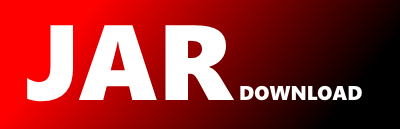
com.geeoz.ean.dsl.GetLocationInfoBuilder Maven / Gradle / Ivy
/*
* Copyright 2013 Geeoz Software
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.geeoz.ean.dsl;
import com.ean.wsapi.hotel.v3.LocationInfoRequest;
import com.ean.wsapi.hotel.v3.LocationInfoResponse;
/**
* Obtain location data such as a specific destinationId, latitude/longitude
* coordinates, and the number of active properties available within
* the location. You can also acquire a list of searchable landmark names
* within a location, including museums, schools, sports arenas,
* neighborhoods, and for some cities, transit stations.
*
* @author Eugene Shevchenko
* @version 1.0 7/13/2013
*/
public final class GetLocationInfoBuilder
extends AbstractHotelBuilder {
/**
* Obtain location data such as a specific destinationId, latitude/longitude
* coordinates, and the number of active properties available within
* the location.
*/
private final LocationInfoRequest request;
/**
* An empty constructor with request initialization.
*/
public GetLocationInfoBuilder() {
request = new LocationInfoRequest();
baseHotelInit(request);
}
@Override
public LocationInfoResponse response() {
return getHotelServices().getGeoLocation(request());
}
@Override
protected LocationInfoRequest request() {
return request;
}
@Override
protected GetLocationInfoBuilder self() {
return this;
}
/**
* Determines the type of destination to return. Use in conjunction with
* destinationString.
*
* @param type omission defaults the parameter to 1, or
* city locations, but sending with a value of 2,
* or landmarks, will return destinationIds for
* useful in-city locations such as famous
* buildings, museums, and even transit stations
* for major cities such asLondon and New York.
* @param destinationString A string containing at least a city name.
* You can also send city and state, city and
* country, city/state/country, etc. Some
* requests for locations in Germany and the
* UK may erroneously return results in the
* US or globally - send country codes of DEU
* and UK rather than DE or GB, respectively,
* to avoid these issues.
* @return a location info request builder
*/
public GetLocationInfoBuilder type(final String type,
final String destinationString) {
request().setType(type);
request().setDestinationString(destinationString);
return self();
}
/**
* Set the unique hex key value for a specific city,
* metropolitan area, or landmark.
*
* @param destinationId unique hex key value. Use a value returned
* in an earlier geo functions request, or
* from the landmark or destination ID files
* in the special content section of our
* database catalog.
* @return a location info request builder
*/
public GetLocationInfoBuilder destinationId(final String destinationId) {
request().setDestinationId(destinationId);
return self();
}
/**
* Opportunity to return all possible destinations rather than the
* highest-weighted popular destination.
*
* @param ignoreSearchWeight For example, there are four cities named
* London in our databases, but a simple
* destinationString search for "London" will
* only return the UK location since it is the
* most popular candidate. Setting this parameter
* to true will return the high-weighted UK
* location as well as info for the cities of
* London in Ontario, Kentucky, and Ohio.
* Omit this parameter if this behavior
* is not needed for your request.
* @return a location info request builder
*/
public GetLocationInfoBuilder ignoreSearchWeight(
final boolean ignoreSearchWeight) {
request().setIgnoreSearchWeight(ignoreSearchWeight);
return self();
}
/**
* Opportunity to additional mapping resources in an attempt to return
* more searchable locations.
*
* @param useGeoCoder setting this parameter to true will call upon
* additional mapping resources in attempt to
* return more searchable locations than the
* standard mapping system. Using this parameter
* may result in "empty" locations without any
* active properties. In these situations, the
* locations will not include a destinationId.
* Omit these locations from any future requests.
* @return a location info request builder
*/
public GetLocationInfoBuilder useGeoCoder(final boolean useGeoCoder) {
request().setUseGeocoder(useGeoCoder);
return self();
}
/**
* Search by address specific information.
*
* @param postalCode destination postal code. If used, must be
* sent with city, stateProvinceCode,
* and countryCode.
* @param city specify a city to search for. Must also
* include stateProvinceCode and countryCode.
* @param stateProvinceCode Specify the state to search within. For US,
* CA, and AU only. Must also include city
* and countryCode.
*
* References:
* * US State Codes
* * Canadian Province/Territory Codes
* * Australian Province/Territory Codes
* @param countryCode Two character ISO-3166 code for the country
* containing the specified city. Use only
* country codes designated as "officially
* assigned" in the ISO-3166 decoding table.
* Must also include city and stateProvinceCode.
* @param address Enter an address to search for
* latitude/longitude coordinates and valid
* nearby hotel location(s). Currently works
* for US addresses only. Must also include
* city and countryCode.
* @return a location info request builder
*/
public GetLocationInfoBuilder address(final String postalCode,
final String city,
final String stateProvinceCode,
final String countryCode,
final String address) {
request().setPostalCode(postalCode);
request().setCity(city);
request().setStateProvinceCode(stateProvinceCode);
request().setCountryCode(countryCode);
request().setAddress(address);
return self();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy