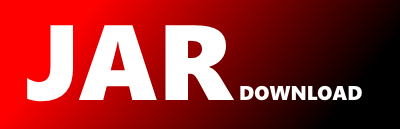
com.ean.wsapi.hotel.v3.HotelServices Maven / Gradle / Ivy
package com.ean.wsapi.hotel.v3;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.ws.RequestWrapper;
import javax.xml.ws.ResponseWrapper;
/**
* This class was generated by the JAX-WS RI.
* JAX-WS RI 2.2.8
* Generated source version: 2.2
*
*/
@WebService(name = "HotelServices", targetNamespace = "http://v3.hotel.wsapi.ean.com/")
@XmlSeeAlso({
ObjectFactory.class
})
public interface HotelServices {
/**
*
* @param hotelRoomImageRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelRoomImageResponse
*/
@WebMethod
@WebResult(name = "HotelRoomImageResponse", targetNamespace = "")
@RequestWrapper(localName = "getRoomImage", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetRoomImage")
@ResponseWrapper(localName = "getRoomImageResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetRoomImageResponse")
public HotelRoomImageResponse getRoomImage(
@WebParam(name = "HotelRoomImageRequest", targetNamespace = "")
HotelRoomImageRequest hotelRoomImageRequest);
/**
*
* @param hotelListRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelListResponse
*/
@WebMethod
@WebResult(name = "HotelListResponse", targetNamespace = "")
@RequestWrapper(localName = "getList", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetList")
@ResponseWrapper(localName = "getListResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetListResponse")
public HotelListResponse getList(
@WebParam(name = "HotelListRequest", targetNamespace = "")
HotelListRequest hotelListRequest);
/**
*
* @param hotelRoomAvailabilityRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelRoomAvailabilityResponse
*/
@WebMethod
@WebResult(name = "HotelRoomAvailabilityResponse", targetNamespace = "")
@RequestWrapper(localName = "getAvailability", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetAvailability")
@ResponseWrapper(localName = "getAvailabilityResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetAvailabilityResponse")
public HotelRoomAvailabilityResponse getAvailability(
@WebParam(name = "HotelRoomAvailabilityRequest", targetNamespace = "")
HotelRoomAvailabilityRequest hotelRoomAvailabilityRequest);
/**
*
* @param hotelRoomCancellationRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelRoomCancellationResponse
*/
@WebMethod
@WebResult(name = "HotelRoomCancellationResponse", targetNamespace = "")
@RequestWrapper(localName = "getCancellation", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetCancellation")
@ResponseWrapper(localName = "getCancellationResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetCancellationResponse")
public HotelRoomCancellationResponse getCancellation(
@WebParam(name = "HotelRoomCancellationRequest", targetNamespace = "")
HotelRoomCancellationRequest hotelRoomCancellationRequest);
/**
*
* @param locationInfoRequest
* @return
* returns com.ean.wsapi.hotel.v3.LocationInfoResponse
*/
@WebMethod
@WebResult(name = "LocationInfoResponse", targetNamespace = "")
@RequestWrapper(localName = "getGeoLocation", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetGeoLocation")
@ResponseWrapper(localName = "getGeoLocationResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetGeoLocationResponse")
public LocationInfoResponse getGeoLocation(
@WebParam(name = "LocationInfoRequest", targetNamespace = "")
LocationInfoRequest locationInfoRequest);
/**
*
* @param alternatePropertyListRequest
* @return
* returns com.ean.wsapi.hotel.v3.AlternatePropertyListResponse
*/
@WebMethod
@WebResult(name = "AlternatePropertyListResponse", targetNamespace = "")
@RequestWrapper(localName = "getAlternateProperties", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetAlternateProperties")
@ResponseWrapper(localName = "getAlternatePropertiesResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetAlternatePropertiesResponse")
public AlternatePropertyListResponse getAlternateProperties(
@WebParam(name = "AlternatePropertyListRequest", targetNamespace = "")
AlternatePropertyListRequest alternatePropertyListRequest);
/**
*
* @param hotelRoomReservationRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelRoomReservationResponse
*/
@WebMethod
@WebResult(name = "HotelRoomReservationResponse", targetNamespace = "")
@RequestWrapper(localName = "getReservation", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetReservation")
@ResponseWrapper(localName = "getReservationResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetReservationResponse")
public HotelRoomReservationResponse getReservation(
@WebParam(name = "HotelRoomReservationRequest", targetNamespace = "")
HotelRoomReservationRequest hotelRoomReservationRequest);
/**
*
* @param hotelItineraryRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelItineraryResponse
*/
@WebMethod
@WebResult(name = "HotelItineraryResponse", targetNamespace = "")
@RequestWrapper(localName = "getItinerary", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetItinerary")
@ResponseWrapper(localName = "getItineraryResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetItineraryResponse")
public HotelItineraryResponse getItinerary(
@WebParam(name = "HotelItineraryRequest", targetNamespace = "")
HotelItineraryRequest hotelItineraryRequest);
/**
*
* @param hotelRateRulesRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelRateRulesResponse
*/
@WebMethod
@WebResult(name = "HotelRateRulesResponse", targetNamespace = "")
@RequestWrapper(localName = "getRateRules", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetRateRules")
@ResponseWrapper(localName = "getRateRulesResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetRateRulesResponse")
public HotelRateRulesResponse getRateRules(
@WebParam(name = "HotelRateRulesRequest", targetNamespace = "")
HotelRateRulesRequest hotelRateRulesRequest);
/**
*
* @param hotelPaymentRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelPaymentResponse
*/
@WebMethod
@WebResult(name = "HotelPaymentResponse", targetNamespace = "")
@RequestWrapper(localName = "getPaymentInfo", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetPaymentInfo")
@ResponseWrapper(localName = "getPaymentInfoResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetPaymentInfoResponse")
public HotelPaymentResponse getPaymentInfo(
@WebParam(name = "HotelPaymentRequest", targetNamespace = "")
V3HotelPaymentRequest hotelPaymentRequest);
/**
*
* @param pingRequest
* @return
* returns com.ean.wsapi.hotel.v3.PingResponse
*/
@WebMethod
@WebResult(name = "PingResponse", targetNamespace = "")
@RequestWrapper(localName = "getPing", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetPing")
@ResponseWrapper(localName = "getPingResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetPingResponse")
public PingResponse getPing(
@WebParam(name = "PingRequest", targetNamespace = "")
PingRequest pingRequest);
/**
*
* @param hotelInformationRequest
* @return
* returns com.ean.wsapi.hotel.v3.HotelInformationResponse
*/
@WebMethod
@WebResult(name = "HotelInformationResponse", targetNamespace = "")
@RequestWrapper(localName = "getInformation", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetInformation")
@ResponseWrapper(localName = "getInformationResponse", targetNamespace = "http://v3.hotel.wsapi.ean.com/", className = "com.ean.wsapi.hotel.v3.GetInformationResponse")
public HotelInformationResponse getInformation(
@WebParam(name = "HotelInformationRequest", targetNamespace = "")
HotelInformationRequest hotelInformationRequest);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy