOutTime(String value) {
this.checkOutTime = value;
}
/**
* Gets the value of the extraPersonCharge property.
*
* @return
* possible object is
* {@link Float }
*
*/
public Float getExtraPersonCharge() {
return extraPersonCharge;
}
/**
* Sets the value of the extraPersonCharge property.
*
* @param value
* allowed object is
* {@link Float }
*
*/
public void setExtraPersonCharge(Float value) {
this.extraPersonCharge = value;
}
/**
* Gets the value of the propertyInformation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPropertyInformation() {
return propertyInformation;
}
/**
* Sets the value of the propertyInformation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPropertyInformation(String value) {
this.propertyInformation = value;
}
/**
* Gets the value of the areaInformation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAreaInformation() {
return areaInformation;
}
/**
* Sets the value of the areaInformation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAreaInformation(String value) {
this.areaInformation = value;
}
/**
* Gets the value of the propertyDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPropertyDescription() {
return propertyDescription;
}
/**
* Sets the value of the propertyDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPropertyDescription(String value) {
this.propertyDescription = value;
}
/**
* Gets the value of the hotelPolicy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHotelPolicy() {
return hotelPolicy;
}
/**
* Sets the value of the hotelPolicy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHotelPolicy(String value) {
this.hotelPolicy = value;
}
/**
* Gets the value of the guaranteePolicy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGuaranteePolicy() {
return guaranteePolicy;
}
/**
* Sets the value of the guaranteePolicy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGuaranteePolicy(String value) {
this.guaranteePolicy = value;
}
/**
* Gets the value of the depositPolicy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDepositPolicy() {
return depositPolicy;
}
/**
* Sets the value of the depositPolicy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDepositPolicy(String value) {
this.depositPolicy = value;
}
/**
* Gets the value of the guaranteeCreditCardsAccepted property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGuaranteeCreditCardsAccepted() {
return guaranteeCreditCardsAccepted;
}
/**
* Sets the value of the guaranteeCreditCardsAccepted property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGuaranteeCreditCardsAccepted(String value) {
this.guaranteeCreditCardsAccepted = value;
}
/**
* Gets the value of the depositCreditCardsAccepted property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDepositCreditCardsAccepted() {
return depositCreditCardsAccepted;
}
/**
* Sets the value of the depositCreditCardsAccepted property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDepositCreditCardsAccepted(String value) {
this.depositCreditCardsAccepted = value;
}
/**
* Gets the value of the roomInformation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoomInformation() {
return roomInformation;
}
/**
* Sets the value of the roomInformation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRoomInformation(String value) {
this.roomInformation = value;
}
/**
* Gets the value of the drivingDirections property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDrivingDirections() {
return drivingDirections;
}
/**
* Sets the value of the drivingDirections property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDrivingDirections(String value) {
this.drivingDirections = value;
}
/**
* Gets the value of the checkInInstructions property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCheckInInstructions() {
return checkInInstructions;
}
/**
* Sets the value of the checkInInstructions property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCheckInInstructions(String value) {
this.checkInInstructions = value;
}
/**
* Gets the value of the nationalRatingsDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNationalRatingsDescription() {
return nationalRatingsDescription;
}
/**
* Sets the value of the nationalRatingsDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNationalRatingsDescription(String value) {
this.nationalRatingsDescription = value;
}
/**
* Gets the value of the knowBeforeYouGoDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKnowBeforeYouGoDescription() {
return knowBeforeYouGoDescription;
}
/**
* Sets the value of the knowBeforeYouGoDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKnowBeforeYouGoDescription(String value) {
this.knowBeforeYouGoDescription = value;
}
/**
* Gets the value of the roomFeesDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoomFeesDescription() {
return roomFeesDescription;
}
/**
* Sets the value of the roomFeesDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRoomFeesDescription(String value) {
this.roomFeesDescription = value;
}
/**
* Gets the value of the mandatoryFeesDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMandatoryFeesDescription() {
return mandatoryFeesDescription;
}
/**
* Sets the value of the mandatoryFeesDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMandatoryFeesDescription(String value) {
this.mandatoryFeesDescription = value;
}
/**
* Gets the value of the renovationsDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRenovationsDescription() {
return renovationsDescription;
}
/**
* Sets the value of the renovationsDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRenovationsDescription(String value) {
this.renovationsDescription = value;
}
/**
* Gets the value of the locationDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLocationDescription() {
return locationDescription;
}
/**
* Sets the value of the locationDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLocationDescription(String value) {
this.locationDescription = value;
}
/**
* Gets the value of the diningDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDiningDescription() {
return diningDescription;
}
/**
* Sets the value of the diningDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDiningDescription(String value) {
this.diningDescription = value;
}
/**
* Gets the value of the amenitiesDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAmenitiesDescription() {
return amenitiesDescription;
}
/**
* Sets the value of the amenitiesDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAmenitiesDescription(String value) {
this.amenitiesDescription = value;
}
/**
* Gets the value of the businessAmenitiesDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBusinessAmenitiesDescription() {
return businessAmenitiesDescription;
}
/**
* Sets the value of the businessAmenitiesDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBusinessAmenitiesDescription(String value) {
this.businessAmenitiesDescription = value;
}
/**
* Gets the value of the roomDetailDescription property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoomDetailDescription() {
return roomDetailDescription;
}
/**
* Sets the value of the roomDetailDescription property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRoomDetailDescription(String value) {
this.roomDetailDescription = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy