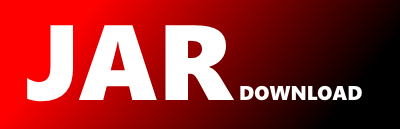
com.geeoz.ean.dsl.GetHotelListBuilder Maven / Gradle / Ivy
/*
* Copyright 2013 Geeoz Software
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.geeoz.ean.dsl;
import com.ean.wsapi.hotel.v3.HotelListRequest;
import com.ean.wsapi.hotel.v3.HotelListResponse;
import com.ean.wsapi.hotel.v3.Room;
import com.ean.wsapi.hotel.v3.RoomGroup;
import com.ean.wsapi.hotel.v3.SearchRadiusUnitType;
import com.ean.wsapi.hotel.v3.SortType;
/**
* Retrieve a list of hotels by location or a list of specific hotelIds.
*
* This method can be used to return hotels with available rooms for a provided
* date range, or to return a list of all active properties within the specified
* location without any availability information.
*
* This method supports multiple filters and methods of specifying the desired
* location to allow a variety of front-end search options, such as searching by
* airport code or a visualization on a map.
*
* @author Alex Voloshyn
* @version 1.0 7/13/2013
*/
public final class GetHotelListBuilder extends
AbstractHotelBuilder {
/**
* Every search for available hotels requires a minimum of a date range,
* room count and adult guest count, and a location or hotelId list.
*/
private final HotelListRequest request;
/**
* An empty constructor with request initialization.
*/
public GetHotelListBuilder() {
request = new HotelListRequest();
baseHotelInit(request);
}
/**
* Setup a date range for hotel search.
*
* @param arrival check-in date, in MM/DD/YYYY format.
*
* Availability for Expedia Collect properties can be
* searched up to 500 days in advance.
*
* Hotel Collect/Hotel Collect availability can be searched
* up to 320 days in advance.
* @param departure check-out date, in MM/DD/YYYY format.
*
* Total length of stay cannot exceed 29 nights.
* @return a hotel list request builder
*/
public GetHotelListBuilder dates(final String arrival,
final String departure) {
request.setArrivalDate(arrival);
request.setDepartureDate(departure);
return self();
}
/**
* Maximum number of hotels to return. Acceptable value range is 1 to 200.
* Default: 20
*
* Does not limit results for a dateless list request.
*
* @param value maximum number of hotels to return
* @return a hotel list request builder
*/
public GetHotelListBuilder numberOfResults(final int value) {
request.setNumberOfResults(value);
return self();
}
/**
* @param adults adult guest count for the room.
*
* Expedia Collect properties typically accommodate no more
* than 4 guests before incurring extra person fees.
*
* Do not offer more than 8 guests per room, as results will
* not return above this guest count for most markets.
*
* Hotel Collect guest counts over 2 people are forwarded as
* direct requests to the hotel, which are not guaranteed.
* @param children always require this info from customers when child guests
* are specified.
*
* For example, to declare that two children ages 5 and 12,
* you would put 5,12.
* @return a hotel list request builder
*/
public GetHotelListBuilder room(final int adults, final int... children) {
final Room room = new Room();
room.setNumberOfAdults(adults);
room.setNumberOfChildren(children.length);
for (int child : children) {
room.getChildAges().add(child);
}
RoomGroup group = request.getRoomGroup();
if (group == null) {
group = new RoomGroup();
request.setRoomGroup(group);
}
group.getRoom().add(room);
return self();
}
/**
* Include additional hotel details.
*
* @param details determines whether or not a rate key,
* cancellation policies, bed types, and smoking
* preferences should be returned with each room
* option.
*
* Requesting these values at this level allows you
* to offer a two-step booking path directly from
* the list results.
*
* Returns additional elements only with
* minorRev=22 or above.
*
* V3 Turbo users: please note that including this
* parameter will prevent you from receiving a V3
* Turbo cached response. Only standard, uncached
* responses will be returned.
* @param hotelFeeBreakdown returns a more detailed response structure for
* the HotelFees array that includes how often each
* fee applies and how it is applied. Available
* with minorRev=24 and higher.
* @return a hotel list request builder
*/
public GetHotelListBuilder include(final boolean details,
final boolean hotelFeeBreakdown) {
request.setIncludeDetails(details);
request.setIncludeHotelFeeBreakdown(hotelFeeBreakdown);
return self();
}
/**
* City/state/country hotel search.
*
* @param city city to search within. Use only full city names.
* @param stateProvinceCode two character code for the state/province
* containing the specified city.
*
* References:
* * US State Codes
* * Canadian Province/Territory Codes
* * Australian Province/Territory Codes
* @param countryCode two character ISO-3166 code for the country
* containing the specified city. Use only country
* codes designated as "officially assigned" in
* the ISO-3166 decoding table.
* @return a hotel list request builder
*/
public GetHotelListBuilder search(final String city,
final String stateProvinceCode,
final String countryCode) {
request.setCity(city);
request.setStateProvinceCode(stateProvinceCode);
request.setCountryCode(countryCode);
return self();
}
/**
* Use a free text destination string.
*
* @param destination a string containing at least a city name. You can also
* send city and state, city and country,
* city/state/country, etc.
*
* This parameter is the best option for taking direct
* customer input.
*
* Ambiguous entries will return an error containing
* a list of likely intended locations, including their
* destinationId (see below) whenever possible.
* @return a hotel list request builder
*/
public GetHotelListBuilder searchByDestination(final String destination) {
request.setDestinationString(destination);
return self();
}
/**
* Use a destinationId.
*
* @param destinationId the unique hex key value for a specific city,
* metropolitan area, or landmark.
*
* Obtain this value via a geo function request, or
* from a multiple locations error returned by
* a destinationString availability request.
*
* Values for landmarks such as buildings, major
* neighborhoods, train stations, etc. can be obtained
* via a geo function request for landmarks. All
* available destinationIds can also be obtained via
* select files in our database catalog.
* @return a hotel list request builder
*/
public GetHotelListBuilder searchById(final String destinationId) {
request.setDestinationId(destinationId);
return self();
}
/**
* Use a list of hotelIds.
*
* @param hotelIdList check for availability against a fixed set of hotels.
*
* Send the hotelId values for the hotels you want to
* search for as a comma-separated list as a value for
* this element.
*
* For SOAP, send each individual value in a separate
* instance of hotelId.
*
* When using long lists, be aware that response times
* may increase noticably vs. smaller lists across
* multiple requests.
* @return a hotel list request builder
*/
public GetHotelListBuilder searchByHotelIdList(final long... hotelIdList) {
for (long hotelId : hotelIdList) {
request.getHotelIdList().add(hotelId);
}
return self();
}
/**
* Search within a geographical area.
*
* Note: you can also use this method for searching for airports in
* conjunction with the airport coordinates file.
*
* @param latitude latitude coordinate for the search's origin point, in
* DDD.MMmmm format.
* @param longitude longitude coordinate for the search's origin point, in
* DDD.MMmmm format.
* @param radius defines size of a square area to be searched within -
* not an actual radius of a circle. The value is still
* treated as a radius in the sense that it is half the
* width of the search area.
* Factor in the added area and maximum distances this
* creates vs a circular search area when exposing this
* value directly.
* Minimum of 1 MI or 2 KM, maximum of 50 MI or 80 KM.
* Defaults to 20 MI.
* @param unit sets the unit of distance for the search radius. Send
* MI or KM. Defaults to MI if empty or not included.
* @param sort you must send a value of proximity if you want the
* results to be sorted by distance from the origin point.
* Otherwise the default sort order is applied to any
* hotels that fall within the search radius.
* See the full definition of this element in the next
* section for all available values.
* @return a hotel list request builder
*/
public GetHotelListBuilder searchByArea(final float latitude,
final float longitude,
final int radius,
final SearchRadiusUnitType unit,
final SortType sort) {
request.setLatitude(latitude);
request.setLongitude(longitude);
request.setSearchRadius(radius);
request.setSearchRadiusUnit(unit);
request.setSort(sort);
return self();
}
@Override
protected HotelListResponse response() {
return getHotelServices().getList(request());
}
@Override
protected HotelListRequest request() {
return request;
}
@Override
protected GetHotelListBuilder self() {
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy