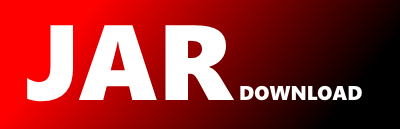
com.ean.wsapi.hotel.v3.HotelImage Maven / Gradle / Ivy
package com.ean.wsapi.hotel.v3;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for HotelImage complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="HotelImage">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="hotelImageId" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="category" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="type" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="caption" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="url" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="thumbnailUrl" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="supplierId" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="width" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="height" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="byteSize" type="{http://www.w3.org/2001/XMLSchema}long"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "HotelImage", propOrder = {
"hotelImageId",
"name",
"category",
"type",
"caption",
"url",
"thumbnailUrl",
"supplierId",
"width",
"height",
"byteSize"
})
public class HotelImage {
protected long hotelImageId;
protected String name;
protected int category;
protected int type;
protected String caption;
protected String url;
protected String thumbnailUrl;
protected long supplierId;
protected long width;
protected long height;
protected long byteSize;
/**
* Gets the value of the hotelImageId property.
*
*/
public long getHotelImageId() {
return hotelImageId;
}
/**
* Sets the value of the hotelImageId property.
*
*/
public void setHotelImageId(long value) {
this.hotelImageId = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the category property.
*
*/
public int getCategory() {
return category;
}
/**
* Sets the value of the category property.
*
*/
public void setCategory(int value) {
this.category = value;
}
/**
* Gets the value of the type property.
*
*/
public int getType() {
return type;
}
/**
* Sets the value of the type property.
*
*/
public void setType(int value) {
this.type = value;
}
/**
* Gets the value of the caption property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCaption() {
return caption;
}
/**
* Sets the value of the caption property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCaption(String value) {
this.caption = value;
}
/**
* Gets the value of the url property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUrl() {
return url;
}
/**
* Sets the value of the url property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUrl(String value) {
this.url = value;
}
/**
* Gets the value of the thumbnailUrl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getThumbnailUrl() {
return thumbnailUrl;
}
/**
* Sets the value of the thumbnailUrl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setThumbnailUrl(String value) {
this.thumbnailUrl = value;
}
/**
* Gets the value of the supplierId property.
*
*/
public long getSupplierId() {
return supplierId;
}
/**
* Sets the value of the supplierId property.
*
*/
public void setSupplierId(long value) {
this.supplierId = value;
}
/**
* Gets the value of the width property.
*
*/
public long getWidth() {
return width;
}
/**
* Sets the value of the width property.
*
*/
public void setWidth(long value) {
this.width = value;
}
/**
* Gets the value of the height property.
*
*/
public long getHeight() {
return height;
}
/**
* Sets the value of the height property.
*
*/
public void setHeight(long value) {
this.height = value;
}
/**
* Gets the value of the byteSize property.
*
*/
public long getByteSize() {
return byteSize;
}
/**
* Sets the value of the byteSize property.
*
*/
public void setByteSize(long value) {
this.byteSize = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy